
- •Preface
- •Who Should Read This Book
- •Organization and Presentation
- •Contacting the Authors
- •Acknowledgments
- •Contents
- •Introduction
- •Why Microsoft .NET?
- •The Microsoft .NET Architecture
- •Internet Standards
- •The Evolution of ASP
- •The Benefits of ASP.NET
- •What Is .NET?
- •.NET Experiences
- •.NET Clients
- •.NET Services
- •.NET Servers
- •Review
- •Quiz Yourself
- •Installation Requirements
- •Installing ASP.NET and ADO.NET
- •Installing the .NET Framework SDK
- •Testing Your Installation
- •Support for .NET
- •Review
- •Quiz Yourself
- •Designing a Database
- •Normalization of Data
- •Security Considerations
- •Review
- •Quiz Yourself
- •Creating a Database
- •Creating SQL Server Tables
- •Creating a View
- •Creating a Stored Procedure
- •Creating a Trigger
- •Review
- •Quiz Yourself
- •INSERT Statements
- •DELETE Statements
- •UPDATE Statements
- •SELECT Statements
- •Review
- •Quiz Yourself
- •The XML Design Specs
- •The Structure of XML Documents
- •XML Syntax
- •XML and the .NET Framework
- •Review
- •Quiz Yourself
- •ASP.NET Events
- •Page Directives
- •Namespaces
- •Choosing a Language
- •Review
- •Quiz Yourself
- •Introducing HTML Controls
- •Using HTML controls
- •How HTML controls work
- •Intrinsic HTML controls
- •HTML Control Events
- •The Page_OnLoad event
- •Custom event handlers
- •Review
- •Quiz Yourself
- •Intrinsic Controls
- •Using intrinsic controls
- •Handling intrinsic Web control events
- •List Controls
- •Rich Controls
- •Review
- •Quiz Yourself
- •Creating a User Control
- •Adding User Control Properties
- •Writing Custom Control Methods
- •Implementing User Control Events
- •Review
- •Quiz Yourself
- •Common Aspects of Validation Controls
- •Display property
- •Type Property
- •Operator Property
- •Using Validation Controls
- •RequiredFieldValidator
- •RegularExpressionValidator
- •CompareValidator
- •RangeValidator
- •CustomValidator
- •ValidationSummaryx
- •Review
- •Quiz Yourself
- •Maintaining State Out of Process for Scalability
- •No More Cookies but Plenty of Milk!
- •Out of Process State Management
- •Review
- •Quiz Yourself
- •Introducing the Key Security Mechanisms
- •Web.config and Security
- •Special identities
- •Using request types to limit access
- •New Tricks for Forms-based Authentication
- •Using the Passport Authentication Provider
- •Review
- •Quiz Yourself
- •ASP.NET Updates to the ASP Response Model
- •Caching with ASP.NET
- •Page Output Caching
- •Absolute cache expiration
- •Sliding cache expiration
- •Fragment Caching
- •Page Data Caching
- •Expiration
- •File and Key Dependency and Scavenging
- •Review
- •Quiz Yourself
- •A Brief History of Microsoft Data Access
- •Differences between ADO and ADO.NET
- •Transmission formats
- •Connected versus disconnected datasets
- •COM marshaling versus text-based data transmission
- •Variant versus strongly typed data
- •Data schema
- •ADO.NET Managed Provider Versus SQL Managed Provider
- •Review
- •Quiz Yourself
- •Review
- •Quiz Yourself
- •Creating a Connection
- •Opening a Connection
- •Using Transactions
- •Review
- •Quiz Yourself
- •Building a Command
- •Connection property
- •CommandText property
- •CommandType property
- •CommandTimeout property
- •Appending parameters
- •Executing a Command
- •ExecuteNonQuery method
- •Prepare method
- •ExecuteReader method
- •Review
- •Quiz Yourself
- •Introducing DataReaders
- •Using DataReader Properties
- •Item property
- •FieldCount property
- •IsClosed property
- •RecordsAffected property
- •Using DataReader Methods
- •Read method
- •GetValue method
- •Get[Data Type] methods
- •GetOrdinal method
- •GetName method
- •Close method
- •Review
- •Quiz Yourself
- •Constructing a DataAdapter Object
- •SelectCommand property
- •UpdateCommand, DeleteCommand, and InsertCommand properties
- •Fill method
- •Update method
- •Dispose method
- •Using DataSet Objects
- •DataSetName property
- •CaseSensitive property
- •Review
- •Quiz Yourself
- •Constructing a DataSet
- •Tables property
- •TablesCollection Object
- •Count property
- •Item property
- •Contains method
- •CanRemove method
- •Remove method
- •Add method
- •DataTable Objects
- •CaseSensitive property
- •ChildRelations property
- •Columns property
- •Constraints property
- •DataSet property
- •DefaultView property
- •ParentRelations property
- •PrimaryKey property
- •Rows property
- •Dispose method
- •NewRow method
- •Review
- •Quiz Yourself
- •What Is Data Binding?
