
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
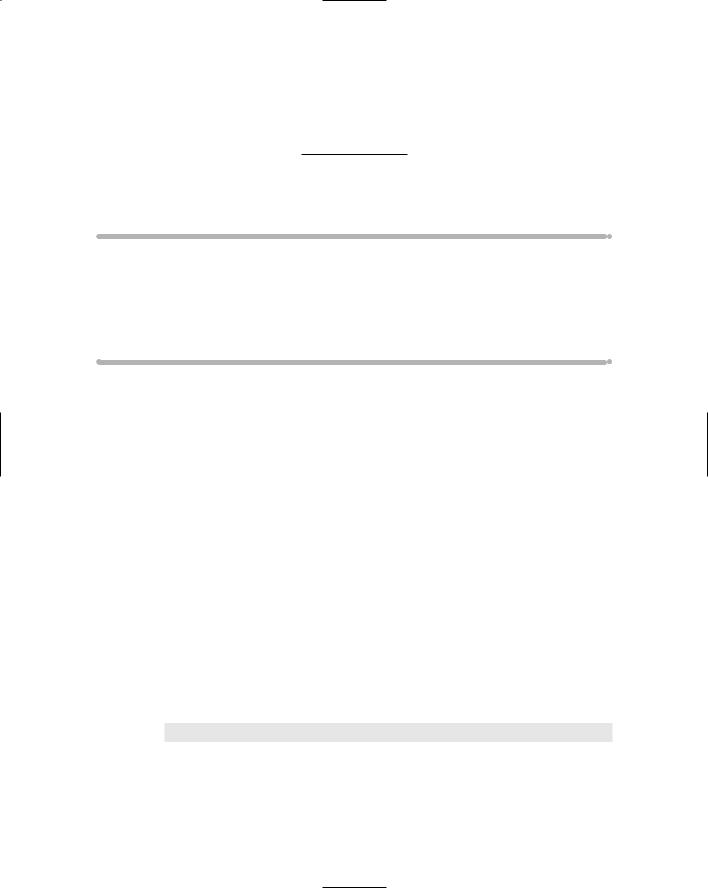
Chapter 13
What If C==C?
In This Chapter
Comparing characters with if
Understanding standard input
Fixing the flaws of getchar()
Making a yes-or-no decision
Apologies are in order for the preceding chapter. Yes, I definitely drift into Number Land while introducing the virtues of the if command. Computer
programs aren’t all about numbers, and the judgment that the if keyword makes isn’t limited to comparing dollar amounts, ecliptic orbits, subatomic particle masses, or calories in various fudgy cookie snacks. No, you can also compare letters of the alphabet in an if statement. That should finally answer the mystery of whether the T is “greater than” the S and why the dollar sign is less than the minus sign. This chapter explains the details.
The World of if without Values
I ask you: How can one compare two letters of the alphabet? Truly, this sub ject is right up there with “How many angels can dance on the head of a pin?” and “Would it be a traditional dance, gavotte, rock, or perhaps heavenly hokey-pokey?”
