
Pro ASP.NET 2.0 In CSharp 2005 (2005) [eng]
.pdf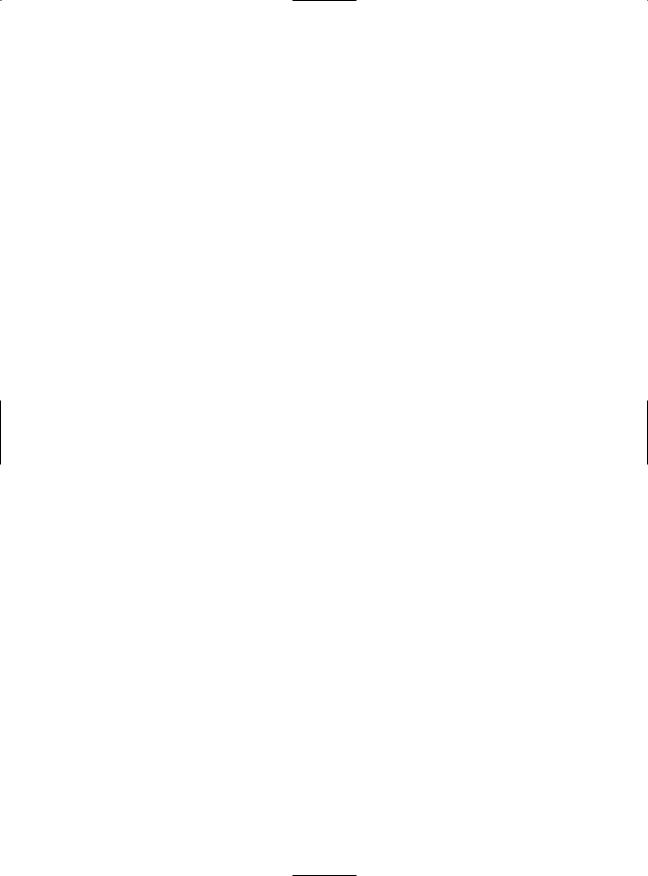
xx |
■C O N T E N T S |
|
|
|
|
Using the SqlProfileProvider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
806 |
|
|
Creating the Profile Tables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
807 |
|
|
Configuring the Provider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
809 |
|
|
Defining Profile Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
810 |
|
|
Using Profile Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
811 |
|
|
Profile Serialization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
812 |
|
|
Profile Groups . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
814 |
|
|
Profiles and Custom Data Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
814 |
|
|
The Profiles API . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
818 |
|
|
Anonymous Profiles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
820 |
|
|
Building a Shopping Cart . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
822 |
|
|
The Shopping Cart Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
823 |
|
|
The Test Page . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
826 |
|
|
Multiple Selection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
828 |
|
|
Custom Profiles Providers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
829 |
|
|
The Custom Profiles Provider Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
829 |
|
|
Designing the FactoredProfileProvider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
831 |
|
|
Coding the FactoredProfileProvider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
832 |
|
|
Testing the FactoredProfileProvider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
836 |
|
|
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
838 |
|
■CHAPTER 25 |
Cryptography . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
839 |
|
|
Encrypting Data: Confidentiality Matters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
839 |
|
|
The .NET Cryptography Namespace . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
840 |
|
|
Understanding the .NET Cryptography Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
843 |
|
|
Symmetric Encryption Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
844 |
|
|
Asymmetric Encryption . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
845 |
|
|
The Abstract Encryption Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
846 |
|
|
The ICryptoTransform Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
847 |
|
|
The CryptoStream Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
847 |
|
|
Encrypting Sensitive Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
848 |
|
|
Managing Secrets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
849 |
|
|
Using Symmetric Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
850 |
|
|
Using Asymmetric Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
855 |
|
|
Encrypting Sensitive Data in a Database . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
858 |
|
|
Encrypting the Query String . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
861 |
|
|
Wrapping the Query String . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
862 |
|
|
Creating a Test Page . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
864 |
|
|
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
866 |
|
■CHAPTER 26 |
Custom Membership Providers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
867 |
|
|
