
- •Preface
- •Introduction
- •1.01 INCLUDES.H
- •1.02 Compiler Independent Data Types
- •1.03 Global Variables
- •1.04 OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL()
- •1.05 PC Based Services
- •1.05.01 PC Based Services, Character Based Display
- •1.05.02 PC Based Services, Elapsed Time Measurement
- •1.05.03 PC Based Services, Miscellaneous
- •1.07 Example #1
- •1.08 Example #2
- •1.09 Example #3
- •2.00 Foreground/Background Systems
- •2.01 Critical Section of Code
- •2.02 Resource
- •2.03 Shared Resource
- •2.04 Multitasking
- •2.05 Task
- •2.06 Context Switch (or Task Switch)
- •2.07 Kernel
- •2.08 Scheduler
- •2.09 Non-Preemptive Kernel
- •2.10 Preemptive Kernel
- •2.11 Reentrancy
- •2.12 Round Robin Scheduling
- •2.13 Task Priority
- •2.14 Static Priorities
- •2.15 Dynamic Priorities
- •2.16 Priority Inversions
- •2.17 Assigning Task Priorities
- •2.19 Mutual Exclusion
- •2.20 Deadlock (or Deadly Embrace)
- •2.21 Synchronization
- •2.22 Event Flags
- •2.23 Intertask Communication
- •2.24 Message Mailboxes
- •2.25 Message Queues
- •2.26 Interrupts
- •2.27 Interrupt Latency
- •2.28 Interrupt Response
- •2.29 Interrupt Recovery
- •2.30 Interrupt Latency, Response, and Recovery
- •2.31 ISR Processing Time
- •2.32 Non-Maskable Interrupts (NMIs)
- •2.33 Clock Tick
- •2.34 Memory Requirements
- •2.35 Advantages and Disadvantages of Real-Time Kernels
- •2.36 Real-Time Systems Summary
- •3.00 Critical Sections
- •3.01 Tasks
- •3.02 Task States
- •3.03 Task Control Blocks (OS_TCBs)
- •3.04 Ready List
- •3.05 Task Scheduling
- •3.06 Locking and Unlocking the Scheduler
- •3.07 Idle Task
- •3.08 Statistics Task
- •3.10 Clock Tick
- •3.14 OSEvent???() functions
- •4.00 Creating a Task, OSTaskCreate()
- •4.01 Creating a Task, OSTaskCreateExt()
- •4.02 Task Stacks
- •4.03 Stack Checking, OSTaskStkChk()
- •4.04 Deleting a Task, OSTaskDel()
- •4.05 Requesting to delete a task, OSTaskDelReq()
- •4.06 Changing a Task’s Priority, OSTaskChangePrio()
- •4.07 Suspending a Task, OSTaskSuspend()
- •4.08 Resuming a Task, OSTaskResume()
- •4.09 Getting Information about a Task, OSTaskQuery()
- •5.00 Delaying a task, OSTimeDly()
- •5.01 Delaying a task, OSTimeDlyHMSM()
- •5.02 Resuming a delayed task, OSTimeDlyResume()
- •5.03 System time, OSTimeGet() and OSTimeSet()
- •6.00 Event Control Blocks
- •6.01 Initializing an ECB, OSEventWaitListInit()
- •6.02 Making a task ready, OSEventTaskRdy()
- •6.03 Making a task wait for an event, OSEventTaskWait()
- •6.04 Making a task ready because of a timeout, OSEventTO()
- •6.05 Semaphores
- •6.06 Message Mailboxes
- •6.07 Message Queues
- •7.00 Memory Control Blocks
- •7.01 Creating a partition, OSMemCreate()
- •7.02 Obtaining a memory block, OSMemGet()
- •7.03 Returning a memory block, OSMemPut()
- •7.04 Obtaining status about memory partition, OSMemQuery()
- •7.05 Using memory partitions
- •7.06 Waiting for memory blocks from a partition
- •8.00 Development Tools
- •8.01 Directories and Files
- •8.02 INCLUDES.H
- •9.00 Development Tools
- •9.01 Directories and Files
- •9.02 INCLUDES.H
- •9.06 Memory requirements
- •9.07 Execution times
- •10.00 Directories and Files
- •10.01 INCLUDES.H
- •10.02.01 OS_CPU.H, Compiler specific data types
- •10.02.02 OS_CPU.H, OS_ENTER_CRITICAL() and OS_EXIT_CRITICAL()
- •10.02.03 OS_CPU.H, OS_STK_GROWTH
- •10.02.04 OS_CPU.H, OS_TASK_SW()
- •10.03.01 OS_CPU_A.ASM, OSStartHighRdy()
- •10.03.02 OS_CPU_A.ASM, OSCtxSw()
- •10.03.03 OS_CPU_A.ASM, OSIntCtxSw()
- •10.03.04 OS_CPU_A.ASM, OSTickISR()
- •10.04.01 OS_CPU_C.C, OSTaskStkInit()
- •10.04.02 OS_CPU_C.C, OSTaskCreateHook()
- •10.04.03 OS_CPU_C.C, OSTaskDelHook()
- •10.04.04 OS_CPU_C.C, OSTaskSwHook()
- •10.04.05 OS_CPU_C.C, OSTaskStatHook()
- •10.04.06 OS_CPU_C.C, OSTimeTickHook()
- •10.05 Summary
- •OSInit()
- •OSIntEnter()
- •OSIntExit()
- •OSMboxAccept()
- •OSMboxCreate()
- •OSMboxPend()
