
- •Contents
- •Introduction
- •Who This Book Is For
- •What This Book Covers
- •How This Book Is Structured
- •What You Need to Use This Book
- •Conventions
- •Source Code
- •Errata
- •p2p.wrox.com
- •The Basics of C++
- •The Obligatory Hello, World
- •Namespaces
- •Variables
- •Operators
- •Types
- •Conditionals
- •Loops
- •Arrays
- •Functions
- •Those Are the Basics
- •Diving Deeper into C++
- •Pointers and Dynamic Memory
- •Strings in C++
- •References
- •Exceptions
- •The Many Uses of const
- •C++ as an Object-Oriented Language
- •Declaring a Class
- •Your First Useful C++ Program
- •An Employee Records System
- •The Employee Class
- •The Database Class
- •The User Interface
- •Evaluating the Program
- •What Is Programming Design?
- •The Importance of Programming Design
- •Two Rules for C++ Design
- •Abstraction
- •Reuse
- •Designing a Chess Program
- •Requirements
- •Design Steps
- •An Object-Oriented View of the World
- •Am I Thinking Procedurally?
- •The Object-Oriented Philosophy
- •Living in a World of Objects
- •Object Relationships
- •Abstraction
- •Reusing Code
- •A Note on Terminology
- •Deciding Whether or Not to Reuse Code
- •Strategies for Reusing Code
- •Bundling Third-Party Applications
- •Open-Source Libraries
- •The C++ Standard Library
- •Designing with Patterns and Techniques
- •Design Techniques
- •Design Patterns
- •The Reuse Philosophy
- •How to Design Reusable Code
- •Use Abstraction
- •Structure Your Code for Optimal Reuse
- •Design Usable Interfaces
- •Reconciling Generality and Ease of Use
- •The Need for Process
- •Software Life-Cycle Models
- •The Stagewise and Waterfall Models
- •The Spiral Method
- •The Rational Unified Process
- •Software-Engineering Methodologies
- •Extreme Programming (XP)
- •Software Triage
- •Be Open to New Ideas
- •Bring New Ideas to the Table
- •Thinking Ahead
- •Keeping It Clear
- •Elements of Good Style
- •Documenting Your Code
- •Reasons to Write Comments
- •Commenting Styles
- •Comments in This Book
- •Decomposition
- •Decomposition through Refactoring
- •Decomposition by Design
- •Decomposition in This Book
- •Naming
- •Choosing a Good Name
- •Naming Conventions
- •Using Language Features with Style
- •Use Constants
- •Take Advantage of const Variables
- •Use References Instead of Pointers
- •Use Custom Exceptions
- •Formatting
- •The Curly Brace Alignment Debate
- •Coming to Blows over Spaces and Parentheses
- •Spaces and Tabs
- •Stylistic Challenges
- •Introducing the Spreadsheet Example
- •Writing Classes
- •Class Definitions
- •Defining Methods
- •Using Objects
- •Object Life Cycles
- •Object Creation
- •Object Destruction
- •Assigning to Objects
- •Distinguishing Copying from Assignment
- •The Spreadsheet Class
- •Freeing Memory with Destructors
- •Handling Copying and Assignment
- •Different Kinds of Data Members
- •Static Data Members
- •Const Data Members
- •Reference Data Members
- •Const Reference Data Members
- •More about Methods
- •Static Methods
- •Const Methods
- •Method Overloading
- •Default Parameters
- •Inline Methods
- •Nested Classes
- •Friends
- •Operator Overloading
- •Implementing Addition
- •Overloading Arithmetic Operators
- •Overloading Comparison Operators
- •Building Types with Operator Overloading
- •Pointers to Methods and Members
- •Building Abstract Classes
- •Using Interface and Implementation Classes
- •Building Classes with Inheritance
- •Extending Classes
- •Overriding Methods
- •Inheritance for Reuse
- •The WeatherPrediction Class
- •Adding Functionality in a Subclass
- •Replacing Functionality in a Subclass
- •Respect Your Parents
- •Parent Constructors
- •Parent Destructors
- •Referring to Parent Data
- •Casting Up and Down
