
- •Front Matter
- •Copyright, Trademarks, and Attributions
- •Attributions
- •Print Production
- •Contacting The Publisher
- •HTML Version and Source Code
- •Typographical Conventions
- •Author Introduction
- •Audience
- •Book Content
- •The Genesis of repoze.bfg
- •The Genesis of Pyramid
- •Thanks
- •Pyramid Introduction
- •What Makes Pyramid Unique
- •URL generation
- •Debug Toolbar
- •Debugging settings
- •Class-based and function-based views
- •Extensible templating
- •Rendered views can return dictionaries
- •Event system
- •Built-in internationalization
- •HTTP caching
- •Sessions
- •Speed
- •Exception views
- •No singletons
- •View predicates and many views per route
- •Transaction management
- •Flexible authentication and authorization
- •Traversal
- •Tweens
- •View response adapters
- •Testing
- •Support
- •Documentation
- •What Is The Pylons Project?
- •Pyramid and Other Web Frameworks
- •Installing Pyramid
- •Before You Install
- •Installing Pyramid on a UNIX System
- •Installing the virtualenv Package
- •Creating the Virtual Python Environment
- •Installing Pyramid Into the Virtual Python Environment
- •Installing Pyramid on a Windows System
- •What Gets Installed
- •Application Configuration
- •Summary
- •Creating Your First Pyramid Application
- •Hello World
- •Imports
- •View Callable Declarations
- •WSGI Application Creation
- •WSGI Application Serving
- •Conclusion
- •References
- •Creating a Pyramid Project
- •Scaffolds Included with Pyramid
- •Creating the Project
- •Installing your Newly Created Project for Development
- •Running The Tests For Your Application
- •Running The Project Application
- •Reloading Code
- •Viewing the Application
- •The Debug Toolbar
- •The Project Structure
- •The MyProject Project
- •development.ini
- •production.ini
- •MANIFEST.in
- •setup.py
- •setup.cfg
- •The myproject Package
- •__init__.py
- •views.py
- •static
- •templates/mytemplate.pt
- •tests.py
- •Modifying Package Structure
- •Using the Interactive Shell
- •What Is This pserve Thing
- •Using an Alternate WSGI Server
- •Startup
- •The Startup Process
- •Deployment Settings
- •Request Processing
- •URL Dispatch
- •High-Level Operational Overview
- •Route Pattern Syntax
- •Route Declaration Ordering
- •Route Matching
- •The Matchdict
- •The Matched Route
- •Routing Examples
- •Example 1
- •Example 2
- •Example 3
- •Matching the Root URL
- •Generating Route URLs
- •Static Routes
- •Debugging Route Matching
- •Using a Route Prefix to Compose Applications
- •Custom Route Predicates
- •Route Factories
- •Using Pyramid Security With URL Dispatch
- •Route View Callable Registration and Lookup Details
- •References
- •Views
- •View Callables
- •View Callable Responses
- •Using Special Exceptions In View Callables
- •HTTP Exceptions
- •How Pyramid Uses HTTP Exceptions
- •Custom Exception Views
- •Using a View Callable to Do an HTTP Redirect
- •Handling Form Submissions in View Callables (Unicode and Character Set Issues)
- •Alternate View Callable Argument/Calling Conventions
- •Renderers
- •Writing View Callables Which Use a Renderer
- •Built-In Renderers
- •string: String Renderer
- •json: JSON Renderer
- •JSONP Renderer
- •*.pt or *.txt: Chameleon Template Renderers
- •*.mak or *.mako: Mako Template Renderer
- •Varying Attributes of Rendered Responses
- •Deprecated Mechanism to Vary Attributes of Rendered Responses
- •Adding and Changing Renderers
- •Adding a New Renderer
- •Changing an Existing Renderer
- •Overriding A Renderer At Runtime
- •Templates
- •Using Templates Directly
- •System Values Used During Rendering
- •Chameleon ZPT Templates
