
- •Front Matter
- •Copyright, Trademarks, and Attributions
- •Attributions
- •Print Production
- •Contacting The Publisher
- •HTML Version and Source Code
- •Typographical Conventions
- •Author Introduction
- •Audience
- •Book Content
- •The Genesis of repoze.bfg
- •The Genesis of Pyramid
- •Thanks
- •Pyramid Introduction
- •What Makes Pyramid Unique
- •URL generation
- •Debug Toolbar
- •Debugging settings
- •Class-based and function-based views
- •Extensible templating
- •Rendered views can return dictionaries
- •Event system
- •Built-in internationalization
- •HTTP caching
- •Sessions
- •Speed
- •Exception views
- •No singletons
- •View predicates and many views per route
- •Transaction management
- •Flexible authentication and authorization
- •Traversal
- •Tweens
- •View response adapters
- •Testing
- •Support
- •Documentation
- •What Is The Pylons Project?
- •Pyramid and Other Web Frameworks
- •Installing Pyramid
- •Before You Install
- •Installing Pyramid on a UNIX System
- •Installing the virtualenv Package
- •Creating the Virtual Python Environment
- •Installing Pyramid Into the Virtual Python Environment
- •Installing Pyramid on a Windows System
- •What Gets Installed
- •Application Configuration
- •Summary
- •Creating Your First Pyramid Application
- •Hello World
- •Imports
- •View Callable Declarations
- •WSGI Application Creation
- •WSGI Application Serving
- •Conclusion
- •References
- •Creating a Pyramid Project
- •Scaffolds Included with Pyramid
- •Creating the Project
- •Installing your Newly Created Project for Development
- •Running The Tests For Your Application
- •Running The Project Application
- •Reloading Code
- •Viewing the Application
- •The Debug Toolbar
- •The Project Structure
- •The MyProject Project
- •development.ini
- •production.ini
- •MANIFEST.in
- •setup.py
- •setup.cfg
- •The myproject Package
- •__init__.py
- •views.py
- •static
- •templates/mytemplate.pt
- •tests.py
- •Modifying Package Structure
- •Using the Interactive Shell
- •What Is This pserve Thing
- •Using an Alternate WSGI Server
- •Startup
- •The Startup Process
- •Deployment Settings
- •Request Processing
- •URL Dispatch
- •High-Level Operational Overview
- •Route Pattern Syntax
- •Route Declaration Ordering
- •Route Matching
- •The Matchdict
- •The Matched Route
- •Routing Examples
- •Example 1
- •Example 2
- •Example 3
- •Matching the Root URL
- •Generating Route URLs
- •Static Routes
- •Debugging Route Matching
- •Using a Route Prefix to Compose Applications
- •Custom Route Predicates
- •Route Factories
- •Using Pyramid Security With URL Dispatch
- •Route View Callable Registration and Lookup Details
- •References
- •Views
- •View Callables
- •View Callable Responses
- •Using Special Exceptions In View Callables
- •HTTP Exceptions
- •How Pyramid Uses HTTP Exceptions
- •Custom Exception Views
- •Using a View Callable to Do an HTTP Redirect
- •Handling Form Submissions in View Callables (Unicode and Character Set Issues)
- •Alternate View Callable Argument/Calling Conventions
- •Renderers
- •Writing View Callables Which Use a Renderer
- •Built-In Renderers
- •string: String Renderer
- •json: JSON Renderer
- •JSONP Renderer
- •*.pt or *.txt: Chameleon Template Renderers
- •*.mak or *.mako: Mako Template Renderer
- •Varying Attributes of Rendered Responses
- •Deprecated Mechanism to Vary Attributes of Rendered Responses
- •Adding and Changing Renderers
- •Adding a New Renderer
- •Changing an Existing Renderer
- •Overriding A Renderer At Runtime
- •Templates
- •Using Templates Directly
- •System Values Used During Rendering
- •Chameleon ZPT Templates