- •Inheritance for Polymorphism
- •Return of the Spreadsheet
- •Designing the Polymorphic Spreadsheet Cell
- •The Spreadsheet Cell Base Class
- •The Individual Subclasses
- •Leveraging Polymorphism
- •Future Considerations
- •Multiple Inheritance
- •Inheriting from Multiple Classes
- •Naming Collisions and Ambiguous Base Classes
- •Interesting and Obscure Inheritance Issues
- •Special Cases in Overriding Methods
- •Copy Constructors and the Equals Operator
- •The Truth about Virtual
- •Runtime Type Facilities
- •Non-Public Inheritance
- •Virtual Base Classes
- •Class Templates
- •Writing a Class Template
- •How the Compiler Processes Templates
- •Distributing Template Code between Files
- •Template Parameters
- •Method Templates
- •Template Class Specialization
- •Subclassing Template Classes
- •Inheritance versus Specialization
- •Function Templates
- •Function Template Specialization
- •Function Template Overloading
- •Friend Function Templates of Class Templates
- •Advanced Templates
- •More about Template Parameters
- •Template Class Partial Specialization
- •Emulating Function Partial Specialization with Overloading
- •Template Recursion
- •References
- •Reference Variables
- •Reference Data Members
- •Reference Parameters
- •Reference Return Values
- •Deciding between References and Pointers
- •Keyword Confusion
- •The const Keyword
- •The static Keyword
- •Order of Initialization of Nonlocal Variables
- •Types and Casts
- •typedefs
- •Casts
- •Scope Resolution
- •Header Files
- •C Utilities
- •Variable-Length Argument Lists
- •Preprocessor Macros
- •How to Picture Memory
- •Allocation and Deallocation
- •Arrays
- •Working with Pointers
- •Array-Pointer Duality
- •Arrays Are Pointers!
- •Not All Pointers Are Arrays!
- •Dynamic Strings
- •C-Style Strings
- •String Literals
- •The C++ string Class
- •Pointer Arithmetic
- •Custom Memory Management
- •Garbage Collection
- •Object Pools
- •Function Pointers
- •Underallocating Strings
- •Memory Leaks
- •Double-Deleting and Invalid Pointers
- •Accessing Out-of-Bounds Memory
- •Using Streams
- •What Is a Stream, Anyway?
- •Stream Sources and Destinations
- •Output with Streams
- •Input with Streams
- •Input and Output with Objects
- •String Streams
- •File Streams
- •Jumping around with seek() and tell()
- •Linking Streams Together
- •Bidirectional I/O
- •Internationalization
- •Wide Characters
- •Non-Western Character Sets
- •Locales and Facets
- •Errors and Exceptions
- •What Are Exceptions, Anyway?
- •Why Exceptions in C++ Are a Good Thing
- •Why Exceptions in C++ Are a Bad Thing
- •Our Recommendation
- •Exception Mechanics
- •Throwing and Catching Exceptions
- •Exception Types
- •Throwing and Catching Multiple Exceptions
- •Uncaught Exceptions
- •Throw Lists
- •Exceptions and Polymorphism
- •The Standard Exception Hierarchy
- •Catching Exceptions in a Class Hierarchy
- •Writing Your Own Exception Classes
- •Stack Unwinding and Cleanup
- •Catch, Cleanup, and Rethrow
- •Use Smart Pointers
- •Common Error-Handling Issues
- •Memory Allocation Errors
- •Errors in Constructors
- •Errors in Destructors
- •Putting It All Together
- •Why Overload Operators?
- •Limitations to Operator Overloading
- •Choices in Operator Overloading
- •Summary of Overloadable Operators
- •Overloading the Arithmetic Operators
- •Overloading Unary Minus and Unary Plus
- •Overloading Increment and Decrement
- •Overloading the Subscripting Operator
- •Providing Read-Only Access with operator[]
- •Non-Integral Array Indices
- •Overloading the Function Call Operator
- •Overloading the Dereferencing Operators
- •Implementing operator*
- •Implementing operator->
- •What in the World Is operator->* ?
