
- •Foreword
- •About Programmer’s Heaven
- •About Faraz Rasheed
- •Contents In Summary
- •Tools of the trade
- •The C# Language
- •The .Net Architecture and .Net Framework
- •The Common Language Runtime (CLR)
- •MSIL (Microsoft Intermediate Language) Code
- •Just In Time Compilers (JITers)
- •The Framework Class Library (FCL)
- •The Common Language Specification (CLS)
- •The Common Type System (CTS)
- •Garbage Collection (GC)
- •The .Net Framework
- •C# compared to C++
- •The Visual Studio.Net IDE
- •Projects and Solutions
- •Toolbox, Properties and Class View Tabs
- •Writing Your First Hello World Console Application in C#
- •Working Without Visual Studio.Net
- •With Visual Studio.Net
- •Understanding the Hello World Application Code:
- •Namespaces in C#
- •The using Keyword
- •The class Keyword
- •The Main() Method
- •Printing on the Console
- •Comments
- •Important points to remember
- •A more interactive Hello World Application
- •Discussing a more interactive Hello World Application
- •2. C# Language Fundamentals
- •Lesson Plan
- •Basic Data Types and their mapping to CTS (Common Type System)
- •Integral Types
- •Floating Point Types
- •Other Types
- •Variables
- •Constant Variables or Symbols
- •Naming Conventions for variables and methods
- •Operators in C#
- •Arithmetic Operators
- •Operand
- •Prefix and Postfix notation
- •Assignment Operators
- •Operand
- •Relational Operators
- •Operand
- •Operand
- •Other Operators
- •Operand
- •Operator Precedence
- •Flow Control And Conditional Statements
- •The if...else statement
- •The switch...case statement
- •Loops In C#
- •The for Loop
- •Some important points about the for loop
- •The do...while Loop
- •while Loop
- •Arrays in C#
- •Array Declaration
- •Accessing the values stored in an array
- •foreach Loop
- •3. Classes and Objects
- •Lesson Plan
- •Concept of a Class
- •Objects
- •Fields
- •Data Type
- •Methods
- •Instantiating the class
- •Accessing the members of a class
- •Access Modifiers or Accessibility Levels
- •Access Modifier
- •Properties
- •Using Properties
- •Precautions when using properties
- •Static Members of the class
- •Some More about Methods
- •Constructors
- •Finalize() Method of Object class
- •Destructors
- •Method and Constructor Overloading
- •Overloading Constructors
- •Value types (out & ref Keywords)
- •Reference types
- •Some more about references and objects
- •4. Inheritance & Polymorphism
- •Lesson Plan
- •Inheritance
- •Inheritance in C#
- •Implementing inheritance in C#
- •Constructor calls in Inheritance
- •The base keyword - Calling Constructors of the base-class explicitly
- •Protected Access Modifier
- •The Protected internal Access Modifier
- •The sealed keyword
- •Object class - the base of all classes
- •Polymorphism
- •Using the reference of the base type for referencing the objects of child types
- •Using methods with the same name in the Base and the Sub-class
- •Overriding the methods - virtual and override keywords
- •The new keyword
- •Type casting the objects - Up-casting and Down-casting
- •The is and as keywords
- •Boxing and Un-boxing
- •5. Structures, Enumeration, Garbage Collection & Nested Classes
- •Lesson Plan
- •Structures (struct)
- •Defining a struct
- •Instantiating the struct
- •structs as Value Types
- •Enumeration
- •The Need for Enumeration
- •Using Enumeration (enum)
- •More about Enumerations
- •Garbage Collection in .Net
- •Destructors and Performance Overhead
- •System.GC.Collect() method
- •Nested Classes in C#
- •6. Abstract Classes & Interfaces
- •Lesson Plan
- •Abstract Classes
- •Interfaces
- •Implementing More Than One Interface
- •Explicit implementation of methods
- •Casting to an interface using is and as operators
- •An interface inheriting one or more interfaces
- •7. Arrays, Collections & String Manipulation
- •Lesson Plan
- •Arrays Revisited
- •Multidimensional Arrays
- •Instantiating and accessing the elements of multidimensional arrays
- •Instantiating and accessing Jagged Arrays