- •OSMboxPost()
- •OSMboxQuery()
- •OSMemCreate()
- •OSMemGet()
- •OSMemPut()
- •OSMemQuery()
- •OSQAccept()
- •OSQCreate()
- •OSQFlush()
- •OSQPend()
- •OSQPost()
- •OSQPostFront()
- •OSQQuery()
- •OSSchedLock()
- •OSSchedUnlock()
- •OSSemAccept()
- •OSSemCreate()
- •OSSemPend()
- •OSSemPost()
- •OSSemQuery()
- •OSStart()
- •OSStatInit()
- •OSTaskChangePrio()
- •OSTaskCreate()
- •OSTaskCreateExt()
- •OSTaskDel()
- •OSTaskDelReq()
- •OSTaskResume()
- •OSTaskStkChk()
- •OSTaskSuspend()
- •OSTaskQuery()
- •OSTimeDly()
- •OSTimeDlyHMSM()
- •OSTimeDlyResume()
- •OSTimeGet()
- •OSTimeSet()
- •OSTimeTick()
- •OSVersion()

.
msg = OSMboxPend(CommMbox, 10, &err); if (err == OS_NO_ERR) {
.
. /* Code for received message */
.
} else {
.
. /* Code for message not received within timeout */
.
}
.
.
}
}
OSMboxPost()
INT8U OSMboxPost(OS_EVENT *pevent, void *msg);
File |
Called from |
Code enabled by |
OS_MBOX.C |
Task or ISR |
OS_MBOX_EN |
OSMboxPost() is used to send a message to a task through a mailbox. A message is a pointer size variable and its use is application specific. If there is already a message in the mailbox, an error code is returned indicating that the mailbox is full. OSMboxPost() will then immediately return to its caller and the message will not be placed in the mailbox. If any task is waiting for a message at the mailbox then, the highest priority task waiting will receive the message. If the task waiting for the message has a higher priority than the task sending the message then, the higher priority task will be resumed and the task sending the message will be suspended. In other words, a context switch will occur.
Arguments
pevent is a pointer to the mailbox where the message is to be deposited into. This pointer is returned to your application when the mailbox is created (see OSMboxCreate()).
msg is the actual message sent to the task. msg is a pointer size variable and is application specific. You MUST never post a NULL pointer because this indicates that the mailbox is empty.
Returned Value
OSMboxPost() returns one of these two error codes:
1)OS_NO_ERR, if the message was deposited in the mailbox
2)OS_MBOX_FULL, if the mailbox already contained a message
Notes/Warnings
Mailboxes must be created before they are used.
Example

OS_EVENT *CommMbox;
INT8U CommRxBuf[100];
void CommTaskRx(void *pdata)
{
INT8U err;
pdata = pdata; for (;;) {
.
.
err = OSMboxPost(CommMbox, (void *)&CommRxBuf[0]);
.
.
}
}
OSMboxQuery()
INT8U OSMboxQuery(OS_EVENT *pevent, OS_MBOX_DATA *pdata);
File |
Called from |
Code enabled by |
OS_MBOX.C |
Task or ISR |
OS_MBOX_EN |
OSMboxQuery() is used to obtain information about a message mailbox. Your application must allocate an OS_MBOX_DATA data structure which will be used to receive data from the event control block of the message mailbox. OSMboxQuery() allows you to determine whether any task is waiting for message(s) at the mailbox, how many tasks are waiting (by counting the number of 1s in the .OSEventTbl[] field, and examine the contents of the contents of the message. You can use the tableOSNBitsTbl[] to find out how many ones are set in a given byte. Note that the size of .OSEventTbl[] is established by the #define constant OS_EVENT_TBL_SIZE (see ).
Arguments
pevent is a pointer to the mailbox. This pointer is returned to your application when the mailbox is created (see
OSMboxCreate()).
pdata is a pointer to a data structure of type OS_MBOX_DATA which contains the following fields:
void OSMsg; /* Copy of the message stored in the mailbox */ INT8U OSEventTbl[OS_EVENT_TBL_SIZE]; /* Copy of the mailbox wait list */ INT8U OSEventGrp;
Returned Value
OSMboxQuery() returns one of these two error codes:
1) OS_NO_ERR, if the call was successful

2) OS_ERR_EVENT_TYPE, if you didn’t pass a pointer to a message mailbox
Notes/Warnings
Message mailboxes must be created before they are used.
