
- •Acknowledgments
- •Preface
- •Who Should Read This Book
- •What’s in This Book
- •Arduino Uno and the Arduino Platform
- •Code Examples and Conventions
- •Online Resources
- •The Parts You Need
- •Starter Packs
- •Complete Parts List
- •1. Welcome to the Arduino
- •What You Need
- •What Exactly Is an Arduino?
- •Exploring the Arduino Board
- •Installing the Arduino IDE
- •Meeting the Arduino IDE
- •Hello, World!
- •Compiling and Uploading Programs
- •What If It Doesn’t Work?
- •Exercises
- •2. Creating Bigger Projects with the Arduino
- •What You Need
- •Managing Projects and Sketches
- •Changing Preferences
- •Using Serial Ports
- •What If It Doesn’t Work?
- •Exercises
- •3. Building Binary Dice
- •What You Need
- •Working with Breadboards
- •Using an LED on a Breadboard
- •First Version of a Binary Die
- •Working with Buttons
- •Adding Your Own Button
- •Building a Dice Game
- •What If It Doesn’t Work?
- •Exercises
- •4. Building a Morse Code Generator Library
- •What You Need
- •Learning the Basics of Morse Code
- •Building a Morse Code Generator
- •Fleshing Out the Morse Code Generator’s Interface
- •Outputting Morse Code Symbols
- •Installing and Using the Telegraph Class
- •Publishing Your Own Library
- •What If It Doesn’t Work?
- •Exercises
- •5. Sensing the World Around Us
- •What You Need
- •Measuring Distances with an Ultrasonic Sensor
- •Increasing Precision Using Floating-Point Numbers
- •Increasing Precision Using a Temperature Sensor
- •Creating Your Own Dashboard
- •What If It Doesn’t Work?
- •Exercises
- •What You Need
- •Wiring Up the Accelerometer
- •Bringing Your Accelerometer to Life
- •Finding and Polishing Edge Values
- •Building Your Own Game Controller
- •More Projects
- •What If It Doesn’t Work?
- •Exercises
- •7. Writing a Game for the Motion-Sensing Game Controller
- •Writing a GameController Class
- •Creating the Game
- •What If It Doesn’t Work?
- •Exercises
- •8. Generating Video Signals with an Arduino
- •What You Need
- •How Analog Video Works
- •Building a Digital-to-Analog Converter (DAC)
- •Connecting the Arduino to Your TV Set
- •Using the TVout Library
- •Building a TV Thermometer
- •Working with Graphics in TVout
- •What If It Doesn’t Work?
- •Exercises
- •9. Tinkering with the Wii Nunchuk
- •What You Need
- •Wiring a Wii Nunchuk
- •Talking to a Nunchuk
- •Building a Nunchuk Class
- •Using Our Nunchuk Class
- •Creating Your Own Video Game Console
- •Creating Your Own Video Game
- •What If It Doesn’t Work?
- •Exercises
- •10. Networking with Arduino
- •What You Need
- •Using Your PC to Transfer Sensor Data to the Internet
- •Registering an Application with Twitter
- •Tweeting Messages with Processing
- •Communicating Over Networks Using an Ethernet Shield
- •Using DHCP and DNS
- •What If It Doesn’t Work?
- •Exercises
- •11. Creating a Burglar Alarm with Email Notification
- •What You Need
- •Emailing from the Command Line
- •Emailing Directly from an Arduino
- •Detecting Motion Using a Passive Infrared Sensor
- •Bringing It All Together
- •What If It Doesn’t Work?
- •Exercises
- •What You Need
- •Understanding Infrared Remote Controls
- •Grabbing Remote Control Codes
- •Cloning a Remote
- •Controlling Infrared Devices Remotely with Your Browser
- •Building an Infrared Proxy
- •What If It Doesn’t Work?
- •Exercises
- •13. Controlling Motors with Arduino
- •What You Need
- •Introducing Motors
- •First Steps with a Servo Motor
- •Building a Blaminatr
- •What If It Doesn’t Work?
- •Exercises
- •Current, Voltage, and Resistance
- •Electrical Circuits
- •Learning How to Use a Wire Cutter
- •Learning How to Solder
- •Learning How to Desolder
- •The Arduino Programming Language
- •Bit Operations
- •Learning More About Serial Communication
- •Serial Communication Using Various Languages
- •What Are Google Chrome Apps?