- •Some other important points about multidimensional arrays
- •The foreach Loop
- •Collections
- •The ArrayList class
- •The Stack class
- •The Queue class
- •Dictionaries
- •The Hashtable class
- •Constructing a Hashtable
- •Adding items to a Hashtable
- •Retrieving items from the Hashtable
- •Removing a particular item
- •Getting the collection of keys and values
- •Checking for the existence of a particular item in a hashtable
- •The SortedList class
- •String Handling in C#
- •The string class and its members
- •The StringBuilder class
- •8. Exception Handling
- •Lesson Plan
- •Exceptions Basics
- •The need for Exceptions
- •Exceptions in C# and .Net
- •Handling Exceptions using the try...catch...finally blocks
- •Use of the try...catch block
- •Exception class' Message and StackTrace Properties
- •The finally block
- •Catching Multiple Exceptions using multiple catch blocks
- •An important point to remember in multiple catch blocks
- •Other important points about Exception Handling in C#
- •Defining your own custom exceptions
- •Exception Hierarchy in the .Net Framework
- •Throwing an exception: the throw keyword
- •9. Delegates & Events
- •Lesson Plan
- •Delegates Basics
- •The type or signature of the method the delegate can point to
- •The delegate reference, that can be used to reference a method
- •3.The actual method referenced by the delegate
- •Calling the actual method through its delegate
- •Confusion in terminology
- •Delegates in the .Net Framework
- •Passing delegates to methods
- •Multicast Delegates
- •Implementing a Multicast Delegate
- •Removing a method from the multicast delegate's invocation list
- •Events and Event Handling
- •Event Handling in C#
- •A Clock Timer Example
- •Multicast events
- •Passing some data with the Event: Sub-classing System.EventArgs
- •10. WinForms & Windows Applications
- •Lesson Plan
- •Windows Applications and .Net
- •WinForm Basics
- •Building the "Hello WinForm" Application
- •Understanding the Code
- •Adding Event Handling
- •Visual Studio.Net & its IDE (Integrated Development Environment)
- •IntelliSense and Hot Compiler
- •Code Folding
- •Integrated Compiler, Solution builder and Debugger
- •Form Designer
- •Solution Explorer
- •Menus in the Visual Studio .Net IDE
- •Using Visual Studio.Net to build the "Hello WinForm" Application
- •Creating a new Project
- •Setting various properties of the form
- •Adding Controls to the Form
- •Adding Event Handling
- •Executing the application
- •The code generated by the Form Designer
- •Using More Controls
- •Using various controls in an application: Programmer's Shopping Cart
- •Designing the form and placing the controls
- •Writing Code for Event Handling
- •Some Important Points for designing Windows Applications
- •11. More Windows Controls & Standard Dialog Boxes
- •Lesson Plan
- •Collection Controls
- •List Box Control
- •Adding items to the list box
- •Accessing items in the list box
- •Removing items from the list box
- •List Box Events
- •Combo Box Control
- •Tree View
- •The TreeNode Editor
- •Adding/Removing items at runtime
- •Tree View Events
- •Image List Control
- •Attaching An Image List to different controls
- •List View Control
- •Two Image Lists in the List View Control
- •Adding items to the list view control using designer
- •Adding Items at runtime using code
- •Events for List View Control
- •Main Menu
- •Tool Bar
- •Date Time Picker
- •Windows Standard Dialog Boxes
- •Open File Dialog Box
- •Using the Open File Dialog Box
- •Save File Dialog Box
- •Font and Color Dialog Boxes
- •12. Data Access using ADO.Net
- •Lesson Plan
- •Introducing ADO.Net
- •Different components of ADO.Net
- •A review of basic SQL queries
- •SQL SELECT Statement
- •SQL INSERT Statement
- •SQL UPDATE Statement
- •SQL DELETE Statement
- •Performing common data access tasks with ADO.Net
- •Accessing Data using ADO.Net
- •Defining the connection string
- •Defining a Connection
- •Defining the command or command string