Yet, comparing letters is a necessary task. If you write a menu program, you have to see whether the user selects option A, B, or C. That’s done by using the handy if keyword. For example:
if(key==’A’)
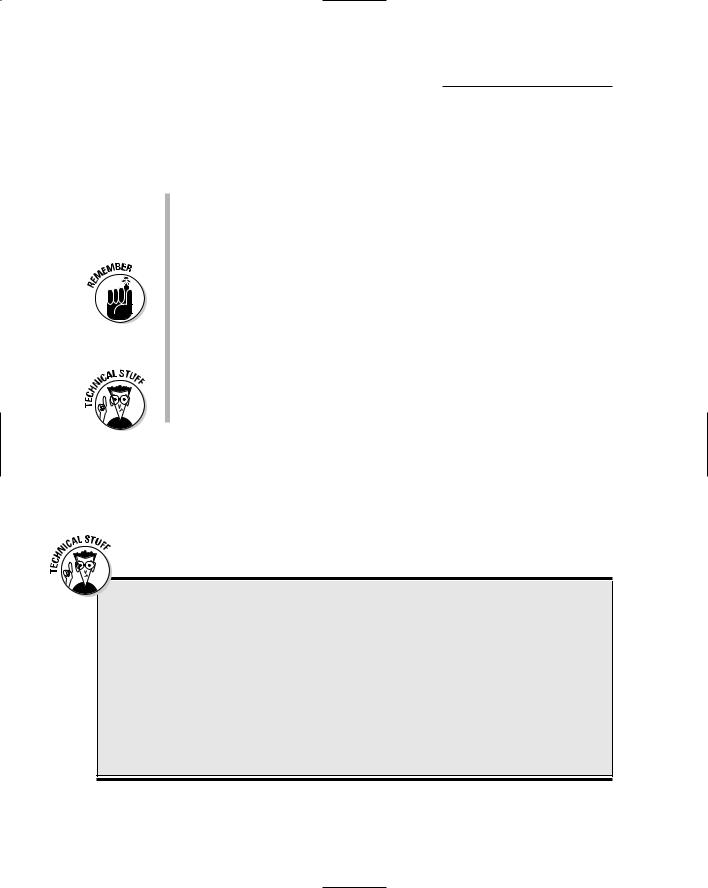
166 Part III: Giving Your Programs the Ability to Run Amok
Here, key is a single-character variable holding input from the keyboard. The comparison that if makes is to see whether the content of that variable is equal to (two equal signs) the letter A, which is enclosed in single quotes. This comparison is legitimate. Did the user type an A?
To compare a single-character variable with a character — letter, number, or symbol — you must enclose that character in single quotes. Single character, single quotes.
When if tests to see whether two things are equal, two equal signs are used. Think “is equal to” rather than “equals.”
When you compare a variable — numeric or single character — to a con stant, the variable always comes first in the comparison.
On a good day, 4.9E3 angels can dance on the head of a pin, given the rela tive size of the pin and the size of the angels and whether they’re dancing all at once or taking turns.
When you compare two letters or numbers, what you’re ‘really compar
ing are their ASCII code values. This is one of those weird instances when the single-character variable acts more like an integer than a letter, num ber, or symbol.
Which is greater: S or T, $ or –?
Is the T greater than the S? Alphabetically speaking, yes. T comes after S. But, what about the dollar sign and the minus sign? Which of those is greater? And, why should Q be lesser than U, because everyone knows that U always follows Q? Hmmm.
Computers and math (do I even have to remind you to skip this stuff?)
Sad to say, too much about computers does deal with math. The computer evolved from the cal culator, which has its roots in the abacus, which is somehow related to human fingers and thumbs. After all, working with numbers is called computing, which comes from the ancient Latin term computare. That word literally means “hire