- •A Sample ZPT Template
- •Using ZPT Macros in Pyramid
- •Templating with Chameleon Text Templates
- •Side Effects of Rendering a Chameleon Template
- •Debugging Templates
- •Chameleon Template Internationalization
- •Templating With Mako Templates
- •A Sample Mako Template
- •Automatically Reloading Templates
- •Available Add-On Template System Bindings
- •View Configuration
- •Mapping a Resource or URL Pattern to a View Callable
- •@view_defaults Class Decorator
- •NotFound Errors
- •Debugging View Configuration
- •Static Assets
- •Serving Static Assets
- •Generating Static Asset URLs
- •Advanced: Serving Static Assets Using a View Callable
- •Root-Relative Custom Static View (URL Dispatch Only)
- •Overriding Assets
- •The override_asset API
- •Request and Response Objects
- •Request
- •Special Attributes Added to the Request by Pyramid
- •URLs
- •Methods
- •Unicode
- •Multidict
- •Dealing With A JSON-Encoded Request Body
- •Cleaning Up After a Request
- •More Details
- •Response
- •Headers
- •Instantiating the Response
- •Exception Responses
- •More Details
- •Sessions
- •Using The Default Session Factory
- •Using a Session Object
- •Using Alternate Session Factories
- •Creating Your Own Session Factory
- •Flash Messages
- •Using the session.flash Method
- •Using the session.pop_flash Method
- •Using the session.peek_flash Method
- •Preventing Cross-Site Request Forgery Attacks
- •Using the session.get_csrf_token Method
- •Using the session.new_csrf_token Method
- •Using Events
- •An Example
- •Reloading Templates
- •Reloading Assets
- •Debugging Authorization
- •Debugging Not Found Errors
- •Debugging Route Matching
- •Preventing HTTP Caching
- •Debugging All
- •Reloading All
- •Default Locale Name
- •Including Packages
- •pyramid.includes vs. pyramid.config.Configurator.include()
- •Mako Template Render Settings
- •Mako Directories
- •Mako Module Directory
- •Mako Input Encoding
- •Mako Error Handler
- •Mako Default Filters
- •Mako Import
- •Mako Preprocessor
- •Examples
- •Understanding the Distinction Between reload_templates and reload_assets
- •Adding A Custom Setting
- •Logging
- •Sending Logging Messages
- •Filtering log messages
- •Logging Exceptions
- •PasteDeploy Configuration Files
- •PasteDeploy
- •Entry Points and PasteDeploy .ini Files
- •[DEFAULTS] Section of a PasteDeploy .ini File
- •Command-Line Pyramid
- •Displaying Matching Views for a Given URL
- •The Interactive Shell
- •Extending the Shell
- •IPython or bpython
- •Displaying All Application Routes
- •Invoking a Request
- •Writing a Script
- •Changing the Request
- •Cleanup
- •Setting Up Logging
- •Making Your Script into a Console Script
- •Internationalization and Localization
- •Creating a Translation String
- •Using The TranslationString Class
- •Using the TranslationStringFactory Class
- •Working With gettext Translation Files
- •Installing Babel and Lingua
- •Extracting Messages from Code and Templates
- •Initializing a Message Catalog File
- •Updating a Catalog File
- •Compiling a Message Catalog File
- •Using a Localizer
- •Performing a Translation
- •Performing a Pluralization
- •Obtaining the Locale Name for a Request
- •Performing Date Formatting and Currency Formatting
- •Chameleon Template Support for Translation Strings
- •Mako Pyramid I18N Support
- •Localization-Related Deployment Settings
- •Activating Translation
- •Adding a Translation Directory
- •Setting the Locale
- •Locale Negotiators
- •The Default Locale Negotiator
- •Using a Custom Locale Negotiator
- •Virtual Hosting
- •Virtual Root Support
- •Further Documentation and Examples
- •Test Set Up and Tear Down
- •What?