Architecture of Custom Providers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
867 |
|
|
Basic Steps for Creating Custom Providers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
869 |
|
|
Overall Design of the Custom Provider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
869 |
|
|
Designing and Implementing the Custom Store . . . . . . . . . . . . . . . . . . . . . . . . |
870 |
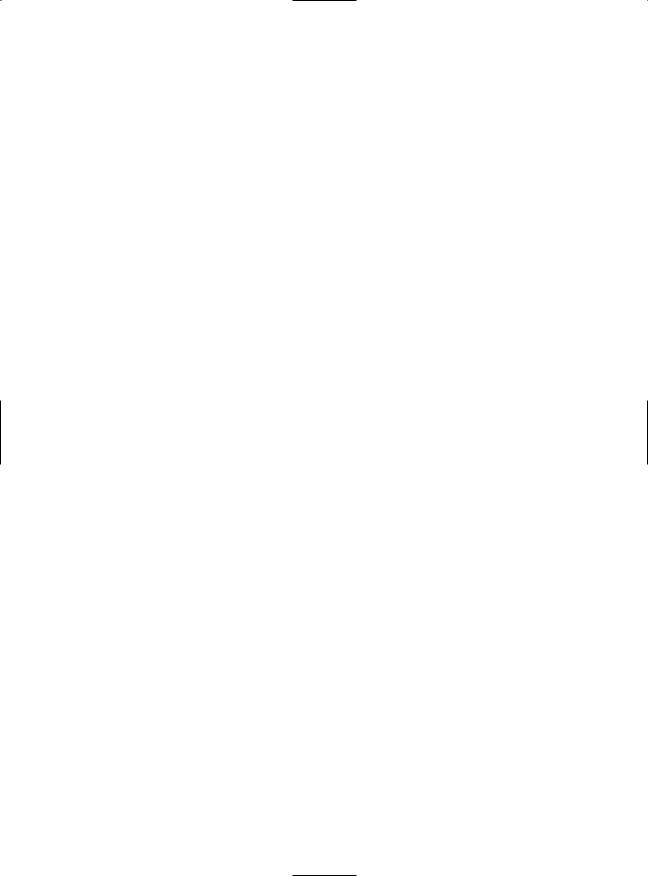
■C O N T E N T S xxi
Implementing the Provider Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 876
Using the Custom Provider Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 894
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 896
PART 5 ■ ■ ■ Advanced User Interface
■CHAPTER 27 Custom Server Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 899
Custom Server Control Basics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 900
Creating a Bare-Bones Custom Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 900
Using a Custom Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 902
Custom Controls in the Toolbox . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 903
Creating a WebControl That Supports Style Properties . . . . . . . . . . . . . . . . . . . 904
The Rendering Process . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 908
Dealing with Different Browsers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 909
The HtmlTextWriter . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 909
Browser Detection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 910
Browser Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 912
Adaptive Rendering . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 914
Control State and Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 915
View State . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 915
Control State . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 917
Postback Data and Change Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 918
Triggering a Postback . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 921
Extending Existing Web Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 923
Composite Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 923
Derived Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 925
Templated Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 930
Creating a Templated Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 930
Using Customized Templates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 933
Styles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 936
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 940
■CHAPTER 28 Design-Time Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 941
Design-Time Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 942
The Properties Window . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 942
Attributes and Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 945
The Toolbox Icon . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 946
Web Resources . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 947
Code Serialization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 949
Type Converters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 949
Serialization Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 957
Type Editors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 962
Control Designers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 965
A Basic Control Designer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 966