- •Binding to Arrays and Extended Object Types
- •Binding to Database Data
- •Binding to XML
- •TreeView Control
- •Implement the TreeView server control
- •Review
- •Quiz Yourself
- •DataGrid Control Basics
- •Binding a set of data to a DataGrid control
- •Formatting the output of a DataGrid control
- •Master/Detail Relationships with the DataGrid Control
- •Populating the Master control
- •Filtering the detail listing
- •Review
- •QUIZ YOURSELF
- •Updating Your Data
- •Handling the OnEditCommand Event
- •Handling the OnCancelCommand Event
- •Handling the OnUpdateCommand Event
- •Checking that the user input has been validated
- •Executing the update process
- •Deleting Data with the OnDeleteCommand Event
- •Sorting Columns with the DataGrid Control
- •Review
- •Quiz Yourself
- •What Is Data Shaping?
- •Why Shape Your Data?
- •DataSet Object
- •Shaping Data with the Relations Method
- •Review
- •Quiz Yourself
- •OLEDBError Object Description
- •OLEDBError Object Properties
- •OLEDBError Object Methods
- •OLEDBException Properties
- •Writing Errors to the Event Log
- •Review
- •Quiz Yourself
- •Introducing SOAP
- •Accessing Remote Data with SOAP
- •SOAP Discovery (DISCO)
- •Web Service Description Language (WSDL)
- •Using SOAP with ASP.NET
- •Review
- •Quiz Yourself
- •Developing a Web Service
- •Consuming a Web Service
- •Review
- •Quiz Yourself
- •ASP and ASP.NET Compatibility
- •Scripting language limitations
- •Rendering HTML page elements
- •Using script blocks
- •Syntax differences and language modifications
- •Running ASP Pages under Microsoft.NET
- •Using VB6 Components with ASP.NET
- •Review
- •Quiz Yourself
- •Preparing a Migration Path
- •ADO and ADO.NET Compatibility
- •Running ADO under ASP.NET
- •Early Binding ADO COM Objects in ASP.NET
- •Review
- •Quiz Yourself
- •Answers to Part Reviews
- •Friday Evening Review Answers
- •Saturday Morning Review Answers
