
- •Table of Contents
- •Preface
- •Additional Material
- •Basic Electronics
- •1.0 The Atom
- •1.1 Isotopes and Ions
- •1.2 Static Electricity
- •1.3 Electrical Charge
- •1.4 Electrical Circuits
- •1.5 Circuit Elements
- •1.6 Semiconductors
- •Number Systems
- •2.0 Counting
- •2.1 The Origins of the Decimal System
- •2.2 Types of Numbers
- •2.3 Radix Representations
- •2.4 Number System Conversions
- •Data Types and Data Storage
- •3.0 Electronic-Digital Machines
- •3.1 Character Representations
- •3.2 Storage and Encoding of Integers
- •3.3 Encoding of Fractional Numbers
- •3.4 Binary-Coded Decimals (BCD)
- •Digital Logic, Arithmetic, and Conversions
- •4.0 Microcontroller Logic and Arithmetic
- •4.1 Logical Instructions
- •4.2 Microcontroller Arithmetic
- •4.3 Bit Manipulations and Auxiliary Operations
- •4.4 Unsigned Binary Arithmetic
- •4.5 Signed Binary Arithmetic
- •4.6 Data Format Conversions
- •Circuits and Logic Gates
- •5.0 Digital Circuits
- •5.1 The Diode Revisited
- •5.2 The Transistor
- •5.3 Logic Gates
- •5.4 Transistor-Transistor Logic
- •5.5 Other TTL Logic Families
- •5.6 CMOS Logic Gates
- •Circuit Components
- •6.0 Power Supplies
- •6.1 Clocked Logic and Flip-flops
- •6.2 Clocks
- •6.3 Frequency Dividers and Counters
- •6.4 Multiplexers and Demultiplexers
- •6.5 Input Devices
- •The Microchip PIC
- •7.0 The PICMicro Microcontroller
- •7.1 PIC Architecture
- •Mid-range PIC Architecture
- •8.0 Processor Architecture and Design
- •8.1 The Mid-range Core Features
- •8.2 Mid-Range CPU and Instruction Set
- •8.3 EEPROM Data Storage
- •8.4 Data Memory Organization
- •8.5 Mid-range I/O and Peripheral Modules
- •PIC Programming: Tools and Techniques
- •9.0 Microchip’s MPLAB
- •9.1 Integrated Development Environment
- •9.2 Simulators and Debuggers
- •9.3 Programmers
- •9.4 Engineering PIC Software
- •9.5 Pseudo Instructions
- •Programming Essentials: Input and Output
- •10.0 16F84A Programming Template
- •10.1 Introducing the 16F84A
- •10.2 Simple Circuits and Programs
- •10.3 Programming the Seven-segment LED
- •10.4 A Demonstration Board
- •Interrupts
- •11.0 Interrupts on the 16F84
- •11.1 Interrupt Sources
- •11.2 Interrupt Handlers
- •11.3 Interrupt Programming
- •11.4 Sample Programs
- •Timers and Counters
- •12.0 The 16F84 Timer0 Module
- •12.1 Delays Using Timer0
- •12.2 Timer0 as a Counter
- •12.3 Timer0 Programming
- •12.4 The Watchdog Timer
- •12.5 Sample Programs
- •LCD Interfacing and Programming
- •13.0 LCD Features and Architecture
- •13.1 Interfacing with the HD44780
- •13.2 HD44780 Instruction Set
- •13.3 LCD Programming
- •13.4 Sample Programs
- •Communications
- •14.0 PIC Communications Overview
- •14.1 Serial Data Transmission
- •14.2 Parallel Data Transmission
- •14.4 PIC Protocol-based Serial Programming
- •14.5 Sample Programs
- •Data EEPROM Programming
- •15.0 PIC Internal EEPROM Memory
- •15.1 EEPROM Devices and Interfaces
- •15.2 Sample Programs
- •Analog to Digital and Realtime Clocks
- •16.0 A/D Converters
- •16.1 A/D Integrated Circuits
- •16.2 PIC On-Board A/D Hardware
- •16.3 Realtime Clocks
- •16.4 Sample Programs
- •Index

Chapter 10
Programming Essentials: Input and Output
In this chapter, we discuss the simplest circuits and programming operations. Using a PIC to control an LED or read a switch is as elementary as it gets. However, neither of these operations is trivial, since there is more to it than a few lines of code. Other input/output devices that are also considered are seven-segment LED displays and multiple switches, sometimes called toggle switches. A bank of multiple LEDs can also function as a binary output device.