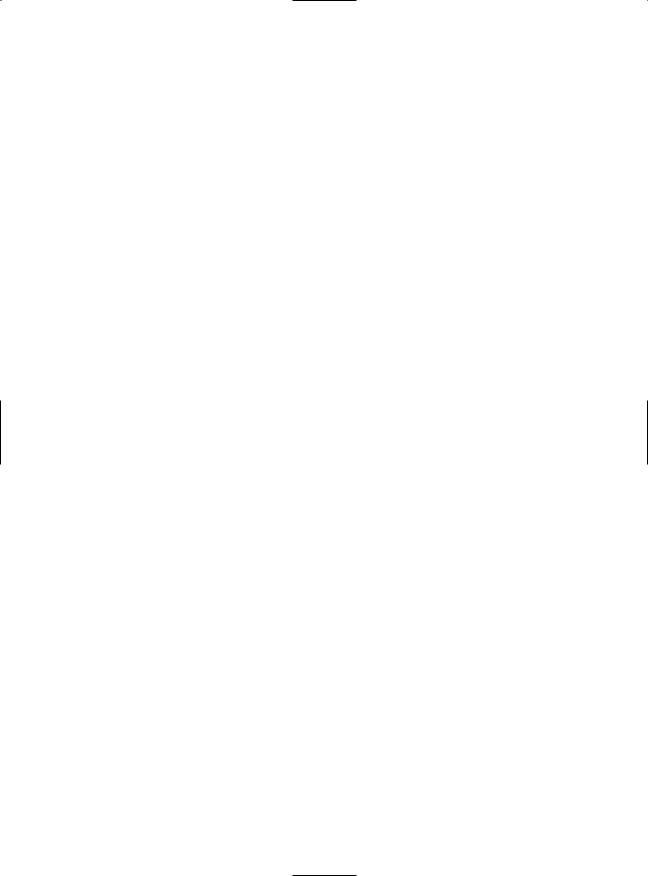
xxii ■C O N T E N T S
Smart Tags . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 968
The Action List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 969
The DesignerActionItem Collection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 970
The Control Designer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 972
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 973
■CHAPTER 29 JavaScript . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
975 |
JavaScript Essentials . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 975
JavaScript Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 976
Script Blocks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 978
Rendering Script Blocks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 986
Script Injection Attacks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 987
Request Validation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 988
Disabling Request Validation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 988
Client Callbacks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 991
Creating a Client Callback . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 991
Client Callbacks “Under the Hood” . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 995
Custom Controls with JavaScript . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 996
Pop-Up Windows . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 997
Rollover Buttons . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1000
Dynamic Panels . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1003
Frames . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1006
Frame Navigation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1007
Inline Frames . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1008
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1010
■CHAPTER 30 Dynamic Graphics and GDI+ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1011 |
The ImageMap Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1011
Creating Hotspots . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1012
Handling Hotspot Clicks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1013
A Custom Hotspot . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1014
Drawing with GDI+ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1016
Simple Drawing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1017
Image Format and Quality . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1019
The Graphics Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1020
Using a GraphicsPath . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1023
Pens . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1024
Brushes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1026
Embedding Dynamic Graphics in a Web Page . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1028
Using the PNG Format . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1029
Passing Information to Dynamic Images . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1030
Custom Controls That Use GDI+ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1033
Charting with GDI+ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1037
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1042
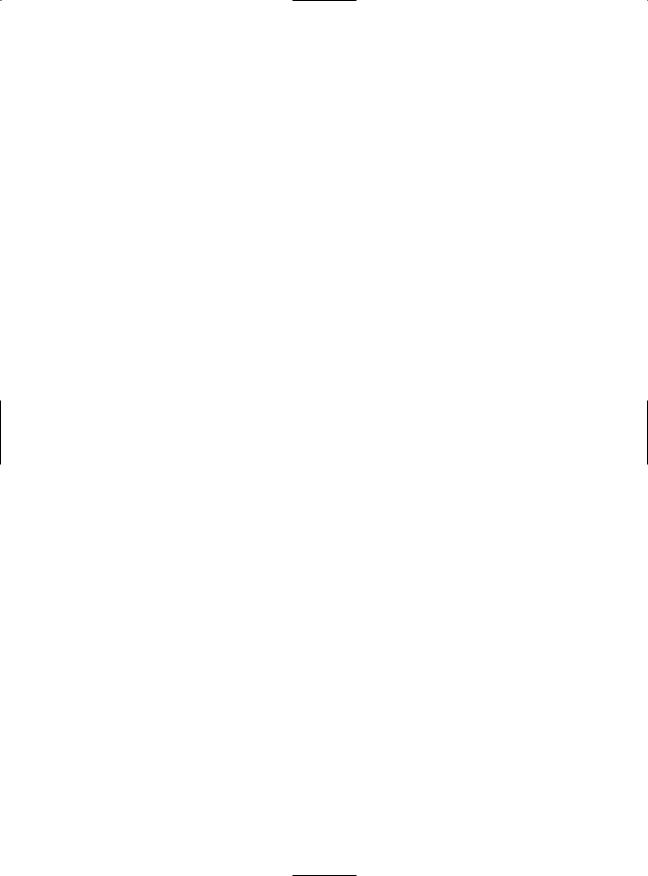
■C O N T E N T S xxiii
■CHAPTER 31 |
Portals with Web Part Pages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1043 |
|
Typical Portal Pages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1043 |
|