- •Writing Conversion Operators
- •Ambiguity Problems with Conversion Operators
- •Conversions for Boolean Expressions
- •How new and delete Really Work
- •Overloading operator new and operator delete
- •Overloading operator new and operator delete with Extra Parameters
- •Two Approaches to Efficiency
- •Two Kinds of Programs
- •Is C++ an Inefficient Language?
- •Language-Level Efficiency
- •Handle Objects Efficiently
- •Use Inline Methods and Functions
- •Design-Level Efficiency
- •Cache as Much as Possible
- •Use Object Pools
- •Use Thread Pools
- •Profiling
- •Profiling Example with gprof
- •Cross-Platform Development
- •Architecture Issues
- •Implementation Issues
- •Platform-Specific Features
- •Cross-Language Development
- •Mixing C and C++
- •Shifting Paradigms
- •Linking with C Code
- •Mixing Java and C++ with JNI
- •Mixing C++ with Perl and Shell Scripts
- •Mixing C++ with Assembly Code
- •Quality Control
- •Whose Responsibility Is Testing?
- •The Life Cycle of a Bug
- •Bug-Tracking Tools
- •Unit Testing
- •Approaches to Unit Testing
- •The Unit Testing Process
- •Unit Testing in Action
- •Higher-Level Testing
- •Integration Tests
- •System Tests
- •Regression Tests
- •Tips for Successful Testing
- •The Fundamental Law of Debugging
- •Bug Taxonomies
- •Avoiding Bugs
- •Planning for Bugs
- •Error Logging
- •Debug Traces
- •Asserts
- •Debugging Techniques
- •Reproducing Bugs
- •Debugging Reproducible Bugs
- •Debugging Nonreproducible Bugs
- •Debugging Memory Problems
- •Debugging Multithreaded Programs
- •Debugging Example: Article Citations
- •Lessons from the ArticleCitations Example
- •Requirements on Elements
- •Exceptions and Error Checking
- •Iterators
- •Sequential Containers
- •Vector
- •The vector<bool> Specialization
- •deque
- •list
- •Container Adapters
- •queue
- •priority_queue
- •stack
- •Associative Containers
- •The pair Utility Class
- •multimap
- •multiset
- •Other Containers
- •Arrays as STL Containers
- •Strings as STL Containers
- •Streams as STL Containers
- •bitset
- •The find() and find_if() Algorithms
- •The accumulate() Algorithms
- •Function Objects
- •Arithmetic Function Objects
- •Comparison Function Objects
- •Logical Function Objects
- •Function Object Adapters
- •Writing Your Own Function Objects
- •Algorithm Details
- •Utility Algorithms
- •Nonmodifying Algorithms
- •Modifying Algorithms
- •Sorting Algorithms
- •Set Algorithms
- •The Voter Registration Audit Problem Statement
- •The auditVoterRolls() Function
- •The getDuplicates() Function
- •The RemoveNames Functor
- •The NameInList Functor
- •Testing the auditVoterRolls() Function
- •Allocators
- •Iterator Adapters
- •Reverse Iterators
- •Stream Iterators
- •Insert Iterators
- •Extending the STL
- •Why Extend the STL?