- •Defining the Data Adapter
- •Creating and filling the DataSet
- •A Demonstration Application
- •Loading tables
- •Filling the controls on the Form
- •Navigating through the records
- •Updating the table
- •Building the Application
- •Loading the table and displaying data in the form's controls
- •Initialing Commands
- •Adding Parameters to the commands
- •The ToggleControls() method of our application
- •Editing (or Updating) Records
- •Event Handler for the Save Button
- •Event Handler for the Cancel Button
- •Inserting Records
- •Deleting a Record
- •Using Stored Procedures
- •Sample Stored Procedures
- •UPDATE Stored Procedure
- •INSERT Stored Procedure
- •DELETE Stored Procedure
- •SELECT Stored Procedure
- •Using Stored Procedures with ADO.Net in C#
- •The modified InitializeCommands() method
- •Using Data Grid Control to View .Net data
- •A Demonstration Application for Data Grid Control
- •Second Demonstration - Using multiple related tables
- •Retrieving data using the SELECT command
- •Updating Records using INSERT, UPDATE and DELETE commands
- •13. Multithreading
- •Lesson Plan
- •What is Multithreading
- •Multithreading in C#
- •Thread Functionality
- •Static members of the System.Threading.Thread class
- •Instance members of the System.Threaing.Thread class
- •Thread Demonstration Example - Basic Operations
- •Thread Demonstration Example - Thread Priority
- •Thread Demonstration Example - Thread Execution Control
- •Using Join() to wait for running threads
- •Thread Synchronization
- •The C# Locking Mechanism
- •Threads may cause Deadlock
- •14. The File System & Streams
- •Lesson Plan
- •Working with the File System
- •Obtaining the Application’s Environment Information – The System.Environment class
- •Demonstration Application – Environment Information
- •Obtaining the paths of various Windows Standard folders – Environment.GetFolderPath()
- •Manipulating Files using System.IO.File and System.IO.FileInfo classes
- •System.IO.File class
- •Creating a file using Create() method
- •Copying and Moving a file using Copy() and Move() methods
- •Checking the existence of the file using Exists() method
- •Getting Attributes of a file using GetAttributes() method
- •System.IO.FileInfo class
- •A quick and simple example
- •Manipulating Directories (folders) using System.IO.Directory and System.IO.DirectoryInfo classes
- •System.IO.Directory class
- •Creating, deleting and checking for the existence of directories
- •Getting the contents (files and sub-directories) of a directory
- •System.IO.DirectoryInfo class
- •Demonstration application for the DirectoryInfo class
- •Streams
- •An overview of the different types of streams
- •The System.Stream class – the base of all streams in the .Net framework
- •Different types of file streams – Reading and Writing to files
- •Using System.IO.FileStream to read and write data to files
- •A string representing the path and name of the file
- •Opening and reading from a file
- •Using BinaryReader and BinaryWriter to read and write primitives to files
- •Using StreamReader and StreamWriter to read and write text files
- •Serialization and De-serialization
- •Implementing Serialization and Deserialization – A simple example
- •Formatters in Serialization
- •Preventing certain elements from Serializing – The [NonSerialized] attribute
- •Getting notified when Deserializing - the IDeserializationCallBack interface
- •Asynchronous Reading and Writing with Streams
- •A demonstration application
- •Issues Regarding Asynchronous Read/Write
- •Important points regarding the use of Streams
- •15. New Features In C# 2.0
- •C# evolves
- •The need for generics
- •Generic collections
- •Creating generic types
- •Constraining type parameters
- •Final thoughts on generics
- •Partial types
- •Nullable types
- •Anonymous methods in event handling
- •Adventures with anonymous methods
- •Final thoughts on C# 2.0
- •16. The Road Ahead
- •Learning More
- •Getting Help
- •Book.revision++
- •Good Luck!