Basic Web Part Pages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1045 |
|
Creating the Page Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1045 |
|
WebPartManager and WebPartZones . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1047 |
|
Adding Web Parts to the Page . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1048 |
|
Customizing the Page . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1051 |
|
Creating Web Parts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1054 |
|
Simple Web Part Tasks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1054 |
|
Developing Advanced Web Parts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1062 |
|
Web Part Editors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1070 |
|
Connecting Web Parts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1076 |
|
Authorizing Web Parts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1083 |
|
Final Tasks for Personalization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1084 |
|
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1084 |
PART 6 ■ ■ ■ Web Services
■CHAPTER 32 Creating Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1087
Web Services Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1088
The History of Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1088
Distributed Computing and Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1089
The Problems with Distributed Component Technologies . . . . . . . . . . . . . . . . 1091
The Benefits of Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1091
Making Money with Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1093
The Web Service Stack . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1093
Building a Basic Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1096
The Web Service Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1096
Web Service Requirements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1097
Exposing a Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1100
Testing a Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1103
Consuming a Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1106
The Proxy Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1112
Creating an ASP.NET Client . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1113
Creating a Windows Forms Client . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1115
Creating an ASP Client with MSXML . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1117
Creating an ASP Client with the SOAP Toolkit . . . . . . . . . . . . . . . . . . . . . . . . . 1119
Refining a Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1120
CacheDuration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1120
EnableSession . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1123
BufferResponse . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1126
TransactionOption . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1126
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1128
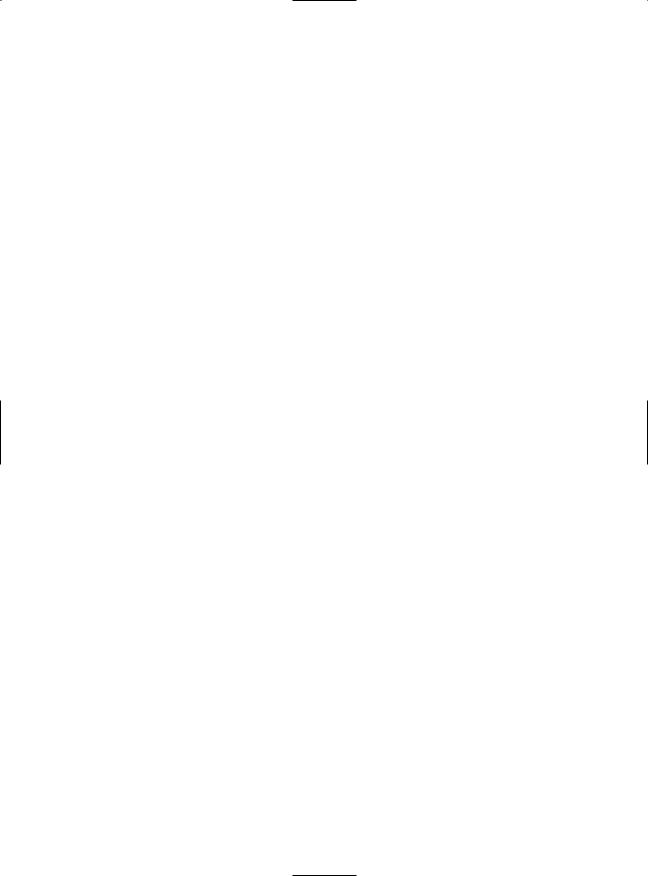
xxiv |
■C O N T E N T S |
|
|
|
■CHAPTER 33 |
Web Service Standards and Extensions . . . . . . . . . . . . . . . . . . . |
1129 |
|
|
WS-Interoperability . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1129 |
|
|
SOAP . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1131 |
|
|
SOAP Encoding . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1132 |
|
|
SOAP Versions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1133 |
|
|
