
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
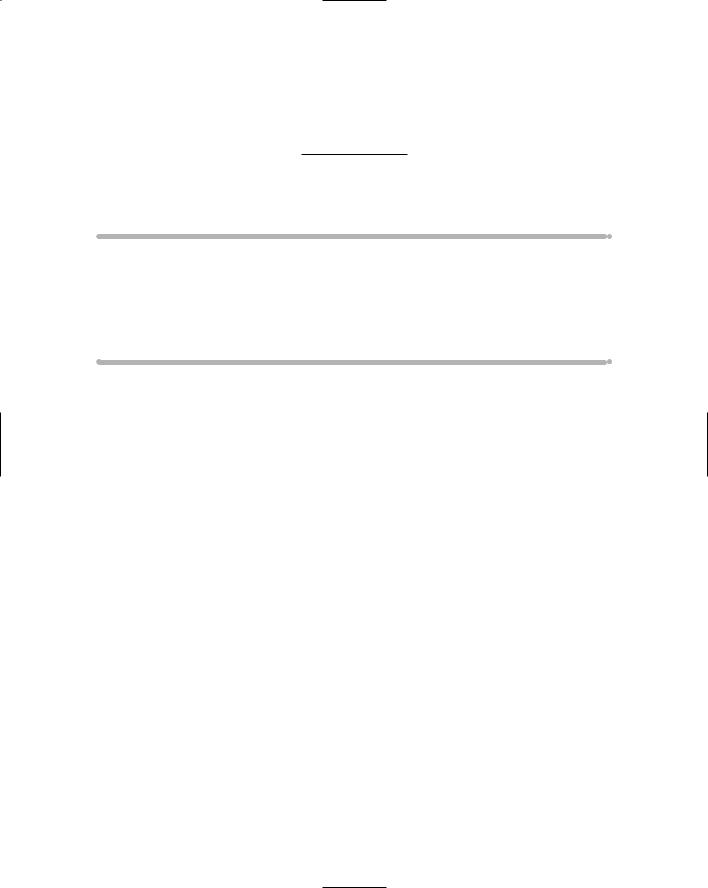
Chapter 17
C You in a While Loop
In This Chapter
Using a while loop
Choosing between for and while
Making infinite while loops
Beating a dead horse
When it comes time to create a loop, the C language gives you a choice. You can go with the complex for loop, which has all the gizmos and
options to make most programmers happy, or you can choose the more exotic, free-wheeling while loop for your programs. Whereas for is more official and lays out all its plans in one spot, while is fanciful and free — like those care free days of youth when Mommy would kiss your boo-boos and make them better and Daddy paid for everything.
This chapter introduces you to the happy-go-lucky while loop. Looping is a concept you should already be familiar with if you have been toiling with for loops for the past few chapters. But, I have good news! while loops are a heck of a lot simpler to understand. Ha-ha! Now, I tell you. . . .
The Lowdown on while Loops
While loops shouldn’t be strange to you. Consider the following:
While the light is red, keep your foot on the brake.
This simple example shows a while loop in real life. It means, roughly, “While this thing is true, keep repeating this action” (that is, a loop). Your foot is stepping on the brake as long as the light is red — a loop. Easy enough.
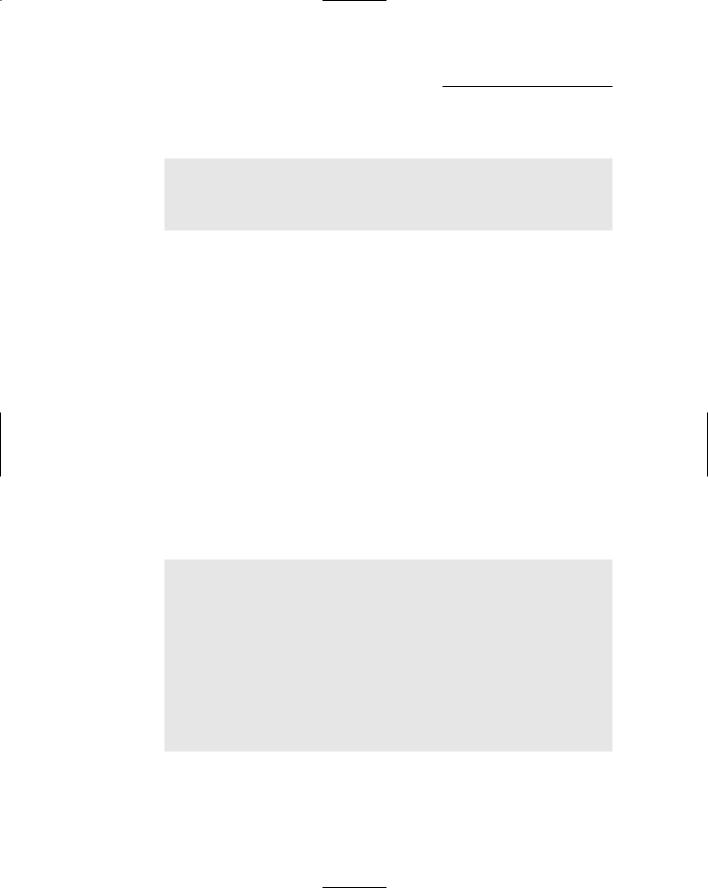
216 Part III: Giving Your Programs the Ability to Run Amok
If you want to rewrite this instruction by using a C-like syntax and a while loop, it could look like this:
while(light==RED)
{
foot_on_brake(); light = check_light();
}
light==RED is a condition that can be either TRUE or FALSE — as in an if statement. While that condition is TRUE, the statements held in the while loop’s curly braces are repeated.
