
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index

Chapter 8: Charting Unknown Cs with Variables |
95 |
Declare your variables near the beginning of your program, just after the line with the initial curly brace. Cluster them all up right there.
Obviously, you don’t know all the variables a program requires before you write it. (Though they teach otherwise at the universities, such mental overhead isn’t required by you and me.) If you need a new vari able, use your editor to declare it in the program. Rogue variables — those undeclared — generate syntax or linker errors (depending on how they’re used).
If you don’t declare a variable, your program doesn’t compile. A suitable complaint message is issued by the proper authorities.
Most C programmers put a blank line between the variable declarations and the rest of the program.
There’s nothing wrong with commenting a variable to describe what it contains. For example:
int count; /* busy signals from tech support. */
However, cleverly named variables can avoid this situation:
int busysignals;
Or, even better:
int busy_signal_count;
Variable names verboten and not
What you can name your variables depends on your compiler. You have to follow a few rules, and you cannot use certain names for variables. When you break the rules, the compiler lets you know by flinging an error message your way. To avoid that, try to keep the following guidelines in the back of your head when you create new variables:
The shortest variable name is a letter of the alphabet.
Use variable names that mean something. Single-letter variables are just hunky-dory. But index is better than i, count is better than c, and name is better than n. Short, descriptive variable names are best.
Variables are typically in lowercase. (All of C is lowercase, for the most part.) They can contain letters and numbers.
Uppercase letters can be used in your variables, but most compilers tend to ignore the differences between upperand lowercase letters. (You can tell the compiler to be case-sensitive by setting one of its options; refer to your compiler’s online help system for the details.)

96 |
Part II: Run and Scream from Variables and Math |
You shouldn’t begin a variable name with a number. It can contain num bers, but you begin it with a letter. Even if your compiler says that it’s okay, other C compilers don’t, so you should not begin a variable name with a letter.
C lords use the underline, or underscore, character in their variable names: first_name and zip_code, for example. This technique is fine, though it’s not recommended to begin a variable name with an under line.
Avoid naming your variables the same as C language keywords or func
tions. Don’t name your integer variable int, for example, or your string variable char. This may not generate an error with your compiler, but it makes your source code confusing. (Refer to Table 3-1, in Chapter 3, for a list of the C language keywords.)
Also avoid using the single letters l (lowercase L) and o (lowercase O) to name variables. Little L looks too much like a 1 (one), and O looks too much like a 0 (zero).
Don’t give similar names to your variables. For example, the compiler may assume that forgiveme and forgivemenot are the same variable. If so, an ugly situation can occur.
Buried somewhere in the cryptic help files that came with your compiler are the official rules for naming variables. These rules are unique to each compiler, which is why I’m not mentioning them all here. After all, I’m not paid by the hour. And it’s not part of my contract.
Presetting variable values
Suppose that this guy named Methuselah is 969 years old. I understand that this may be a new, bold concept to grasp, but work with me here.
If you were going to use Methuselah’s age as a value in a program, you could create the variable methus and then shove the value 969 into it. That requires two steps. First comes the declaration:
int methus;
This line tells the compiler that methus is capable of holding an integer-size value in its mouth and all that. Then comes the assignment, when 969 is put into the variable methus:
methus=969;