Tracing SOAP Messages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1134 |
|
|
The SOAP Envelope . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1136 |
|
|
The SOAP Header . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1140 |
|
|
WSDL . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1144 |
|
|
Viewing the WSDL for a Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1144 |
|
|
The Basic Structure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1146 |
|
|
Implementing an Existing Contract . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1151 |
|
|
Customizing SOAP Messages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1152 |
|
|
Serializing Complex Data Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1152 |
|
|
Customizing XML Serialization with Attributes . . . . . . . . . . . . . . . . . . . . . . . . |
1156 |
|
|
Type Sharing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1159 |
|
|
Customizing XML Serialization with IXmlSerializable . . . . . . . . . . . . . . . . . . . |
1161 |
|
|
Custom Serialization for Large Data Types . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1165 |
|
|
Schema Importer Extensions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1170 |
|
|
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1173 |
|
■CHAPTER 34 |
Advanced Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
1175 |
Asynchronous Calls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1175
Asynchronous Delegates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1176
A Simple Asynchronous Call . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1178
Concurrent Asynchronous Calls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1180
Responsive Windows Clients . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1181
Asynchronous Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1185
Securing Web Services . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1186
Windows Authentication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1186
Custom Ticket-Based Authentication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1189
Tracking the User Identity . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1190
Authenticating the User . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1191
Authorizing the User . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1192
Testing the SOAP Authentication System . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1192
SOAP Extensions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1194
Creating a SOAP Extension . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1196
The Web Services Enhancements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1203
Installing the WSE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1204
Performing Authentication with the WSE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1206
Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1210
■INDEX . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1211
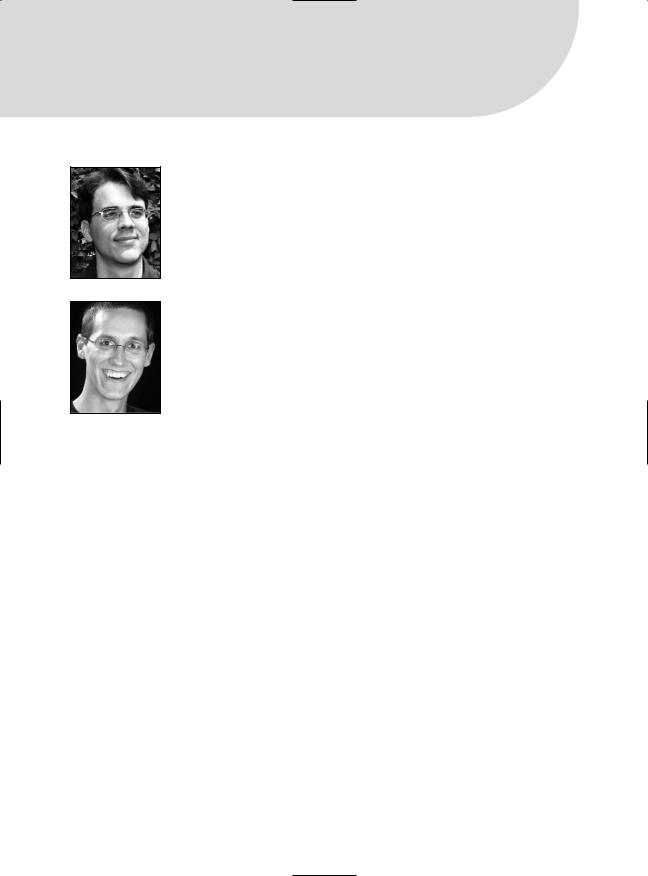
About the Revising Authors
■MATTHEW MACDONALD is an author, educator, and MCSD developer. He’s a regular contributor to programming journals and the author of more than a dozen books about .NET programming, including ASP.NET: The Complete Reference (Osborne McGraw-Hill, 2002), Programming .NET Web Services
(O’Reilly, 2002), Beginning ASP.NET in C (Apress, 2004), and Microsoft .NET Distributed Applications (Microsoft Press, 2003). In a dimly remembered past life, he studied English literature and theoretical physics.