- •A Sample ZPT Template
- •Using ZPT Macros in Pyramid
- •Templating with Chameleon Text Templates
- •Side Effects of Rendering a Chameleon Template
- •Debugging Templates
- •Chameleon Template Internationalization
- •Templating With Mako Templates
- •A Sample Mako Template
- •Automatically Reloading Templates
- •Available Add-On Template System Bindings
- •View Configuration
- •Mapping a Resource or URL Pattern to a View Callable
- •@view_defaults Class Decorator
- •NotFound Errors
- •Debugging View Configuration
- •Static Assets
- •Serving Static Assets
- •Generating Static Asset URLs
- •Advanced: Serving Static Assets Using a View Callable
- •Root-Relative Custom Static View (URL Dispatch Only)
- •Overriding Assets
- •The override_asset API
- •Request and Response Objects
- •Request
- •Special Attributes Added to the Request by Pyramid
- •URLs
- •Methods
- •Unicode
- •Multidict
- •Dealing With A JSON-Encoded Request Body
- •Cleaning Up After a Request
- •More Details
- •Response
- •Headers
- •Instantiating the Response
- •Exception Responses
- •More Details
- •Sessions
- •Using The Default Session Factory
- •Using a Session Object
- •Using Alternate Session Factories
- •Creating Your Own Session Factory
- •Flash Messages
- •Using the session.flash Method
- •Using the session.pop_flash Method
- •Using the session.peek_flash Method
- •Preventing Cross-Site Request Forgery Attacks
- •Using the session.get_csrf_token Method
- •Using the session.new_csrf_token Method
- •Using Events
- •An Example
- •Reloading Templates
- •Reloading Assets
- •Debugging Authorization
- •Debugging Not Found Errors
- •Debugging Route Matching
- •Preventing HTTP Caching
- •Debugging All
- •Reloading All
- •Default Locale Name
- •Including Packages
- •pyramid.includes vs. pyramid.config.Configurator.include()
- •Mako Template Render Settings
- •Mako Directories
- •Mako Module Directory
- •Mako Input Encoding
- •Mako Error Handler
- •Mako Default Filters
- •Mako Import
- •Mako Preprocessor
- •Examples
- •Understanding the Distinction Between reload_templates and reload_assets
- •Adding A Custom Setting
- •Logging
- •Sending Logging Messages
- •Filtering log messages
- •Logging Exceptions
- •PasteDeploy Configuration Files
- •PasteDeploy
- •Entry Points and PasteDeploy .ini Files
- •[DEFAULTS] Section of a PasteDeploy .ini File
- •Command-Line Pyramid
- •Displaying Matching Views for a Given URL
- •The Interactive Shell
- •Extending the Shell
- •IPython or bpython
- •Displaying All Application Routes
- •Invoking a Request
- •Writing a Script
- •Changing the Request
- •Cleanup
- •Setting Up Logging
- •Making Your Script into a Console Script
- •Internationalization and Localization
- •Creating a Translation String
- •Using The TranslationString Class
- •Using the TranslationStringFactory Class
- •Working With gettext Translation Files
- •Installing Babel and Lingua
- •Extracting Messages from Code and Templates
- •Initializing a Message Catalog File
- •Updating a Catalog File
- •Compiling a Message Catalog File
- •Using a Localizer
- •Performing a Translation
- •Performing a Pluralization
- •Obtaining the Locale Name for a Request
- •Performing Date Formatting and Currency Formatting
- •Chameleon Template Support for Translation Strings
- •Mako Pyramid I18N Support
- •Localization-Related Deployment Settings
- •Activating Translation
- •Adding a Translation Directory
- •Setting the Locale
- •Locale Negotiators
- •The Default Locale Negotiator
- •Using a Custom Locale Negotiator
- •Virtual Hosting
- •Virtual Root Support
- •Further Documentation and Examples
- •Test Set Up and Tear Down
- •What?
