
ДискретнаяМатематика / Student Solutions Manual / chapter 9
.pdf5.Write a divide-and-conquer algorithm to nd the largest and smallest element in a set with 2k elements for some k > 0. Determine the complexity of your algorithm.
5. Arrange the elements (using 2k 1 comparisons) so that every element in an odd numbered position is larger than or equal to the element in the
adjacent even numbered position (1 with 2, 3 with 4, etc.). Now nd the largest element in the odd numbered position using 2k 2 1 comparisons.
Similarly nd the smallest element in the even numbered positions using 2k 2 1 comparisons. The total number of comparisons is 2n 3: The algorithm can also be implemented using a divide and conquer strategy.
7.Determine the di erence in performance between the methods described for calculating the Fibonacci numbers.
a)Directly implement the relation Fn = Fn 1 + Fn 2:
b)De ne a recursive function that has Fn 1 and Fn 2 passed as parameters when calculating Fn:
c)Use the relations
F2n = (Fn + 2Fn 1) Fn
F2n 1 = Fn2 + Fn 12
F2n 2 = (2Fn Fn 1) Fn 1 to generate pairs of Fibonacci numbers.
7. (a) The di culty with code such as if n 1 then F ib = n else F ib = F ib(n 1) + F ib(n 2) is that the same Fibonacci number will be calculated several times. Draw a computation tree for F ib(4) to see F ib(2) is evaluated twice and F ib(1) is evaluated three times. It is obviously possible to have an O(n) solution by storing the numbers as they are generated and then looking them up when they are needed again. It is not necessary that a recursive procedure be so ine cient. Consider if i = n then F := this else F = F (i+1; this; last+this) where last is a parameter passed into the function. The calling routing can have the form if n < 1 then F ib = n else F ib = F (1; 0; 1): This is also an O(n) algorithm. Using the identities you should be able to construct an O(log(n)) procedure.
7. (b) Solution Required
7. (c) Solution Required
9.12 End of Chapter Materials
Starting to Review
1.For n = 0; 1; 2; and 3, show that yn = 2 3n is a solution for y0 = 2 and yn = 3yn 1 for n > 0:
1.
y0 |
= |
2 |
30 = 2 |
|
|
y0 = 2 |
|
y1 |
= |
2 |
31 = 6 |
|
|
y1 = 3y0 = 3 2 = 6 |
|
y2 |
= |
2 |
32 = 18 |
|
= y2 = 3y1 = 3 6 = 18 |
||
y3 |
= |
2 |
33 = 54 |
y3 = 3y2 = 3 18 = 54
3. Find the value of an for n = 0; 1; 2; 3; 4; 5 where an = 3an 1 2an 2 for
n > 2 where a0 = 2 and a1 = 3: |
|
|
|
|
|||
3. |
|
|
|
|
|
|
|
a0 |
= |
2 |
|
|
|
|
|
a1 |
= |
3 |
|
|
|
|
|
a2 |
= |
3a1 2a0 = |
3 |
( 3) 2 |
(2) = 13 |
||
a3 |
= |
3a2 2a1 = |
3 |
( 13) |
2 |
( 3) = 33 |
|
a4 |
= |
3a3 3a2 |
= |
3 |
( 33) |
2 |
( 13) = 73 |
a5 |
= |
3a4 2a3 |
= |
3 |
( 73) |
2 |
( 33) = 80 |
5.Find the characteristic equation for the recurrence relation Fn = Fn 1 + 3Fn 2 6Fn 3:
5. x3 x2 3x + 6 = 0
7.Let an = A2n + B3n be the general solution of a recurrence relation with initial conditions a0 = 0 and a1 = 1: Find the particular solution that satis es these initial conditions.
7. an = 2n + 3n

9.Solve the Lucas recurrence relation: Ln = Ln 1 + Ln 2 where L0 = 2 and L1 = 1: (A de nition of Lucas numbers is given in Exercise 25 in Section 1.9.)
9. |
|
+ p |
|
! |
n |
|
p |
|
! |
n |
|
|
|
|
|
||||||
|
1 |
5 |
1 |
5 |
||||||
Ln = |
|
|
+ |
|
|
|
||||
|
2 |
|
|
2 |
|
Review Questions
1. Prove that the sequence bn = C where C is a constant and n 2 N, is a solution to the recurrence relation an an 1 = 0 for n 1 where a0 = C.
1. Since bn an 1 = nn bn 1 = C C = 0, it is clear that bn is a solution for n 1.
3.Prove that the sequence bn = C1 + C22n, where C1 and C2 are constants is a solution to the recurrence relation an 3an 1 + 2an 2 = 0:
3.
an 3an 1 + 2an 2 = C1 + C22n 3(C1 + C22n 1 + 2(C1 + C22n 2
=C1 3C1 + 2C1 + C2(2n 32n 1 + 22n 2)
=C2(2n 2n 2n 1 + 2n 1)
=0
Therefore, bn = C1 + C22n is a solution of an 3an 1 + 2an 2.
5.Conjecture and prove a formula for the sum of the elements of the Lucas sequence given
L0 + L1 + L2 + + Ln;
where n 2 N.
5.L0 + L1 + + Ln = Ln+2 1
7.Solve an = an 1 + n(n 1) for n 1 where a0 = 1:
7. an = |
n |
n |
i=1 i2 |
i=1 i + 1 |

