
- •Practical Unit Testing with JUnit and Mockito
- •Table of Contents
- •About the Author
- •Acknowledgments
- •Preface
- •Preface - JUnit
- •Part I. Developers' Tests
- •Chapter 1. On Tests and Tools
- •1.1. An Object-Oriented System
- •1.2. Types of Developers' Tests
- •1.2.1. Unit Tests
- •1.2.2. Integration Tests
- •1.2.3. End-to-End Tests
- •1.2.4. Examples
- •1.2.5. Conclusions
- •1.3. Verification and Design
- •1.5. Tools Introduction
- •Chapter 2. Unit Tests
- •2.1. What is a Unit Test?
- •2.2. Interactions in Unit Tests
- •2.2.1. State vs. Interaction Testing
- •2.2.2. Why Worry about Indirect Interactions?
- •Part II. Writing Unit Tests
- •3.2. Class To Test
- •3.3. Your First JUnit Test
- •3.3.1. Test Results
- •3.4. JUnit Assertions
- •3.5. Failing Test
- •3.6. Parameterized Tests
- •3.6.1. The Problem
- •3.6.2. The Solution
- •3.6.3. Conclusions
- •3.7. Checking Expected Exceptions
- •3.8. Test Fixture Setting
- •3.8.1. Test Fixture Examples
- •3.8.2. Test Fixture in Every Test Method
- •3.8.3. JUnit Execution Model
- •3.8.4. Annotations for Test Fixture Creation
- •3.9. Phases of a Unit Test
- •3.10. Conclusions
- •3.11. Exercises
- •3.11.1. JUnit Run
- •3.11.2. String Reverse
- •3.11.3. HashMap
- •3.11.4. Fahrenheits to Celcius with Parameterized Tests
- •3.11.5. Master Your IDE
- •Templates
- •Quick Navigation
- •Chapter 4. Test Driven Development
- •4.1. When to Write Tests?
- •4.1.1. Test Last (AKA Code First) Development
- •4.1.2. Test First Development
- •4.1.3. Always after a Bug is Found
- •4.2. TDD Rhythm
- •4.2.1. RED - Write a Test that Fails
- •How To Choose the Next Test To Write
- •Readable Assertion Message
- •4.2.2. GREEN - Write the Simplest Thing that Works
- •4.2.3. REFACTOR - Improve the Code
- •Refactoring the Tests
- •Adding Javadocs
- •4.2.4. Here We Go Again
- •4.3. Benefits
- •4.4. TDD is Not Only about Unit Tests
- •4.5. Test First Example
- •4.5.1. The Problem
- •4.5.2. RED - Write a Failing Test
- •4.5.3. GREEN - Fix the Code
- •4.5.4. REFACTOR - Even If Only a Little Bit
- •4.5.5. First Cycle Finished
- •‘The Simplest Thing that Works’ Revisited
- •4.5.6. More Test Cases
- •But is It Comparable?
- •Comparison Tests
- •4.6. Conclusions and Comments
- •4.7. How to Start Coding TDD
- •4.8. When not To Use Test-First?
- •4.9. Should I Follow It Blindly?
- •4.9.1. Write Good Assertion Messages from the Beginning
- •4.9.2. If the Test Passes "By Default"
- •4.10. Exercises
- •4.10.1. Password Validator
- •4.10.2. Regex
- •4.10.3. Booking System
- •Chapter 5. Mocks, Stubs, Test Spies
- •5.1. Introducing Mockito
- •5.1.1. Creating Test Doubles
- •5.1.2. Expectations
- •5.1.3. Verification
- •5.1.4. Conclusions
- •5.2. Types of Test Double
- •5.2.1. Code To Be Tested with Test Doubles
- •5.2.2. The Dummy Object
- •5.2.3. Test Stub
- •5.2.4. Test Spy
- •5.2.5. Mock
- •5.3. Putting it All Together
- •5.4. Example: TDD with Test Doubles
- •5.4.2. The Second Test: Send a Message to Multiple Subscribers
- •Refactoring
- •5.4.3. The Third Test: Send Messages to Subscribers Only
- •5.4.4. The Fourth Test: Subscribe More Than Once
- •Mockito: How Many Times?
