
Asp Net 2.0 Security Membership And Role Management
.pdf

SqlRoleProvider
Role Manager ships with a number of different providers in the Framework: WindowsToken RoleProvider, which was covered at the end of the previous chapter; SqlRoleProvider, which is the topic of this chapter; and AuthorizationStoreRoleProvider, which is discussed in the next chapter. SqlRoleProvider is already configured in machine.config as the default provider for the Role Manager feature. As with SqlMembershipProvider, SqlRoleProvider is the reference provider for the feature because it implements all of the functionality defined on the
RoleProvider base class.
This chapter will cover the following areas of the SqlRoleProvider:
The database schema used by the SqlRoleProvider
Database security and trust level requirements for the provider, including how to configure the provider for use in partially trusted non-ASP.NET environments
Using the SqlRoleProvider with Windows-authenticated websites
Extending the provider to support “run-with-limited-roles” scenarios
Leveraging role data for authorization checks in the data layer
Supporting multiple applications with a single provider
SqlRoleProvider Database Schema
The database schema contains tables, views, and stored procedures used by the provider. As with the Membership feature, SqlRoleProvider’s schema integrates with the common set of tables covered in Chapter 11. This allows you to use SqlMembershipProvider for authentication and then use SqlRoleProvider to associate one or more roles with the users already registered in the Membership feature. Keying off of the common tables also allows SqlRoleProvider to be used in conjunction with the other SQL-based providers (SqlProfileProvider and SqlPersonalizationProvider)


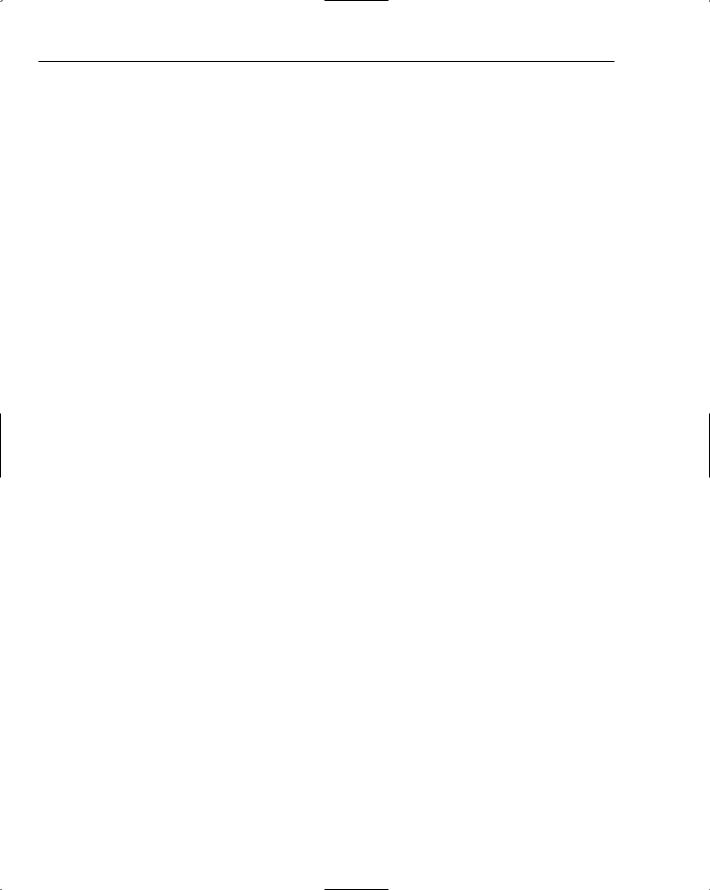



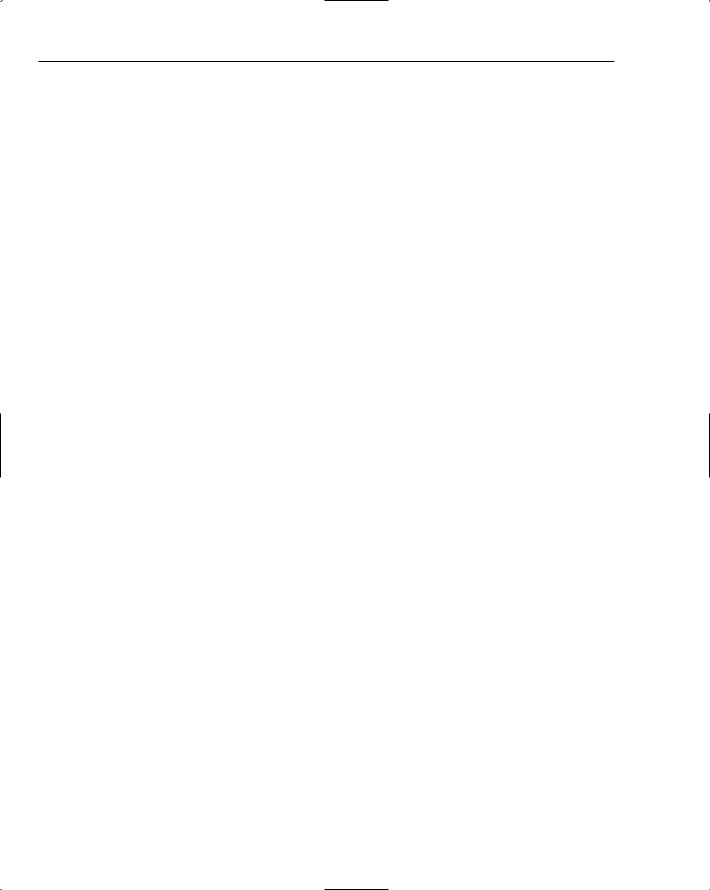