- •Writing an STL Algorithm
- •Writing an STL Container
- •The Appeal of Distributed Computing
- •Distribution for Scalability
- •Distribution for Reliability
- •Distribution for Centrality
- •Distributed Content
- •Distributed versus Networked
- •Distributed Objects
- •Serialization and Marshalling
- •Remote Procedure Calls
- •CORBA
- •Interface Definition Language
- •Implementing the Class
- •Using the Objects
- •A Crash Course in XML
- •XML as a Distributed Object Technology
- •Generating and Parsing XML in C++
- •XML Validation
- •Building a Distributed Object with XML
- •SOAP (Simple Object Access Protocol)
- •. . . Write a Class
- •. . . Subclass an Existing Class
- •. . . Throw and Catch Exceptions
- •. . . Read from a File
- •. . . Write to a File
- •. . . Write a Template Class
- •There Must Be a Better Way
- •Smart Pointers with Reference Counting
- •Double Dispatch
- •Mix-In Classes
- •Object-Oriented Frameworks
- •Working with Frameworks
- •The Model-View-Controller Paradigm
- •The Singleton Pattern
- •Example: A Logging Mechanism
- •Implementation of a Singleton
- •Using a Singleton
- •Example: A Car Factory Simulation
- •Implementation of a Factory
- •Using a Factory
- •Other Uses of Factories
- •The Proxy Pattern
- •Example: Hiding Network Connectivity Issues
- •Implementation of a Proxy
- •Using a Proxy
- •The Adapter Pattern
- •Example: Adapting an XML Library
- •Implementation of an Adapter
- •Using an Adapter
- •The Decorator Pattern
- •Example: Defining Styles in Web Pages
- •Implementation of a Decorator
- •Using a Decorator
- •The Chain of Responsibility Pattern
- •Example: Event Handling
- •Implementation of a Chain of Responsibility
- •Using a Chain of Responsibility
- •Example: Event Handling
- •Implementation of an Observer
- •Using an Observer
- •Chapter 1: A Crash Course in C++
- •Chapter 3: Designing with Objects
- •Chapter 4: Designing with Libraries and Patterns
- •Chapter 5: Designing for Reuse
- •Chapter 7: Coding with Style
- •Chapters 8 and 9: Classes and Objects
- •Chapter 11: Writing Generic Code with Templates
- •Chapter 14: Demystifying C++ I/O
- •Chapter 15: Handling Errors
- •Chapter 16: Overloading C++ Operators
- •Chapter 17: Writing Efficient C++
- •Chapter 19: Becoming Adept at Testing
- •Chapter 20: Conquering Debugging
- •Chapter 24: Exploring Distributed Objects
- •Chapter 26: Applying Design Patterns
- •Beginning C++
- •General C++
- •I/O Streams
- •The C++ Standard Library
- •C++ Templates
- •Integrating C++ and Other Languages
- •Algorithms and Data Structures
- •Open-Source Software
- •Software-Engineering Methodology
- •Programming Style
- •Computer Architecture
- •Efficiency
- •Testing
- •Debugging
- •Distributed Objects
- •CORBA
- •XML and SOAP
- •Design Patterns
- •Index

Writing Generic Code with Templates
Class Templates
Class templates are useful primarily for containers, or data structures, that store objects. This section uses a running example of a Grid container. In order to keep the examples reasonable in length and simple enough to illustrate specific points, different sections of the chapter will add features to the Grid container that are not used in subsequent sections.
Writing a Class Template
Suppose that you want a generic game board class that you can use as a chessboard, checkers board, Tic- Tac-Toe board, or any other two-dimensional game board. In order to make it general-purpose, you should be able to store chess pieces, checkers pieces, Tic-Tac-Toe pieces, or any type of game piece.
Coding without Templates
Without templates, the best approach to build a generic game board is to employ polymorphism to store generic GamePiece objects. Then, you could subclass the pieces for each game from the GamePiece class. For example, in the chess game, the ChessPiece would be a subclass of GamePiece. Through polymorphism, the GameBoard, written to store GamePieces, can also store ChessPieces. Your class definition might look like similar to the Spreadsheet class from Chapter 9, which used a dynamically allocated two-dimensional array as the underlying grid structure:
// GameBoard.h
class GameBoard
{
public:
// The general-purpose GameBoard allows the user to specify its dimensions GameBoard(int inWidth = kDefaultWidth, int inHeight = kDefaultHeight); GameBoard(const GameBoard& src); // Copy constructor
~GameBoard();
GameBoard &operator=(const GameBoard& rhs); // Assignment operator
void setPieceAt(int x, int y, const GamePiece& inPiece); GamePiece& getPieceAt(int x, int y);
const GamePiece& getPieceAt(int x, int y) const;
int getHeight() const { return mHeight; } int getWidth() const { return mWidth; } static const int kDefaultWidth = 10; static const int kDefaultHeight = 10;
protected:
void copyFrom(const GameBoard& src);
// Objects dynamically allocate space for the game pieces. GamePiece** mCells;
int mWidth, mHeight;
};
getPieceAt() returns a reference to the piece at a specified spot instead of a copy of the piece. The GameBoard serves as an abstraction of a two-dimensional array, so it should provide array access semantics by giving the actual object at an index, not a copy of the object.