- •Using the Configurator and pyramid.testing APIs in Unit Tests
- •Creating Integration Tests
- •Creating Functional Tests
- •Resources
- •Location-Aware Resources
- •Generating The URL Of A Resource
- •Overriding Resource URL Generation
- •Generating the Path To a Resource
- •Finding a Resource by Path
- •Obtaining the Lineage of a Resource
- •Determining if a Resource is In The Lineage of Another Resource
- •Finding the Root Resource
- •Resources Which Implement Interfaces
- •Finding a Resource With a Class or Interface in Lineage
- •Pyramid API Functions That Act Against Resources
- •Much Ado About Traversal
- •URL Dispatch
- •Historical Refresher
- •Traversal (aka Resource Location)
- •View Lookup
- •Use Cases
- •Traversal
- •Traversal Details
- •The Resource Tree
- •The Traversal Algorithm
- •A Description of The Traversal Algorithm
- •Traversal Algorithm Examples
- •References
- •Security
- •Enabling an Authorization Policy
- •Enabling an Authorization Policy Imperatively
- •Protecting Views with Permissions
- •Setting a Default Permission
- •Assigning ACLs to your Resource Objects
- •Elements of an ACL
- •Special Principal Names
- •Special Permissions
- •Special ACEs
- •ACL Inheritance and Location-Awareness
- •Changing the Forbidden View
- •Debugging View Authorization Failures
- •Debugging Imperative Authorization Failures
- •Creating Your Own Authentication Policy
- •Creating Your Own Authorization Policy
- •Combining Traversal and URL Dispatch
- •A Review of Non-Hybrid Applications
- •URL Dispatch Only
- •Traversal Only
- •Hybrid Applications
- •The Root Object for a Route Match
- •Using *traverse In a Route Pattern
- •Using *subpath in a Route Pattern
- •Corner Cases
- •Registering a Default View for a Route That Has a view Attribute
- •Using Hooks
- •Changing the Not Found View
- •Changing the Forbidden View
- •Changing the Request Factory
- •Using The Before Render Event
- •Adding Renderer Globals (Deprecated)
- •Using Response Callbacks
- •Using Finished Callbacks
- •Changing the Traverser
- •Changing How pyramid.request.Request.resource_url() Generates a URL
- •Changing How Pyramid Treats View Responses
- •Using a View Mapper
- •Creating a Tween Factory
- •Registering an Implicit Tween Factory
- •Suggesting Implicit Tween Ordering
- •Explicit Tween Ordering
- •Displaying Tween Ordering
- •Pyramid Configuration Introspection
- •Using the Introspector
- •Introspectable Objects
- •Pyramid Introspection Categories
- •Introspection in the Toolbar
- •Disabling Introspection
- •Rules for Building An Extensible Application
- •Fundamental Plugpoints
- •Extending an Existing Application
- •Extending the Application
- •Overriding Views
- •Overriding Routes
- •Overriding Assets
- •Advanced Configuration
- •Two-Phase Configuration
- •Using config.action in a Directive
- •Adding Configuration Introspection
- •Introspectable Relationships
- •Thread Locals
- •Why and How Pyramid Uses Thread Local Variables
- •Using the Zope Component Architecture in Pyramid
- •Using the ZCA Global API in a Pyramid Application
- •Disusing the Global ZCA API
- •Enabling the ZCA Global API by Using hook_zca
- •Enabling the ZCA Global API by Using The ZCA Global Registry
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Make a Project
- •Run the Tests
- •Expose Test Coverage Information
- •Start the Application
- •Visit the Application in a Browser
- •Decisions the zodb Scaffold Has Made For You