- •5.4.5. The Fifth Test: Remove a Subscriber
- •5.4.6. TDD and Test Doubles - Conclusions
- •More Test Code than Production Code
- •The Interface is What Really Matters
- •Interactions Can Be Tested
- •Some Test Doubles are More Useful than Others
- •5.5. Always Use Test Doubles… or Maybe Not?
- •5.5.1. No Test Doubles
- •5.5.2. Using Test Doubles
- •No Winner So Far
- •5.5.3. A More Complicated Example
- •5.5.4. Use Test Doubles or Not? - Conclusion
- •5.6. Conclusions (with a Warning)
- •5.7. Exercises
- •5.7.1. User Service Tested
- •5.7.2. Race Results Enhanced
- •5.7.3. Booking System Revisited
- •5.7.4. Read, Read, Read!
- •Part III. Hints and Discussions
- •Chapter 6. Things You Should Know
- •6.1. What Values To Check?
- •6.1.1. Expected Values
- •6.1.2. Boundary Values
- •6.1.3. Strange Values
- •6.1.4. Should You Always Care?
- •6.1.5. Not Only Input Parameters
- •6.2. How to Fail a Test?
- •6.3. How to Ignore a Test?
- •6.4. More about Expected Exceptions
- •6.4.1. The Expected Exception Message
- •6.4.2. Catch-Exception Library
- •6.4.3. Testing Exceptions And Interactions
- •6.4.4. Conclusions
- •6.5. Stubbing Void Methods
- •6.6. Matchers
- •6.6.1. JUnit Support for Matcher Libraries
- •6.6.2. Comparing Matcher with "Standard" Assertions
- •6.6.3. Custom Matchers
- •6.6.4. Advantages of Matchers
- •6.7. Mockito Matchers
- •6.7.1. Hamcrest Matchers Integration
- •6.7.2. Matchers Warning
- •6.8. Rules
- •6.8.1. Using Rules
- •6.8.2. Writing Custom Rules
- •6.9. Unit Testing Asynchronous Code
- •6.9.1. Waiting for the Asynchronous Task to Finish
- •6.9.2. Making Asynchronous Synchronous
- •6.9.3. Conclusions
- •6.10. Testing Thread Safe
- •6.10.1. ID Generator: Requirements
- •6.10.2. ID Generator: First Implementation
- •6.10.3. ID Generator: Second Implementation
- •6.10.4. Conclusions
- •6.11. Time is not on Your Side
- •6.11.1. Test Every Date (Within Reason)
- •6.11.2. Conclusions
- •6.12. Testing Collections
- •6.12.1. The TDD Approach - Step by Step
- •6.12.2. Using External Assertions
- •Unitils
- •Testing Collections Using Matchers
- •6.12.3. Custom Solution
- •6.12.4. Conclusions
- •6.13. Reading Test Data From Files
- •6.13.1. CSV Files
- •6.13.2. Excel Files
- •6.14. Conclusions
- •6.15. Exercises
- •6.15.1. Design Test Cases: State Testing
- •6.15.2. Design Test Cases: Interactions Testing
- •6.15.3. Test Collections
- •6.15.4. Time Testing
- •6.15.5. Redesign of the TimeProvider class
- •6.15.6. Write a Custom Matcher
- •6.15.7. Preserve System Properties During Tests
- •6.15.8. Enhance the RetryTestRule
- •6.15.9. Make an ID Generator Bulletproof
- •Chapter 7. Points of Controversy
- •7.1. Access Modifiers
- •7.2. Random Values in Tests
- •7.2.1. Random Object Properties
- •7.2.2. Generating Multiple Test Cases
- •7.2.3. Conclusions
- •7.3. Is Set-up the Right Thing for You?