273

Chapter 11
This implementation of the class provides two versions of getPieceAt(), one of which returns a reference and one of which returns a const reference. Chapter 16 explains how this overload works.
Here are the method and static member definitions. The implementation is almost identical to the Spreadsheet class from Chapter 9. Production code would, of course, perform bounds checking in setPieceAt() and getPieceAt(). That code is omitted because it is not the point of this chapter.
// GameBoard.cpp #include “GameBoard.h”
const int GameBoard::kDefaultWidth; const int GameBoard::kDefaultHeight;
GameBoard::GameBoard(int inWidth, int inHeight) : mWidth(inWidth), mHeight(inHeight)
{
mCells = new GamePiece* [mWidth]; for (int i = 0; i < mWidth; i++) {
mCells[i] = new GamePiece[mHeight];
}
}
GameBoard::GameBoard(const GameBoard& src)
{
copyFrom(src);
}
GameBoard::~GameBoard()
{
// Free the old memory
for (int i = 0; i < mWidth; i++) { delete[] mCells[i];
}
delete[] mCells;
}
void GameBoard::copyFrom(const GameBoard& src)
{
int i, j;
mWidth = src.mWidth; mHeight = src.mHeight;
mCells = new GamePiece* [mWidth]; for (i = 0; i < mWidth; i++) {
mCells[i] = new GamePiece[mHeight];
}
for (i = 0; i < mWidth; i++) {
for (j = 0; j < mHeight; j++) { mCells[i][j] = src.mCells[i][j];
}
}
}
274

Writing Generic Code with Templates
GameBoard& GameBoard::operator=(const GameBoard& rhs)
{
//Check for self-assignment if (this == &rhs) {
return (*this);
}
//Free the old memory
for (int i = 0; i < mWidth; i++) { delete[] mCells[i];
}
delete[] mCells;
// Copy the new memory copyFrom(rhs);
return (*this);
}
void GameBoard::setPieceAt(int x, int y, const GamePiece& inElem)
{
mCells[x][y] = inElem;
}
GamePiece& GameBoard::getPieceAt(int x, int y)
{
return (mCells[x][y]);
}
const GamePiece& GameBoard::getPieceAt(int x, int y) const
{
return (mCells[x][y]);
}
This GameBoard class works pretty well. Assuming that you wrote a ChessPiece class, you can create GameBoard objects and use them like this:
GameBoard chessBoard(10, 10);
ChessPiece pawn;
chessBoard.setPieceAt(0, 0, pawn);
A Template Grid Class
The GameBoard class in the previous section is nice, but insufficient. For example, it’s quite similar to the Spreadsheet class from chapter 9, but the only way you could use it as a spreadsheet would be to make the SpreadsheetCell class a subclass of GamePiece. That doesn’t make sense because it doesn’t fulfill the is-a principle of inheritance: a SpreadsheetCell is not a GamePiece. It would be nice if you could write a generic grid class that you could use for purposes as diverse as a Spreadsheet or a ChessBoard. In C++, you can do this by writing a class template, which allows you to write a class without specifying one or more types. Clients then instantiate the template by specifying the types they want to use.
