
Lectures / lecture2_2
.pdf
Java Programming Language
Lecture 3.
Using Loop Constructs
9 - 1 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |

Topics
•Create a while loop
•Develop a for loop
•Nest a for loop and a while loop
•Use an array in a for loop
•Code and nest a do/while loop
•Compare loop constructs
9 - 2 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |

Loops
Loops are frequently used in programs to repeat blocks of statements until an expression is false.
There are three main types of loops:
•while loop: Repeats while an expression is true
•do/while loop: Executes once and then continues to repeat while true
•for loop: Repeats a set number of times
9 - 3 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |
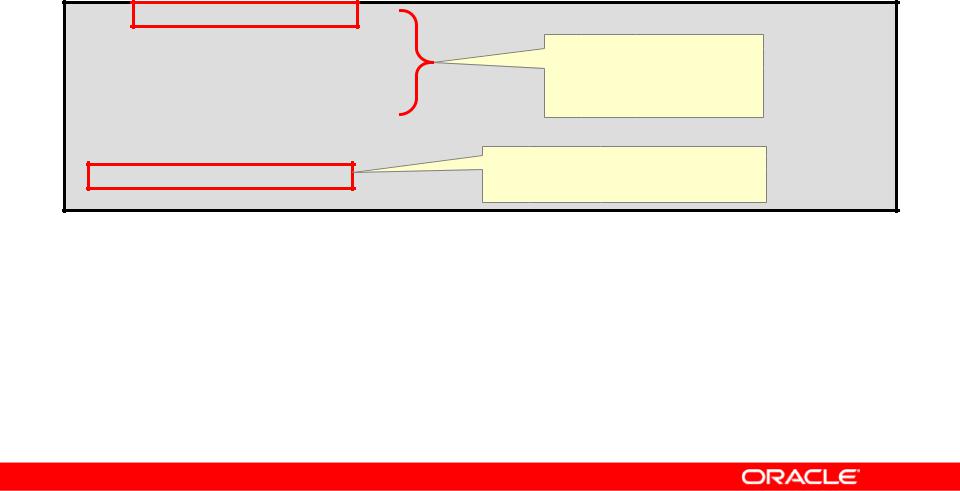
Creating while Loops
Syntax:
while (boolean_expression) {
code_block;
} // end of while construct // program continues here
If the boolean expression is true, this code block executes.
If the boolean expression is false, program continues here.
9 - 4 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |

while Loop in Elevator
public void setFloor() {
//Normally you would pass the desiredFloor as an argument to the
//setFloor method. However, because you have not learned how to
//do this yet, desiredFloor is set to a specific number (5)
// below. |
|
If the boolean |
||
|
|
|
expression returns |
|
int desiredFloor = 5; |
|
true, execute the |
||
|
|
|
while loop. |
|
while ( |
currentFloor != desiredFloor |
){ |
||
|
||||
if (currentFloor < desiredFloor) { |
|
|
||
goUp(); |
|
|
||
} |
|
|
|
|
else { |
|
|
||
goDown(); |
|
|
||
} |
|
|
|
|
} |
|
|
|
|
} |
|
|
|
9 - 5 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |
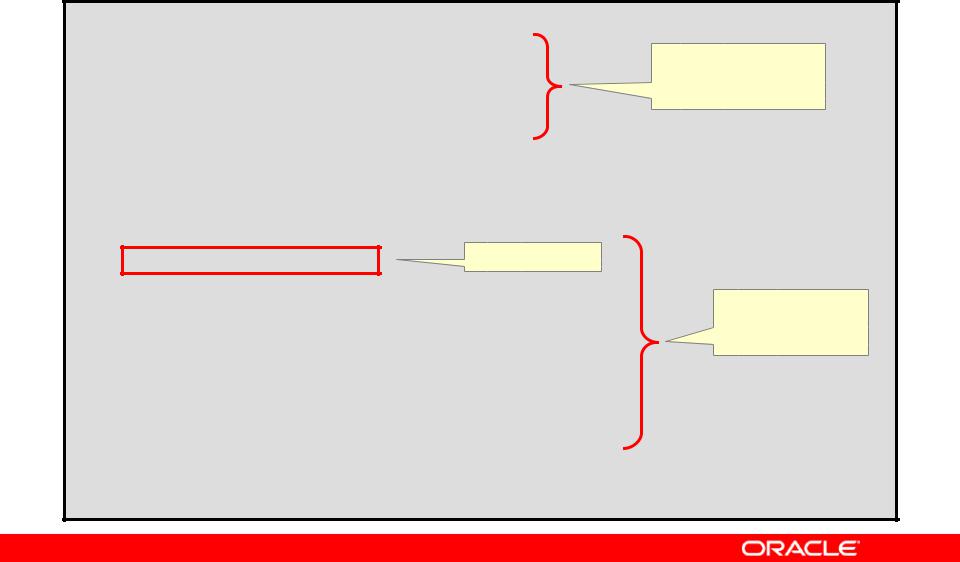
Types of Variables
public class Elevator {
public boolean doorOpen=false; public int currentFloor = 1; public final int TOP_FLOOR = 10; public final int BOTTOM_FLOOR = 1;
... < lines of code omitted > ...