- •Creating a Minimal Chrome App
- •Starting the Chrome App
- •Exploring the Chrome Serial API
- •Writing a SerialDevice Class
- •Index
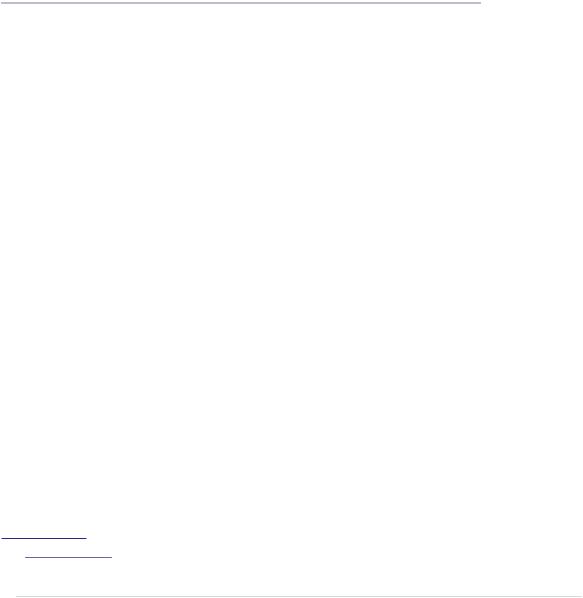
APPENDIX 4
Controlling the Arduino with a Browser
For many hardware projects, you’ll need an application on your computer that visualizes some sensor data or that controls your device. In the Arduino scene, many people use Processing1 for this purpose. Processing is a good choice. It’s fast, it has excellent multimedia support, and it supports a lot of libraries because it uses the Java Virtual Machine (JVM).
Processing has some disadvantages, too. It is very similar to Java; that is, it’s a statically typed programming language. As such, it isn’t a good tool for building prototypes interactively and incrementally. Also, you have to install Java and the Processing environment to use it.
In many cases it’s a better choice to use a regular web browser to write applications that communicate with the Arduino. Web browsers have excellent multimedia support, too, and the JavaScript programming language is easy to learn and widely available.
The only problem is that most web browsers don’t support serial port programming. But fortunately, Google Chrome comes with native support for serial port programming. Due to security restrictions, you can access the corresponding library only in Chrome Web apps and not on regular websites. Fortunately, it’s not difficult to create Chrome apps, and in this appendix you’ll learn how.
What Are Google Chrome Apps?
Over the years, web browsers have evolved from simple applications used for rendering HTML documents to full-blown programming environments. They’ve become so powerful that you can barely distinguish them from operating
1.http://processing.org
report erratum • discuss
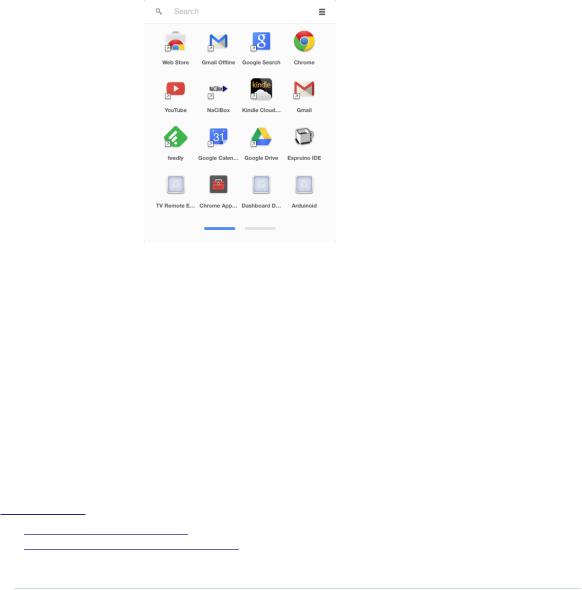
Appendix 4. Controlling the Arduino with a Browser • 268
systems today. Chrome OS,2 for example, is an operating system that was designed for executing web applications.
Very early on, Google realized that applications made using standard web technologies such as HTML5, CSS3, and JavaScript could also work well on the desktop. So they invented Google Chrome apps, a technology for using web technologies to create desktop applications.
Google operates a web shop for Chrome apps.3 Also, the company has released an application launcher that allows you to start Chrome apps without starting the browser.
Unlike regular websites, you can use many Chrome apps when you’re offline. You can even find complete office suites that were implemented as Chrome apps.
In contrast to native applications, web applications and JavaScript programs are often limited in a number of ways. Usually that’s a good thing, because it prevents malicious websites from compromising your computer. Still, there are situations where a bit more freedom would be advantageous—for example, if you’d like to access serial devices from your browser.
Chrome apps address exactly this issue and provide APIs to access serial, USB, or Bluetooth devices. They also come with a mature permission management, so users can decide whether a certain application should get access to a particular resource.
