
Lectures / lecture3_1
.pdf
Methods Using Variable Arguments
A variation of method overloading is when you need a method that takes any number of arguments of the same type:
public class Statistics {
public float average (int x1, int x2) {}
public float average (int x1, int x2, int x3) {}
public float average (int x1, int x2, int x3, int x4) {}
}
–These three overloaded methods share the same functionality. It would be nice to collapse these
methods into one method.
Statistics |
stats = new Statistics (); |
||||
float avg1 |
= stats.average(100, 200); |
||||
float |
avg2 |
= |
stats.average(100, |
200, |
300); |
float |
avg3 |
= |
stats.average(100, |
200, |
300, 400); |
|
|
|
|
|
|
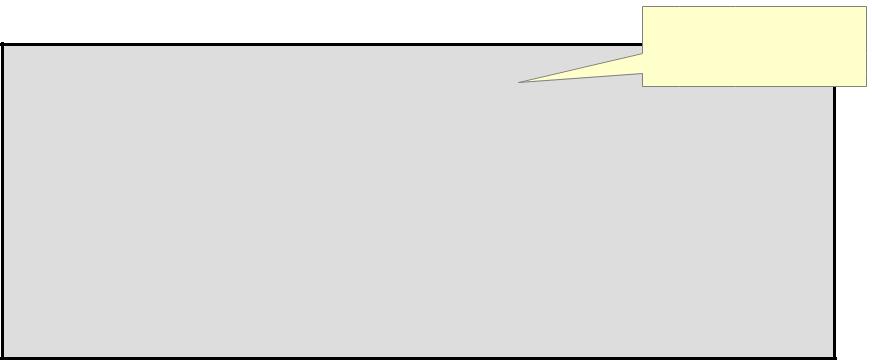
Methods Using Variable Arguments
–Java provides a feature called varargs or variable arguments.
The varargs notation treats the nums
parameter as an array.
public class Statistics {
public float average(int... nums) { int sum = 0;
for (int x : nums) { // iterate int array nums sum += x;
}
return ((float) sum / nums.length);
}
}
–Note that the nums argument is actually an array object of type int[]. This permits the method to iterate over and allow any number of elements.
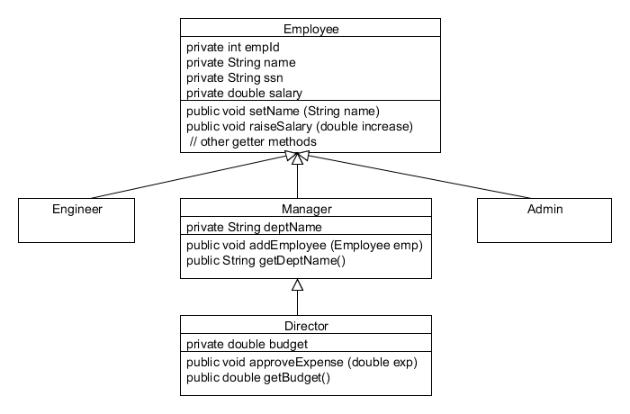
Single Inheritance
•The Java programming language permits a class to extend only one other class. This is called single inheritance.

Quiz
Given the diagram in the slide titled “Single Inheritance” and the following Java statements, which statements do not compile?
Employee e = new Director();
Manager m = new Director();
Admin a = new Admin();
a.
b.
c.
e.addEmployee (a);
m.addEmployee(a);
m.approveExpense(100000.00);
d.All of them fail to compile.
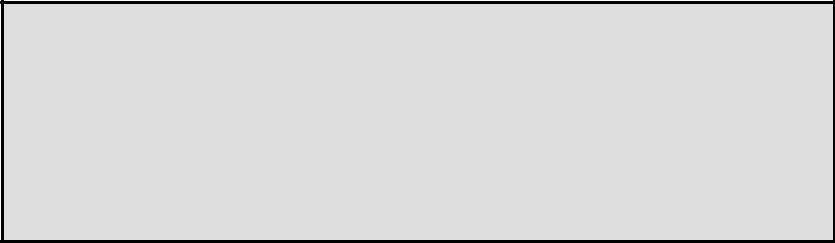
Quiz
Consider the following classes that do not compile:
public class Account { private double balance;
public Account(double balance) { this.balance = balance; } //... getter and setter for balance
}
public class Savings extends Account { private double interestRate;
public Savings(double rate) { interestRate = rate; }
}
What fix allows these classes to compile?
a.Add a no-arg constructor to Savings.
b.Call the setBalance method of Account from Savings.
c.Change the access of interestRate to public.
d.Replace the constructor in Savings with one that calls the constructor of Account using super.
Quiz
Which of the following declarations demonstrates the application of good Java naming conventions?
a.public class repeat { }
b.public void Screencoord (int x, int y){}
c.private int XCOORD;
d.public int calcOffset (int x1, int y1,
int x2, int y2) { }
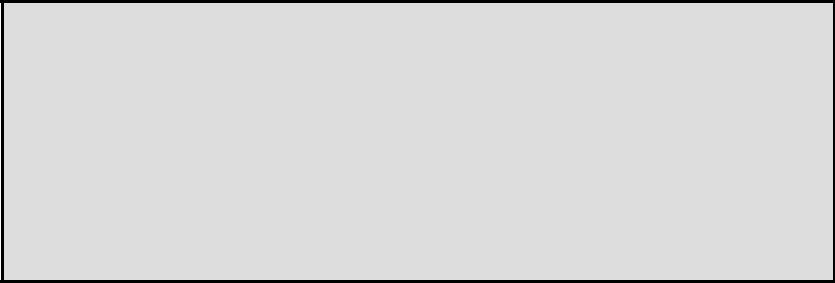
Quiz
What changes would you perform to make this class immutable? (Choose all that apply.)
public class Stock { public String symbol;
public double price; public int shares;
public double getStockValue() { }
public void setSymbol(String symbol) { } public void setPrice(double price) { }
public void setShares(int number) { }
}
a.Make the fields symbol, shares, and price private.
b.Remove setSymbol, setPrice, and setShares.
c.Make the getStockValue method private.
d.Add a constructor that takes symbol, shares, and price as arguments.