
Федеральное агентство связи ФЕДЕРАЛЬНОЕ ГОСУДАРСТВЕННОЕ БЮДЖЕТНОЕ
ОБРАЗОВАТЕЛЬНОЕ УЧРЕЖДЕНИЕ ВЫСШЕГО ОБРАЗОВАНИЯ «САНКТ-ПЕТЕРБУРГСКИЙ ГОСУДАРСТВЕННЫЙ УНИВЕРСИТЕТ ТЕЛЕКОММУНИКАЦИЙ ИМ. ПРОФ. М. А. БОНЧ-БРУЕВИЧА» (СПбГУТ)
Факультет инфокоммуникационных сетей и систем Кафедра программной инженерии и вычислительной техники
ЛАБОРАТОРНАЯ РАБОТА №6
по дисциплине «Разработка Java-приложений управления телекоммуникациями»
Выполнил: студент 3-го курса дневного отделения группы ИКПИ-85
Коваленко Леонид Александрович Преподаватель:
доцент кафедры ПИиВТ Белая Татьяна Иоанновна
Санкт-Петербург
2020
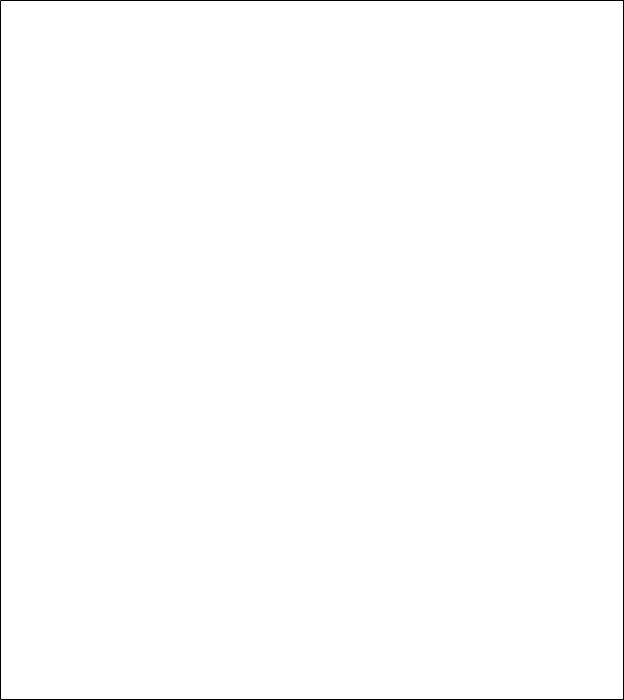
Цель работы
Ознакомиться с методами класса Object и расширить функциональность имеющегося пакета.
Ход работы
Задание №1.
Добавим в классы векторов Array и CircularLinkedList реализации метода String toString(). Будем использовать для формирования строки экземпляр класса StringBuffer (табл. 1, 2).
Таблица 1 — Измененный код vectors/Array.java
package vectors;
import java.io.Serializable;
import java.util.NoSuchElementException; import java.util.Iterator;
import java.lang.Iterable;
public class Array implements IVector, Serializable, Iterable<Double> {
//Конструктор public Array(int n) {
data = new double[n];
}
//Получение элемента по индексу
public double get(int i) throws VectorIndexOutOfBoundsException { if (i >= 0 && i < data.length) {
return data[i];
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Установка значения элемента по индексу public void set(int i, double value) throws
VectorIndexOutOfBoundsException {
if (i >= 0 && i < data.length) { data[i] = value;
return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
//Получение размера вектора public int size() {
return data.length;
}
//Нахождение нормы Евклида public double normEuclidean() {
double r = 0;
for (double x : data) { r += x * x;
}
return Math.sqrt(r);
}
2
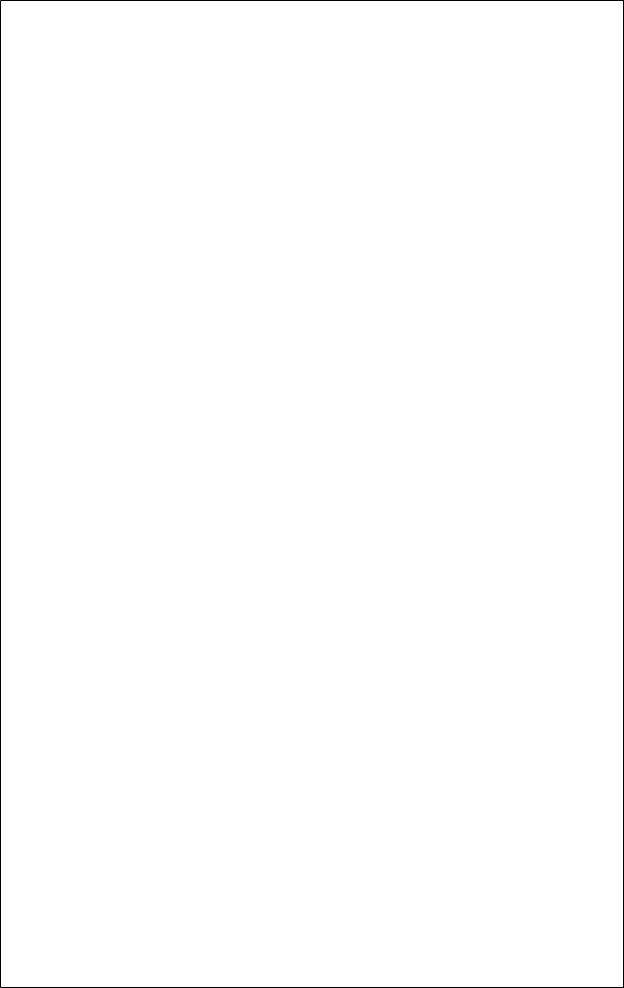
//Вывод вектора на экран public void print() {
if (data.length > 0) { System.out.print(data[0]);
}
for (int i = 1; i < data.length; ++i) { System.out.print(" " + data[i]);
}
System.out.println();
}
//Получение итератора
public Iterator<Double> iterator() { return new ArrayIterator(this);
}
// Класс итератора для массива
public static class ArrayIterator implements Iterator<Double>, Serializable {
//Конструктор ArrayIterator(Array obj) {
pos = 0; data = obj;
}
//Имеется ли следующий элемент public boolean hasNext() {
return (pos + 1) < data.size();
}
//Переход к следующему элементу
public Double next() throws NoSuchElementException { if (!hasNext())
throw new NoSuchElementException(); try {
return data.get(pos++);
}
catch(Exception e) {
throw new NoSuchElementException();
}
}
// Удаление элемента посредством итератора запрещено: вызывается исключение
public void remove() throws UnsupportedOperationException { throw new UnsupportedOperationException();
}
private int pos; private Array data;
}
// Преобразование в строку public String toString() {