- •7.4. How Many Assertions per Test Method?
- •7.4.1. Code Example
- •7.4.2. Pros and Cons
- •7.4.3. Conclusions
- •7.5. Private Methods Testing
- •7.5.1. Verification vs. Design - Revisited
- •7.5.2. Options We Have
- •7.5.3. Private Methods Testing - Techniques
- •Reflection
- •Access Modifiers
- •7.5.4. Conclusions
- •7.6. New Operator
- •7.6.1. PowerMock to the Rescue
- •7.6.2. Redesign and Inject
- •7.6.3. Refactor and Subclass
- •7.6.4. Partial Mocking
- •7.6.5. Conclusions
- •7.7. Capturing Arguments to Collaborators
- •7.8. Conclusions
- •7.9. Exercises
- •7.9.1. Testing Legacy Code
- •Part IV. Listen and Organize
- •Chapter 8. Getting Feedback
- •8.1. IDE Feedback
- •8.1.1. Eclipse Test Reports
- •8.1.2. IntelliJ IDEA Test Reports
- •8.1.3. Conclusion
- •8.2. JUnit Default Reports
- •8.3. Writing Custom Listeners
- •8.4. Readable Assertion Messages
- •8.4.1. Add a Custom Assertion Message
- •8.4.2. Implement the toString() Method
- •8.4.3. Use the Right Assertion Method
- •8.5. Logging in Tests
- •8.6. Debugging Tests
- •8.7. Notifying The Team
- •8.8. Conclusions
- •8.9. Exercises
- •8.9.1. Study Test Output
- •8.9.2. Enhance the Custom Rule
- •8.9.3. Custom Test Listener
- •8.9.4. Debugging Session
- •Chapter 9. Organization Of Tests
- •9.1. Package for Test Classes
- •9.2. Name Your Tests Consistently
- •9.2.1. Test Class Names
- •Splitting Up Long Test Classes
- •Test Class Per Feature
- •9.2.2. Test Method Names
- •9.2.3. Naming of Test-Double Variables
- •9.3. Comments in Tests
- •9.4. BDD: ‘Given’, ‘When’, ‘Then’
- •9.4.1. Testing BDD-Style
- •9.4.2. Mockito BDD-Style
- •9.5. Reducing Boilerplate Code
- •9.5.1. One-Liner Stubs
- •9.5.2. Mockito Annotations
- •9.6. Creating Complex Objects
- •9.6.1. Mummy Knows Best
- •9.6.2. Test Data Builder
- •9.6.3. Conclusions
- •9.7. Conclusions
- •9.8. Exercises
- •9.8.1. Test Fixture Setting
- •9.8.2. Test Data Builder
- •Part V. Make Them Better
- •Chapter 10. Maintainable Tests
- •10.1. Test Behaviour, not Methods
- •10.2. Complexity Leads to Bugs
- •10.3. Follow the Rules or Suffer
- •10.3.1. Real Life is Object-Oriented
- •10.3.2. The Non-Object-Oriented Approach
- •Do We Need Mocks?
- •10.3.3. The Object-Oriented Approach
- •10.3.4. How To Deal with Procedural Code?
- •10.3.5. Conclusions
- •10.4. Rewriting Tests when the Code Changes
- •10.4.1. Avoid Overspecified Tests
- •10.4.2. Are You Really Coding Test-First?
- •10.4.3. Conclusions
- •10.5. Things Too Simple To Break
- •10.6. Conclusions
- •10.7. Exercises
- •10.7.1. A Car is a Sports Car if …
- •10.7.2. Stack Test
- •Chapter 11. Test Quality
- •11.1. An Overview
- •11.2. Static Analysis Tools
- •11.3. Code Coverage
- •11.3.1. Line and Branch Coverage
- •11.3.2. Code Coverage Reports
- •11.3.3. The Devil is in the Details
- •11.3.4. How Much Code Coverage is Good Enough?
