
- •Preface
- •Introduction
- •1.01 INCLUDES.H
- •1.02 Compiler Independent Data Types
- •1.03 Global Variables
- •1.04 OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL()
- •1.05 PC Based Services
- •1.05.01 PC Based Services, Character Based Display
- •1.05.02 PC Based Services, Elapsed Time Measurement
- •1.05.03 PC Based Services, Miscellaneous
- •1.07 Example #1
- •1.08 Example #2
- •1.09 Example #3
- •2.00 Foreground/Background Systems
- •2.01 Critical Section of Code
- •2.02 Resource
- •2.03 Shared Resource
- •2.04 Multitasking
- •2.05 Task
- •2.06 Context Switch (or Task Switch)
- •2.07 Kernel
- •2.08 Scheduler
- •2.09 Non-Preemptive Kernel
- •2.10 Preemptive Kernel
- •2.11 Reentrancy
- •2.12 Round Robin Scheduling
- •2.13 Task Priority
- •2.14 Static Priorities
- •2.15 Dynamic Priorities
- •2.16 Priority Inversions
- •2.17 Assigning Task Priorities
- •2.19 Mutual Exclusion
- •2.20 Deadlock (or Deadly Embrace)
- •2.21 Synchronization
- •2.22 Event Flags
- •2.23 Intertask Communication
- •2.24 Message Mailboxes
- •2.25 Message Queues
- •2.26 Interrupts
- •2.27 Interrupt Latency
- •2.28 Interrupt Response
- •2.29 Interrupt Recovery
- •2.30 Interrupt Latency, Response, and Recovery
- •2.31 ISR Processing Time
- •2.32 Non-Maskable Interrupts (NMIs)
- •2.33 Clock Tick
- •2.34 Memory Requirements
- •2.35 Advantages and Disadvantages of Real-Time Kernels
- •2.36 Real-Time Systems Summary
- •3.00 Critical Sections
- •3.01 Tasks
- •3.02 Task States
- •3.03 Task Control Blocks (OS_TCBs)
- •3.04 Ready List
- •3.05 Task Scheduling
- •3.06 Locking and Unlocking the Scheduler
- •3.07 Idle Task
- •3.08 Statistics Task
- •3.10 Clock Tick
- •3.14 OSEvent???() functions
- •4.00 Creating a Task, OSTaskCreate()
- •4.01 Creating a Task, OSTaskCreateExt()
- •4.02 Task Stacks
- •4.03 Stack Checking, OSTaskStkChk()
- •4.04 Deleting a Task, OSTaskDel()
- •4.05 Requesting to delete a task, OSTaskDelReq()
- •4.06 Changing a Task’s Priority, OSTaskChangePrio()
- •4.07 Suspending a Task, OSTaskSuspend()
- •4.08 Resuming a Task, OSTaskResume()
- •4.09 Getting Information about a Task, OSTaskQuery()
- •5.00 Delaying a task, OSTimeDly()
- •5.01 Delaying a task, OSTimeDlyHMSM()
- •5.02 Resuming a delayed task, OSTimeDlyResume()
- •5.03 System time, OSTimeGet() and OSTimeSet()
- •6.00 Event Control Blocks
- •6.01 Initializing an ECB, OSEventWaitListInit()
- •6.02 Making a task ready, OSEventTaskRdy()
- •6.03 Making a task wait for an event, OSEventTaskWait()
- •6.04 Making a task ready because of a timeout, OSEventTO()
- •6.05 Semaphores
- •6.06 Message Mailboxes
- •6.07 Message Queues
- •7.00 Memory Control Blocks
- •7.01 Creating a partition, OSMemCreate()
- •7.02 Obtaining a memory block, OSMemGet()
- •7.03 Returning a memory block, OSMemPut()
- •7.04 Obtaining status about memory partition, OSMemQuery()
- •7.05 Using memory partitions
- •7.06 Waiting for memory blocks from a partition
- •8.00 Development Tools
- •8.01 Directories and Files
- •8.02 INCLUDES.H
- •9.00 Development Tools
- •9.01 Directories and Files
- •9.02 INCLUDES.H
- •9.06 Memory requirements
- •9.07 Execution times
- •10.00 Directories and Files
- •10.01 INCLUDES.H
- •10.02.01 OS_CPU.H, Compiler specific data types
- •10.02.02 OS_CPU.H, OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL()
- •10.02.03 OS_CPU.H, OS_STK_GROWTH
- •10.02.04 OS_CPU.H, OS_TASK_SW()
- •10.03.01 OS_CPU_A.ASM, OSStartHighRdy()
- •10.03.02 OS_CPU_A.ASM, OSCtxSw()
- •10.03.03 OS_CPU_A.ASM, OSIntCtxSw()
- •10.03.04 OS_CPU_A.ASM, OSTickISR()
- •10.04.01 OS_CPU_C.C, OSTaskStkInit()
- •10.04.02 OS_CPU_C.C, OSTaskCreateHook()
- •10.04.03 OS_CPU_C.C, OSTaskDelHook()
- •10.04.04 OS_CPU_C.C, OSTaskSwHook()
- •10.04.05 OS_CPU_C.C, OSTaskStatHook()
- •10.04.06 OS_CPU_C.C, OSTimeTickHook()
- •10.05 Summary