StringBuffer stringBuffer = new StringBuffer(); if (data.length > 0) {
stringBuffer.append(data[0]);
}
for (int i = 1; i < data.length; ++i) { stringBuffer.append(" " + data[i]);
}
return stringBuffer.toString();
}
3

// Данные
private final double[] data;
}
Таблица 2 — Измененный код vectors/CircularLinkedList.java package vectors;
import java.io.Serializable;
import java.util.NoSuchElementException; import java.util.Iterator;
import java.lang.Iterable;
public class CircularLinkedList implements IVector, Serializable, Iterable<Double> {
// Конструктор
public CircularLinkedList(int n) {
current = head = new Node(0, null, null); head.prev = head.next = head; current_index = -1;
if (n > 0) {
Node temp = head;
for (int i = 0; i < n; ++i) {
temp = (temp.next = new Node(0, temp, null));
}
temp.next = head; head.prev = temp; size = n;
} else { size = 0;
}
}
// Получение элемента по индексу
public double get(int i) throws VectorIndexOutOfBoundsException { if (i >= 0 && i < size) {
if (Math.abs(i - current_index) > Math.min(i + 1, size - i)) { current = head;
current_index = (i + i < size) ? -1 : size;
}
for (; current_index < i; ++current_index) { current = current.next;
}
for (; current_index > i; --current_index) { current = current.prev;
}
return current.value;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Изменение значения элемента по индексу public void set(int i, double value) throws
VectorIndexOutOfBoundsException { if (i >= 0 && i < size) {
if (Math.abs(i - current_index) > Math.min(i + 1, size - i)) { current = head;
current_index = (i + i < size) ? -1 : size;
}
for (; current_index < i; ++current_index) { current = current.next;
}
for (; current_index > i; --current_index) { current = current.prev;
4

}
current.value = value; return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
//Получение длины списка public int size() {
return size;
}
//Добавление элемента в список
public void add(int i, double elem) throws VectorIndexOutOfBoundsException {
if (i >= 0 && i <= size) {
if (Math.abs(i - current_index) > Math.min(i + 1, size - i)) { current = head;
current_index = (i + i < size) ? -1 : size;
}
for (; current_index < i; ++current_index) { current = current.next;
}
for (; current_index > i; --current_index) { current = current.prev;
}
current = current.prev;
current.next = new Node(elem, current, current.next); current.next.next.prev = current.next;
current = current.next; ++size;
return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Удаление элемента из списка
public void remove(int i) throws VectorIndexOutOfBoundsException { if (i >= 0 && i < size) {
if (Math.abs(i - current_index) > Math.min(i + 1, size - i)) { current = head;
current_index = (i + i < size) ? -1 : size;
}
for (; current_index < i; ++current_index) { current = current.next;
}
for (; current_index > i; --current_index) { current = current.prev;
}
current = current.prev; current.next = current.next.next; current.next.prev = current; current = current.next;
