
C++ For Mathematicians (2006) [eng]
.pdf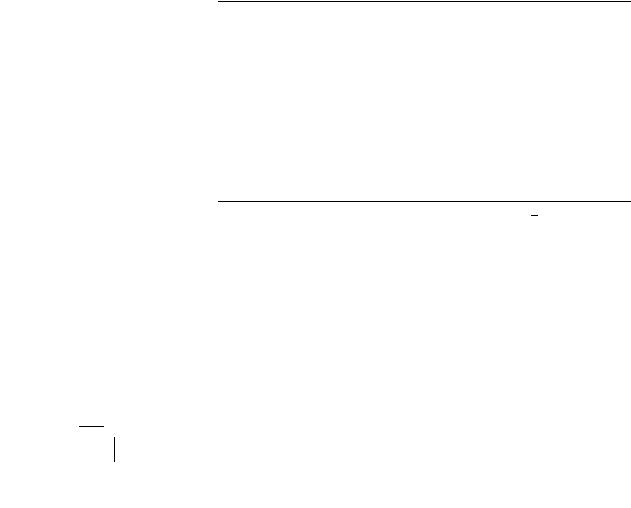


428 |
C++ for Mathematicians |
No delete[] is necessary in this second instance because fibs was not allocated with new.
5.10 Here is such a procedure.
long fibs(int n) {
const int MAX_ARG = 40; static bool first_time = true; static long values[MAX_ARG+1];
if (first_time) { values[0] = 1; values[1] = 1;
for (int k=2; k<=MAX_ARG; k++) { values[k] = values[k-1] + values[k-2];
}
first_time = false;
}
if ((n<0) || (n>MAX_ARG)) return -1; return values[n];
}
The key idea is the use of static variables. The Boolean first_time is initialized to true so the code can detect when it is first run. The array values is then populated with Fibonacci numbers and first_time is set to false so the initialization is not repeated on subsequent calls. The array values is also declared to be static so that its contents persist between invocations.
5.11 Here is such a program.
#include <iostream> using namespace std;
int main() {
long size = 10000000; // ten million long* array;
long count = 0; while (true) {
array = new long[size]; ++count;
cout << "Success with array #" << count << endl;
}
}
|
When this program is run on my computer, we see the following output. |
|
|
|
|
||
|
Success with array #1 |
|
|
|
|
|
|
|
Success with array #2 |
|
|
|
Success with array #3 |
|
|
|
Success with array #4 |
|
|
... |
|
|
|
|
Success with array #90 |
|
|
|
Success with array #91 |
|
|
|
*** malloc: vm_allocate(size=40001536) failed (error code=3) |
|
|
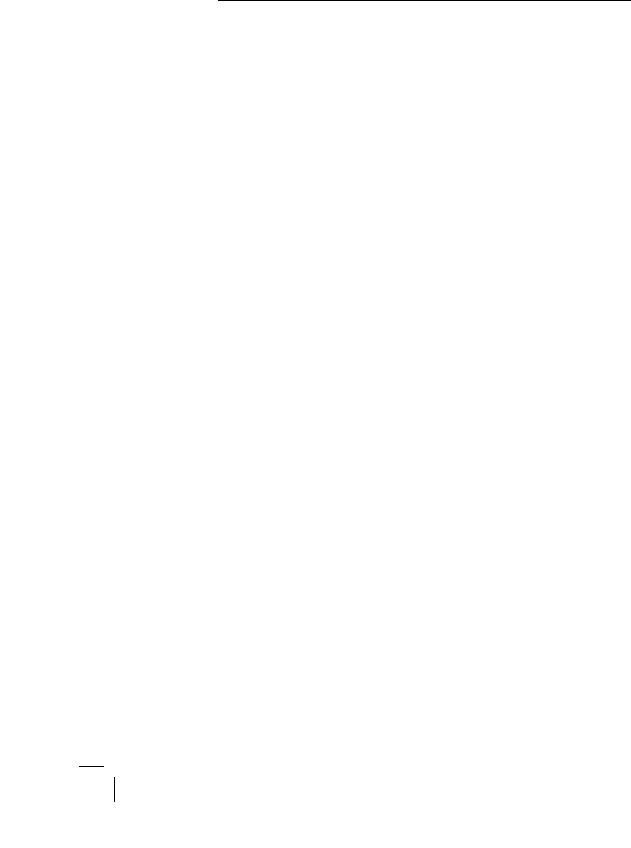