- •OSInit()
- •OSIntEnter()
- •OSIntExit()
- •OSMboxAccept()
- •OSMboxCreate()
- •OSMboxPend()
- •OSMboxPost()
- •OSMboxQuery()
- •OSMemCreate()
- •OSMemGet()
- •OSMemPut()
- •OSMemQuery()
- •OSQAccept()
- •OSQCreate()
- •OSQFlush()
- •OSQPend()
- •OSQPost()
- •OSQPostFront()
- •OSQQuery()
- •OSSchedLock()
- •OSSchedUnlock()
- •OSSemAccept()
- •OSSemCreate()
- •OSSemPend()
- •OSSemPost()
- •OSSemQuery()
- •OSStart()
- •OSStatInit()
- •OSTaskChangePrio()
- •OSTaskCreate()
- •OSTaskCreateExt()
- •OSTaskDel()
- •OSTaskDelReq()
- •OSTaskResume()
- •OSTaskStkChk()
- •OSTaskSuspend()
- •OSTaskQuery()
- •OSTimeDly()
- •OSTimeDlyHMSM()
- •OSTimeDlyResume()
- •OSTimeGet()
- •OSTimeSet()
- •OSTimeTick()
- •OSVersion()
OSQFlush()
INT8U *OSQFlush(OS_EVENT *pevent);
File |
Called from |
Code enabled by |
OS_Q.C |
Task or ISR |
OS_Q_EN |
OSQFlush() is used to empty the contents of the message queue and basically eliminate all the messages sent to the queue. This function takes the same amount of time to execute whether tasks are waiting on the queue (and thus no messages are present) or the queue contains one or more messages.
Arguments
pevent is a pointer to the message queue. This pointer is returned to your application when the message queue is created (see OSQCreate()).
Returned Value
One of the following codes:
1)OS_NO_ERR, the message queue was flushed.
2)OS_ERR_EVENT_TYPE, if you attempted to flush an object other than a message queue.
Notes/Warnings
Queues must be created before they are used.
Example
OS_EVENT *CommQ; |
|
|
void main(void) |
|
|
{ |
|
|
INT8U err; |
|
|
OSInit(); |
/* Initialize µC/OS-II |
*/ |
. |
|
|
. |
|
|
err = OSQFlush(CommQ); |
|
|
. |
|
|
. |
|
|
OSStart(); |
/* Start Multitasking |
*/ |
} |
|
|
OSQPend()
void *OSQPend(OS_EVENT *pevent, INT16U timeout, INT8U *err);
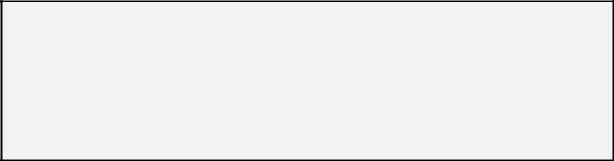
File |
Called from |
Code enabled by |
OS_Q.C |
Task only |
OS_Q_EN |
OSQPend() is used when a task desires to receive messages from a queue. The messages are sent to the task either
by an ISR or by another task. The messages received are pointer size variables and their use is application specific. If a at least one message is present at the queue when OSQPend() is called, the message is retrieved and returned to the caller. If no message is present at the queue, OSQPend() will suspend the current task until either a message
is received or a user specified timeout expires. If a message is sent to the queue and multiple tasks are waiting for such
a message then, µC/OS-II will resume the highest priority task that is waiting. A pended task that has been suspended with OSTaskSuspend() can receive a message. The task will, however, remain suspended until the task is
resumed by calling OSTaskResume().
Arguments
pevent is a pointer to the queue where the messages are to be received from. This pointer is returned to your application when the queue is created (see OSQCreate()).
timeout is used to allow the task to resume execution if a message is not received from the mailbox within the specified number of clock ticks. A timeout value of 0 indicates that the task desires to wait forever for the message. The maximum timeout is 65535 clock ticks. The timeout value is not synchronized with the clock tick. The timeout count starts being decremented on the next clock tick which could potentially occur immediately.
err is a pointer to a variable which will be used to hold an error code. OSQPend() sets *err to either:
1)OS_NO_ERR, a message was received
2)OS_TIMEOUT, a message was not received within the specified timeout
3)OS_ERR_PEND_ISR, you called this function from an ISR and µC/OS-II would have to suspend the ISR. In general, you should not call OSQPend(). µC/OS-II checks for this situation in case you do anyway.
