
Tree part 1
.pdf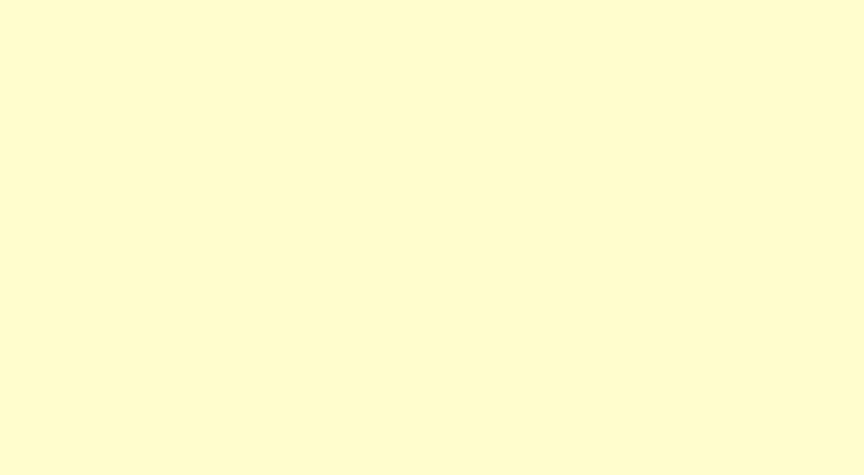
Tree Joint Right
TREE *TreeJointRight(TREE *a, TREE *b) { TREE *p;
if(!a) return b; if(!b) return a; p=TreeMin(b);
p->parent=0; // delete old p->left=a; p->right=b; return p;
} // TreeJointRight
31
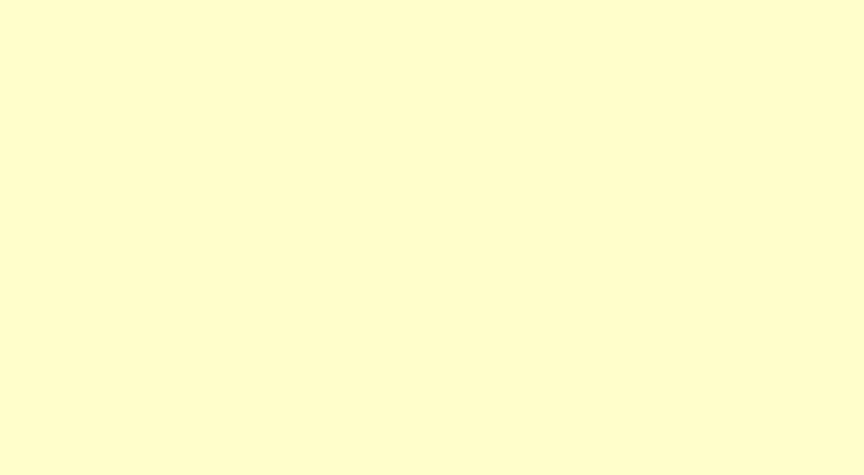
Tree Joint Left
TREE *TreeJointLeft(TREE *a, TREE *b) { TREE *p;
if(!a) return b; if(!b) return a; p=TreeMax(a); if(!(p->key<b->key) ) return 0; p->parent=0; // delete old p->left=a; p->right=b;
return p;
} // TreeJointLeft
32
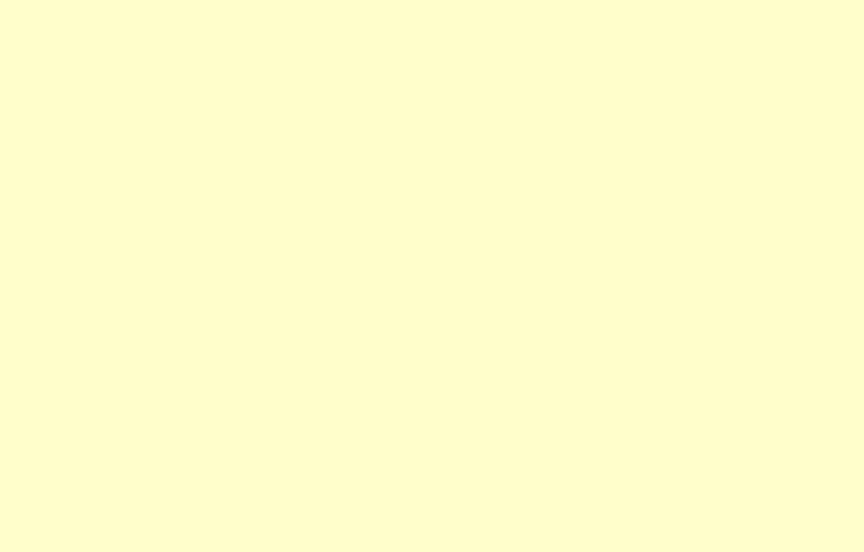
Node Remove
TREE *NodeRemove(TREE *&tree, int key)
{TREE *p,*q,*a,*b; if(!r) return tree;
p=TreeSearchIter(tree, key); a=p->left; b=p->right; q=TreeJointLeft(a, b);