■MARIO SZPUSZTA works in the Developer and Platform Group of Microsoft Austria. Before he started working for Microsoft, Mario was involved in several projects based on COM+ and DCOM with Visual Basic and Visual C++ as well as projects based on Java and J2SE. With beta 2 of the first version of the .NET Framework, he started developing web applications with ASP.NET. Currently, as a developer evangelist for Microsoft Austria, he conducts workshops, trainings, and proof-of-concept projects with independent software vendors in Austria based on .NET web services and Office 2003 technologies.
xxv
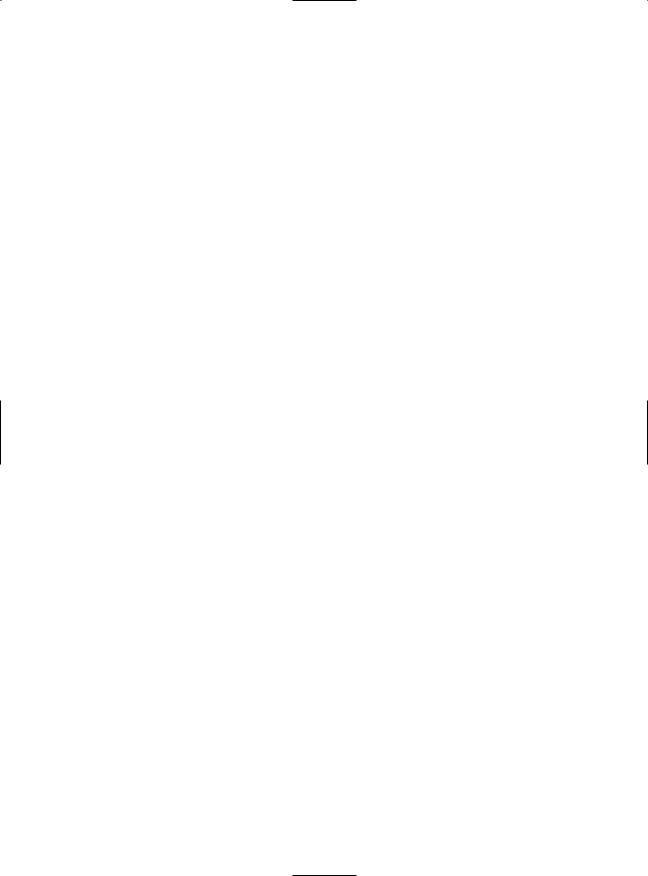
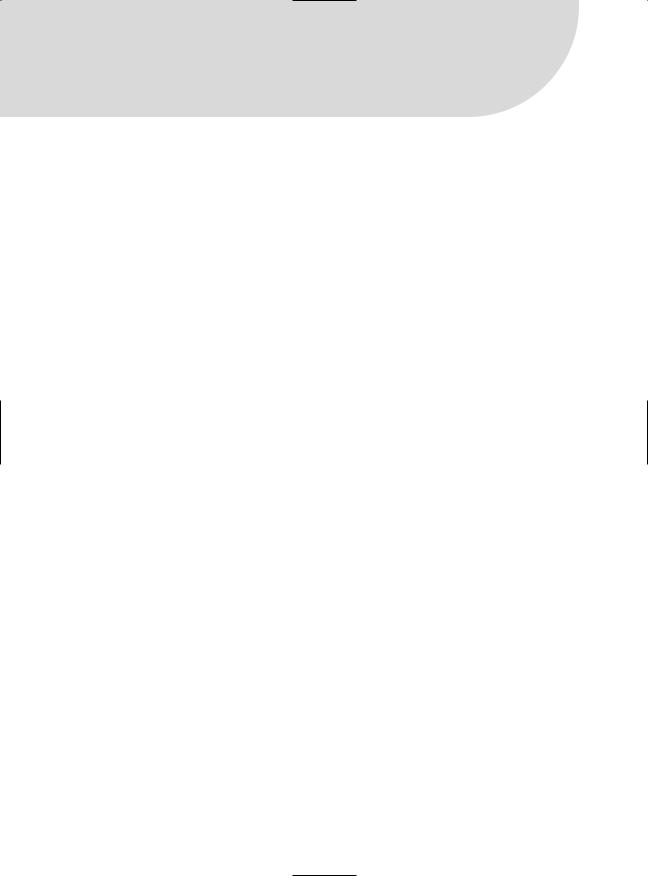
About the Technical Reviewers
■ROBERT LAIR is the president and CEO of Intensity Software (http://www.intensitysoftware.com), which specializes in Microsoft .NET consulting services. In addition to consulting services, Intensity offers Kicks for .NET, a CICS-to-ASP.NET migration utility that automates the migration process while maintaining the existing business logic’s source code. Robert was one of the developers who created the original IBuySpy Store and Portal demo applications as well as the NetCOBOL for .NET version of IBuySpy and the QuickStart samples. Robert has been a participating author for a number of books and has written numerous articles about Microsoft .NET–related topics. Robert’s personal website is at http://www.robertlair.com, and his blog is at http://www.robertlair.com/blogs/lair.