10.0 16F84A Programming Template
We have found that program development can be simplified considerably by using code templates. A code template is a program devoid of functionality that serves to implement the most common and typical features of an application. The template not only saves the effort of redoing the same tasks, but reminds the programmer of program elements that could otherwise be forgotten. A professional developer will have collected many different templates over the years for different types of applications on various processors. The following template is for the 16F84A PIC:
;============================================================
;File name:
;Date:
;Author:
;Processor:
;Reference circuit: ;============================================================
;Copyright notice: ;============================================================
;Program Description:
;
;===========================
;configuration switches ;===========================
;Switches used in __config directive:
189
190 |
Chapter 10 |
|
; |
_CP_ON |
Code protection ON/OFF |
; * _CP_OFF |
|
|
; |
* _PWRTE_ON |
Power-up timer ON/OFF |
;_PWRTE_OFF
; |
_WDT_ON |
Watchdog timer ON/OFF |
; * _WDT_OFF |
|
|
; |
_LP_OSC |
Low power crystal osccillator |
; * _XT_OSC |
External parallel resonator |
|
; |
_HS_OSC |
High speed crystal resonator (8 to 10 MHz) |
; |
_RC_OSC |
Resistor/capacitor oscillator |
; |
|
(simplest, 20% error) |
;|
;|_____ * indicates setup values
;========================= ; setup and configuration ;=========================
|
processor 16f84A |
|
|
include |
<p16f84A.inc> |
|
__config |
_XT_OSC & _WDT_OFF & _PWRTE_ON & _CP_OFF |
;===================================================== |
||
; |
|
constant definitions |
;===================================================== |
||
;===================================================== |
||
; |
|
PIC register equates |
;===================================================== |
||
;===================================================== |
||
; |
variables in PIC RAM |
|
;===================================================== |
||
|
cblock |
0x0c |
|
endc |
|
;============================================================
; program
;============================================================
org |
0 |
; start at address |
goto |
main |
|
; Space for interrupt handlers |
|
|
org |
0x08 |
|
main:
;============================================================ end ; END OF PROGRAM
;============================================================
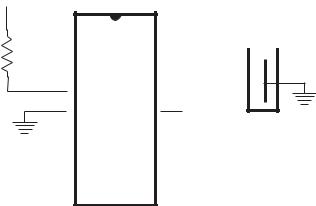
Programming Essentials: Input and Output |
191 |
In addition to the template file the program developer should keep at hand the necessary include files. In this case, p16f84a.inc.
10.1 Introducing the 16F84A
The circuits and programs in this chapter use the 16F84A, probably the most popular of all mid-range PIC microcontrollers. Although we have discussed the mid-range architecture, we start with a review of this processor in order to establish a base for the material that follows.
10.1.1 Template Circuit for 16F84A
Like the programmer uses a programming template for developing 16F84A code, the circuit designer uses a template circuit. This circuit contains the components that most 16F84A boards require. The elements include a diagram of the PIC itself with the pin-out, as well as the wiring of the standard components, including the power source, ground, the reset pin (MCLR), and the most commonly used oscillator. Figure 10-1 shows a circuit template for the 16F84A.
+5v
1 |
|
18 |
||
|
RA2 |
RA1 |
|
|
|
2 |
|
17 |
Osc |
||
|
|
|
||||
R=10K |
|
RA3 |
RA0 |
|
|
|
|
|
|
|
|
|
3 |
|
16F84A |
16 |
|
|||||
|
|
||||||||
|
|
RA4/TOCKI |
OSC1 |
|
|
|
|||
4 |
|
|
|
|
|
|
15 |
|
|
5 |
|
MCLR |
OSC2 |
|
|
|
|||
|
|
|
|
|
|
14 |
|
||
|
|
|
|
|
|
+5v |
|||
|
|
Vss |
Vdd |
|
|
|
|
|
|
6 |
|
|
|
|
|
|
13 |
|
|
|
|
RB0/INT |
RB7 |
|
|
|
|
||
7 |
|
|
|
|
|
|
12 |
|
|
|
|
RB1 |
RB6 |
|
|
|
|||
8 |
|
|
|
|
|
|
11 |
|
|
|
|
|
|
|
|
|
|
||
|
|
RB2 |
RB5 |
|
|
|
|
||
9 |
|
|
|
|
|
|
10 |
|
|
|
|
RB3 |
RB4 |
|
|
|
|
Figure 10-1 16F84A Circuit Template
The circuit template in Figure 10-1 does not suit every possible circuit. Even the simplest components must sometimes be configured differently; for example, the reset line could be wired to a pushbutton switch, or a different oscillator may be used. In any case, it is always easier to make modifications to an existing base than to start from scratch every time.
10.1.2 Power Supplies
Every PIC-based circuit board requires a +5V power source. A possible source of power is one or more batteries. There is an enormous selection of battery types, sizes, and qualities. The most common ones for use in experimental circuits are listed in Table 10.1.