- •Saturday Afternoon Review Answers
- •Saturday Evening Review Answers
- •Sunday Morning Review Answers
- •Sunday Afternoon Review Answers
- •What’s on the CD-ROM
- •System Requirements
- •Using the CD with Windows
- •What’s on the CD
- •The Software Directory
- •Troubleshooting
- •ADO.NET Class Descriptions
- •Coding Differences in ASP and ASP.NET
- •Retrieving a Table from a Database
- •Displaying a Table from a Database
- •Variable Declarations
- •Statements
- •Comments
- •Indexed Property Access
- •Using Arrays
- •Initializing Variables
- •If Statements
- •Case Statements
- •For Loops
- •While Loops
- •String Concatenation
- •Error Handling
- •Conversion of Variable Types
- •Index
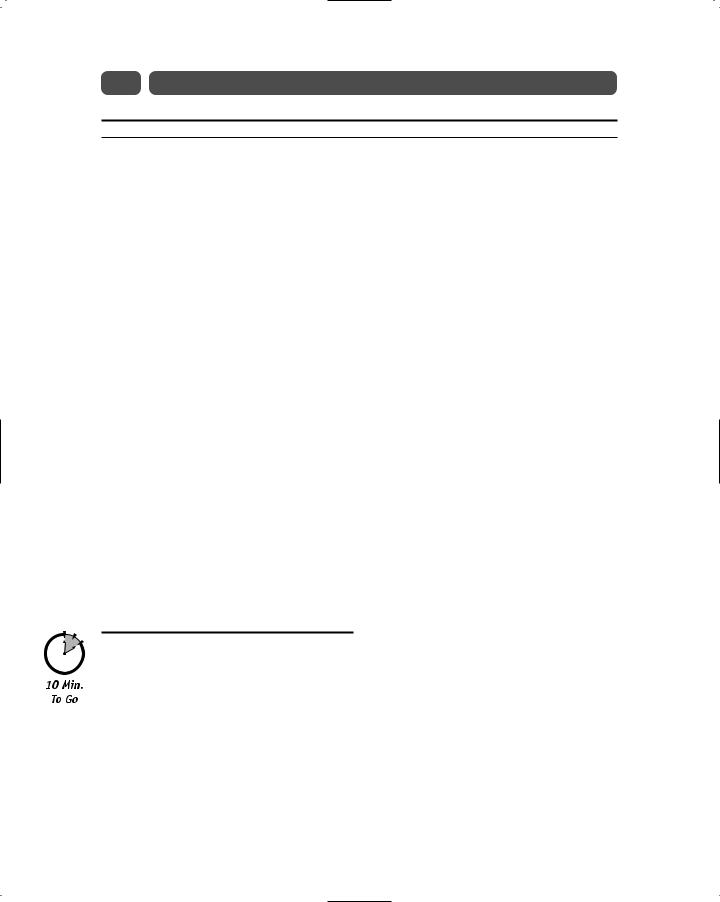
94 |
Saturday Morning |
Listing 10-2 |
Continued |
End Property
Public Property BGColor As System.Drawing.Color Get
BGColor = Address.BackColor
End Get Set
Address.BackColor = value End Set
End Property </script>
Add this code to the top of the Address User Control file. Notice the last two properties listed, FontColor and BGColor. These properties will get and set the font color for all the User Control’s labels and the background color of the panel that contains the User Control’s controls. Now add the following code to the script block at the top of your ASP.NET page to test your new properties:
<script language=”VB” runat=”server”>
Sub Page_Load(Sender As Object, E As EventArgs) With AddressControl
.BGColor = System.Drawing.Color.Blue
.FontColor = System.Drawing.Color.White
.Address1 = “100 ASP.NET St.”
.City = “Microsoft”
.StateID = 3
.ZipCode = “11111” End With
End Sub </script>
Pretty cool, huh? As you can see, the amount of code you’ll need to write will be greatly reduced once you create a decent sized library of User Controls. And this is just the beginning; you could expose even more properties to make your controls infinitely customizable.
