
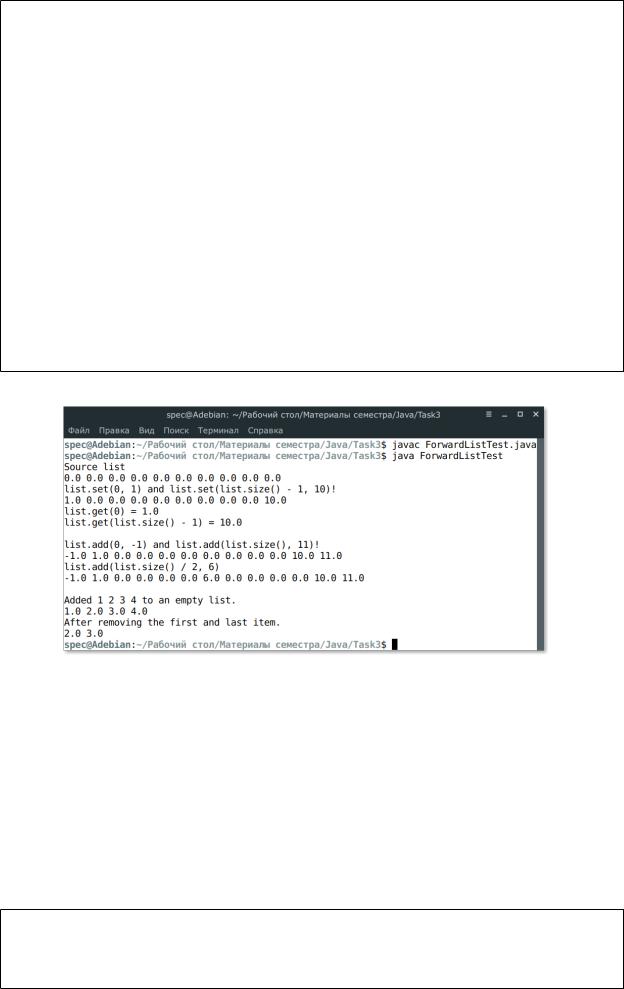
System.out.println("\nlist.add(0, -1) and list.add(list.size(),
11)!");
list.add(0, -1); list.add(list.size(), 11); list.print();
System.out.println("list.add(list.size() / 2, 6)"); list.add(list.size() / 2, 6);
list.print();
ForwardList newList = new ForwardList(0); newList.add(0, 1);
newList.add(1, 2); newList.add(2, 3); newList.add(3, 4);
System.out.println("\nAdded 1 2 3 4 to an empty list."); newList.print();
newList.remove(3);
newList.remove(0);
System.out.println("After removing the first and last item."); newList.print();
}
}
Результат компиляции и запуска приведен на рис. 5.
Рисунок 5 — Компиляция и запуск ForwardListTest.java Задание №6.
В этом же пакете создадим интерфейс IVector взаимодействия с векторами, имеющий методы, соответствующие общей функциональности двух созданных классов Array и ForwardList. Сделаем так, чтобы оба этих класса реализовывали этот интерфейс.
Код интерфейса приведен в табл. 10.
Таблица 10 — Код vectors/IVector.java package vectors;
public interface IVector {
// Получение элемента по индексу
public double get(int i) throws VectorIndexOutOfBoundsException;
13

// Изменение значения элемента по индексу public void set(int i, double value) throws
VectorIndexOutOfBoundsException;
//Получение длины вектора public int size();
//Нахождение нормы Евклида public double normEuclidean();
//Вывод всех элементов вектора public void print();
}
Измененные коды Array и ForwardList приведены в табл. 11, 12. Таблица 11 — Измененный код vectors/Array.java
package vectors;
public class Array implements IVector {
//Конструктор public Array(int n) {
data = new double[n];
}
//Получение элемента по индексу
public double get(int i) throws VectorIndexOutOfBoundsException { if (i >= 0 && i < data.length) {
return data[i];
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Установка значения элемента по индексу public void set(int i, double value) throws
VectorIndexOutOfBoundsException {
if (i >= 0 && i < data.length) { data[i] = value;
return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
//Получение размера вектора public int size() {
return data.length;
}
//Нахождение нормы Евклида public double normEuclidean() {
double r = 0;
for (double x : data) { r += x * x;
}
return Math.sqrt(r);
}
//Вывод вектора на экран public void print() {
if (data.length > 0) { System.out.print(data[0]);
14

}
for (int i = 1; i < data.length; ++i) { System.out.print(" " + data[i]);
}
System.out.println();
}
// Данные
private final double[] data;
}
Таблица 12 — Измененный код vectors/ForwardList.java package vectors;
public class ForwardList implements IVector { // Конструктор
public ForwardList(int n) { if (n > 0) {
head = new Node(0, null); Node temp = head;
for (int i = 1; i < n; ++i) {
temp = (temp.next = new Node(0, null));
}
this.size = n; } else {
this.head = null; this.size = 0;
}
}
// Получение элемента по индексу
public double get(int i) throws VectorIndexOutOfBoundsException { if (i >= 0 && i < size) {
Node temp = head;
for (int j = 0; j < i; ++j) { temp = temp.next;
}
return temp.value;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Изменение значения элемента по индексу public void set(int i, double value) throws
VectorIndexOutOfBoundsException { if (i >= 0 && i < size) {
Node temp = head;
for (int j = 0; j < i; ++j) { temp = temp.next;
}
temp.value = value; return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
//Получение длины списка public int size() {
return size;
}
//Добавление элемента в список
15

public void add(int i, double elem) throws VectorIndexOutOfBoundsException {
if (i >= 0 && i <= size) { if (i != 0) {
Node temp = head;
for (int j = 1; j < i; ++j) { temp = temp.next;
}
temp.next = new Node(elem, temp.next); } else {
head = new Node(elem, head);
}
++size; return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Удаление элемента из списка
public void remove(int i) throws VectorIndexOutOfBoundsException { if (i >= 0 && i < size) {
if (i != 0) {
Node temp = head;
for (int j = 1; j < i; ++j) { temp = temp.next;
}
temp.next = temp.next.next; } else {
head = head.next;
}
--size; return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
//Нахождение нормы Евклида public double normEuclidean() {
double r = 0;
for (Node temp = head; temp != null; temp = temp.next) { r += temp.value * temp.value;
}
return Math.sqrt(r);
}
//Вывод всех элементов списка
public void print() { Node temp = head; if (temp != null) {
System.out.print(temp.value); temp = temp.next;
}
for (; temp != null; temp = temp.next) { System.out.print(" " + temp.value);
}
System.out.println();
}
// Класс "Узел"
public static class Node {
public Node(double value, Node next) { this.value = value;
this.next = next;
16