Example
In this example, we check the contents of the mailbox to see how many tasks are waiting for it.
OS_EVENT *CommMbox;
void Task (void *pdata) |
|
|
|
{ |
|
|
|
OS_MBOXDATA mbox_data; |
|
|
|
INT8U |
err; |
|
|
INT8U |
nwait; |
/* Number of tasks waiting on mailbox */ |
|
INT8U |
i; |
|
|
pdata = pdata; |
|
|
|
for (;;) { |
|
|
|
. |
|
|
|
. |
|
|
|
err = OSMboxQuery(CommMbox, &mbox_data); |
|
||
if (err == OS_NO_ERR) |
{ |
|
|
|
nwait = 0; |
/* Count # tasks waiting on mailbox |
*/ |
|
if (mbox_data.OSEventGrp != 0x00) { |
|
|
|
for (i = 0; i < OS_EVENT_TBL_SIZE; i++) { |
|
nwait += OSNBitsTbl[mbox_data.OSEventTbl[i]];
}
}
}
.
.
}
}
OSMemCreate()
OS_MEM *OSMemCreate(void *addr, INT32U nblks, INT32U blksize, INT8U *err);
File |
Called from |
Code enabled by |
OS_MEM.C |
Task or startup code |
OS_MEM_EN |
OSMemCreate() is used to c reate and initialize a memory partition. A memory partition contains a user-specified number of fixed-sized memory blocks. Your application can obtain one of these memory blocks and when done, release the block back to the partition.

Arguments
addr is the address of the start of a memory area that will be used to create fixed-sized memory blocks. Memory partitions can either be created using static arrays or malloc’ed during startup.
nblks contains the number of memory blocks available from the specified partition. You MUST specify at least 2 memory blocks per partition.
blksize specifies the size (in bytes) of each memory block within a partition. A memory block MUST be large enough to hold at least a pointer.
err is a pointer to a variable which will be used to hold an error code. OSMemCreate() sets *err to either:
1)OS_NO_ERR, if the memory partition was created successfully.
2)OS_MEM_INVALID_PART, if a free memory partition is not available.
3)OS_MEM_INVALID_BLKS, if you didn’t specify at least 2 memory blocks per partition.
4)OS_MEM_INVALID_SIZE, if you didn’t specify a block size that can at least contain a pointer variable.
Returned Value
OSMemCreate() returns a pointer to the created memory partition control block if one is available. If no memory partition control block is available, OSMemCreate() will return a NULL pointer.
Notes/Warnings
Memory partitions must be created before they are used.
Example
OS_MEM *CommMem;
INT8U CommBuf[16][128];
void main(void)
{
INT8U err; |
|
|
OSInit(); |
/* Initialize µC/OS-II |
*/ |
. |
|
|
. |
|
|
CommMem = OSMemCreate(&CommBuf[0][0], 16, 128, &err); |
|
|
. |
|
|
. |
|
|
OSStart(); |
/* Start Multitasking |
*/ |
}
OSMemGet()
void *OSMemGet(OS_MEM *pmem, INT8U *err);
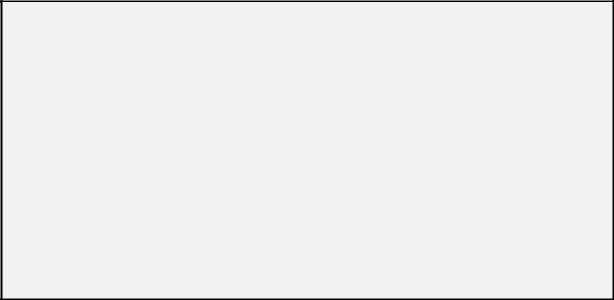
File |
Called from |
Code enabled by |
OS_MEM.C |
Task or ISR |
OS_MEM_EN |
OSMemGet() is used to obtain a memory block from a memory partition. It is assumed that your application will know the size of each memory block obtained. Also, your application MUST return the memory block (using OSMemPut()) when it no longer needs it. You can call OSMemGet() more than once until all memory blocks are allocated.
Arguments
pmem is a pointer to the memory partition control block that was returned to your application from the OSMemCreate() call.
err is a pointer to a variable which will be used to hold an error code. OSMemGet() sets *err to either:
1)OS_NO_ERR, if a memory block was available and returned to your application.
2)OS_MEM_NO_FREE_BLKS, if the memory partition doesn’t contain any more memory blocks to allocate.
Returned Value
OSMemGet() returns a pointer to the allocated memory block if one is available. If no memory block is available from the memory partition, OSMemGet() will return a NULL pointer.
Notes/Warnings
Memory partitions must be created before they are used.
Example
OS_MEM *CommMem;
void Task (void *pdata)
{
INT8U *msg;
pdata = pdata; for (;;) {
msg = OSMemGet(CommMem, &err); if (msg != (INT8U *)0) {
. |
/* Memory block allocated, use it. */ |
. |
|
} |
|
. |
|
. |
|
} |
|
}