Chapter 8: Charting Unknown Cs with Variables |
97 |
In C, you can combine both steps into one. For example:
int methus=969;
This statement creates the integer variable methus and assigns it the value 969 — all at once. It’s your first peek at C language shortcut. (C is full of short cuts and alternatives — enough to make you kooky.)
You can do the same thing with string variables — but it’s a little weird. Normally, string variables are created and given a size. For example:
char prompt[22];
Here, a character string variable, prompt, is created and given room for 22 characters. Then you use gets() or scanf() to stick text into that variable. (You don’t use an equal sign!) When you create the variable and assign it a string, however, it’s given this format:
char prompt[] = “So how fat are you, anyway?”
This command creates a string variable, prompt. That string variable already contains the text “So how fat are you, anyway?” Notice that you see no number in the brackets. The reason is that the compiler is smart enough to figure out how long the string is and use that value automatically. No guesswork — what joy!
Numeric variables can be assigned a value when they’re declared. Just follow the variable name with an equal sign and its value. Remember to end the line with a semicolon.
You can even assign the variable a value concocted by using math. For example:
int video=800*600;
This statement creates the integer variable video and sets its value equal to 800 times 600, or 480,000. (Remember that * is used for multi plication in C.)
Even though a variable may be assigned a value, that value can still change. If you create the integer variable methus and assign it the value 969, there’s nothing wrong with changing that value later in the program. After all, a variable is still a variable.
Here’s a trick that’s also possible, but not necessary, to remember: int start = begin = first = count = 0;
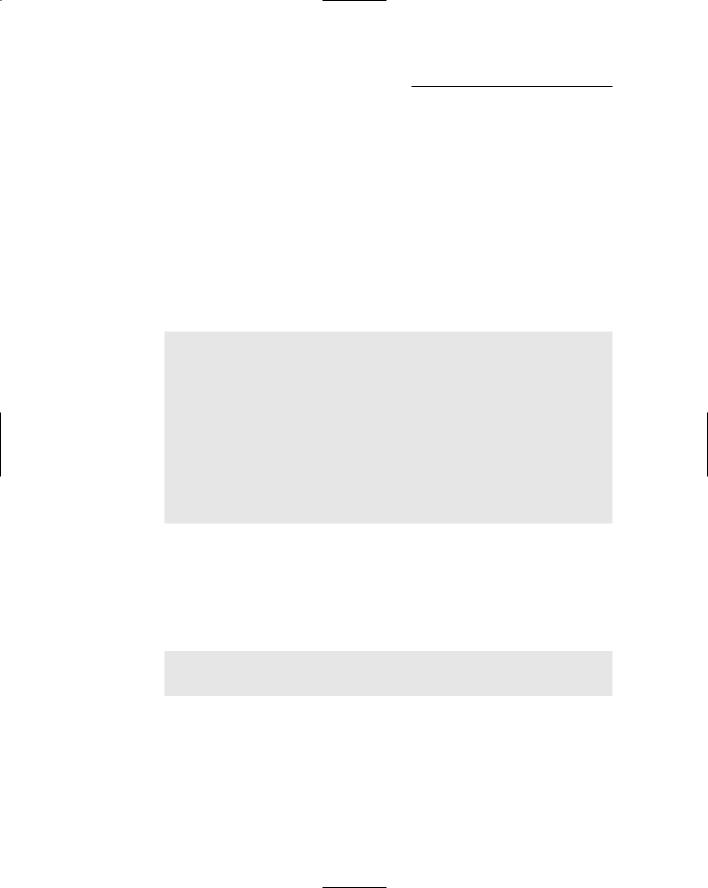
98 |
Part II: Run and Scream from Variables and Math |
This statement declares four integer variables: start, begin, first, and count. Each of them is set equal to 0. start is equal to begin, which is equal to first, which is equal to count, which is equal to 0. You probably see this type of declaration used more often than you end up using it yourself.
The old random-sampler variable program
To demonstrate how variables can be defined with specific values, the ICKYGU.C program was concocted. It works like those old Chinese all-you- can-eat places, where steaming trays of yummy glop lie waiting under greasesmeared panes of sneeze-protecting glass. Ah . . . reminds me of my college days and that bowel infection I had. Here’s the source code:
#include <stdio.h>
int main()
{
char menuitem[] = “Slimy Orange Stuff \”Icky Woka Gu\””;
int pints=1;
float price = 1.45;
printf(“Today special - %s\n”,menuitem); printf(“You want %d pint.\n”,pints); printf(“That be $%f, please.\n”,price); return(0);
}
Type this source code into your editor. Double-check everything. Save the program as ICKYGU.C.
Compile the program. Repair any unexpected errors — as well as those you may have been expecting — and recompile if need be.
Run the final program. You see something like the following example displayed:
Today special - Slimy Orange Stuff “Icky Woka Gu”
You want 1 pint.
That be $1.450000, please.
Whoa! Is that lira or dollars? Of course, it’s dollars — the dollar sign in printf()’s formatting string is a normal character, not anything special. But the 1.45 value was printed with four extra zeroes. Why? Because you didn’t tell the compiler not to. That’s just the way the %f, or floating-point conver sion character, displays numbers.