// q=TreeJointRight(a, b); p->parent=q;
return q;
} // NodeRemove
33
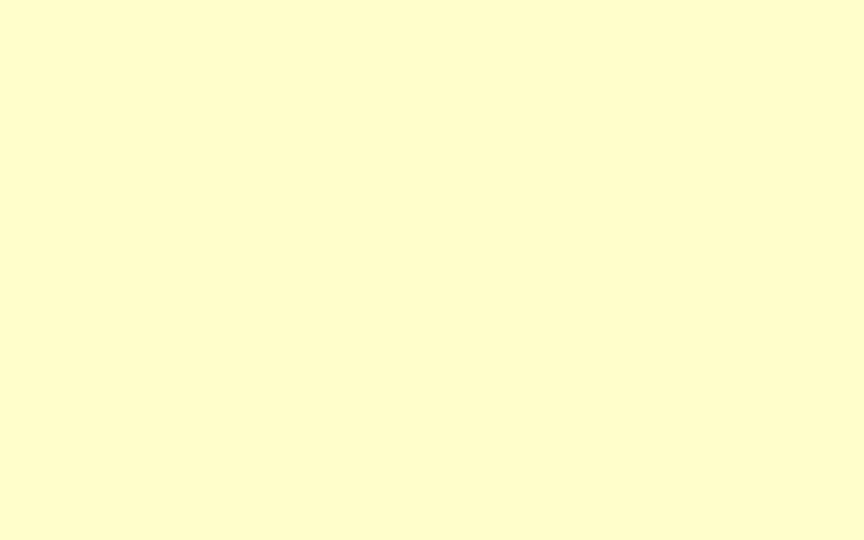
Tree Remove
TREE *TreeRemove(TREE *& tree) { if(!tree) return 0;
TraversePost(r, freep); return tree;
} // TreeRemove
int freep(TREE *p) { free(p);
return 1; } //
34
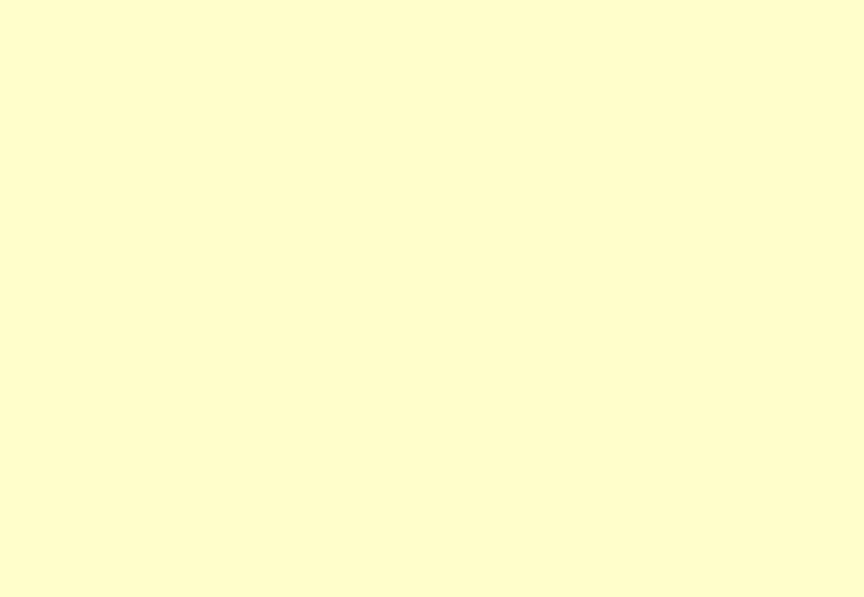
Create Random Tree
TREE *CreateRandTree(TREE *&tree, int n, int m) { int i; TREE *p;
for(i=0; i<n; i++)
{p=(TREE *) malloc( sizeof(TREE)); p->key=rand()%m;
p->left=0; p->right=0;
if(i==0) p->parent=0; TreeInsert (tree, p);
}
return tree;
}// CreateRandTree
35
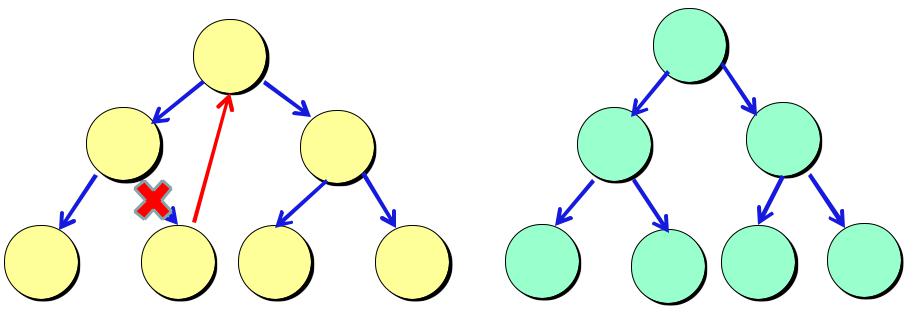
Rotation BST Right
|
r |
|
a |
a |
b |
c |
r |
c |
d |
e |
f |
d |
b |
TREE *RotateRight (TREE *&root) |
|
|
|||
{ TREE *a=root->left; |