9. Verify by induction that a function of the form an = A1n + A2 where A1; A2 2 R is a solution to an = dan=d + e where n = dk :
9. For k = 0, we have n = 1 and a1 = A1 + A2, which has the required form A1 n + A2. Assume the result is true for n = dk and show it is true for n = dk+1. adk+1 = dadk + e = d(A1dk + A2) + e by the induction hypothesis. Simplifying the algebra gives an = A1n+dA2 +e: Since dA2 +e is a constant, the result follows.
11.Find a recurrence relation for the number of ways to make a pile of n chips using garnet, gold, red, white, and blue chips such that no two gold chips are together.
11. T (n) = (n 1)T (n 2) + (n 1)T (n 1) for n 2, where T (0) = 0 and T (1) = 5.
Using Discrete Mathematics in Computer Science
1.Find a recurrence relation for the sum of the rst n natural numbers for any n 2 N. Solve the recurrence relation. (This closed form evaluated at n 1 represents the complexity of selection sort for n elements that was described in Section 1.7.1.)
1.T (n) = T (n 1) + n 1 for n > 1, where T (1) = 0.
3.(a) Find a recurrence relation to describe the complexity of the recursive code for computing the nth Fibonacci number.
ALGORITHM: Compute Fn
INPUT: n 2 N OUTPUT: Fn recursiveF ibonacci(n) if n = 0 then
recursiveF ibonacci(0) = 1
else
if n = 1 then
recursiveF ibonacci(1) = 1
else
recursiveF ibonacci(n) = recursiveF ibonacci(n 1) +recursiveF ibonacci(n 2)
(b) Find a closed form for the function found in part (a).
3. (a) T (n) = T (n 1) + T (n 2) for n 2, where T (0) = T (1) = 1.
3. (b) See Section 9.4.2 (Solving the Fibonacci Recurrence).

5.Find and solve a recurrence relation that describes the complexity of each of the blocks of code shown. The blocks of code evaluate a polynomial at s point. Compare your answers to what you found for Exercise 6 in Section 5.6.4.
(a)
ALGORITHM: Evaluate P (x) = a0 + a1x + a2x2 + + anxn at x0
INPUT: n 2 N, the coe cients of P stored in a[0..n], and a real number x0
OUTPUT: P (x0)
P oly = a[0] x = 1
for i = 1 to n x = x0 x
P oly = a[i] x + P oly print P oly
(b)
ALGORITHM: Evaluate P (x) = a0 + a1x + a2x2 + + anxn at x0
INPUT: n 2 N, the coe cients of P stored in a[0::n], and a real number x0
OUTPUT: P (x0)
P oly = a[0] for i = 0 to n
x = x0
for j = 1 to i
x = x x0
P oly = P oly + a[i] x print P oly
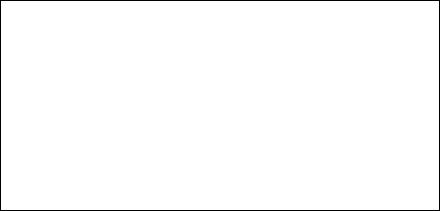
(c) The code shown implements Horner's algorithm.
ALGORITHM: Evaluate P (x) = a0 + a1x + a2x2 + + anxn at x0
INPUT: n 2 N, the coe cients of P stored in a[0..n], and a real number x0
OUTPUT: P (x0)
P oly = x0 a[n] + a[n 1]
= down to means to subtract 1 each time through the loop until i < 0 */
for i = n 2 down to 0
P oly = P oly x0 + a[i] print P oly
5. (a) P (0) = 0 and P (n) = 2n for n 1
Pn
5. (b) P (0) = 0 and P (n) = j=1 j
5.(c) P (1) = 1 and P (n) = P (n 1) + 1
7.(a) Find a recurrence relation for the number of binary decision trees on a set of n distinct numbers. (Hint: See Figure 6-42.)
(b) Use the recurrence relation found in part (a) to determine the number of binary search trees there are for 5, 7, 9, and 10 items.
7.(a) Project
7. (b) Project