
Lectures / lecture4
.pdf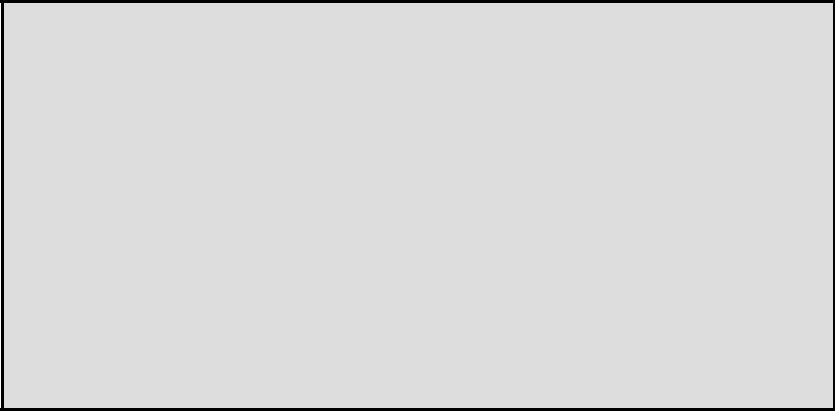
Overriding equals in Employee
An example of overriding the equals method in the Employee class compares every field for equality:
1public boolean equals (Object o) {
2boolean result = false;
3if ((o != null) && (o instanceof Employee)) {
4Employee e = (Employee)o;
5if ((e.empId == this.empId) &&
6 |
(e.name.equals(this.name)) && |
7 |
(e.ssn.equals(this.ssn)) && |
8 |
(e.salary == this.salary)) { |
9 |
result = true; |
10 |
} |
11}
12return result;
13}
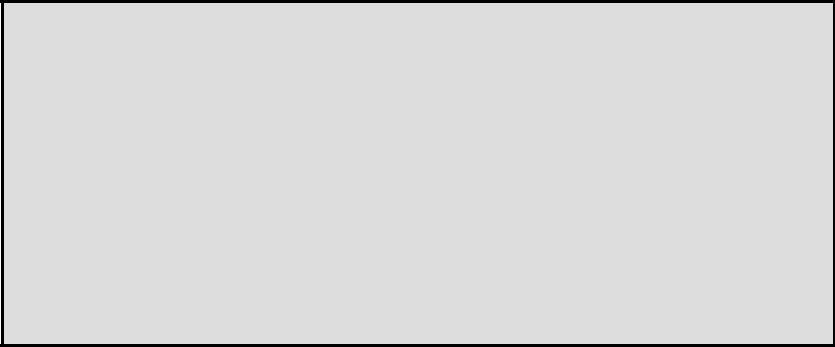
Overriding Object hashCode
The general contract for Object states that if two objects are considered equal (using the equals method), then integer hashcode returned for the two objects should also be equal.
1// Generated by NetBeans
2public int hashCode() {
3int hash = 7;
4hash = 83 * hash + this.empId;
5hash = 83 * hash + Objects.hashCode(this.name);
6hash = 83 * hash + Objects.hashCode(this.ssn);
7hash = 83 * hash +
8 |
(int)(Double.doubleToLongBits(this.salary) ^ |
9 |
(Double.doubleToLongBits(this.salary) >>> 32)); |
10return hash;
11}
Quiz
What is the purpose of the else block in an if/else statement?
a.To contain the remainder of the code for a method
b.To contain code that is executed when the expression in an if statement is false
c.To test if an expression is false
Quiz
Which of the following sentences is suitable for testing a value in a switch construct?
a.The switch construct tests whether values are greater than or less than a single value.
b.The switch construct tests against a single variable.
c.The switch construct tests the value of a float, double, or boolean data type and String.

Modeling Business Problems with
Classes
Inheritance (or subclassing) is an essential feature of the Java programming language. Inheritance provides code reuse through:
–Method inheritance: Subclasses avoid code duplication by inheriting method implementations.
–Generalization: Code that is designed to rely on the most generic type possible is easier to maintain.
Class Inheritance Diagram

Enabling Generalization
Coding to a common base type allows for the introduction of new subclasses with little or no modification of any code that depends on the more generic base type.
ElectronicDevice dev = new Television();
dev.turnOn(); // all ElectronicDevices can be turned on
Always use the most generic reference type possible.
•Generalization is the process of extracting shared characteristics from two or more classes, and combining them into a generalized superclass. Shared characteristics can be attributes, associations, or methods.
•In contrast to generalization, specialization means creating new subclasses from an existing class. If it turns out that certain attributes, associations, or methods only apply to some of the objects of the class, a subclass can be created. The most inclusive class in a generalization/specialization is called the superclass and is generally located at the top of the diagram. The more specific classes are called subclasses and are generally placed below the superclass.

Identifying the Need for Abstract Classes
Subclasses may not need to inherit a method implementation if the method is specialized.
public class Television extends ElectronicDevice {
public void turnOn() { changeChannel(1); initializeScreen();
}
public void turnOff() {}
public void changeChannel(int channel) {} public void initializeScreen() {}
}
•An abstract class is a class that is declared abstract—it may or may not include abstract methods. Abstract classes cannot be instantiated, but they can be subclassed.
•An abstract method is a method that is declared without an implementation (without braces, and followed by a semicolon), like this:
abstract void moveTo(double deltaX, double deltaY);
•If a class includes abstract methods, then the class itself must be declared abstract, as in:
public abstract class GraphicObject {
//declare fields
//declare nonabstract methods abstract void draw();
}
•When an abstract class is subclassed, the subclass usually provides implementations for all of the abstract methods in its parent class. However, if it does not, then the subclass must also be
declared abstract.
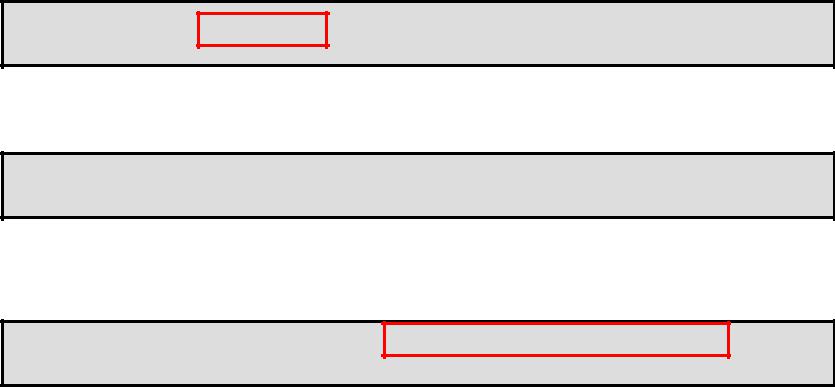
Defining Abstract Classes
A class can be declared as abstract by using the abstract class-level modifier.
public abstract class ElectronicDevice { }
– An abstract class can be subclassed.
public class Television extends ElectronicDevice { }
– An abstract class cannot be instantiated.
ElectronicDevice dev = new ElectronicDevice(); // error