public void setFloor() { |
|
int desiredFloor = 5; |
Local variable |
while ( currentFloor != desiredFloor ){ if (currentFloor < desiredFloor) {
goUp(); } else {
goDown();
}
} // end of while loop } // end of method
}// end of class
Instance variables (fields)
Scope of desiredFloor
9 - 6 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |
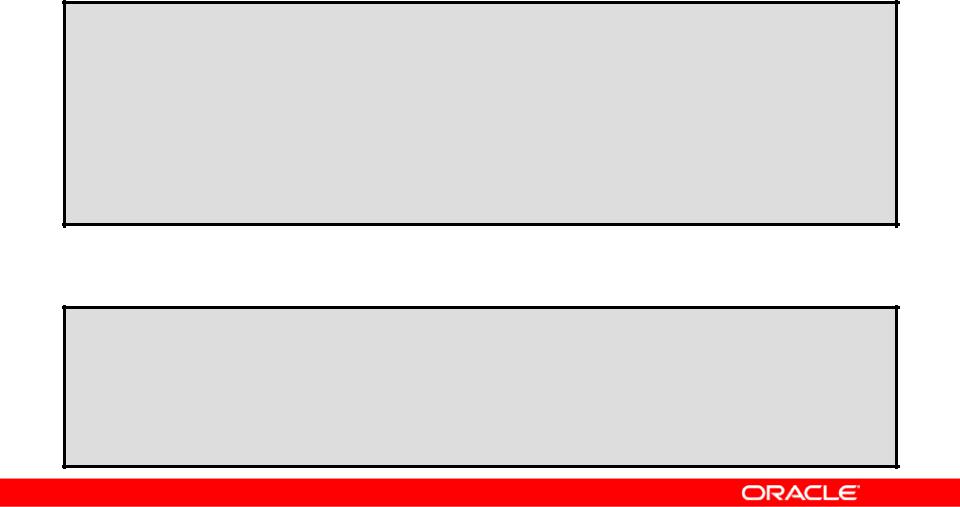
while Loop: Example 1
Example:
float square = 4; // number to find sq root of float squareRoot = square; // first guess
while (squareRoot * squareRoot - square > 0.001) { // How accurate? squareRoot = (squareRoot + square/squareRoot)/2; System.out.println("Next try will be " + squareRoot);
}
System.out.println("Square root of " + square + " is " + squareRoot);
Result:
Next try will be 2.5
Next try will be 2.05
Next try will be 2.0006099
Next try will be 2.0
The square root of 4.0 is 2.0
9 - 7 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |
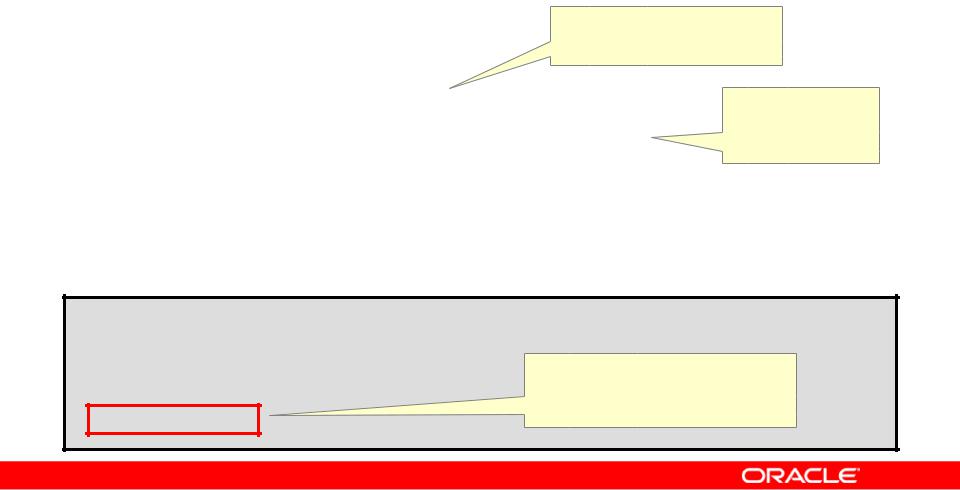
while Loop: Example 2
Example:
int initialSum = 500; |
|
|
|
|
|
||
int interest = 7; |
// per cent |
Check if money has |
|||||
int years = 0; |
|
|
doubled yet. |
|
|||
int currentSum = initialSum * 100; // Convert to pennies |
|||||||
while ( |
currentSum <= 100000 |
) { |
|
|
If not doubled, |
||
|
|
|
|
|
|
|
