
C# Bible - Jeff Ferguson, Brian Patterson, Jason Beres
.pdfThe string CompanyName property returns the company name associated with the application. This property is read-only and cannot be set. By default, this value is set to the name of the class containing the application's Main() method. If your application specifies a company name with the [AssemblyCompany] attribute, the value of that attribute is used as the value of the application's CompanyName property.
CurrentCulture
The CurrentCulture property enables you to work with the culture information for the application. This property can be read from and written to. The property has a class of type CultureInfo, which is a class defined by the .NET Framework and found in the System.Globalization namespace. The CultureInfo class contains methods and properties that enable your code to work with data specific to the cultural environment in which the application is executing. Information, such as the preferred date/time format, calendar settings, and numbering formats, are available from CultureInfo objects.
CurrentInputLanguage
The CurrentInputLanguage property enables you to work with the application's current input language. The property has a class of type InputLanguage, which is a class defined by the
.NET Framework and found in the System.Windows.Forms namespace. The InputLanguage class contains methods and properties that enable your code to work with the application's understanding of the physical keys on a keyboard and how they relate to characters that can be input into an application. Different language-specific versions of Windows map the physical keyboard keys to different language-specific characters, and the CurrentInputLanguage class specifies what this mapping looks like.
ExecutablePath
The string ExecutablePath property returns the path of the application's executable. This property is read-only and cannot be set.
LocalUserAppDataPath
The string LocalUserAppDataPath property references a path on the file system that the application can use to store file-based data that should be available to the user currently logged into the machine. This property is read-only and cannot be set. It is used for local users with operating system profiles on the local machine. Users who have roaming profiles that are used across the network use a separate property, called UserAppDataPath, to specify where their application data should be stored.
Like the CommonAppDataPath property, the local user data path points to a folder beneath the logged in user's documents path with a folder structure having the form CompanyName\ ProductName\ ProductVersion. The CompanyName, ProductName, and ProductVersion folder names are based on the values of the application properties of the same name. If you do not set these properties, the Application class provides reasonable defaults. If your code sets the Application class's CompanyName, ProductName, or ProductVersion properties, the folder names in the local user application data path change to reflect the values of those properties.
Like the common application data path, the product version folder path uses only the major and minor version numbers specified in your application, regardless of the number of values you set in your application's [AssemblyVersion] attribute. If your application uses an [AssemblyVersion] attribute with a value of 1.2.3.4, for example, the version number portion of the local user application data path is 1.2. The letter V always prefixes your application's major version number in the version number portion of the data path.
MessageLoop
The Boolean MessageLoop property returns True if a message loop exists for the application and False otherwise. This property is read-only and cannot be set. Because all WindowsForms applications need a message loop so that Windows messages can be routed to the correct form, WindowsForms applications return True for this property.
ProductName
The string ProductName property returns the product name associated with the application. This property is read-only and cannot be set. By default, this value is set to the name of the class containing the application's Main() method. If your application specifies a product name with the [AssemblyProduct] attribute, the value of that attribute is used as the value of the application's ProductName property.
ProductVersion
The string ProductVersion property returns the version number associated with the application. This property is read-only and cannot be set. By default, this value is set to 0.0.0.0. If your application specifies a version number with the [AssemblyVersion] attribute, the value of that attribute is used as the value of the application's ProductVersion property.
SafeTopLevelCaptionFormat
The string SafeTopLevelCaptionFormat property references a format string that the runtime applies to top-level window captions when the applications execute from an unsafe context.
Security is an integral part of the .NET Framework and the Common Language Runtime (CLR). The CLR honors the different security zones set up in Internet Explorer (Internet, Local Intranet, Trusted Sites, and Restricted Sites), and restricts runtime services to applications that run in untrusted zones. WindowsForms applications that are run from untrusted zones, such as the Internet zone, are adorned with a warning label that describes the application as originating from an untrusted location. The text of this warning label is formatted based on the string format template stored in the SafeTopLevelCaptionFormat property.
StartupPath
The string StartupPath property returns the path to the executable file that launched the application. This property returns only the path. It does not include the executable file's name. This property is read-only and cannot be set.
UserAppDataPath

The string UserAppDataPath property references a path on the file system that the application can use to store file-based data that should be available to the network user currently logged into the machine. This property is read-only and cannot be set. It is used for local users with operating system profiles on the network. Users who have local machine profiles that are not used across the network use a separate property, called LocalUserAppDataPath, to specify where their application data should be stored.
Like the CommonAppDataPath property, the local user data path points to a folder beneath the logged in user's documents path with a folder structure having the form CompanyName\ ProductName\ ProductVersion. The CompanyName, ProductName, and ProductVersion folder names are based on the values of the application properties of the same name. If you do not set these properties, the Application class provides reasonable defaults. If your code sets the Application class's CompanyName, ProductName, or ProductVersion properties, the folder names in the local user application data path change to reflect the values of those properties.