Writing Custom Control Methods
In addition to creating properties, you can write custom methods for a User Control. Custom methods can be used to populate a list box, validate controls, and so on. The possibilities are endless. In the following example, we will add a method to Address User Control that will validate user input and return a Boolean value (true or false) based on the result of the validation. Include the following code within the <script> block in the address.ascx page:
Public Function ValidateAddress() As Boolean If Trim(txtAddress1.text) = “” Then
return False Exit Function
End If
If Trim(txtCity.text) = “” Then return False
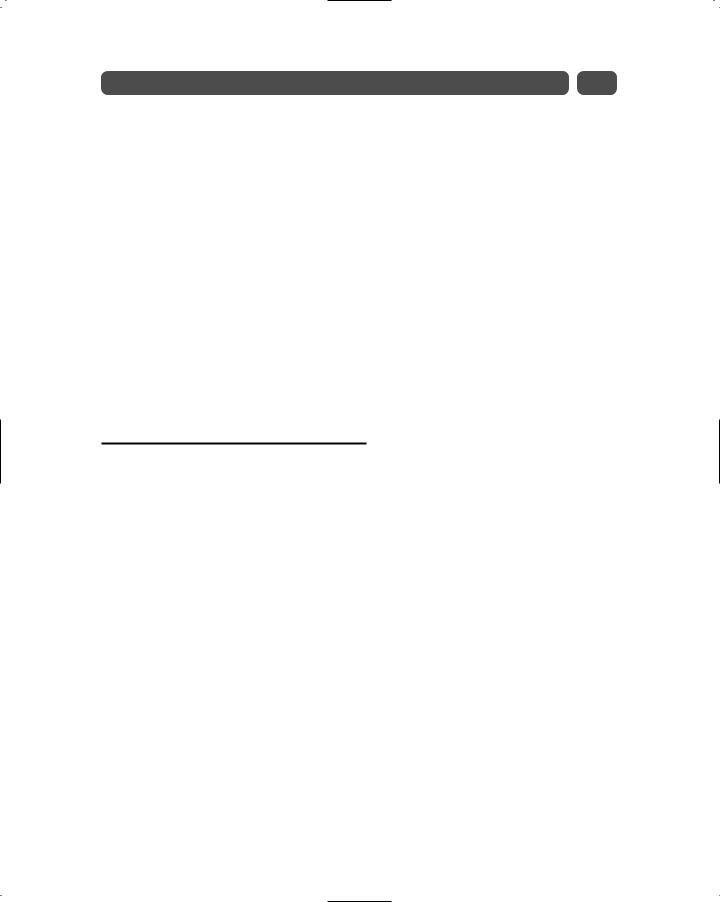
Session 10—Introducing User Controls |
95 |
Exit Function End If
If cmbState.SelectedIndex = 0 Then return False
Exit Function End If
If Trim(txtZipCode.text) = “” Then return False
Exit Function End If
return True End Function
As you can see in the ValidateAddress method, we do a simple check of a few selected fields and return a Boolean value based on the result. Pretty simple stuff, but it illustrates implementing a User Control method fairly well. To test this method, simply add the following line of code to the ASP.NET page after declaring the server control:
<%
Response.Write(AddressControl.ValidateAddress)
%>
Implementing User Control Events
User Controls can also respond to events and even respond to ASP.NET events like
Page_OnLoad.
Try putting the following code in the <script> block of address.ascx:
Sub Page_Load(Sender As Object, E As EventArgs) If Not Page.isPostBack Then
Dim oItem As New ListItem With oItem
.Value = 10
.Text = “Georgia” End With cmbState.Items.Add(oItem)
End If End Sub
Now try running the ASP.NET page. A Georgia item should now appear in the State list box. Imagine writing a little routine in the User Control that could populate controls from a database.
Because User Controls are a collection of ASP.NET controls, you have to use the same methods as with ASP.NET if you want to implement a control event handler. The first thing you want to do is write an event handling method. Add the following method, called btnSubmit_Click for example, to the address.ascx file:
Private Sub btnSubmit_Click(Sender As Object, E As EventArgs) Response.Write(“Submit button was clicked!”)