2.http://en.wikipedia.org/wiki/Chrome_OS
3.https://chrome.google.com/webstore/category/apps
report erratum • discuss
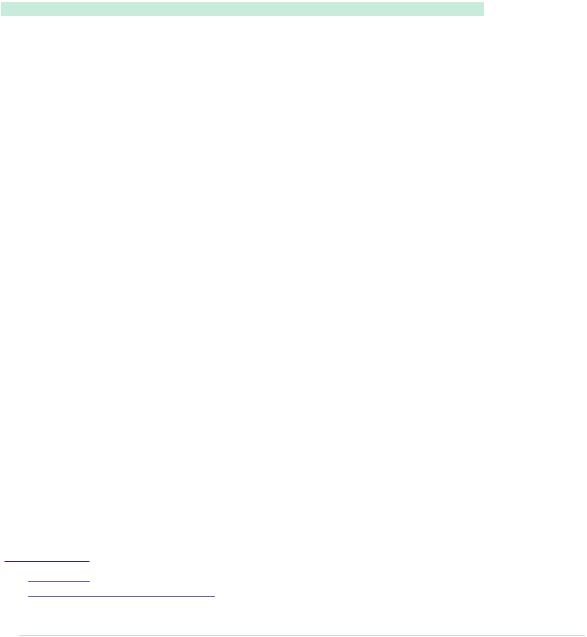
Creating a Minimal Chrome App • 269
Creating a Minimal Chrome App
Chrome apps are zip files that contain all the assets you’d usually expect in a web application. They contain HTML files, JavaScript files, images, videos, and so on. In addition, each zip archive contains two special files: manifest.json and background.js.
manifest.json describes the Chrome app’s metadata and typically looks like this:
ChromeApps/SimpleApp/manifest.json
{
"manifest_version": 2, "name": "My First Chrome App", "version": "1", "permissions": ["serial"], "app": {
"background": {
"scripts": ["background.js"]
}
}
}
It uses JavaScript Object Notation (JSON)4 and supports a lot of different options.5
Only name and version are mandatory. They specify the app’s name and version. These attributes will appear in the Chrome Web Store, if you decide to make your application available to the public. So choose them carefully if you’re going to release your application.
manifest_version contains the version of the manifest file’s specification. Currently, you must set it to 2 for Chrome apps.
With the permissions option, your Chrome app can request permissions that applications do not have by default. We set it to serial so the Chrome app is allowed to access the computer’s serial port. Users who install a Chrome app have to agree to all permissions it requests.
Eventually, manifest.json sets the Chrome app’s background page to a file named background.js. Which brings us to the second mandatory file in each Chrome app: background.js.
Not only does every Chrome app need a starting point, but every app also needs some kind of lifecycle management. Like operating systems control a
4.http://json.org/
5.https://developer.chrome.com/apps/manifest
report erratum • discuss
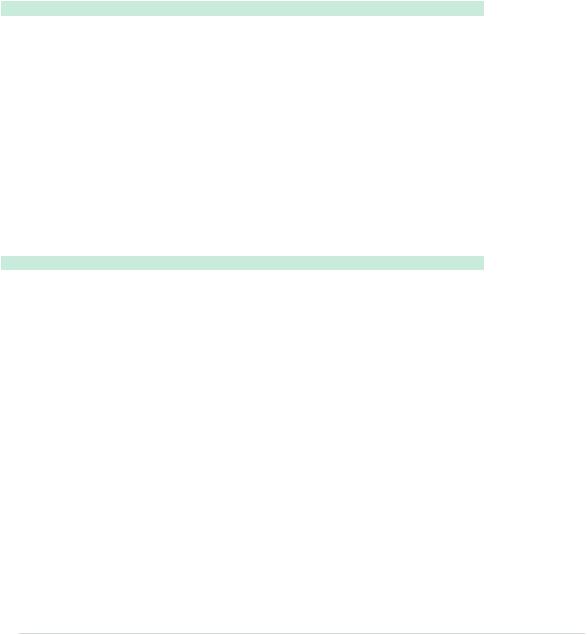
Appendix 4. Controlling the Arduino with a Browser • 270
native application’s lifecycle, the Chrome environment controls a web application’s lifecycle. It provides the web applications with important events, such as alarms or signals.
The background.js file is where you connect your Chrome app to the Chrome runtime environment. You can register for many events, but you at least have to specify what happens when your application gets launched. Here’s how you can do that:
ChromeApps/SimpleApp/background.js chrome.app.runtime.onLaunched.addListener(function() {
chrome.app.window.create('main.html', { id: 'main',
bounds: { width: 200, height: 100 } });
});
The preceding file adds a new listener function to Chrome’s runtime environment. Specifically, it registers a listener function for the onLaunched event—that is, the function will be called when the Chrome app gets launched.
The listener opens a new Chrome app window by calling the chrome.app.window.create function. This function expects the name of an HTML document to be opened and some optional arguments, such as the window’s ID and its bounds. For our first Chrome app, the HTML document looks as follows:
ChromeApps/SimpleApp/main.html
<!DOCTYPE html>
<html>
<head>
<title>My First Chrome App</title>
</head>
<body>
<p>Hello, world!</p>
</body>
</html>
These three files (manifest.json, background.js, and main.html) are all you need for a basic Chrome app. In the next section, you’ll learn how to run the application for the first time.
Starting the Chrome App
During development, it’d be tedious to create zip archives every time you wanted to try your latest changes to a Chrome app. That’s why the Chrome browser supports the execution of unzipped applications. Point the browser
report erratum • discuss