UserAppDataRegistry
The UserAppDataRegistry property returns a reference to an object of class RegistryKey. Like the CommonAppDataRegistry property, the property returns an object of type RegistryKey. The returned RegistryKey object references a key in the Registry that the application can use to store Registry data that should be available only to the current user of the application. This property is read-only and cannot be set.
Understanding Application methods
The Application class supports eight methods that you can call from your C# applications. These methods are described in the following sections.
AddMessageFilter
The AddMessageFilter() method adds a message filter to your application to monitor Windows messages as they are routed to their destinations. The message filter that you install into your application receives the Windows messages before they are sent along to your form. A message filter installed by AddMessageFilter() can choose to handle a message that is sent to it, and the filter can choose whether the message should be forwarded to your form.
Listing 21-4 shows how a message filter can be used in a WindowsForms application. The message handler looks for messages that announce when the left mouse button is clicked on the form.
Listing 21-4: Installing a Message Filter
using System;
using System.Windows.Forms;
public class BlockLeftMouseButtonMessageFilter : IMessageFilter
{
const int WM_LBUTTONDOWN = 0x201; const int WM_LBUTTONUP = 0x202;
public bool PreFilterMessage(ref Message m)

{
if(m.Msg == WM_LBUTTONDOWN)
{
Console.WriteLine("The left mouse button is down."); return true;
}
if(m.Msg == WM_LBUTTONUP)
{
Console.WriteLine("The left mouse button is up."); return true;
}
return false;
}
}
public class MainForm : Form
{
public static void Main()
{
MainForm MyForm = new MainForm(); BlockLeftMouseButtonMessageFilter MsgFilter = new
BlockLeftMouseButtonMessageFilter();
Application.AddMessageFilter(MsgFilter);
Application.Run(MyForm);
}
public MainForm()
{
Text = "Message Filter Test";
}
}
The AddMessageFilter() method takes one argument: an implementation of an interface called IMessageFilter. The IMessageFilter interface is defined by the .NET Framework and is found in the System.Windows.Forms namespace. The IMessageFilter interface declares one method:
public bool PreFilterMessage(ref Message m);
The PreFilterMessage() method takes as input a reference to an instance of a structure called Message. The Message structure describes a Windows message and contains the following properties:
•HWnd, which describes the handle of the window that should receive the message.
•LParam, which describes one piece of integer data that is sent with the message.
•Msg, which describes the integer ID number for the message. Each possible Windows message has its own integer ID.
•Result, which describes the value that should be returned to Windows in response to handling the message.
•WParam, which describes another piece of integer data that is sent with the message.
Listing 21-4 begins by declaring a class called BlockLeftMouseButtonMessage-Filter. This class implements the IMessageFilter interface. The implementation of the class's PreFilterMessage() method checks the message ID of the message passed in to the method. It
checks whether the ID states that the left mouse button has been pressed down. If so, a message reading The left mouse button is down. is written to the console. It then checks whether the ID states that the left mouse button has been released. If so, a message reading The left mouse button is up. is written to the console.
Note The BlockLeftMouseButtonMessageFilter class declares constants to give names to the Windows messages that the filter is looking for. The names start with WM, which stands for Windows Message, and match the names defined by Microsoft. All the available Windows messages, as well as their numeric values, are documented in the Microsoft Platform SDK documentation.
Implementations of the PreFilterMessage() method must return a Boolean value describing whether or not the message should be forwarded to your form after it passes through your filter. If the message does not need to be forwarded to your filter, then the filter should return the True value. If the message needs to be forwarded on to your filter, then the filter should return the False value. The message filter in Listing 21-4 returns True for the two messages that it handles and False for all other messages.
The Main() method in Listing 21-4 creates a new object of the BlockLeftMouseButtonMessageFilter class and uses it in a call to the Application object's AddMessageFilter() method. After the message filter is installed, the main form is created and executed.
You can see the message filter at work by compiling the code in Listing 21-4. When you run the code, the application's main form appears. When the form appears, move the mouse to point the cursor inside the form and click the left mouse button. The mouse click messages is written to the application's console.
DoEvents
The DoEvents() method processes all messages currently in the application's Windows message queue. The method does not take any arguments and does not return a value. You may want to call this method if you want to make sure that waiting Windows messages are sent along to your form while you are doing other work.