- •11.3.5. Conclusion
- •11.4. Mutation Testing
- •11.4.1. How does it Work?
- •11.4.2. Working with PIT
- •11.4.3. Conclusions
- •11.5. Code Reviews
- •11.5.1. A Three-Minute Test Code Review
- •Size Heuristics
- •But do They Run?
- •Check Code Coverage
- •Conclusions
- •11.5.2. Things to Look For
- •Easy to Understand
- •Documented
- •Are All the Important Scenarios Verified?
- •Run Them
- •Date Testing
- •11.5.3. Conclusions
- •11.6. Refactor Your Tests
- •11.6.1. Use Meaningful Names - Everywhere
- •11.6.2. Make It Understandable at a Glance
- •11.6.3. Make Irrelevant Data Clearly Visible
- •11.6.4. Do not Test Many Things at Once
- •11.6.5. Change Order of Methods
- •11.7. Conclusions
- •11.8. Exercises
- •11.8.1. Clean this Mess
- •Appendix A. Automated Tests
- •A.1. Wasting Your Time by not Writing Tests
- •A.1.1. And what about Human Testers?
- •A.1.2. One More Benefit: A Documentation that is Always Up-To-Date
- •A.2. When and Where Should Tests Run?
- •Appendix B. Running Unit Tests
- •B.1. Running Tests with Eclipse
- •B.1.1. Debugging Tests with Eclipse
- •B.2. Running Tests with IntelliJ IDEA
- •B.2.1. Debugging Tests with IntelliJ IDEA
- •B.3. Running Tests with Gradle
- •B.3.1. Using JUnit Listeners with Gradle
- •B.3.2. Adding JARs to Gradle’s Tests Classpath
- •B.4. Running Tests with Maven
- •B.4.1. Using JUnit Listeners and Reporters with Maven
- •B.4.2. Adding JARs to Maven’s Tests Classpath
- •Appendix C. Test Spy vs. Mock
- •C.1. Different Flow - and Who Asserts?
- •C.2. Stop with the First Error
- •C.3. Stubbing
- •C.4. Forgiveness
- •C.5. Different Threads or Containers
- •C.6. Conclusions
- •Appendix D. Where Should I Go Now?
- •Bibliography
- •Glossary
- •Index
- •Thank You!
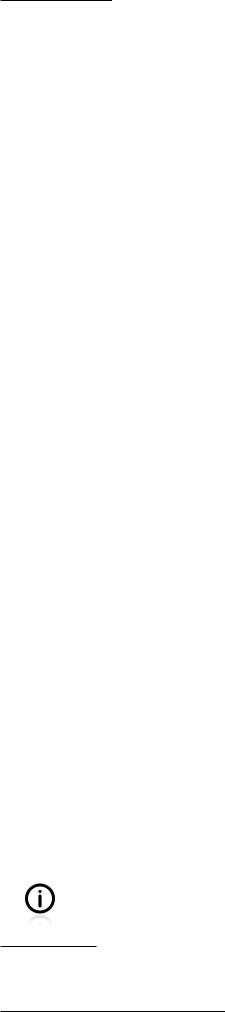
Chapter 1. On Tests and Tools
there? Why couldn’t one smart object deal with the client’s request? Well, the inhabitants of an OO world have very limited knowledge, and only a small set of abilities. Each of them has very constrained functionality or, to put it another way, each of them cannot do much on its own. Thus they are forced to cooperate in order to achieve anything useful (from the user’s point of view). This results in the following way of acting:
I am only a simple web controller, so I cannot fetch the data from the database for you. But I know a guy – call him UserDAO – that might help. So I will pass your request on to him. Ah! I have just remembered that UserDAO does not understand what an HTTP request is. I will extract the information he needs and pass it on to him. Let us wait now for his answer.
— Anonymous Web Controller Anonymous Web Application (circa 2003)
That is how it works. In fact, a lot of classes do nothing more than pass on messages, and maybe also transform them in some manner.