One of the statements in the while loop checks the condition the loop repeats on: light=check_light() updates the status of the light variable. When it changes to something not RED, the while statement becomes FALSE, the block of statements is skipped, and the next part of the program is run. This is the essence of a while loop in C.
Whiling away the hours
As in a for loop, you can set up a while loop to repeat a chunk of statements a given number of times. Unlike a for loop, the while loop’s controls (the doo jabbies that tell the loop when to start and where to finish) are blasted all over the place. This is good because it doesn’t mean that everything is crammed into one line, as with a for loop. This is bad because, well, I get into that in the next section. For now, busy yourself by typing the source code for HEY.C, a bril liant C program shown right next:
#include <stdio.h>
int main()
{
int i;
i=1;
while(i<6)
{
printf(“Ouch! Please stop!\n”); i++;
}
return(0);
}
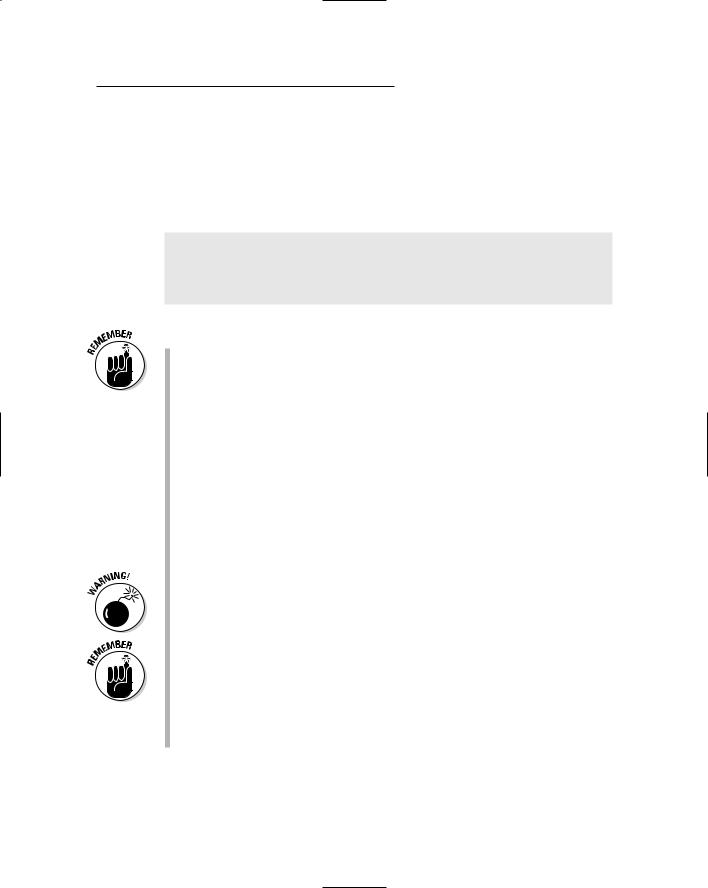
Chapter 17: C You in a While Loop 217
Type this program, which is essentially an update of the OUCH.C program, from Chapter 15. Both programs do the same thing, in fact, but by using dif ferent types of loops. Save the file to disk as HEY.C.
Compile. Run.
Here’s what the output looks like:
Ouch! Please stop!
Ouch! Please stop!
Ouch! Please stop!
Ouch! Please stop!
Ouch! Please stop!
Brilliant. Simply brilliant.
A loop in the C language requires three things: a start, a middle part (the part that is repeated), and an end. This information is from Chapter 15.