Suppose, for example, that you create a form that performs a lengthy calculation. If another window is moved in front of your form while the calculation is performed, Windows sends your application a Windows message stating that your form needs to be repainted. However, because your code is performing your lengthy calculation, the repaint message will be sitting in your application's message queue; after all, your code is busy performing calculations and is not processing messages. You can call the DoEvents() method at certain points during your processing to ensure that waiting Windows messages are handled when your code is busy with other work.
Exit
The Exit() method forcibly ends the application. The method informs your application's message queue that it should terminate, and your forms are closed after the last Windows messages in the queue are processed. It does not take any arguments and does not return a value.

In simple cases, your code does not need to call the Exit() method. The default Windows form includes a Close box in the upper-right corner of the form, and clicking that box sends a quit message to your Windows message queue. You may want to call Exit(), however, if your form includes a control, such as a button or a menu item, that should exit the application when it is selected.
ExitThread
The ExitThread() method exits the message loop and closes all forms on the current thread. The method does not take any arguments and does not return a value.
If your WindowsForms application contains only one thread (and most do), then calling ExitThread() is the same as calling Exit(). If, however, your application uses multiple threads, then the two methods behave differently. The ExitThread() method shuts down one thread but allows the other threads to continue running. The Exit() method, however, shuts down all threads at once. Like all Windows processes, WindowsForms applications continue running until the last thread ends.
OleRequired
The OleRequired() method initializes OLE on the application's current thread. If your application will be working with a COM-related technology, such as COM, DCOM, ActiveX, or OLE, you must call this application method before you use COM.
The method does not take any arguments, but it returns a value from an enumeration called ApartmentState that describes the type of COM apartment that the thread entered. The ApartmentState enumeration is defined in a .NET Framework namespace called System.Threading, and it can have one of the following values:
•STA is returned when the CLR opts to initialize COM for your thread by entering a single-threaded apartment.
•MTA is returned when the CLR opts to initialize COM for your thread by entering a multi-threaded apartment.
OnThreadException
The OnThreadException() method raises a ThreadException event. The event can be caught by an OnThreadException() event handler installed into the Application object.
Listing 21-5 shows how a thread exception can be used in a WindowsForms application.
Listing 21-5: Working with Thread Exceptions
using System;
using System.Threading; using System.Windows.Forms;
public class BlockLeftMouseButtonMessageFilter : IMessageFilter
{
const int WM_LBUTTONDOWN = 0x201;
public bool PreFilterMessage(ref Message m)

{
if(m.Msg == WM_LBUTTONDOWN)
{
Exception LeftButtonDownException;
LeftButtonDownException = new Exception("The left mouse button was pressed.");
Application.OnThreadException(LeftButtonDownException); return true;
}
return false;
}
}
public class ApplicationEventHandlerClass
{
public void OnThreadException(object sender, ThreadExceptionEventArgs e)
{
Exception LeftButtonDownException;
LeftButtonDownException = e.Exception; Console.WriteLine(LeftButtonDownException.Message);
}
}
public class MainForm : Form
{
public static void Main()
{
ApplicationEventHandlerClass AppEvents = new ApplicationEventHandlerClass();
MainForm MyForm = new MainForm(); BlockLeftMouseButtonMessageFilter MsgFilter = new
BlockLeftMouseButtonMessageFilter();
Application.AddMessageFilter(MsgFilter); Application.ThreadException += new
ThreadExceptionEventHandler(AppEvents.OnThreadException);
Application.Run(MyForm);
}
public MainForm()
{
Text = "Application Exception Test";
}
}
Listing 21-5 is similar to Listing 21-4, as it includes a message handler that looks for messages announcing when the left mouse button is clicked on the application's form. The difference is that an exception is thrown in Listing 21-5 when the left mouse button down message is received. The message handler constructs a new Exception object and throws it using the Application object method OnThreadException().
The code in Listing 21-5 also includes an application event handler, which is implemented in a class called ApplicationEventHandlerClass. The class handles the OnThreadException()

event, and the application's main method installs the event handler using the Application object's ThreadException property.
The thread exception handler installed in the ApplicationEventHandlerClass class extracts the exception from the handler's ThreadExceptionEventArgs object and prints the exception's message to the console. When you run the code in Listing 21-5, the application's main form appears. When the form appears, move the mouse to point the cursor inside the form and click the left mouse button. The message handler throws an exception, and the application's exception handler prints exception messages to the application's console.
RemoveMessageFilter
The RemoveMessageFilter() method removes a message filter installed by the AddMessageFilter() method. It removes the message filter from the message pump of the application. The RemoveMessageFilter() method takes one argument: an implementation of an interface called IMessageFilter. This argument should reference a class that implements IMessageFilter and has already been used in a call to AddMessageFilter(). Listing 21-6 shows how this method works.