275
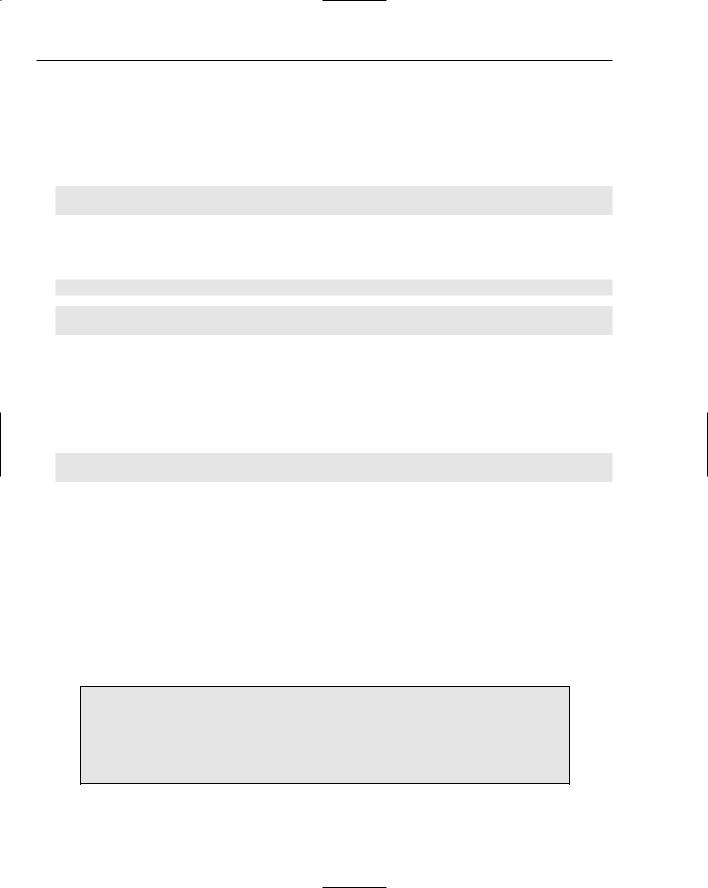
Chapter 11
The Grid Class Definition
In order to understand class templates, it is helpful to examine the syntax. The following example shows how you can tweak your GameBoard class slightly to make a templatized Grid class. Don’t let the syntax scare you — it’s all explained following the code. Note that the class name has changed from
GameBoard to Grid, and setPieceAt() and getPieceAt() have changed to setElementAt() and getElementAt() to reflect the class’ more generic nature.
// Grid.h
template <typename T> class Grid
{
public:
Grid(int inWidth = kDefaultWidth, int inHeight = kDefaultHeight); Grid(const Grid<T>& src);
~Grid();
Grid<T>& operator=(const Grid<T>& rhs);
void setElementAt(int x, int y, const T& inElem); T& getElementAt(int x, int y);
const T& getElementAt(int x, int y) const; int getHeight() const { return mHeight; } int getWidth() const { return mWidth; } static const int kDefaultWidth = 10; static const int kDefaultHeight = 10;
protected:
void copyFrom(const Grid<T>& src);
T** mCells;
int mWidth, mHeight;
};
Now that you’ve seen the full class definition, take another look at it, one line at a time:
template <typename T>
This first line says that the following class definition is a template on one type. Both template and typename are keywords in C++. As discussed earlier, templates “parameterize” types the same way that functions “parameterize” values. Just as you use parameter names in functions to represent the arguments that the caller will pass, you use type names (such as T) in templates to represent the types that the caller will specify. There’s nothing special about the name T — you can use whatever name you want.
For historical reasons, you can use the keyword class instead of typename to specify template type parameters. Thus, many books and existing programs use syntax like this: template <class T>. However, the use of the word “class” in this context is confusing because it implies that the type must be a class, which is not true. Thus, this book uses typename exclusively.
276

Writing Generic Code with Templates
The template specifier holds for the entire statement, which in this case is the class definition.
Several lines further, the copy constructor looks like this:
Grid(const Grid<T>& src);
As you can see, the type of the src parameter is no longer a const Grid&, but a const Grid<T>&. When you write a class template, what you used to think of as the class name (Grid) is actually the template name. When you want to talk about actual Grid classes or types, you discuss them as instantiations of the Grid class template for a certain type, such as int, SpreadsheetCell, or ChessPiece. You haven’t specified the real type yet, so you must use a stand-in template parameter, T, for whatever type might be used later. Thus, when you need to refer to a type for a Grid object as a parameter to, or return value from, a method you must use Grid<T>. You can see this change with the parameter to, and return value from, the assignment operator, and the parameter to the copyFrom() method.