430 C++ for Mathematicians
Line::Line() {
a = b = c = 0.;
}
Line::Line(Point P, Point Q) {
//get the coordinates of the points double x1 = P.getX();
double y1 = P.getY(); double x2 = Q.getX(); double y2 = Q.getY();
//If the points are the same, make the Line horizontal
//through the one point.
if (P==Q) |
{ |
cerr << |
"Warning: Constructing Line from two equal Points" |
<< |
endl; |
a = 0.; |
|
b = 1.; |
|
c = -y1; |
|
return; |
|
} |
|
//If the points are distinct, we continue starting
//with the special case of a vertical line
if (x1 == x2) { a = 1.;
b = 0.; c = -x1; return;
}
// The points are distinct and not vertical a = y2 - y1;
b = x1 - x2;
c = x2*y1 - x1*y2;
}
Line::Line(double aa, double bb, double cc) { // Check if the data are valid
if ((aa==0.) && (bb==0.)) {
cerr << "Warning: Invalid call to Line(aa,bb,cc)" << endl; a = 0.;
b = 1.; c = cc; return;
}
a = aa; b = bb; c = cc;
}
// get methods
double Line::getA() const { return a; } double Line::getB() const { return b; }

Answers |
431 |
double Line::getC() const { return c; }
// reflection methods
void Line::reflectX() { b = -b; } void Line::reflectY() { a = -a; }
//check if a Point is on this Line bool Line::incident(Point P) const {
return a*P.getX() + b*P.getY() + c == 0. ;
}
//generate a Point on a Line
Point Line::find_Point() const {
//if it’s a vertical line, return x-intercept if (b==0.) {
return Point(-c/a, 0);
}
//otherwise, return the x-intercept
return Point(0,-c/b);
}
bool Line::operator==(const Line& that) const {
//Special case when a == 0 if (a==0) {
if (that.a != 0) return false; return c/b == that.c/that.b;
}
//When a is not 0
if (that.a == 0) return false;
return (b/a == that.b/that.a) && (c/a == that.c/that.a);
}
// for writing to an output stream
ostream& operator<<(ostream& os, const Line& L) { os << "[" << L.getA() << "," << L.getB() << ","
<< L.getC() << "]"; return os;
}
// determine the distance from a Point to a Line double dist(Line L, Point P) {
//fetch a,b,c for the line double a = L.getA(); double b = L.getB(); double c = L.getC();
//fetch the coordinates of the point double x = P.getX();
double y = P.getY();
//normalize by dividing by sqrt(a*a+b*b) double d = sqrt(a*a + b*b);
a /= d; b /= d; c /= d;

432 |
C++ for Mathematicians |
// project P onto the unit vector (a,b) and add c double ans = x*a + y*b + c;
return fabs(ans);
}
double dist(Point P, Line L) { return dist(L,P); }
6.2It is natural to suspect that something is wrong with the logic and algebra used to create the procedures, but that is not the case. If we examine the [a,b,c] triples for the two lines, we see that the triple for L is a scalar multiple of the triple for M (the factor is 1.4), so these represent the same line.
The problem is roundoff. Indeed, if we print the distance from Y to M the computer responds 8.88178e-16. Minute errors in the divisions cause the problems; note that the slope of the line is −5/7 and the quotient cannot be held exactly in a double variable.
There is no perfect solution to this problem. A good strategy is to replace exact equality tests with |x − y| < ε where ε is either built into the code or is set by the user.
6.3The modification to Line.h is modest. The old private section would be replaced by this:
private: Point A; Point B;
Nothing else in Line.h would need to be modified.
Extensive repairs to Line.cc are now needed. For example, the get methods would need to be completely reworked.
However, code that uses the Line class would not require any modification at all!
6.4 A good way to do this is with a procedure declared like this:
bool intersect(Line L, Line M, Point& P);
The input Line objects are L and M. If they are parallel, then the procedure should return false (to mean do not intersect) and leave P unaffected. Otherwise, we set P to be the Point of intersection and return true.
6.5It is not unreasonable to make the end points of a LineSegment object be public data elements because if a program modifies these data directly (and not through a set method), there is no possibility of creating an invalid object (we allow the two end points to be the same). By contrast, were we to allow open access to a Line object’s data, an unwary user might set both a and b to 0 creating an invalid Line object.