Listing 21-6: Removing an Installed Message Filter
using System;
using System.Windows.Forms;
public class BlockLeftMouseButtonMessageFilter : IMessageFilter
{
const int WM_LBUTTONDOWN = 0x201;
public bool PreFilterMessage(ref Message m)
{
if(m.Msg == WM_LBUTTONDOWN)
{
Console.WriteLine("The left mouse button is
down.");
Application.RemoveMessageFilter(this); return true;
}
return false;
}
}
public class MainForm : Form
{
public static void Main()
{
MainForm MyForm = new MainForm(); BlockLeftMouseButtonMessageFilter MsgFilter = new
BlockLeftMouseButtonMessageFilter();
Application.AddMessageFilter(MsgFilter);
Application.Run(MyForm);
}
public MainForm()
{
Text = "Message Filter Removal Test";
}

}
The code in Listing 21-6 installs a message filter that looks for the left mouse button down message, just as Listing 21-4 does. The difference here is that the implementation of the message filter in Listing 21-6 removes the message filter when the message is received.
When you run the code in Listing 21-6, note that you get only one message written to the console, regardless of the number of times you click the left mouse button while the mouse pointer is over the form. This is because the message filter is removed from the application when the first message is received; and because the message filter is removed, no further messages will be detected. Messages are still sent to your form, but the code in Listing 21-6 removes the object that gets the first shot at detecting the messages after the object is removed from the application's list of event filters.
Tip Listing 21-6 uses the keyword this as the parameter to the call to the Application object's RemoveMessageFilter() method. Remember that the this keyword is used to reference the object whose code is being executed. You can think of the statement in Listing 21-6 that calls RemoveMessageFilter() as making the statement "remove the reference to this message filter from the Application object."
Run
The Run() method starts the Windows message loop for an application. All the listings in this chapter have used the Run() method, which accepts a reference to a form object as a parameter. You should already be familiar with the way the Run() method works.
Adding Controls to the Form
The default form created by WindowsForms applications isn't very interesting. It contains a caption bar, a default icon, and the Windows standard Minimize, Maximize and Close buttons. Forms found in real-world applications include controls, such as buttons, text boxes, labels, and the like. This section explains how you can add controls to forms in your C# applications.
In this section, you take a look at how controls are supported from within the .NET Framework. The Framework contains .NET class support for the controls built into the Windows operating system, and the examples in this section will illustrate their use in building WindowsForms applications that use controls on the application's forms.
Understanding the control class hierarchy
The .NET Framework includes several classes in the System.Windows.Forms namespace that encapsulate the behavior of a control. User-interface elements, such as buttons, list boxes, check boxes, and the like, are all represented by a control class.
All of these classes inherit from a base class called Control. Figure 21-2 shows the class hierarchy for the control classes. All user interface controls share some functionality: They must all be able to position themselves on their parent container and manage their foreground
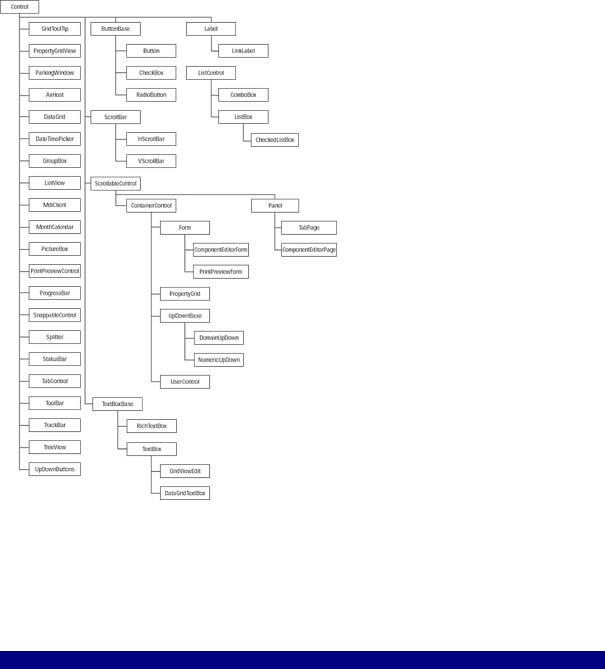
and background colors. Because all the controls share this behavior, it makes sense to encapsulate it in a base class and derive the control-specific functionality in derived classes. The authors of the control classes found in the .NET Framework took this design approach when building the classes.
Figure 21-2: Control class hierarchy
Working with controls on a form
Listing 21-7 shows a WindowsForm application that includes a button. The button displays a message in a message box when clicked.
Listing 21-7: Working with a Button on a Form
using System;
using System.Drawing; using System.Windows.Forms;
public class MainForm : Form
{
public static void Main()
{
MainForm MyForm = new MainForm();
Application.Run(MyForm);