Robert would like to thank his beautiful wife, Debi, and four-year-old son, Max, for the family time that was sacrificed while reviewing this book.
■JASON LEFEBVRE is the vice president and one of the founding partners of Intensity Software. He uses Visual Studio and the Microsoft .NET Framework daily while architecting solutions for clients of Intensity’s consulting services. He is also one of the developers who created the original IBuySpy Store demo application and its NetCOBOL for .NET translation. Jason has been a participating author for a number of books and has written numerous articles about Microsoft .NET–related topics.
He would like to thank his friends’ new puppy, Oliver, for being so cute.
xxvii
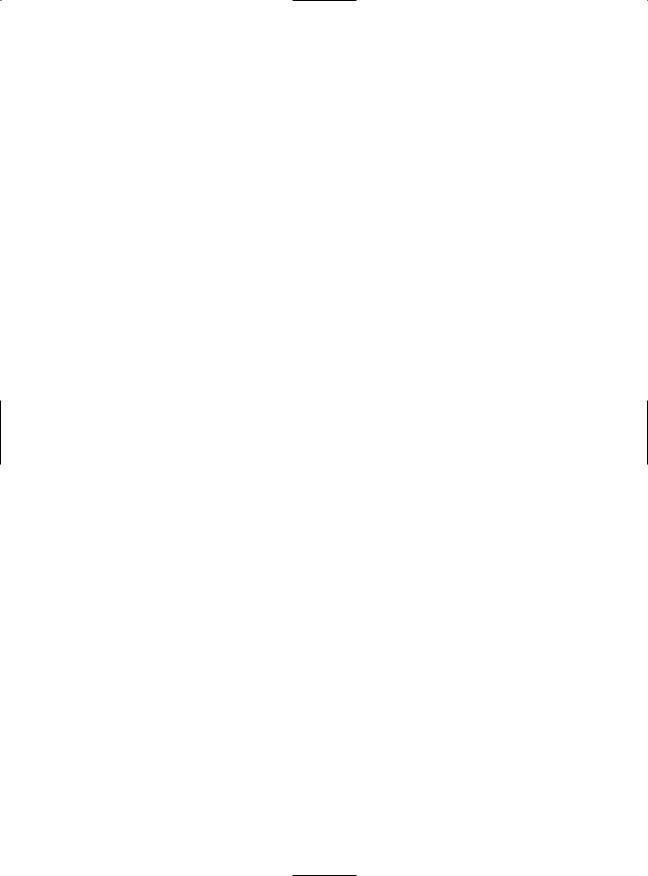
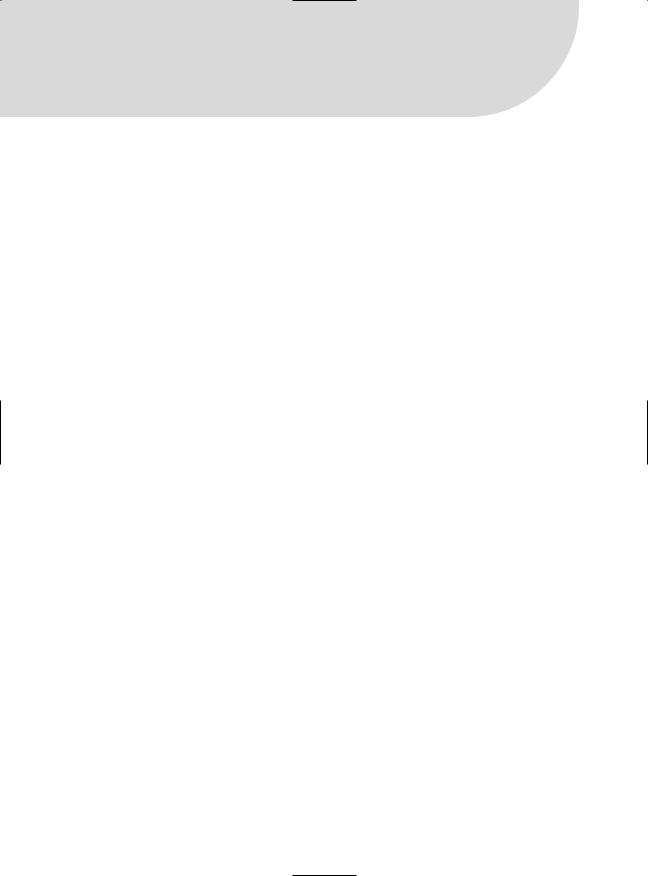
Introduction
It’s not hard to get developers interested in ASP.NET. Without exaggeration, ASP.NET is the most complete platform for web development that’s ever been put together. It far outclasses its predecessor, ASP, which was designed as a quick-and-dirty set of tools for inserting dynamic content into ordinary web pages. By contrast, ASP.NET is a full-blown platform for developing comprehensive, blisteringly fast web applications.
In this book, you’ll learn everything you need to master ASP.NET 2.0. If you’ve programmed with a previous version of ASP.NET, you’ll sail through the basics and quickly begin learning about the exciting new features in version 2.0. If you’ve never programmed with ASP.NET, you’ll find that this book provides a well-paced tour that leads through all the fundamentals, along with a backstage pass that lets you see how the ASP.NET internals really work. The only requirement for this book is that you have a solid understanding of the C# language and the basics of .NET. If you’re a seasoned Java or C++ developer but you’re new to C#, you may find it easier to start with a book about .NET fundamentals before you read this one. Try C# and the .NET 2.0 Platform, Third Edition (Apress, 2005) for a comprehensive introduction or, for a quicker start, read A Programmer’s Introduction to C# 2.0, Third Edition (Apress, 2005).
ASP.NET from 1.0 to 2.0