a few more accountants and we’ll never truly know what’s going on.”
Another word for compute is reckon, which is popular in the South as “I reck’n.” Another word for compute is figure, which is popular in the !South (not-South) as “I figure.” Computers reckon. Go figure.
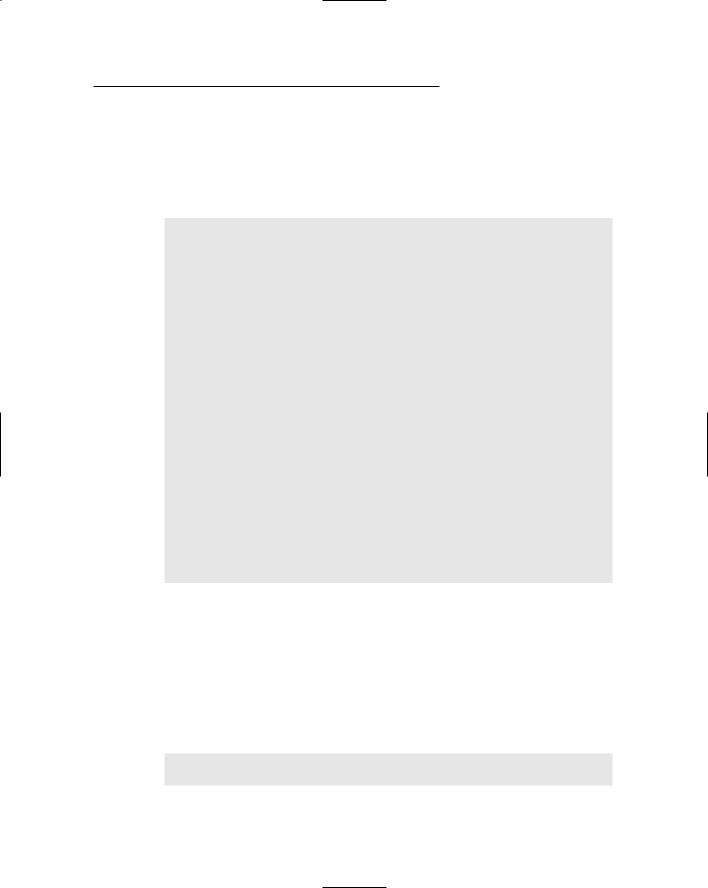
Chapter 13: What If C==C? 167
To solve this great mystery of life, I list next the source code for a program, GREATER.C. It asks you to enter two single characters. These characters are compared by an if statement as well as by if-else. The greater of the two is then displayed. Although this program doesn’t lay to rest the angels-on-the- head-of-a-pin problem, it soothes your frayed nerves over some minor alpha betic conundrums:
#include <stdio.h>
int main()
{
char a,b;
printf(“Which character is greater?\n”); printf(“Type a single character:”); a=getchar();
printf(“Type another character:”); b=getchar();
if(a > b)
{
printf(“‘%c’ is greater than ‘%c’!\n”,a,b);
}
else if (b > a)
{
printf(“‘%c’ is greater than ‘%c’!\n”,b,a);
}
else
{
printf(“Next time, don’t type the same character twice.”);
}
return(0);
}
Enter the source code for GREATER.C into your editor. Make sure that you enter all the proper curly braces, confirm the locations of semicolons, watch your double quotes, and pay attention to the other minor nuances (or nui sances) of the C language.
Save the file to disk as GREATER.C.
Compile GREATER.C.
Run the final program. You’re asked this question:
Which character is greater?
Type a single character:
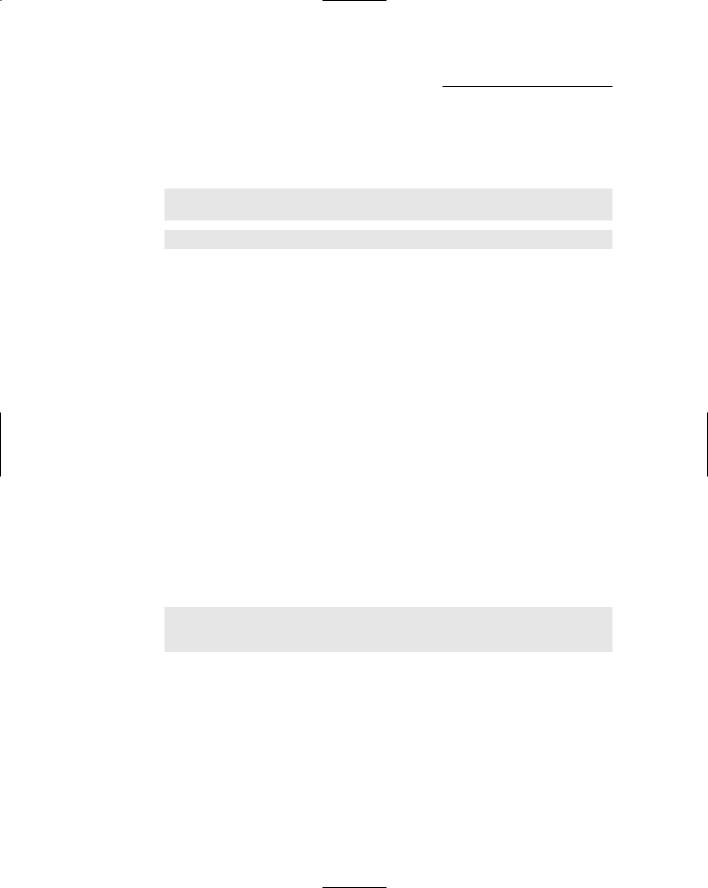
168 Part III: Giving Your Programs the Ability to Run Amok
Type a character, such as the $ (dollar sign). Press Enter after typing the character.
Uh-oh! What happened? You see something like this:
Type another character:’$’ is greater than ‘
‘$
What? What? What?