Answers |
433 |
In general, it is better to keep the data hidden and write simple get and set methods to manipulate those data.
Here is a header file LineSegment.h. Writing the LineSegment.cc file is left to you.
#ifndef LINE_SEGMENT_H #define LINE_SEGMENT_H
#include <iostream> #include "Point.h" using namespace std;
class LineSegment { private:
// the end points of the segment Point A;
Point B;
public:
//constructors LineSegment(); LineSegment(Point P, Point Q);
LineSegment(double x1, double y1, double x2, double y2);
//get & set methods
Point getA() const; Point getB() const; void setA(Point P); void setB(Point Q);
//Length and midpoint methods double length() const;
Point midpoint() const;
//Check for equality
bool operator==(const Point& that);
};
// for output
ostream& operator<<(ostream& os, const LineSegment& LS); #endif
6.6Here are two solutions. In both cases, updating the fields x and y is straightforward. The key issue is: how do we write the return statement at the end of the procedure.
In this first solution, we make a copy of the Point and return that:
Point Point::translate(double dx, double dy) { x += dx;
y += dy;
return Point(x,y);
}

434 |
C++ for Mathematicians |
This code is clear but suffers a minor inefficiency because it makes a copy of the Point and then that copy gets sent back as a return value. We can make this more efficient by returning the Point object itself. Here is how that is done.
Point Point::translate(double dx, double dy) { x += dx;
y += dy; return *this;
}
The object *this is the Point object itself, so no extra copy is needed.
In this simple example, the inefficiency is minimal. However, if this method were to be used extensively then the change from return Point(x,y); to return *this; can make a noticeable difference.
6.7This is a subtle problem. Suppose we define a procedure proc with one argument. There are two ways we can declare this procedure:
Point proc(Point Q);
Point proc(Point &Q);
If we use the first syntax, then a copy of the argument is sent to the procedure, but if we use the second syntax, then the argument itself is sent. That is, when we call proc(W) the first version cannot modify W, but the second version can.
Similarly, the normal behavior of return X is to send back a copy of X. However, if we want to return the object X itself, we need to add an & to the return type in the procedure declaration.
For the problem at hand, we need to change the return type of the translate method from Point to Point&. In the public section in Point.h we declare the procedure like this.
Point& translate(double dx, double dy);
In Point.cc we have the following code.
Point& Point::translate(double dx,double dy) { x += dx;
y += dy; return *this;
}
Let’s examine how the old versions (from the previous problem) and this new version behave for the code (P.translate(1,2)).translate(10,10);.
In either old version P.translate(1,2) returns a copy of P. (The first old version returns a copy of a copy of P!) So the second invocation of translate acts on a copy of P and not the object P itself. Therefore, P is not modified by translate(10,10).

Answers |
435 |
However, in the new version, the statement return *this; returns the object itself and not a copy. Therefore the second translate(10,10) acts on P and not on some unnamed copy of P.
One last point: In the solution to Exercise 6.6, both return Point(x,y); and return *this; have the intended effect of returning a copy of the Point. In this exercise, the code return Point(x,y); is inappropriate. We do not want to return a copy of the Point; we want to return the Point itself. So we must use return *this;.
7.1Here is the file Interval.h. Note that the keyword inline is optional for the class methods but mandatory for the operator<< procedure. We require both
#include <iostream> and using namespace std; to use ostream objects.
#ifndef INTERVAL_H #define INTERVAL_H #include <iostream> using namespace std;
class Interval { private:
double a,b; // end points of [a,b]
public: Interval() {
a = 0.; b = 1.;
}
Interval(double x, double y) { if (x<y) {
a = x; b = y;
}
else {
a = y; b = x;
}
}
double getA() const { return a; } double getB() const { return b; }
bool operator==(const Interval& that) const { return (a==that.a) && (b==that.b);
}
bool operator!=(const Interval& that) const { return !(*this == that);
}
bool operator<(const Interval& that) const { if (a < that.a) return true;
if (a > that.a) return false;