With a for loop, the looping-control information is found right in the for command’s parentheses.
With a while loop, the looping-control information is found before the loop, in the while command’s parentheses, and inside the while loop itself.
In HEY.C, the variable i is set up (or initialized) before the loop. Then, it’s incremented inside the loop. The while statement itself cares only when i is less than 6.
The statements belonging to the while — those lines clutched by the curly braces — are repeated only as long as the condition in parentheses is TRUE. If the condition is FALSE (suppose that the variable i was already greater than 6), those statements are skipped (as in an if statement).
While loops are easy targets for the infinite-loop boo-boo. For this reason, you have to check extra hard to make sure that the condition that while tests for eventually becomes FALSE, which makes the loop stop repeating.
For a while loop to quit, something must happen inside the loop that changes the condition that while examines.
This is probably the first time you have seen two different programs tackle the same problem: OUCH.C uses a for loop, and HEY.C uses a while loop. Neither one is better than the other. See the section “Deciding between a while loop and a for loop,” later in this chapter, for more information.
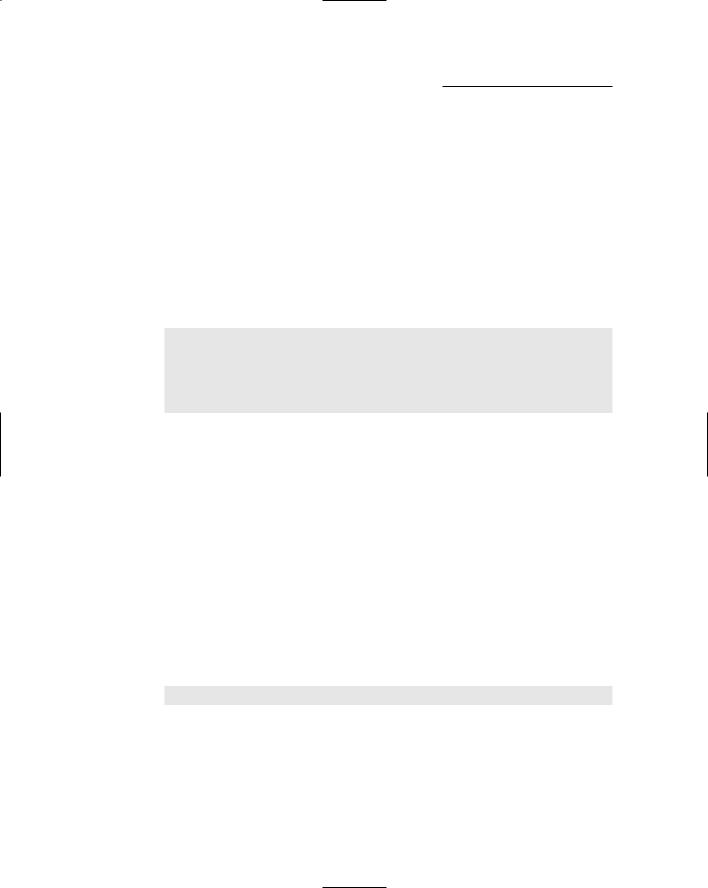
218 Part III: Giving Your Programs the Ability to Run Amok
The while keyword
(a formal introduction)
The while keyword is used in the C language to repeat a block of statements. Unlike the for loop, while only tells the computer when to end the loop. The loop must be set up before the while keyword, and when it’s looping, the ending condition — the sizzling fuse or ticking timer — must be working. Then, the loop goes on, la-de-da, until the condition that while monitors suddenly becomes FALSE. Then, the party’s over, and the program goes on, sadder but content with the fact that it was repeating itself for a while (sic).
Here’s the rough format:
starting; while(while_true)
{
statement(s); do_this;
}
First, the loop must be set up, which is done with the starting statement. For example, this statement (or a group of statements) may declare a variable to be a certain value, to wait for a keystroke, or to do any number of interesting things.
while_true is a condition that while examines. If the condition is TRUE, the statements enclosed in curly braces are repeated. while examines that condi tion after each loop is repeated, and only when the statement is FALSE does the loop stop.
Inside the curly braces are statements repeated by the while loop. One of those statements, do_this, is required in order to control the loop. The do_this part needs to modify the while_true condition somehow so that the loop eventually stops or is broken out of.
While loops have an advantage over for loops in that they’re easier to read in English. For example:
while(ch!=’~’)
This statement says “While the value of variable ch does not equal the tilde character, repeat the following statements.” For this to make sense, you must remember, of course, that ! means not in C. Knowing which sym
bols to pronounce and which are just decorations is important to understand ing C programming.