End Sub
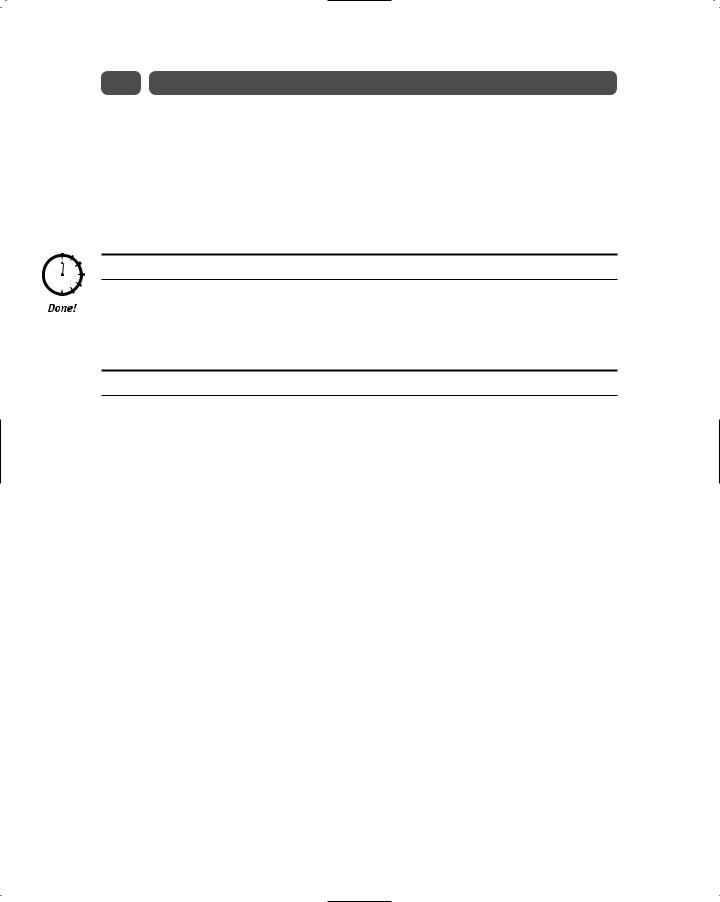
96 |
Saturday Morning |
Now in the address.ascx file, change the btnSubmit button control declaration to the following:
<asp:Button ID=btnSubmit text=”Submit” onClick=”btnSubmit_Click” runat=”server” />
Try it out now! You see that now, not only is the Web Form submitted, but the btnSubmit_Click event is also processed. It’s that simple.
REVIEW
User Controls are a nice feature of ASP.NET. They essentially enable you to write reusable custom controls based on the standard HTML and Web controls. You can also add custom properties, methods, and events to allow runtime User Control customization and interaction. Using User Controls, you can improve application performance as well as maintainability.
QUIZ YOURSELF
1.What is a User Control? (See session introduction.)
2.What directive can you use in an ASP.NET page to import a User Control? (See “Creating a User Control.”)
3.Why shouldn’t you include the <html>, <body>, and <form> tags in a User Control? (See “Creating a User Control.”)
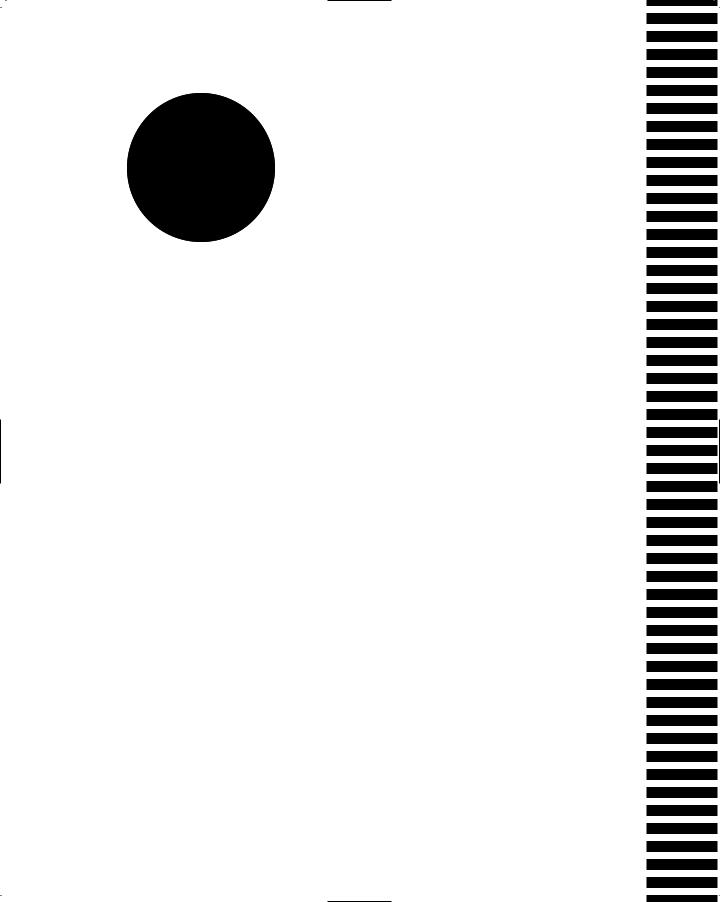
P A R T
II
Saturday Morning
Part Review
The following set of questions is designed to provide you with feedback on how well you understood the topics covered during this part of the book. Please refer to Appendix A for the answers to each question.