- •Using the Configurator and pyramid.testing APIs in Unit Tests
- •Creating Integration Tests
- •Creating Functional Tests
- •Resources
- •Location-Aware Resources
- •Generating The URL Of A Resource
- •Overriding Resource URL Generation
- •Generating the Path To a Resource
- •Finding a Resource by Path
- •Obtaining the Lineage of a Resource
- •Determining if a Resource is In The Lineage of Another Resource
- •Finding the Root Resource
- •Resources Which Implement Interfaces
- •Finding a Resource With a Class or Interface in Lineage
- •Pyramid API Functions That Act Against Resources
- •Much Ado About Traversal
- •URL Dispatch
- •Historical Refresher
- •Traversal (aka Resource Location)
- •View Lookup
- •Use Cases
- •Traversal
- •Traversal Details
- •The Resource Tree
- •The Traversal Algorithm
- •A Description of The Traversal Algorithm
- •Traversal Algorithm Examples
- •References
- •Security
- •Enabling an Authorization Policy
- •Enabling an Authorization Policy Imperatively
- •Protecting Views with Permissions
- •Setting a Default Permission
- •Assigning ACLs to your Resource Objects
- •Elements of an ACL
- •Special Principal Names
- •Special Permissions
- •Special ACEs
- •ACL Inheritance and Location-Awareness
- •Changing the Forbidden View
- •Debugging View Authorization Failures
- •Debugging Imperative Authorization Failures
- •Creating Your Own Authentication Policy
- •Creating Your Own Authorization Policy
- •Combining Traversal and URL Dispatch
- •A Review of Non-Hybrid Applications
- •URL Dispatch Only
- •Traversal Only
- •Hybrid Applications
- •The Root Object for a Route Match
- •Using *traverse In a Route Pattern
- •Using *subpath in a Route Pattern
- •Corner Cases
- •Registering a Default View for a Route That Has a view Attribute
- •Using Hooks
- •Changing the Not Found View
- •Changing the Forbidden View
- •Changing the Request Factory
- •Using The Before Render Event
- •Adding Renderer Globals (Deprecated)
- •Using Response Callbacks
- •Using Finished Callbacks
- •Changing the Traverser
- •Changing How pyramid.request.Request.resource_url() Generates a URL
- •Changing How Pyramid Treats View Responses
- •Using a View Mapper
- •Creating a Tween Factory
- •Registering an Implicit Tween Factory
- •Suggesting Implicit Tween Ordering
- •Explicit Tween Ordering
- •Displaying Tween Ordering
- •Pyramid Configuration Introspection
- •Using the Introspector
- •Introspectable Objects
- •Pyramid Introspection Categories
- •Introspection in the Toolbar
- •Disabling Introspection
- •Rules for Building An Extensible Application
- •Fundamental Plugpoints
- •Extending an Existing Application
- •Extending the Application
- •Overriding Views
- •Overriding Routes
- •Overriding Assets
- •Advanced Configuration
- •Two-Phase Configuration
- •Using config.action in a Directive
- •Adding Configuration Introspection
- •Introspectable Relationships
- •Thread Locals
- •Why and How Pyramid Uses Thread Local Variables
- •Using the Zope Component Architecture in Pyramid
- •Using the ZCA Global API in a Pyramid Application
- •Disusing the Global ZCA API
- •Enabling the ZCA Global API by Using hook_zca
- •Enabling the ZCA Global API by Using The ZCA Global Registry
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Make a Project
- •Run the Tests
- •Expose Test Coverage Information
- •Start the Application
- •Visit the Application in a Browser
- •Decisions the zodb Scaffold Has Made For You
- •Basic Layout
- •Resources and Models with models.py
- •Views With views.py
- •Defining the Domain Model
- •Delete the Database
- •Edit models.py
- •Look at the Result of Our Edits to models.py
- •View the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Adding View Functions