You bet! A problem. Right in the middle of the chapter that talks about compar ing single-character variables, I have to totally pick up the cat and talk about something else vital in C programming: properly reading single characters from the keyboard.
The problem with getchar()
Despite what it says in the brochure, getchar() does not read a single char acter from the keyboard. That’s what the getchar() function returns, but it’s not what the function does.
Internally, getchar() reads what’s called standard input. It grabs the first char acter you type and stores it, but afterward it sits and waits for you to type something that signals “the end.”
Two characters signal the end of input: The Enter key is one. The second is the EOF, or end-of-file, character. In Windows, it’s the Ctrl+Z character. In the Unix-like operating systems, it’s Ctrl+D.
Run the GREATER program, from the preceding section. When it asks for input, type 123 and then press the Enter key. Here’s what you see for output:
Which character is greater?
Type a single character:123
Type another character:’2’ is greater than ‘1’
When you’re first asked to type a single character, you provide standard input for the program: the string 123 plus the press of the Enter key.
The getchar() function reads standard input. First, it reads the 1 and places it in the variable a (Line 9 in GREATER.C). Then, the program uses a second getchar() function to read again from standard input — but it has already been supplied; 2 is read into the variable b (refer to Line 11).
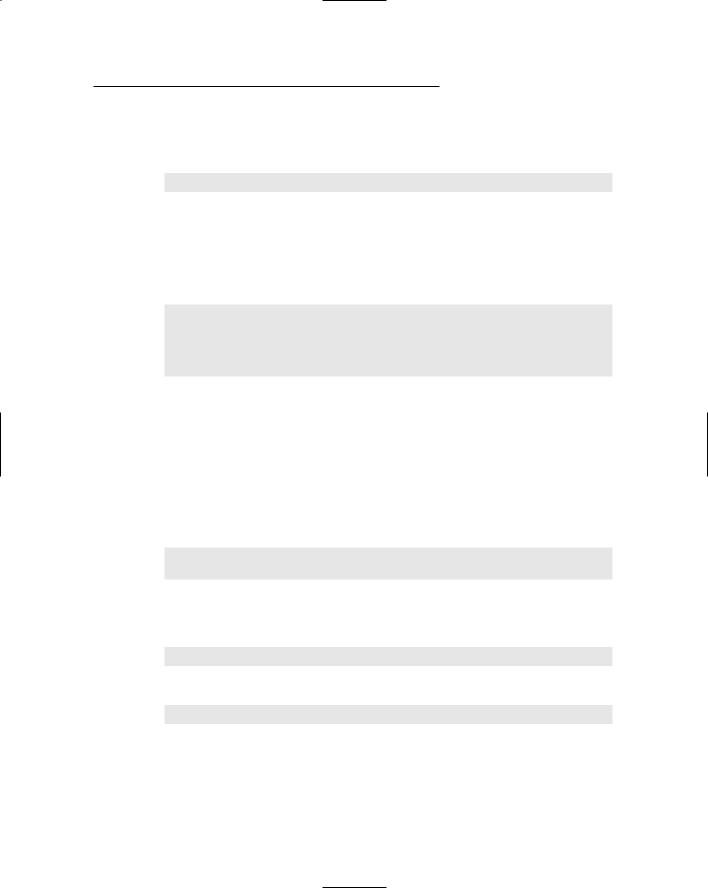
Chapter 13: What If C==C? 169
The 3? It’s ignored; there’s no place to store it. But, the Enter key press signi fies to the program that standard input was entered and the program contin ued, immediately displaying the result, all on the final line:
Type another character:’2’ is greater than ‘1’!
You may think that one way around this problem is to terminate standard input by pressing the Enter key right after entering the first character. After all, Enter ends standard input. But, it doesn’t work that way.
Run the GREATER program again — this time, entering $ and pressing the Enter key at the first prompt. Here’s the output:
Which character is greater?
Type a single character:$
Type another character:’$’ is greater than ‘ ‘!
This matches what you probably saw the first time you entered the program. See the Enter key displayed? It’s what separates the ‘ at the end of the third line and the ‘ at the beginning of the fourth line.
In this case, the Enter key itself is being accepted as standard input. After all, Enter is a key on the keyboard and a proper character code, just like anything else. That rules out Enter as a proper way to end standard input. That leaves you with the EOF character.