1.SQL is used to create and modify data. True/False
2.Fill in the blanks: The CRUD activities are ______, ______, ______, and
______.
3.Which of the following SQL statements is used to add data to a table?
a.CREATE
b.ADD
c.INSERT
d.UPDATE
4.INSERT INTO t_bands (band_title) VALUES (Hootie & The Blowfish) is a valid SQL command. Note: Assume band_title is VARCHAR(100).
True/False
5.XML is the de facto language for the exchange of data between applications.
True/False
6.Fill in the blank: XML data is stored in a ______ format.
7.XML is compatible with SGML. True/False
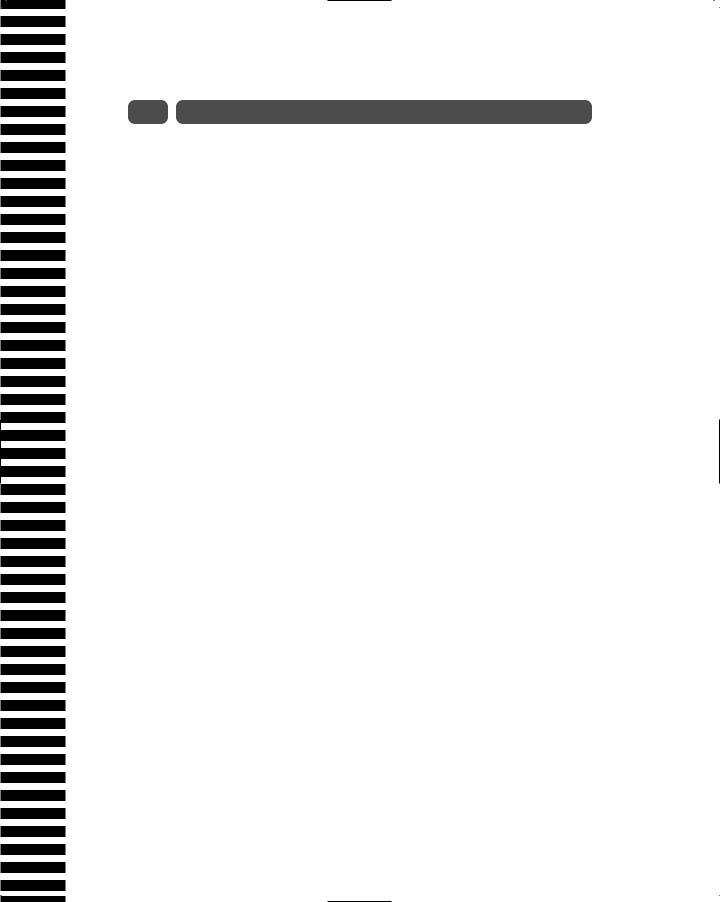
98 |
Part II–Saturday Morning Part Review |
8.XML was designed to work with only a few applications. True/False
9.ASP.NET pages are procedural in nature. True/False
10.Fill in the blank: When an ASP.NET page is loaded, a structured series of
______ are fired in a set order.
11.Fill in the blanks: The first event to be fired when a page is loaded is the
______ event and the last to be fired is the ______ event.
12.Which of the following attributes in the <SCRIPT> element specifies the language used in the code block?
a.SRC
b.TEXT
c.LANGUAGE
d.LINGO
13.HTML controls maintain their state between client requests. True/False
14.HTML controls can be used only when the browser requesting the page is Internet Explorer 4.0 or higher.
True/False
15.The name of the hidden field to maintain control state between client requests in ASP.NET page is
a.__VIEWSTATE
b.__STATEMAINT
c.__STATE
d.__VIEW
16.Which of the following is not an intrinsic HTML control?