Within a class definition, the compiler will interpret Grid as Grid<T> where needed. However, it’s best to get in the habit of specifying Grid<T> explicitly because that’s the syntax you use outside the class to refer to types generated from the template.
The final change to the class is that methods such as setElementAt() and getElementAt() now take and return parameters and values of type T instead of type GamePiece:
void setElementAt(int x, int y, const T& inElem); T& getElementAt(int x, int y);
const T& getElementAt(int x, int y) const;
This type T is a placeholder for whatever type the user specifies. mCells is now a T** instead of a GameBoard** because it will point to a dynamically allocated two-dimensional array of Ts, for whatever type T the user specifies.
Template classes can contain inline methods such as getHeight() and getWidth().
The Grid Class Method Definitions
The template <typename T> specifier must precede each method definition for the Grid template. The constructor looks like this:
template <typename T>
Grid<T>::Grid(int inWidth, int inHeight) : mWidth(inWidth), mHeight(inHeight)
{
mCells = new T* [mWidth];
for (int i = 0; i < mWidth; i++) { mCells[i] = new T[mHeight];
}
}
Note that the class name before the :: is Grid<T>, not Grid. You must specify Grid<T> as the class name in all your methods and static data member definitions. The body of the constructor is identical to the GameBoard constructor except that the placeholder type T replaces the GamePiece type.
277

Chapter 11
The rest of the method and static member definitions are also similar to their equivalents in the GameBoard class with the exception of the appropriate template and Grid<T> syntax changes:
template <typename T>
const int Grid<T>::kDefaultWidth;
template <typename T>
const int Grid<T>::kDefaultHeight;
template <typename T> Grid<T>::Grid(const Grid<T>& src)
{
copyFrom(src);
}
template <typename T>
Grid<T>::~Grid()
{
// Free the old memory.
for (int i = 0; i < mWidth; i++) { delete [] mCells[i];
}
delete [] mCells;
}
template <typename T>
void Grid<T>::copyFrom(const Grid<T>& src)
{
int i, j;
mWidth = src.mWidth; mHeight = src.mHeight;
mCells = new T* [mWidth];
for (i = 0; i < mWidth; i++) { mCells[i] = new T[mHeight];
}
for (i = 0; i < mWidth; i++) {
for (j = 0; j < mHeight; j++) { mCells[i][j] = src.mCells[i][j];
}
}
}
template <typename T>
Grid<T>& Grid<T>::operator=(const Grid<T>& rhs)
{
//Check for self-assignment. if (this == &rhs) {
return (*this);
}
//Free the old memory.
for (int i = 0; i < mWidth; i++) { delete [] mCells[i];
}
delete [] mCells;
278

Writing Generic Code with Templates
// Copy the new memory. copyFrom(rhs);
return (*this);
}
template <typename T>
void Grid<T>::setElementAt(int x, int y, const T& inElem)
{
mCells[x][y] = inElem;
}
template <typename T>
T& Grid<T>::getElementAt(int x, int y)
{
return (mCells[x][y]);
}
template <typename T>
const T& Grid<T>::getElementAt(int x, int y) const
{
return (mCells[x][y]);
}
Using the Grid Template
When you want to create grid objects, you cannot use Grid alone as a type; you must specify the type that will be stored in that Grid. Creating an object of a template class for a specific type is called instantiating the template. Here is an example:
#include “Grid.h”
int main(int argc, char** argv)
{
Grid<int> myIntGrid; // Declares a grid that stores ints myIntGrid.setElementAt(0, 0, 10);
int x = myIntGrid.getElementAt(0, 0);
Grid<int> grid2(myIntGrid);
Grid<int> anotherIntGrid = grid2;
return (0);
}
Note that the type of mIntGrid, grid2, and anotherIntGrid is Grid<int>. You cannot store SpreadsheetCells or ChessPieces in these grids; the compiler will generate an error if you try to do so.
The type specification is important: neither of the following two lines compiles:
Grid test; // WILL NOT COMPILE
Grid<> test; // WILL NOT COMPILE
The first causes your compiler to complain with something like, “use of class template requires template argument list.” The second causes it to say something like, “wrong number of template arguments.”
279