Run the GREATER program one more time:
Which character is greater?
Type a single character:
In Windows, type $ and then press Ctrl+Z and Enter.
In Unix, type $ and then pressCtrl+D.
Type another character:
Then you can type 2 and press Enter:
‘2’ is greater than ‘$’!
The program finally runs properly, but only after you brute-force the end of standard input so that getchar() can properly process it. Obviously, explaining something like that to the users who run your programs would be bothersome. You have a better solution. Keep reading.
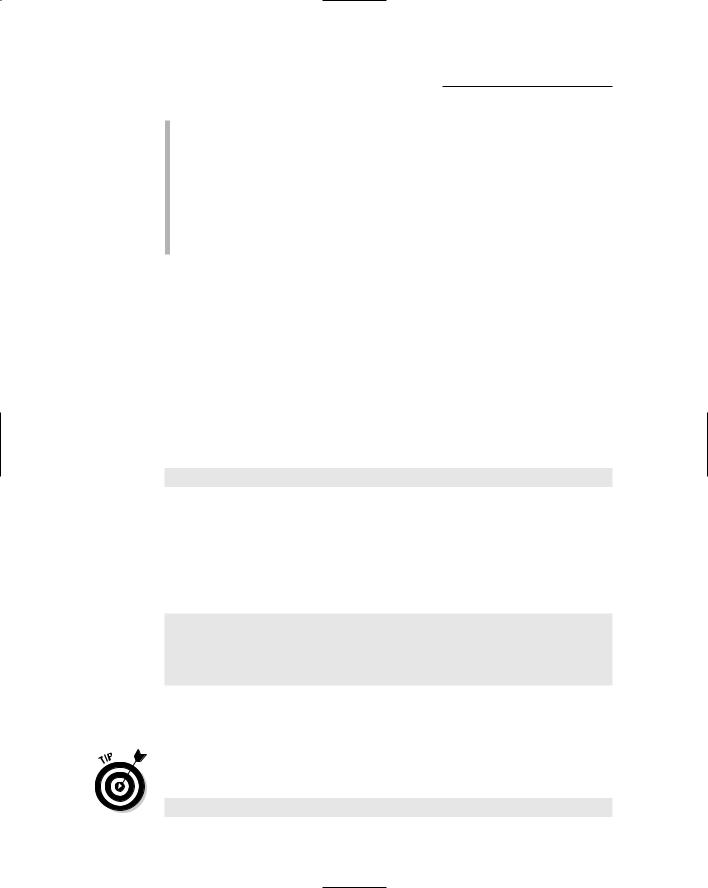
170 Part III: Giving Your Programs the Ability to Run Amok
In Windows, the end-of-file (EOF) character is Ctrl+Z. That’s ASCII code 27.
In Unix (Linux, FreeBSD, Mac OS, and so on), the end-of-file (EOF) char acter is Ctrl+D. That’s ASCII code 4.
To properly enter Ctrl+Z in Windows, you must press Ctrl+Z and then the Enter key. In Unix, the Ctrl+D combo works instantly.
Be careful when you press Ctrl+D at your Unix command prompt! In many instances, Ctrl+D is also the key that terminates the shell, logging you off.
Fixing GREATER.C to easily read standard input
The easy way to fix GREATER.C is to ensure that all standard input is cleared out — purged or flushed — before the second getchar() function reads it. That way, old characters hanging around aren’t mistakenly read.
The C language function fflush() is used to clear out information waiting to be written to a file. Because C treats standard input as a type of file, you can use fflush() to clear out standard input. Here’s the format:
fflush(stdin);
You can insert this statement into any program at any point where you want to ensure that no leftover detritus can accidentally be read from the keyboard. It’s a handy statement to know.
To fix the GREATER.C source code, you need to stick the fflush() function in after the first getchar() function — at Line 10. This code snippet shows you how the fixed-up source code should look:
printf(“Type a single character:”); a=getchar();
fflush(stdin);
printf(“Type another character:”); b=getchar();
Add the new Line 10, as shown here, to your source code for GREATER.C. Save the changes to disk. Compile and run the result. The program now properly runs — and you can continue reading about comparing characters with the if command.
If using fflush(stdin) doesn’t work, replace it with this function:
fpurge(stdin);