- •Viewing the Result of all Our Edits to views.py
- •Adding Templates
- •Viewing the Application in a Browser
- •Adding Authorization
- •Add Authentication and Authorization Policies
- •Add security.py
- •Give Our Root Resource an ACL
- •Add Login and Logout Views
- •Change Existing Views
- •Add permission Declarations to our view_config Decorators
- •Add the login.pt Template
- •Change view.pt and edit.pt
- •See Our Changes To views.py and our Templates
- •View the Application in a Browser
- •Adding Tests
- •Test the Models
- •Test the Views
- •Functional tests
- •View the results of all our edits to tests.py
- •Run the Tests
- •Distributing Your Application
- •SQLAlchemy + URL Dispatch Wiki Tutorial
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Making a Project
- •Running the Tests
- •Exposing Test Coverage Information
- •Initializing the Database
- •Starting the Application
- •Decisions the alchemy Scaffold Has Made For You
- •Basic Layout
- •View Declarations via views.py
- •Content Models with models.py
- •Making Edits to models.py
- •Changing scripts/initializedb.py
- •Reinitializing the Database
- •Viewing the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Running setup.py develop
- •Changing the views.py File
- •Adding Templates
- •Adding Routes to __init__.py
- •Viewing the Application in a Browser
- •Adding Authorization
- •Adding A Root Factory
- •Add an Authorization Policy and an Authentication Policy
- •Adding an authentication policy callback
- •Adding Login and Logout Views
- •Changing Existing Views
- •Adding the login.pt Template
- •Seeing Our Changes To views.py and our Templates
- •Viewing the Application in a Browser
- •Adding Tests
- •Testing the Models
- •Testing the Views
- •Functional tests
- •Viewing the results of all our edits to tests.py
- •Running the Tests
- •Distributing Your Application
- •Converting a repoze.bfg Application to Pyramid
- •Running a Pyramid Application under mod_wsgi
- •pyramid.authorization
- •pyramid.authentication
- •Authentication Policies
- •Helper Classes
- •pyramid.chameleon_text
- •pyramid.chameleon_zpt
- •pyramid.config
- •pyramid.events
- •Functions
- •Event Types
- •pyramid.exceptions
- •pyramid.httpexceptions
- •HTTP Exceptions
- •pyramid.i18n
- •pyramid.interfaces
- •Event-Related Interfaces
- •Other Interfaces
- •pyramid.location
- •pyramid.paster
- •pyramid.registry
- •pyramid.renderers
- •pyramid.request
- •pyramid.response
- •Functions
- •pyramid.scripting
- •pyramid.security
- •Authentication API Functions
- •Authorization API Functions
- •Constants
- •Return Values
- •pyramid.settings
- •pyramid.testing
- •pyramid.threadlocal
- •pyramid.traversal
- •pyramid.url
- •pyramid.view
- •pyramid.wsgi
- •Glossary
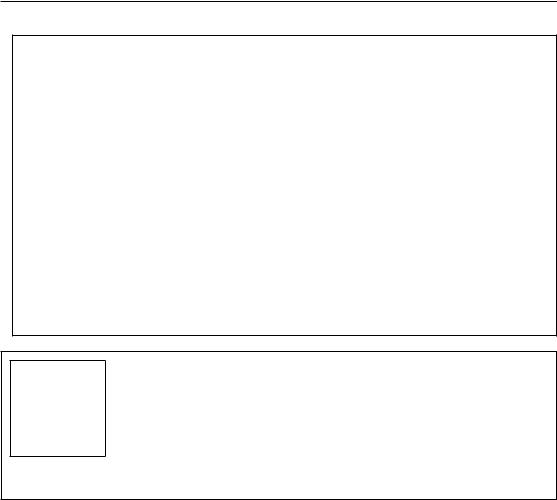
8. URL DISPATCH
1 from pyramid.httpexceptions import HTTPNotFound
2 from pyramid.view import notfound_view_config, view_config
3
4 @notfound_view_config(append_slash=True)
5 def notfound(request):
6return HTTPNotFound(’Not found, bro.’)
7
8 @view_config(route_name=’noslash’) 9 def no_slash(request):
10 return Response(’No slash’)
11
12@view_config(route_name=’hasslash’)
13def has_slash(request):
14return Response(’Has slash’)
15
16def main(g, **settings):
17config = Configurator()
18config.add_route(’noslash’, ’no_slash’)
19config.add_route(’hasslash’, ’has_slash/’)
20config.scan()
latex-warning.png
You should not rely on this mechanism to redirect POST requests. The redirect of the slash-appending not found view will turn a POST request into a GET, losing any POST data in the original request.
See pyramid.view and Changing the Not Found View for for a more general description of how to configure a view and/or a not found view.