|
|
|
||
root->left=a->right; |
|
|
|
||
a->right=root; |
|
|
|
|
|
root=a; |
|
// RotateRight |
|
|
|
return root; } |
|
36 |
|||
|
|
|
|
|
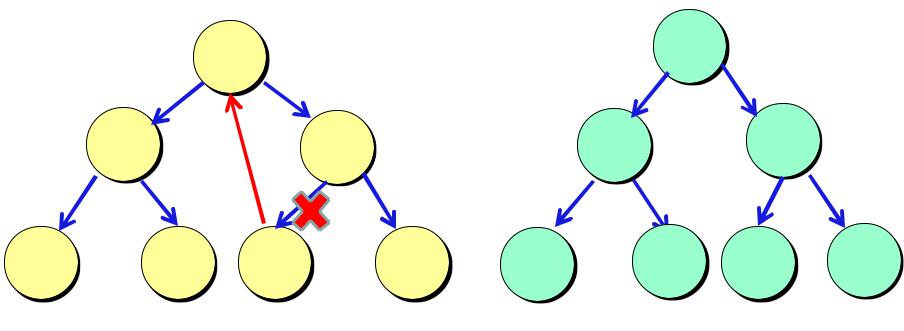
Rotation BST Left
|
r |
|
b |
a |
b |
r |
f |
c |
d |
e |
f |
a |
e |
TREE *RotateLeft(TREE *&root) |
|
|
|||
{ TREE *b=root-> right; |
|
|
|
||
root-> right =b->left; |
|
|
|
||
b->left=root; |
|
|
|
|
|
root=b; |
|
// RotateLeft |
|
|
|
return root; } |
|
|
37 |
||
|
|
|
|
|
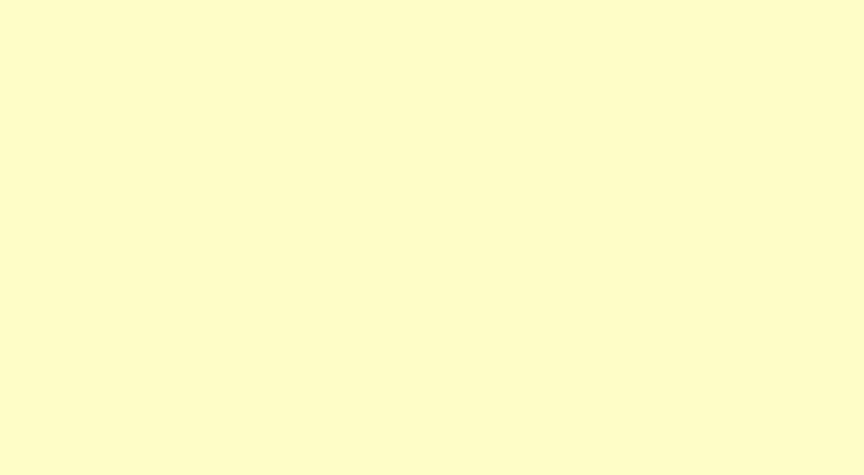
Insert to Root
TREE *InsertRoot(TREE *&root, TREE *q)
//q->parent=q->left=q->right=0
{if(!root) { root=q; return root; } if(q->key < root->key)
{InsertRoot(root->left, q); RotateRight(root);} else
{InsertRoot(root->right, q); RotateLeft(root);} return root;