If you think about it, there are not many workers (that is classes that do a real job) out there. At least, not many workers written by you. Object-relational mapping framework (ORM3)? You surely will not have written one. After all, why should you, when there are so many robust solutions around? DependencyInjection container (DI4)? Not likely. Logging framework? No. If you think about the amount of real business logic in your application you might be surprised how little there is. Of course, you do have some business logic. That is why your customer placed an order for a new application. But you probably used a lot of ready-to-be-used elements that do a lot of work for you. And that is fine, because code reuse is a fantastic way to build applications fast, allowing you to concentrate exclusively on the customdesigned elements. But if so, then quite probably many of your classes are only tying things together by passing appropriate messages to appropriate collaborators. They coordinate the work of others. We will call such classes managers. Their work is substantially different from what workers do.
As you will soon see, this difference has a serious impact on testing.
1.2. Types of Developers' Tests
Having the picture of an OO system in mind, we can try to visualize the parts of the system affected by each type of test. This will help us to understand the scope and purpose of each kind of developers’ test.
But before we proceed, let me introduce two important terms that will be used throughout the book: SUT and DOC. Both were popularized by [meszaros2007] and are used frequently when discussing testing issues.
By SUT, or System Under Test, we understand the part of the system being tested. Depending on the type of test, SUT may be of very different granularity – from a single class to a whole application. A DOC, or Depended On Component, is any entity that is required by an SUT to fulfill its duties. Usually a DOC is of the same granularity as the SUT, e.g. if the SUT is a class, then it uses other classes, if it is a module, then it collaborates with other modules.
I will be using the terms "DOCs" and "collaborators" interchangeably.
3See http://en.wikipedia.org/wiki/Object-relational_mapping
4See http://en.wikipedia.org/wiki/Dependency_injection
3
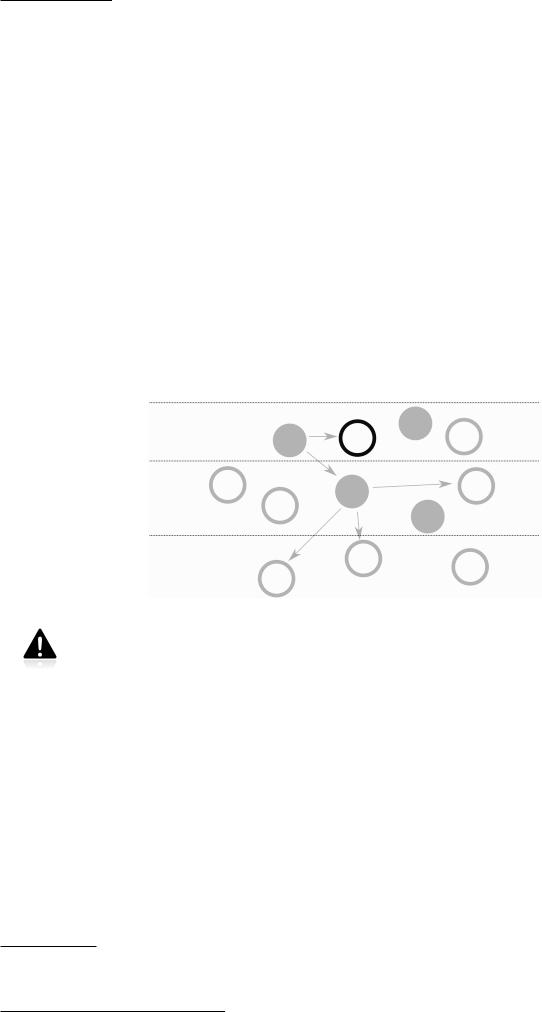
Chapter 1. On Tests and Tools
The following sections will introduce very briefly the various kinds of test. Much more could be said about each of them, but right now let us stick to the picture of the OO system and just try to see which part of it is covered by each kind of test.