8.9 Debugging Route Matching
It’s useful to be able to take a peek under the hood when requests that enter your application arent matching your routes as you expect them to. To debug route matching, use the
PYRAMID_DEBUG_ROUTEMATCH environment variable or the pyramid.debug_routematch configuration file setting (set either to true). Details of the route matching decision for a particular request to the Pyramid application will be printed to the stderr of the console which you started the application from. For example:
86
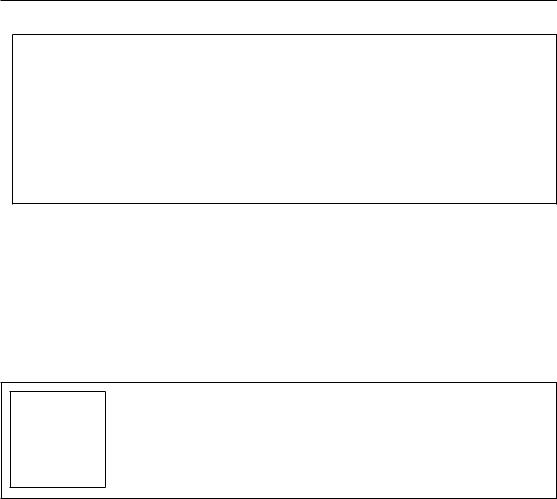
8.10. USING A ROUTE PREFIX TO COMPOSE APPLICATIONS
1[chrism@thinko pylonsbasic]$ PYRAMID_DEBUG_ROUTEMATCH=true \
2 |
bin/pserve development.ini |
3Starting server in PID 13586.
4 serving on 0.0.0.0:6543 view at http://127.0.0.1:6543
52010-12-16 14:45:19,956 no route matched for url \
6 |
http://localhost:6543/wontmatch |
72010-12-16 14:45:20,010 no route matched for url \
8 |
http://localhost:6543/favicon.ico |
92010-12-16 14:41:52,084 route matched for url \
10 |
http://localhost:6543/static/logo.png; \ |
11 |
route_name: ’static/’, .... |
See Environment Variables and .ini File Settings for more information about how, and where to set these values.
You can also use the proutes command to see a display of all the routes configured in your application; for more information, see Displaying All Application Routes.
8.10 Using a Route Prefix to Compose Applications
latex-note.png
This feature is new as of Pyramid 1.2.
The pyramid.config.Configurator.include() method allows configuration statements to be included from separate files. See Rules for Building An Extensible Application for information about this method. Using pyramid.config.Configurator.include() allows you to build your application from small and potentially reusable components.
The pyramid.config.Configurator.include() method accepts an argument named route_prefix which can be useful to authors of URL-dispatch-based applications. If route_prefix is supplied to the include method, it must be a string. This string represents a route prefix that will be prepended to all route patterns added by the included configuration. Any calls to pyramid.config.Configurator.add_route() within the included callable will have their pattern prefixed with the value of route_prefix. This can be used to help mount a set of routes at a different location than the included callable’s author intended while still maintaining the same route names. For example:
87
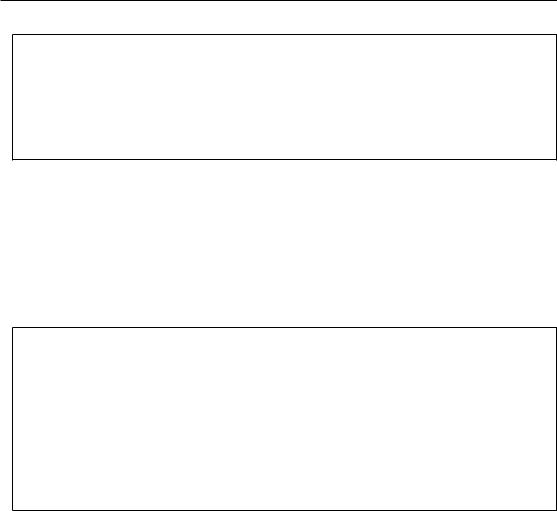
8. URL DISPATCH
1 from pyramid.config import Configurator
2
3 def users_include(config):
4config.add_route(’show_users’, ’/show’)
5
6 def main(global_config, **settings):
7config = Configurator()
8config.include(users_include, route_prefix=’/users’)
In the above configuration, the show_users route will have |
an effective route pattern of |
|
/users/show, |
instead of /show because the route_prefix argument will be prepended |
|
to the pattern. |
The route will then only match if the URL |
path is /users/show, and |
when the pyramid.request.Request.route_url() function is called with the route name show_users, it will generate a URL with that same path.