- •Basic Layout
- •Resources and Models with models.py
- •Views With views.py
- •Defining the Domain Model
- •Delete the Database
- •Edit models.py
- •Look at the Result of Our Edits to models.py
- •View the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Adding View Functions
- •Viewing the Result of all Our Edits to views.py
- •Adding Templates
- •Viewing the Application in a Browser
- •Adding Authorization
- •Add Authentication and Authorization Policies
- •Add security.py
- •Give Our Root Resource an ACL
- •Add Login and Logout Views
- •Change Existing Views
- •Add permission Declarations to our view_config Decorators
- •Add the login.pt Template
- •Change view.pt and edit.pt
- •See Our Changes To views.py and our Templates
- •View the Application in a Browser
- •Adding Tests
- •Test the Models
- •Test the Views
- •Functional tests
- •View the results of all our edits to tests.py
- •Run the Tests
- •Distributing Your Application
- •SQLAlchemy + URL Dispatch Wiki Tutorial
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Making a Project
- •Running the Tests
- •Exposing Test Coverage Information
- •Initializing the Database
- •Starting the Application
- •Decisions the alchemy Scaffold Has Made For You
- •Basic Layout
- •View Declarations via views.py
- •Content Models with models.py
- •Making Edits to models.py
- •Changing scripts/initializedb.py
- •Reinitializing the Database
- •Viewing the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Running setup.py develop
- •Changing the views.py File
- •Adding Templates
- •Adding Routes to __init__.py
- •Viewing the Application in a Browser
- •Adding Authorization
- •Adding A Root Factory
- •Add an Authorization Policy and an Authentication Policy
- •Adding an authentication policy callback
- •Adding Login and Logout Views
- •Changing Existing Views
- •Adding the login.pt Template
- •Seeing Our Changes To views.py and our Templates
- •Viewing the Application in a Browser
- •Adding Tests
- •Testing the Models
- •Testing the Views
- •Functional tests
- •Viewing the results of all our edits to tests.py
- •Running the Tests
- •Distributing Your Application
- •Converting a repoze.bfg Application to Pyramid
- •Running a Pyramid Application under mod_wsgi
- •pyramid.authorization
- •pyramid.authentication
- •Authentication Policies
- •Helper Classes
- •pyramid.chameleon_text
- •pyramid.chameleon_zpt
- •pyramid.config
- •pyramid.events
- •Functions
- •Event Types
- •pyramid.exceptions
- •pyramid.httpexceptions
- •HTTP Exceptions
- •pyramid.i18n
- •pyramid.interfaces
- •Event-Related Interfaces
- •Other Interfaces
- •pyramid.location
- •pyramid.paster
- •pyramid.registry
- •pyramid.renderers
- •pyramid.request
- •pyramid.response
- •Functions
- •pyramid.scripting
- •pyramid.security
- •Authentication API Functions
- •Authorization API Functions
- •Constants
- •Return Values
- •pyramid.settings
- •pyramid.testing
- •pyramid.threadlocal
- •pyramid.traversal
- •pyramid.url
- •pyramid.view
- •pyramid.wsgi
- •Glossary
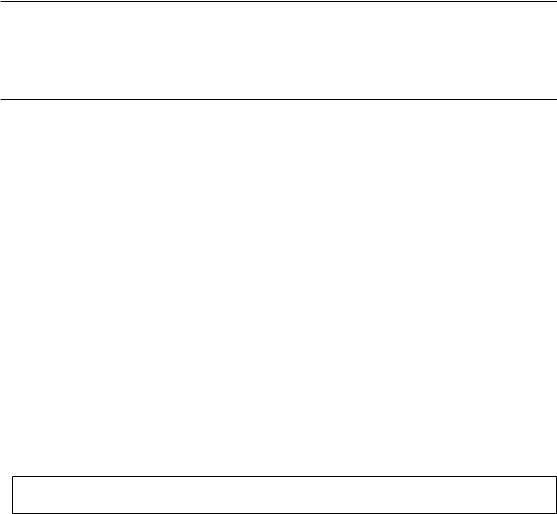
CHAPTER
TWENTYNINE
USING HOOKS
“Hooks” can be used to influence the behavior of the Pyramid framework in various ways.
29.1 Changing the Not Found View
When Pyramid can’t map a URL to view code, it invokes a not found view, which is a view callable. A default notfound view exists. The default not found view can be overridden through application configuration.