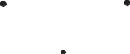
192 |
|
|
Chapter 10 |
|
|
Table 10.1 |
|
|
Common Dry Cell Alkaline Battery Types |
|
|
|
|
|
|
DESIGNATION |
VOLTAGE |
LENGTH |
DIAMETER |
|
MM. |
MM. |
|
|
|
|
|
D |
1.5 |
61.5 |
34.2 |
C |
1.5 |
50 |
26.2 |
AA |
1.5 |
50 |
14.2 |
AAA |
1.5 |
44.5 |
10.4 |
AAAA |
1.5 |
42.5 |
8.3 |
All of the batteries in Table 10.1 produce 1.5V. A PIC with a supply voltage of 2 to 6 volts uses two to four batteries. Note that in selecting the battery power source for a PIC-based circuit, other elements beside the microcontroller itself must be considered, such as the oscillator. Holders for several interconnected batteries are available at electronic supply sources.
Alternatively, the power supply can be a transformer with 120VAC input and 3 to 12VDC called AC/DC adapters. The most useful type for the experimenter are the ones with an ON/OFF switch and several selectable output voltages. Color-coded alligator clips at the output wires are convenient.
Voltage Regulator
A useful device for a typical PIC-based power source is a voltage regulator IC. The 7805 voltage regulator is ubiquitous in most PIC-based boards with AC/DC adapter sources. The IC is a three-pin device whose purpose is to ensure a stable voltage source which does not exceed the device rating. The 7805 is rated for 5V and produces this output from any input source in the range 8 to 35V. Since the excess voltage is dissipated as heat the 7805 is equipped with a metallic plate intended for attaching a heat sink. The heat sink is not required in a typical PIC application but it is a good idea to maintain the supply voltage closer to the device minimum rather than its maximum.
The voltage regulator circuit requires two capacitors: one electrolytic and the other one not. Figure 10-2 shows a power source circuit using the 7805.
|
|
|
|
+5v DC |
|
9 -35v DC |
||||||||||||||
|
|
|
|
output |
|
input |
||||||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
OUT |
IN |
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
|
||||||||||
EC=100mF |
|
|
+ |
|
|
78L05 |
|
|
|
|
|
C=0.1mF |
||||||||
|
|
|
|
|
|
|
|
|
||||||||||||
|
|
|
|
|
|
|
|
|
||||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Figure 10-2 Voltage Stabilizer Circuit
Programming Essentials: Input and Output |
193 |
10.1.3 Comparisons in PIC Programming
The power and usefulness of programs is due, in great measure, to their deci- sion-making ability, and decisions are based on comparison. In a comparison code, it is able to make decisions based on the relative values of two operands. For example, compare the values a and b. If a is greater than b execute a certain code routine. If b is greater than a, execute another one. If both operands have the same value then proceed to a third code branch.
CISC and even some RISC microprocessors contain a compare operator in their instruction set. However, the compare can be substituted, with some inconvenience, by a subtraction. Since there is no compare operation in the PIC instruction set, we have to simulate the comparison by subtracting the w register from a literal value or from a file register. The sublw and subwf instructions can be used. After the subtraction takes place, code can make decisions based on the state of the zero and the carry flags. For example, the following code fragment compares the value in the two registers, labeled OP1 and OP2 respectively, and directs execution to three possible routines:
; Declare variables at 2 memory locations
OP1 |
equ |
0x0c |
|
; First operand |
OP2 |
equ |
0x0d |
|
; second operand |
. |
|
|
|
|
. |
|
|
|
|
. |
|
|
|
|
main: |
|
|
|
|
|
movlw |
0x30 |
; First operand |
|
|
movwf |
OP1 |
; to OP1 register |
|
|
movlw |
0x50 |
; Second operand |
|
|
movwf |
OP2 |
; To OP2 register |
|
|
movf |
OP2,w |
; OP2 to w register (not really |
|
|
|
|
; necessary) |
|
|
subwf |
OP1,w |
; Subtract w (OP2) from OP1 |
|
|
btfsc |
STATUS,2 ; 2 is zero bit. Test zero flag. |
||
|
|
|
; Skip next instruction if Z bit = 0, |
|
|
|
|
; that is if both numbers are not the |
|
|
|
|
; same |
|
|
goto |
ops_are_eq |
; OP2 = w routine |
;At this point the zero flag is not set. Therefore the two operands
;are not equal
;Now test the carry flag for OP1 < OP2, in this case C = 1
btfss |
STATUS,0 ; 0 is carry bit. Test carry flag |
|
|
|
; and skip next instruction if |
|
|
; C bit = 1 |
goto |
op2big |
; OP2 > w routine |
; Processing for the case OP1 > OP2 |
||
nop |
|
|
goto |
done |
|