a.<form>
b.<select>
c.<html>
d.<table>
17.Web controls map one-to-one with HTML elements. True/False
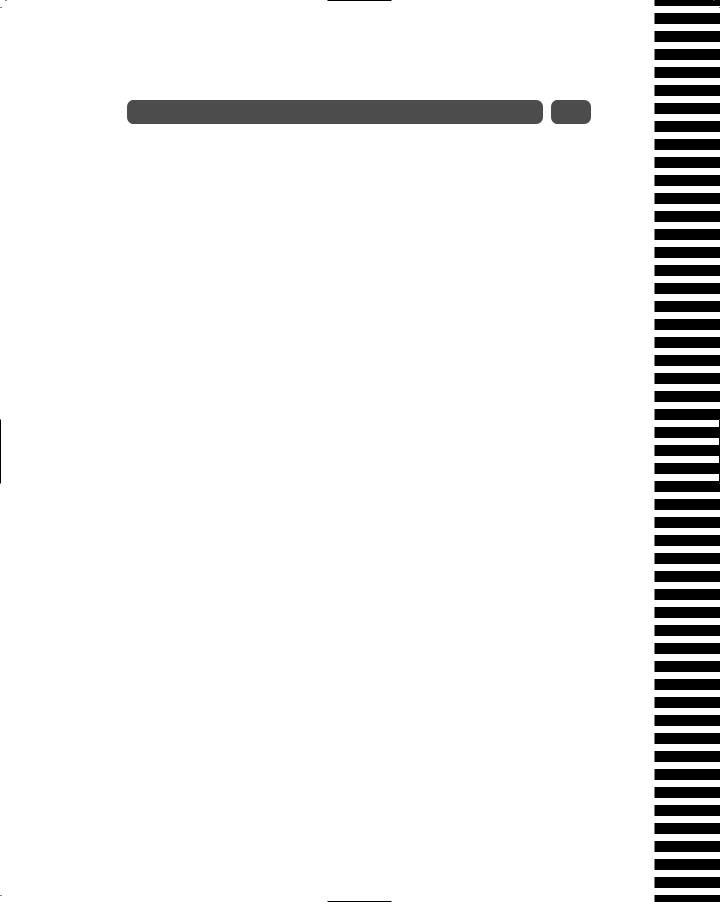
Part II–Saturday Morning Part Review |
99 |
18.Web controls can only be bound to DataSets. True/False
19.Web controls must be added to an ASP.NET page at design-time. True/False
20.Which of the following is not a List control?
a.ListBox
b.DataList
c.Table
d.DataGrid
21.ASP.NET User Controls have an .aspx file extension. True/False
22.User Controls provide an easy way to partition and reuse simple, common user interface (UI) functionality across a Web application.
True/False
23.User Controls should include <html>, <body>, and <form> elements. True/False
24.Which of the following ASP.NET page directives is used to register a User Control?
a.Register
b.Page
c.Control
d.Include
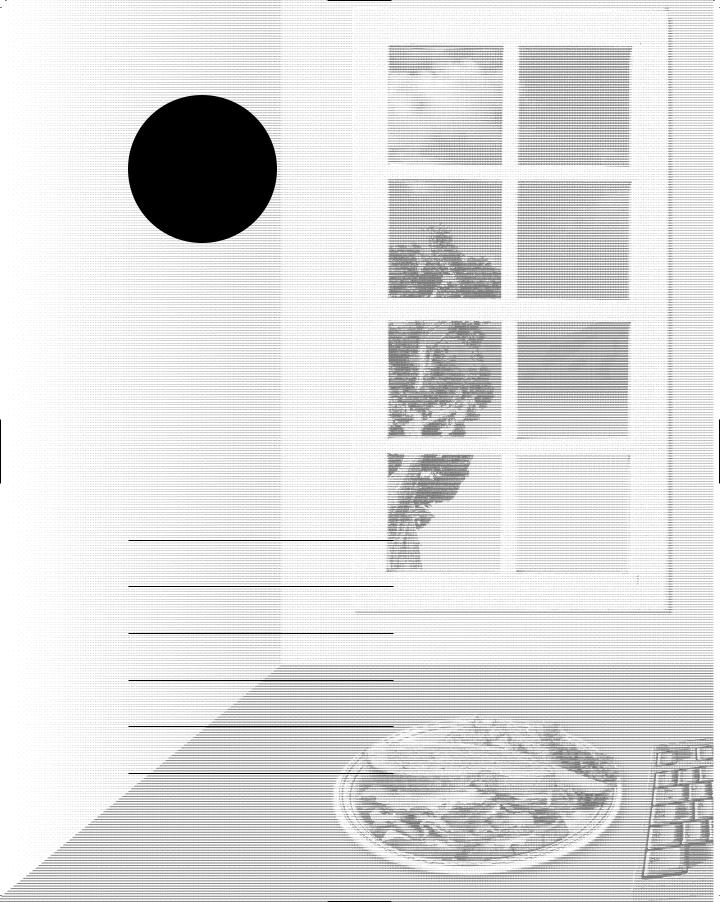
P A R T
III
Saturday
Afternoon
Session 11
Validating User Input
Session 12
Maintaining State in ASP.NET
Session 13
Authentication and Authorization
Session 14
ASP.NET Caching
Session 15
Introducing ADO.NET
Session 16
Navigating the ADO.NET Object Model
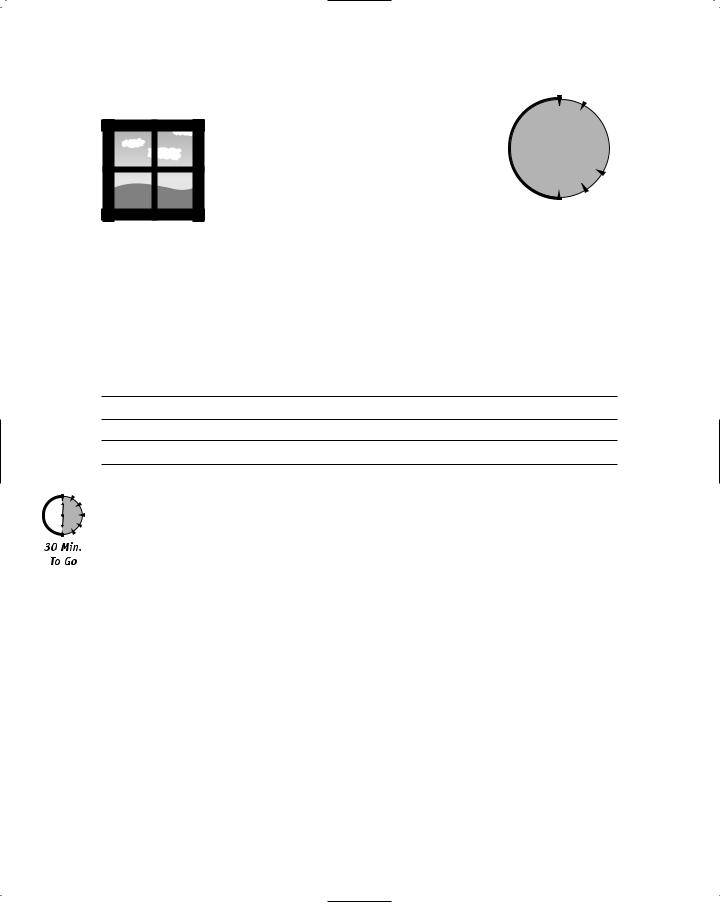
S E S S I O N
11
Validating User Input
Session Checklist
Understanding the use and implementation of ASP.NET validation controls
Using serverand client-side validation with ASP.NET
Building your own custom validation controls
When developing applications that put a heavy emphasis on end-user data, one of the most tedious and time-consuming activities for a developer is validating user input. Fortunately, ASP.NET provides a series of controls that can perform both
client-side and server-side validation. These controls include:
The RequiredFieldValidator control insures that a user either provides a value for a control or in some way modifies the initial values of a control.
The CompareValidator control checks to make sure a control contains a specific value or matches the value contained in a second control.
The RangeValidator control ensures that the user-provided value for a control falls within a specified range, or that the value falls within a range specified by other form controls.
The RegularExpressionValidator control supports the use of regular expressions to validate control values, providing an extensively flexible technique for validating credit card numbering sequences, e-mail addresses, or any other consistent expressions.
The CustomValidator control enables the developer to define any serveror clientside function to validate against, therefore covering any remaining validation not provided for in the first four controls.
The ValidationSummary control allows you to collect all of the validation errors and provide a consolidated listing to the user.