If your application uses imperative configuration, you can replace the Not Found view by using the pyramid.config.Configurator.add_notfound_view() method:
1
2
from helloworld.views import notfound config.add_notfound_view(notfound)
Replace helloworld.views.notfound with a reference to the view callable you want to use to represent the Not Found view. The not found view callable is a view callable like any other.
If your application instead uses pyramid.view.view_config decorators and a scan, you can replace the Not Found view by using the pyramid.view.notfound_view_config decorator:
317
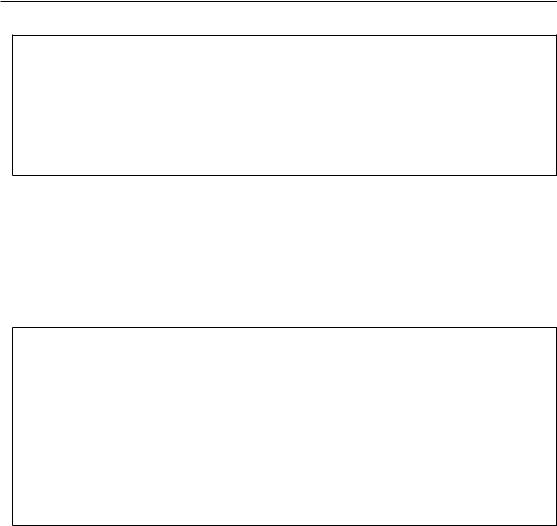
29. USING HOOKS
1 from pyramid.view import notfound_view_config
2
3 @notfound_view_config()
4 def notfound(request):
5return Response(’Not Found, dude’, status=’404 Not Found’)
6
7 def main(globals, **settings):
8config = Configurator()
9config.scan()
This does exactly what the imperative example above showed.
Your |
application |
can |
define |
multiple |
not |
found |
views |
if |
necessary. |
Both |
pyramid.config.Configurator.add_notfound_view() |
and |
pyramid.view.notfound_view_config take most of the same arguments as pyramid.config.Configurator.add_view and pyramid.view.view_config, respectively. This means that not found views can carry predicates limiting their applicability. For example:
1 from pyramid.view import notfound_view_config
2
3 @notfound_view_config(request_method=’GET’) 4 def notfound_get(request):
5return Response(’Not Found during GET, dude’, status=’404 Not Found’)
6
7 @notfound_view_config(request_method=’POST’) 8 def notfound_post(request):
9return Response(’Not Found during POST, dude’, status=’404 Not Found’)
10
11def main(globals, **settings):
12config = Configurator()
13config.scan()
The notfound_get view will be called when a view could not be found and the request method was GET. The notfound_post view will be called when a view could not be found and the request method was POST.
Like any other view, the notfound view must accept at least a request parameter, or both context and request. The request is the current request representing the denied action. The context (if used in the call signature) will be the instance of the HTTPNotFound exception that caused the view to be called.
Both pyramid.config.Configurator.add_notfound_view() and pyramid.view.notfound_view_config can be used to automatically redirect requests to slash-appended routes. See Redirecting to Slash-Appended Routes for examples.
318
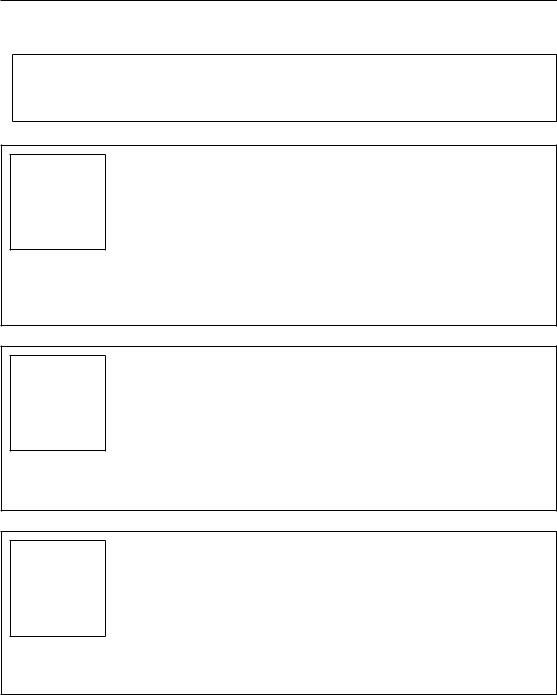
29.1. CHANGING THE NOT FOUND VIEW
Here’s some sample code that implements a minimal NotFound view callable:
1
2
3
4
from pyramid.httpexceptions import HTTPNotFound
def notfound(request): return HTTPNotFound()
latex-note.png
When a NotFound view callable is invoked, it is passed a request. The exception attribute of the request will be an instance of the HTTPNotFound exception that caused the not found view to be called. The value of request.exception.message will be a value explaining why the not found error was raised. This message will be different when the pyramid.debug_notfound environment setting is true than it is when it is false.