1.2.1. Unit Tests
Unit tests focus on single classes. They exist to make sure that your code works. They control all aspects of the context in which the class to be tested is executed, by replacing real collaborators with test doubles5. They know nothing about the users of the system they put to the test, and are unaware of layers, external systems and resources. They run incredibly quickly, and are executed frequently.
This is shown in Figure 1.2, where only one object is clearly visible and all other elements of the system are greyed out. The single visible element is an SUT - the object to be tested. The greyed out elements symbolize those parts of the system not touched fully by the test, or replaced by various test doubles. Horizontal lines represent borders of layers (e.g. view, services, DAO layers). As the picture shows, a unit test is located inside one layer.
Figure 1.2. Scope of a unit test
Not every test run with a unit testing framework is a unit test! Make sure that your unit tests conform to the definition presented in Section 2.1!
1.2.2. Integration Tests
Integration tests focus on the proper integration of different modules of your code, including - and this is especially valuable - with code over which you have no control. An example might be a connection between your business classes and an OSGi container, ORM framework or with a web services framework. Even though the integration tests cover a much wider area of code than unit tests, they still test code as it looks from the developer’s standpoint.
Integration tests run much more slowly than unit tests. They usually require some resources (e.g. an application context) to be set up before they can be executed, and their execution involves calling some entities that tend to respond slowly (e.g. databases, file system or web services). In order to verify the results of integration tests, it is often necessary to look into external resources (e.g. issue an SQL query).
5Test doubles are fake replacements of real parts of the system (e.g. classes or modules). This topic will be discussed in detail in Chapter 5, Mocks, Stubs, Test Spies.
4
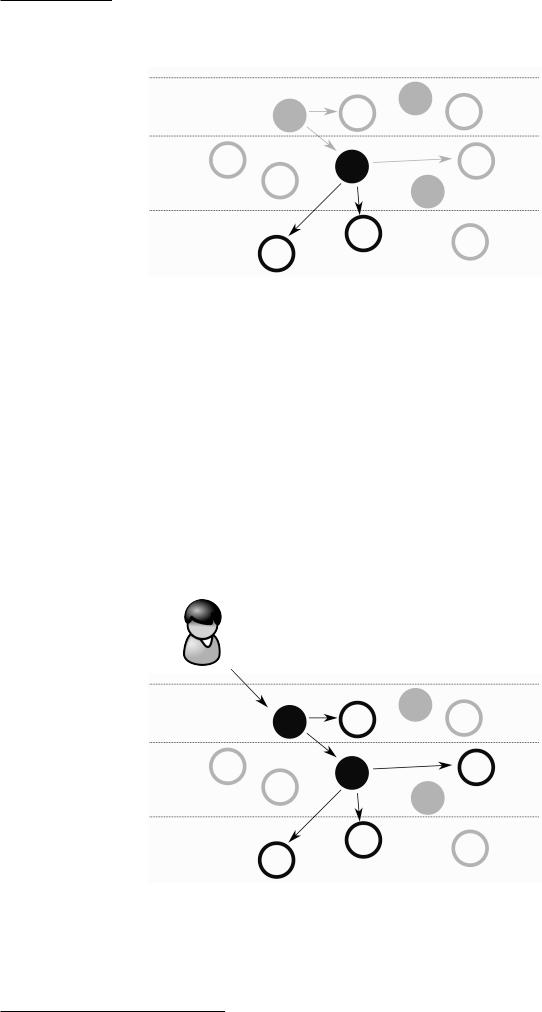
Chapter 1. On Tests and Tools
Figure 1.3. Scope of an integration test
As Figure 1.3 shows, integration tests usually extend across a few layers (e.g. when testing whether your services work correctly with a DAO layer). They execute code written by your team, but also code from third-party libraries used by the tested application. As with unit tests, vast areas of the system are either not touched by integration tests or are replaced by test doubles. Integration tests usually do not touch the user interface (the GUI). Because of this, the client (user of the system) is not shown in the picture.
