
Lectures / lecture2_1
.pdf
Arrays and for-each Loop
1public class ArrayOperations {
2public static void main(String args[]){
4 |
String[] names = new String[3]; |
|
5 |
|
|
6 |
names[0] = "Blue Shirt"; |
|
7 |
names[1] = "Red Shirt"; |
Arrays are objects. |
8 |
names[2] = "Black Shirt"; |
Array objects have a |
9 |
|
final field length. |
|
|
|
10 |
int[] numbers = {100, 200, 300}; |
|
11 |
|
|
12 |
for (String name:names){ |
|
13 |
System.out.println("Name: " + name); |
|
14 |
} |
|
15 |
|
|
16 |
for (int number:numbers){ |
|
17 |
System.out.println("Number: " + number); |
|
18 |
} |
|
19}
20}
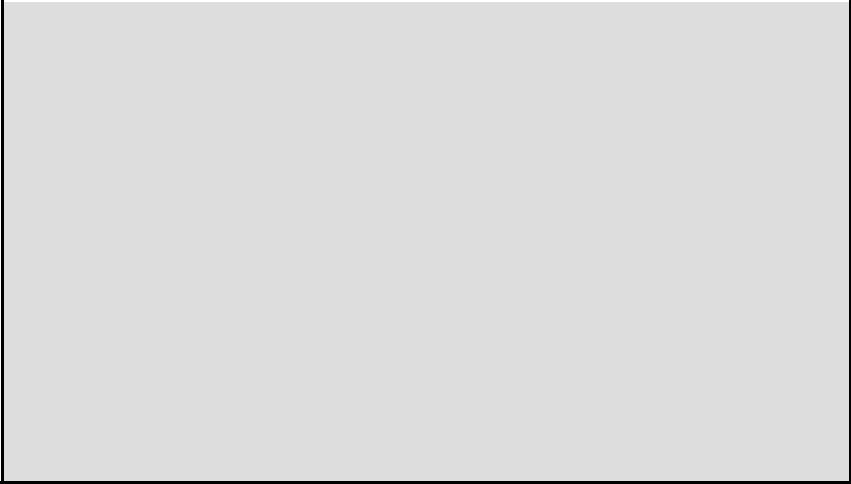
|
while Loop |
|
|
1 |
public class WhileLoop { |
2 |
|
3 |
public static void main(String args[]){ |
4 |
|
5 |
int i = 0; |
6 |
int[] numbers = {100, 200, 300}; |
7 |
|
8 |
while (i < numbers.length ){ |
9 |
System.out.println("Number: " + |
|
numbers[i]); |
10 |
i++; |
11 |
} |
12}
13}

|
do{} while() Loop |
|
|
1 |
public class WhileLoop { |
2 |
|
3 |
public static void main(String args[]){ |
4 |
|
5 |
int i = 0; |
6 |
int[] numbers = {100, 200, 300}; |
7 |
do{ |
8 |
System.out.println("Number: " + |
|
numbers[i]); |
9 |
i++; |
10 } while (i < numbers.length );
11}
12}
13 }

String switch Statement
1public class SwitchStringStatement {
2public static void main(String args[]){
4String color = "Blue";
5String shirt = " Shirt";
7switch (color){
8 |
case "Blue": |
9 |
shirt = "Blue" + shirt; |
10 |
break; |
11 |
case "Red": |
12 |
shirt = "Red" + shirt; |
13 |
break; |
14 |
default: |
15 |
shirt = "White" + shirt; |
16 |
} |
17 |
|
18 |
System.out.println("Shirt type: " + shirt); |
19}
20}
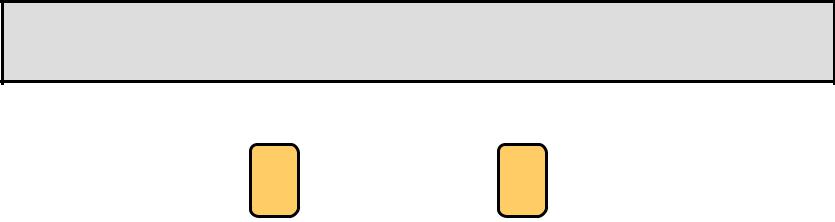
Java Is Pass-By-Value
•The Java language (unlike C++) uses pass-by- value for all assignment operations.
–To visualize this with primitives, consider the following:
int x = 3; int y = x;
– The value of x is copied and passed to y:
3 |
3 |
x y copy the value of x
–If x is later modified (for example, x = 5;), the value of y remains unchanged.
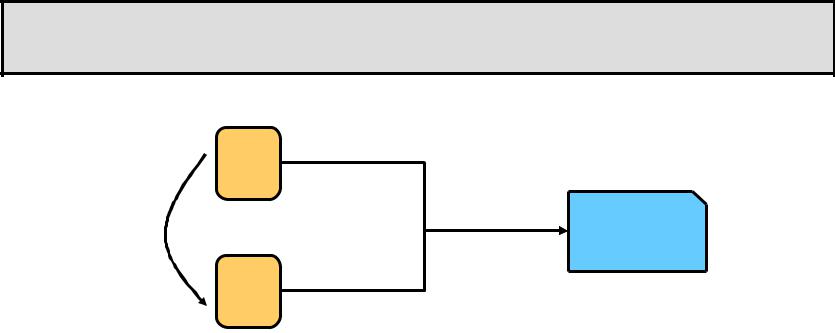
Pass-By-Value for Object References
For Java objects, the value of the right side of an assignment is a reference to memory that stores a Java object.
Employee x = new Employee(); Employee y = x;
– The reference is some address in memory.
|
42 |
memory address = 42 |
y = x; |
x |
Employee |
|
object |
|
|
|
|
|
42 |
|
y
–After the assignment, the value of y is the same as the value of x: a reference to the same Employee object.

Objects Passed as Parameters
–Whenever a new object is created, a new reference is created. Consider the following code fragments:
Employee x = new Employee(); foo(x);
public void foo(Employee e) { e = new Employee();
e.setSalary (1_000_000.00); // What happens to x here?
}
–The value of x is unchanged as a result of the method call foo:
x |
42 |
Employee |
|
object |
|||
|
|
||
e |
99 |
Employee |
|
|
|
object |

How to Compile and Run
Java class files must be compiled before running them. To compile a Java source file, use the Java compiler (javac).
javac –cp <path to other classes> -d <complier output path> <path to source>.java
–You can use the CLASSPATH environment variable to the directory above the location of the package hierarchy.
–After compiling the source .java file, a .class file is generated.
–To run the Java application, run it using the Java interpreter (java):
java –cp <path to other classes> <package name>.<classname>

Compiling a Program
1.Go to the directory where the source code files are stored.
2.Enter the following command for each .java file you want to compile.
– Syntax:
javac filename
– Example:
javac Shirt.java

Executing (Testing) a Program
1.Go to the directory where the class files are stored.
2.Enter the following for the class file that contains the main method:
Syntax:
java classname
Example:
java ShirtTest