--size; return;
}
throw new VectorIndexOutOfBoundsException("VectorIndexOutOfBoundsException", i);
}
// Нахождение нормы Евклида public double normEuclidean() {
double r = 0;
5
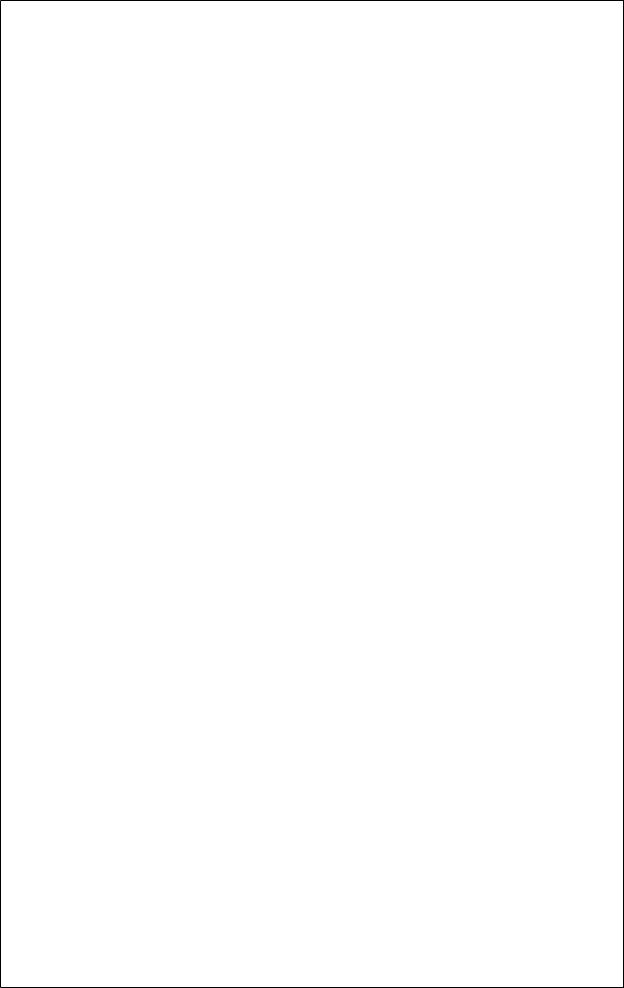
for (Node temp = head.next; temp != head; temp = temp.next) { r += temp.value * temp.value;
}
return Math.sqrt(r);
}
//Вывод всех элементов списка public void print() {
Node temp = head.next; if (temp != head) {
System.out.print(temp.value); temp = temp.next;
}
for (; temp != head; temp = temp.next) { System.out.print(" " + temp.value);
}
System.out.println();
}
//Вывод всех элементов списка в обратном порядке public void rprint() {
Node temp = head.prev; if (temp != head) {
System.out.print(temp.value); temp = temp.prev;
}
for (; temp != head; temp = temp.prev) { System.out.print(" " + temp.value);
}
System.out.println();
}
//Класс "Узел"
public static class Node implements Serializable { public Node(double value, Node prev, Node next) {
this.value = value; this.prev = prev; this.next = next;
}
public double value; public Node prev, next;
}
// Получение итератора
public Iterator<Double> iterator() {
return new CircularLinkedListIterator(this);
}
// Класс итератора для списка
public static class CircularLinkedListIterator implements Iterator<Double>, Serializable {
//Конструктор CircularLinkedListIterator(CircularLinkedList obj) {
pos = 0; data = obj;
}
//Имеется ли следующий элемент
public boolean hasNext() {
return (pos + 1) < data.size();
}
// Переход к следующему элементу
public Double next() throws NoSuchElementException {
6

if (!hasNext())
throw new NoSuchElementException(); try {
return data.get(pos++);
}
catch(Exception e) {
throw new NoSuchElementException();
}
}
// Удаление элемента посредством итератора запрещено: вызывается исключение
public void remove() throws UnsupportedOperationException { throw new UnsupportedOperationException();
}
private int pos;
private CircularLinkedList data;
}
// Преобразование в строку public String toString() {
StringBuffer stringBuffer = new StringBuffer(); Node temp = head.next;
if (temp != head) { stringBuffer.append(temp.value); temp = temp.next;
}
for (; temp != head; temp = temp.next) { stringBuffer.append(" " + temp.value);
}
return stringBuffer.toString();
}
private Node head, current; private int size, current_index;
}
Создадим файл StringBufferTest.java для проверки работы метода toString (табл. 3).
Таблица 3 — Код StringBufferTest.java
import vectors.Array;
import vectors.CircularLinkedList;
public class StringBufferTest {
public static void main(String[] args) throws Exception { Array arr = new Array(8);
CircularLinkedList list = new CircularLinkedList(8); for (int i = 0, len = arr.size(); i < len; ++i) {
arr.set(i, i); list.set(i, i);
}
System.out.println("Array: " + arr.toString()); System.out.println("CircularLinkedList: " + list.toString());
}
}
Результат компиляции и запуска приведен на рис. 1.
7