As you no doubt already know, ASP.NET is Microsoft’s next-generation technology for creating server-side web applications. It’s built on the Microsoft .NET Framework, which is a cluster of closely related new technologies that revolutionizes everything from database access to distributed applications. ASP.NET is one of the most important components of the .NET Framework—it’s the part that enables you to develop high-performance web applications and web services.
ASP.NET 1.0 was a revolution in the web programming world. It was so wildly popular that it was licensed on thousands of commercial web servers through Microsoft’s Go-Live license program while it was still a beta product. ASP.NET 1.0 was finally released in early 2002.
ASP.NET 1.1 wasn’t as ambitious. Instead, it was just a chance for Microsoft architects to pause and catch their collective breath. The focus in ASP.NET 1.1 wasn’t on new features—there weren’t any—but on performance tune-ups, security tweaks, and minor bug fixes. New features were quietly shelved and saved for the next major milestone, ASP.NET 2.0. ASP.NET 1.1 was released late in 2003, solidifying ASP.NET as the web development platform of choice for professional developers.
Two long years later, ASP.NET 2.0 finally appeared on the horizon. Unlike the ASP.NET 1.0 release, ASP.NET 2.0 doesn’t represent the start of a new direction in web development. In fact, almost all the underlying architecture that underpins ASP.NET 1.0 remains the same in ASP.NET 2.0. The difference is that ASP.NET 2.0 adds layers of higher-level features to the existing technology. Essentially, after the success of ASP.NET 1.0, Microsoft poured developers, time, and resources
into planning and preparing ASP.NET 2.0. Seeing as they no longer needed to rewrite the ASP.NET engine, the ASP.NET team members were free to be innovative with new controls, create better data management solutions, build a role-based security framework, and even make a whole toolkit for creating portal websites. In short, ASP.NET 2.0 gives developers a chance to relax and enjoy a wealth of new frills designed for their favorite platform. In this book, you’ll learn how to use, customize, and extend all these features.
xxix