Route prefixes are recursive, so if a callable executed via an include itself turns around and includes another callable, the second-level route prefix will be prepended with the first:
1 from pyramid.config import Configurator
2
3 def timing_include(config):
4config.add_route(’show_times’, /times’)
5
6 def users_include(config):
7config.add_route(’show_users’, ’/show’)
8config.include(timing_include, route_prefix=’/timing’)
9
10def main(global_config, **settings):
11config = Configurator()
12config.include(users_include, route_prefix=’/users’)
In the above configuration, the show_users route will still have an effective route pattern of /users/show. The show_times route however, will have an effective pattern of
/users/timing/show_times.
Route prefixes have no impact on the requirement that the set of route names in any given Pyramid configuration must be entirely unique. If you compose your URL dispatch application out of many small subapplications using pyramid.config.Configurator.include(), it’s wise to use a dotted name for your route names, so they’ll be unlikely to conflict with other packages that may be added in the future. For example:
88

8.11. CUSTOM ROUTE PREDICATES
1 from pyramid.config import Configurator
2
3 def timing_include(config):
4config.add_route(’timing.show_times’, /times’)
5
6 def users_include(config):
7config.add_route(’users.show_users’, ’/show’)
8config.include(timing_include, route_prefix=’/timing’)
9
10def main(global_config, **settings):
11config = Configurator()
12config.include(users_include, route_prefix=’/users’)
8.11 Custom Route Predicates
Each of the predicate callables fed to the custom_predicates argument of add_route() must be a callable accepting two arguments. The first argument passed to a custom predicate is a dictionary conventionally named info. The second argument is the current request object.
The info dictionary has a number of contained values: match is a dictionary: it represents the arguments matched in the URL by the route. route is an object representing the route which was matched (see pyramid.interfaces.IRoute for the API of such a route object).
info[’match’] is useful when predicates need access to the route match. For example:
1 def any_of(segment_name, *allowed):
2def predicate(info, request):
3if info[’match’][segment_name] in allowed:
4 |
return True |
5return predicate
6 |
|
7 |
num_one_two_or_three = any_of(’num’, ’one’, ’two’, ’three’) |
8 |
|
9 |
config.add_route(’route_to_num’, ’/{num}’, |
10 |
custom_predicates=(num_one_two_or_three,)) |
The above any_of function generates a predicate which ensures that the match value named segment_name is in the set of allowable values represented by allowed. We use this any_of function to generate a predicate function named num_one_two_or_three, which ensures that the num segment is one of the values one, two, or three , and use the result as a custom predicate by feeding it inside a tuple to the custom_predicates argument to add_route().
89
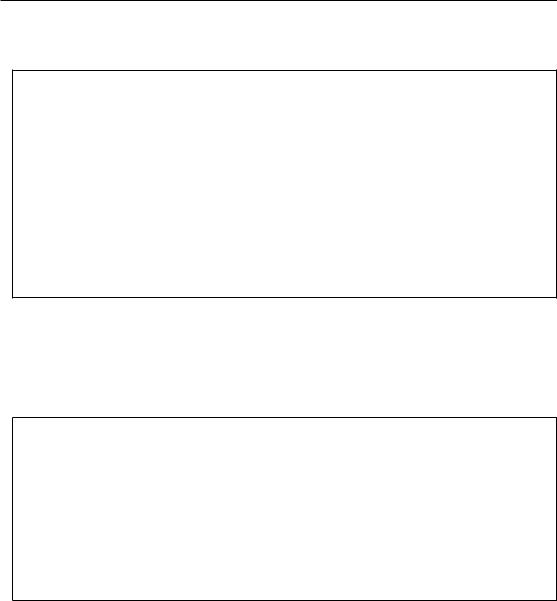
8. URL DISPATCH
A custom route predicate may also modify the match dictionary. For instance, a predicate might do some type conversion of values:
1def integers(*segment_names):
2 def predicate(info, request):
3match = info[’match’]
4for segment_name in segment_names:
5 |
try: |
6 |
match[segment_name] = int(match[segment_name]) |
7 |
except (TypeError, ValueError): |
8 |
pass |
9 |
return True |
10 |
return predicate |
11 |
|
12 |
ymd_to_int = integers(’year’, ’month’, ’day’) |
13 |
|
14 |
config.add_route(’ymd’, ’/{year}/{month}/{day}’, |
15 |
custom_predicates=(ymd_to_int,)) |
Note that a conversion predicate is still a predicate so it must return True or False; a predicate that does only conversion, such as the one we demonstrate above should unconditionally return True.