}// InsertRoot
38
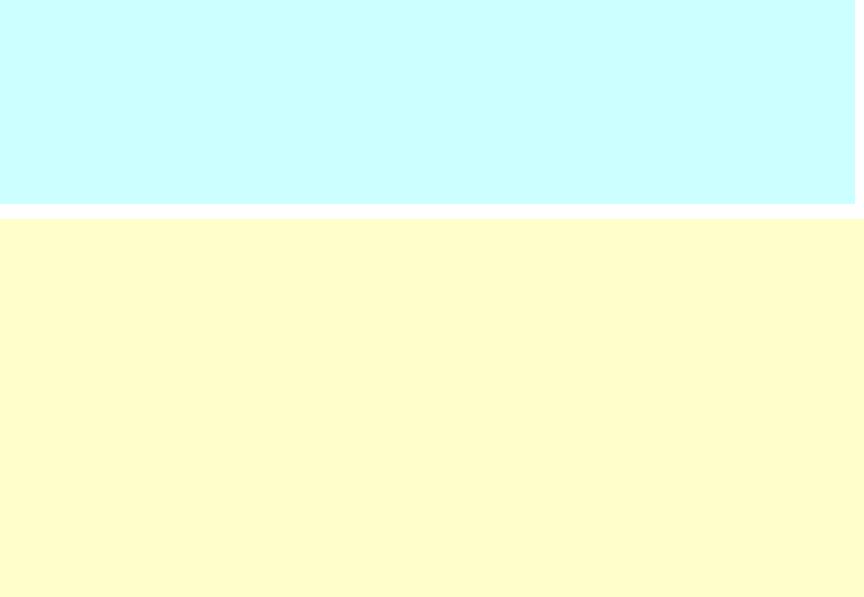
BST Joint
Два BST-дерева объединяются путем выбора (произвольного) корня первого дерева в качестве результирующего корня, вставки этого корня в корень второго дерева, а затем (рекурсивного) объединения пары левых поддеревьев и пары правых поддеревьев.
TREE * TreeJoint(TREE *a, TREE *b)
{ |
if(!a) return b; |
if(!b) return a; |
|
InsertRoot (b, a); |
|
|
b->left = TreeJoint(a->left, b->left); |
|
|
b->right = TreeJoint(a->right, b->right); |
|
|
TreeRemove (a); |
|
|
return b; |
|
} |
// TreeJoint |
39 |
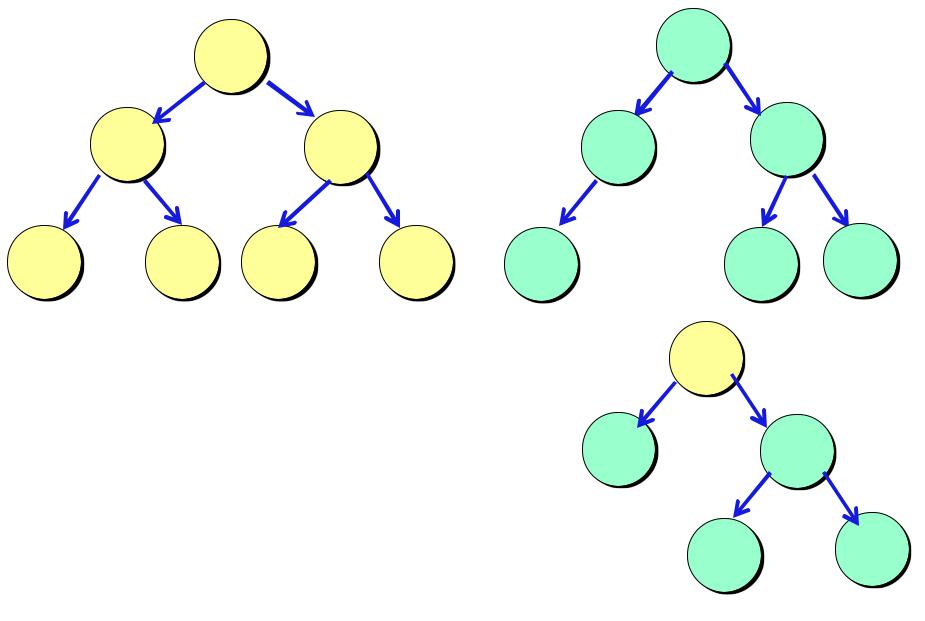
BST Joint
|
a |
|
b |
c |
d |
i |
j |
e f j h k |
m n |
a
<a
Left pair: c & k Right pair: d & b
k b
i |
40j |