1.2.3. End-to-End Tests
End-to-end tests exist to verify that your code works from the client’s point of view. They put the system as a whole to the test, mimicking the way the user would use it. As such they extend across all layers. Test doubles are rarely used in end-to-end tests – the point is to test the real system. End-to-end tests usually require a significant amount of time to execute themselves.
Figure 1.4. Scope of an end-to-end test
Figure 1.4 shows an end-to-end test that puts to the test elements from all layers - from the front end (GUI, web services layer or any other external API of the tested system) to the storage layers (e.g. database storage). End-to-end tests are initiated through a request identical to those issued by real users of the system (e.g. clicks on GUI elements).
5
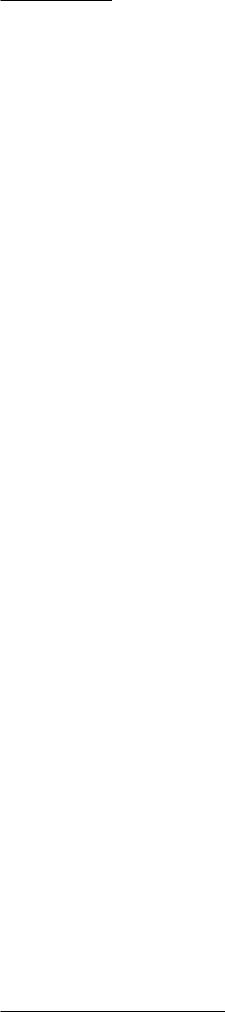
Chapter 1. On Tests and Tools
1.2.4. Examples
Table 1.1 gives examples of each type of tests.
Table 1.1. Types of test example
type of test |
test examples |
|
|
unit test |
• An object of the class FootballPlayer should change its status to fired after |
|
receiving a second yellow card. |
|
• A constructor of the class Product should throw an IllegalArgumentException |
|
(with meaningful message) if the price argument is less than 0. |
|
|
integration |
• An invocation of deleteAccount() method of the class UserService with an |
test |
argument ID of value 1 should result in removal of the account with this ID |
|
from the database. |
|
• When asked for an item with ID = 5 for a second time, the ItemDAO class |
|
should not touch the real database, but fetch the requested item from the cache |
|
instead. |
|
• ParcelService should communicate with some web service, in order to find |
|
the parcel’s details, and send an email with the appropriate error information |
|
(using EmailService), if the parcel is not found. |
|
|
end-to-end |
• A logged on user can add comments to any public picture by clicking on the |
test |
“add comment” button next to it. Guest users (users not logged on) can see |
|
this comment after it is published, but cannot post their own comments. |
|
• When a shop owner adds a new product to his shop using an Add Product |
|
form, it will be possible to locate this product using a Search Form by entering |
|
its name in the search field. |
|
• When a user sends his/her geo-location data using a whatCityIsThis web |
|
service, the system should respond with a city name. |
|
|
Table 1.2 presents examples of SUTs and DOCs for each type of test. It shows how SUTs and DOCs "grow" when moving from unit tests (smaller), via integration tests (medium), to end-to-end tests (large). The difference in granularity is clearly visible. In the case of unit tests, the SUTs and DOCs are simply classes. Integration tests act at the level of modules or layers. In the case of end-to-end tests, it is the whole application that is tested (making the application itself into an SUT), and other applications are collaborators (DOCs).
Table 1.2. Examples of SUT and DOC
type of test |
SUT example |
DOC example |
|
|
|
|
UserService |
UserDAO |
unit test |
|
|
Invoice |
Product |
|
|
|
|
|
Client |
Account |
|
|
|
|
DAO layer (ORM based) |
Hibernate |
|
|
|
integration test |
DAO layer (JDBC based) |
MySQL 5 |
|
|
|
|
FullTextIndexer module |
FileStorage module |
|
|
|
6