Returned Value
OSQPend() returns a message sent by either a task or an ISR and *err is set to OS_NO_ERR. If a timeout occurred,
OSQPend() returns a NULL pointer and sets *err to OS_TIMEOUT.
Notes/Warnings
Queues must be created before they are used.
Example
OS_EVENT *CommQ;
void CommTask(void *data)
{
INT8U err; void *msg;
pdata = pdata;
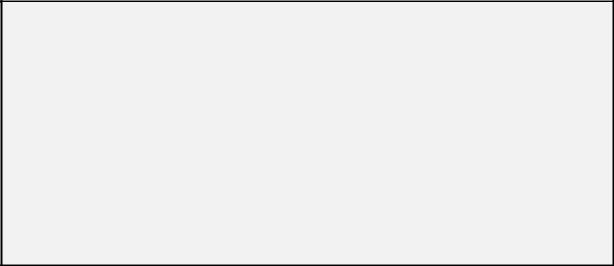
for (;;) {
. |
|
|
. |
|
|
msg = OSQPend(CommQ, 100, &err); |
|
|
if (err == OS_NO_ERR) { |
|
|
. |
|
|
. |
/* Message received within 100 ticks! |
*/ |
. |
|
|
} else { |
|
|
. |
|
|
. |
/* Message not received, must have timed out |
*/ |
. |
|
|
} |
|
|
. |
|
|
. |
|
|
} |
|
|
} |
|
|
OSQPost()
INT8U OSQPost(OS_EVENT *pevent, void *msg);
File |
Called from |
Code enabled by |
OS_Q.C |
Task or ISR |
OS_Q_EN |
OSQPost() is used to send a message to a task through a queue. A message is a pointer size variable and its use is application specific. If the message queue is full an error code is returned to the caller. OSQPost() will then
immediately return to its caller and the message will not be placed in the queue. If any task is waiting for a message at the queue then, the highest priority task will receive the message. If the task waiting for the message has a higher priority than the task sending the message then, the higher priority task will be resumed and the task sending the message will be suspended. In other words, a context switch will occur. Message queues are first-in-first-out (FIFO) which means that the first message sent will be the first message received.
Arguments
peventis a pointer to the queue where the message is to be deposited into. This pointer is returned to your application when the queue is created (see OSQCreate()).
msg is the actual message sent to the task. msg is a pointer size variable and is application specific. You MUST never post a NULL pointer.
Returned Value
OSQPost() returns one of these two error codes
1)OS_NO_ERR, if the message was deposited in the queue
2)OS_Q_FULL, if the queue is already full
Notes/Warnings
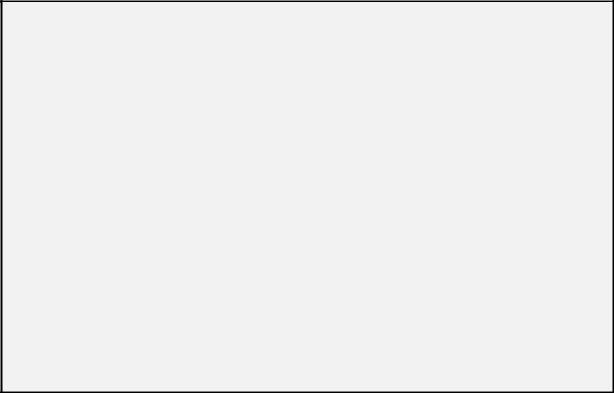
Queues must be created before they are used.
Example
OS_EVENT *CommQ; |
|
|
INT8U |
CommRxBuf[100]; |
|
void CommTaskRx(void *pdata) |
|
|
{ |
|
|
INT8U |
err; |
|
pdata = pdata; |
|
|
for (;;) { |
|
|
. |
|
|
. |
|
|
err = OSQPost(CommQ, (void *)&CommRxBuf[0]); |
|
|
if (err == OS_NO_ERR) { |
|
|
. |
/* Message was deposited into queue |
*/ |
. |
|
|
} else { |
|
|
. |
/* Queue is full |
*/ |
. |
|
|
}
.
.
}
}
OSQPostFront()
INT8U OSQPostFront(OS_EVENT *pevent, void *msg);
File |
Called from |
Code enabled by |
OS_Q.C |
Task or ISR |
OS_Q_EN |
OSQPostFront() is used to send a message to a task through a queue. OSQPostFront() behaves very much like OSQPost() except that the message is inserted at the front of the queue. This means that OSQPostFront() makes the message queue behave like a last-in-first-out (LIFO) queue instead of a
first-in-first-out (FIFO) queue. A message is a pointer size variable and its use is application specific. If the message queue is full an error code is returned to the caller. OSQPostFront() will then immediately return to its caller
and the message will not be placed in the queue. If any task is waiting for a message at the queue then, the highest priority task will receive the message. If the task waiting for the message has a higher priority than the task sending the message then, the higher priority task will be resumed and the task sending the message will be suspended. In other words, a context switch will occur.
Arguments