add another |
|
currentSum += currentSum * interest/100; |
|
|||||
|
|
|
|||||
|
years++; |
|
|
|
|
year’s interest. |
|
|
|
|
|
|
|
||
|
System.out.println("Year " + years + ": " + currentSum/100); |
||||||
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Result: |
|
|
|
|
|
... < some results not shown > ...
Year 9: 919
Year 10: 983
Year 11: 1052
The while loop iterates 11 times before the boolean test evaluates to true.
9 - 8 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |
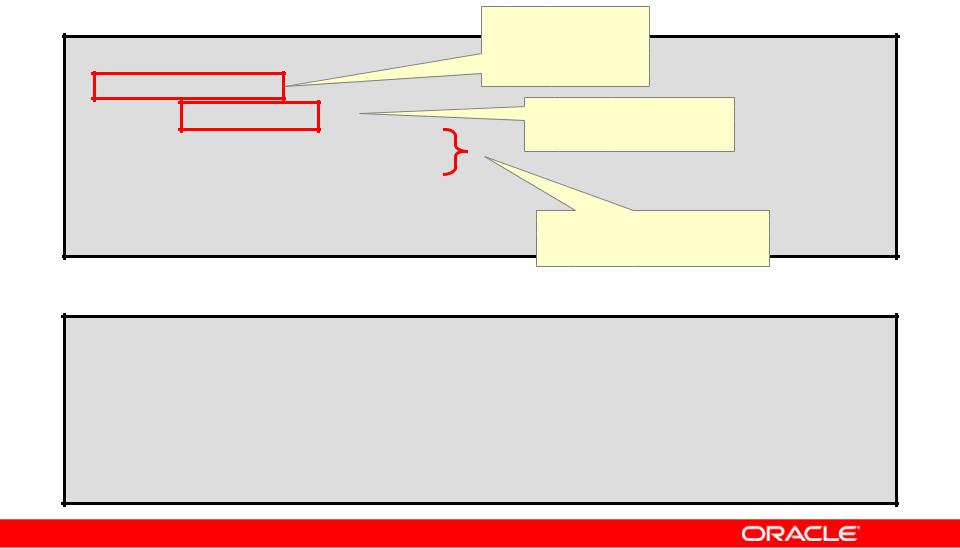
while Loop with Counter
Example: |
|
Declare and |
|
System.out.println(" |
/*"); |
initialize a |
|
counter variable. |
|||
|
|
||
int counter = 0; |
|
|
|
while ( counter < 4 ) { |
|
Check to see if counter |
|
System.out.println(" |
*"); |
has exceeded 4. |
|
|
|||
counter ++; |
|
|
|
} |
|
|
|
System.out.println(" |
*/"); |
Print an asterisk and |
|
|
|
increment the counter. |
|
Output: |
|
|
|
/* |
|
|
|
* |
|
|
|
* |
|
|
|
* |
|
|
|
* |
|
|
|
*/ |
|
|
9 - 9 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |

Topics
•Create a while loop
•Develop a for loop
•Nest a for loop and a while loop
•Use an array in a for loop
•Code and nest a do/while loop
•Compare loop constructs
9 - 10 |
Copyright © 2011, Oracle and/or its affiliates. All rights reserved. |