latex-note.png
Both pyramid.config.Configurator.add_notfound_view() and pyramid.view.notfound_view_config are new as of Pyramid 1.3. Older Pyramid documentation instructed users to use add_view instead, with a context of HTTPNotFound. This still works; the convenience method and decorator are just wrappers around this functionality.
latex-warning.png
When a NotFound view callable accepts an argument list as described in Alternate View Callable Argument/Calling Conventions, the context passed as the first argument to the view callable will be the HTTPNotFound exception instance. If available, the resource context will still be available as request.context.
319
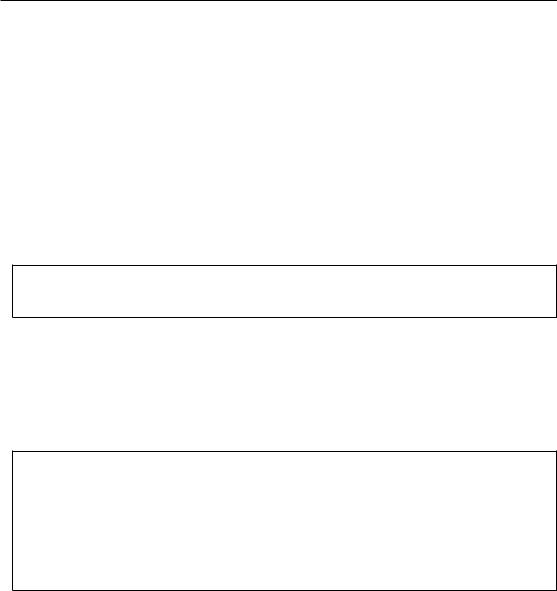
29. USING HOOKS
29.2 Changing the Forbidden View
When Pyramid can’t authorize execution of a view based on the authorization policy in use, it invokes a forbidden view. The default forbidden response has a 403 status code and is very plain, but the view which generates it can be overridden as necessary.
The forbidden view callable is a view callable like any other. The view configuration which causes it to be a “forbidden” view consists of using the meth:pyramid.config.Configurator.add_forbidden_view API or the pyramid.view.forbidden_view_config decorator.
For example, you can add a forbidden view by using the pyramid.config.Configurator.add_forbidden_view() method to register a forbidden view:
1
2
3
from helloworld.views import forbidden_view from pyramid.httpexceptions import HTTPForbidden config.add_forbidden_view(forbidden_view)
Replace helloworld.views.forbidden_view with a reference to the Python view callable you want to use to represent the Forbidden view.
If instead you prefer to use decorators and a scan, you can use the pyramid.view.forbidden_view_config decorator to mark a view callable as a forbidden view:
1 from pyramid.view import forbidden_view_config
2
3 forbidden_view_config()
4 def forbidden(request):
5return Response(’forbidden’)
6
7 def main(globals, **settings):
8config = Configurator()
9config.scan()
Like any other view, the forbidden view must accept at least a request parameter, or both context and request. The context (available as request.context if you’re using the request-only view argument pattern) is the context found by the router when the view invocation was denied. The request is the current request representing the denied action.
Here’s some sample code that implements a minimal forbidden view:
320