To avoid the try/except uncertainty, the route pattern can contain regular expressions specifying requirements for that marker. For instance:
1def integers(*segment_names):
2 def predicate(info, request):
3match = info[’match’]
4for segment_name in segment_names:
5 |
match[segment_name] = int(match[segment_name]) |
6return True
7return predicate
8 |
|
9 |
ymd_to_int = integers(’year’, ’month’, ’day’) |
10 |
|
11 |
config.add_route(’ymd’, ’/{year:\d+}/{month:\d+}/{day:\d+}’, |
12 |
custom_predicates=(ymd_to_int,)) |
Now the try/except is no longer needed because the route will not match at all unless these markers match \d+ which requires them to be valid digits for an int type conversion.
The match dictionary passed within info to each predicate attached to a route will be the same dictionary. Therefore, when registering a custom predicate which modifies the match dict, the code registering
90
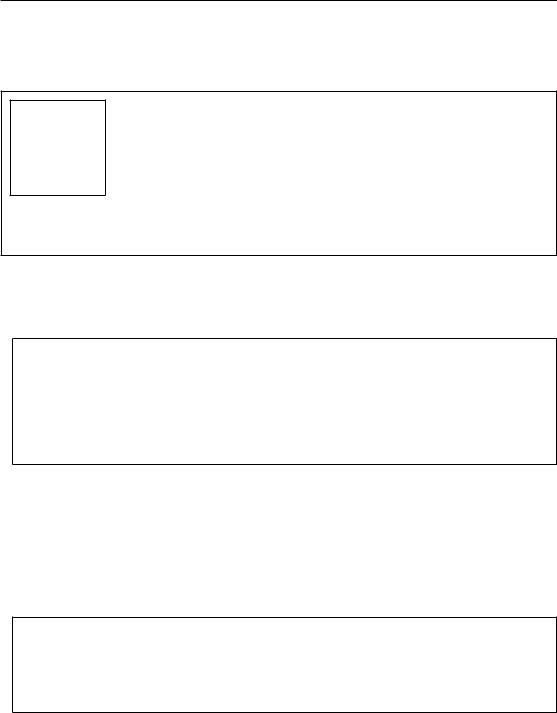
8.11. CUSTOM ROUTE PREDICATES
the predicate should usually arrange for the predicate to be the last custom predicate in the custom predicate list. Otherwise, custom predicates which fire subsequent to the predicate which performs the match modification will receive the modified match dictionary.
latex-warning.png
It is a poor idea to rely on ordering of custom predicates to build a conversion pipeline, where one predicate depends on the side effect of another. For instance, it’s a poor idea to register two custom predicates, one which handles conversion of a value to an int, the next which handles conversion of that integer to some custom object. Just do all that in a single custom predicate.
The route object in the info dict is an object that has two useful attributes: name and pattern. The name attribute is the route name. The pattern attribute is the route pattern. An example of using the route in a set of route predicates:
1def twenty_ten(info, request):
2 if info[’route’].name in (’ymd’, ’ym’, ’y’):
3return info[’match’][’year’] == ’2010’
4
5config.add_route(’y’, ’/{year}’, custom_predicates=(twenty_ten,))
6 config.add_route(’ym’, ’/{year}/{month}’, custom_predicates=(twenty_ten,))
7config.add_route(’ymd’, ’/{year}/{month}/{day}’,
8 |
custom_predicates=(twenty_ten,)) |
The above predicate, when added to a number of route configurations ensures that the year match argument is ‘2010’ if and only if the route name is ‘ymd’, ‘ym’, or ‘y’.
You can also caption the predicates by setting the __text__ attribute. This will help you with the pviews command (see Displaying All Application Routes) and the pyramid_debugtoolbar.
If a predicate is a class just add __text__ property in a standard manner.
1 class DummyCustomPredicate1(object):
2def __init__(self):
3self.__text__ = ’my custom class predicate’
4 |
|
5 |
class DummyCustomPredicate2(object): |
6 |
__text__ = ’my custom class predicate’ |
91