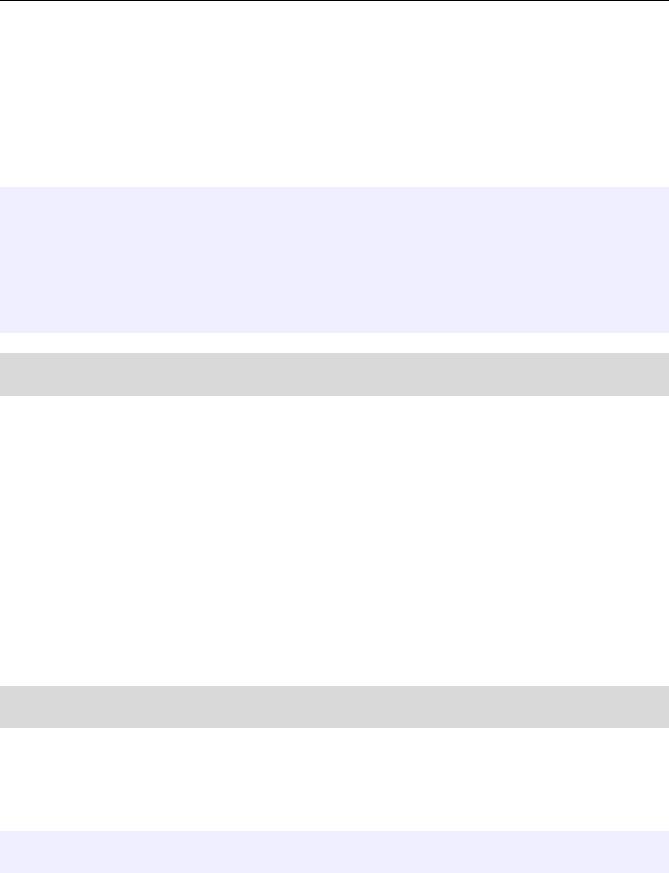
Programmers Heaven: C# School
A more interactive Hello World Application
Up until now, we have seen a very static hello world application that greets the whole world when it is executed. Let’s now make a more interactive hello world that greets the current user of it. This program will ask the user their name and will greet using his/her name, like 'Hello Faraz' when a user named 'Faraz' runs it. Let’s see the code first:
static void Main(string[] args)
{
Console.Write("Please enter your name: "); string name = Console.ReadLine(); Console.WriteLine
("Hello {0}, Good Luck in C#", name);
}
Author's Note: In the above code, we haven't shown the complete program but only the Main Method to save space. We will
follow this strategy in the rest of the course when appropriate.
Discussing a more interactive Hello World Application
In the first line, we have used another method, Write(), of the Console class. This is similar to the WriteLine() method, discussed in the previous program, but does not change the line after printing the string on the console.
In the second line, we declared a variable of the type string and called it 'name'. Then, we took a line of input from the user through the ReadLine() method of the Console class and stored the result in the 'name' variable. The variables are placeholders in memory for storing data temporarily during the execution of program. Variables can hold different types of data depending on their data-type, e.g., int variables can store integers while string variables can store a string (series) of characters. The ReadLine() method of the Console class (contrary to WriteLine()) reads a line of input given at the Console Window. It returns this input as string data, which we stored in our string variable 'name'.
Author's Note:
A string is implicit data-type in C# contrary to other languages. It starts with small 's'.
In the third line, we printed the name given by user in line 2, along with some greeting text using the WriteLine() method of the Console class. Here we used the substitution parameter {0} to state where in the line the data in the variable 'name' should be written when the WriteLine() method is called.
Console.WriteLine
("Hello {0}, Good Luck in C#", name);
When the compiler finds a substitution parameter, {n}, it replaces it with the (n+1)th variable following the string. The string is delimited with double quotation marks and each parameter is separated by a comma. Hence, in our
29
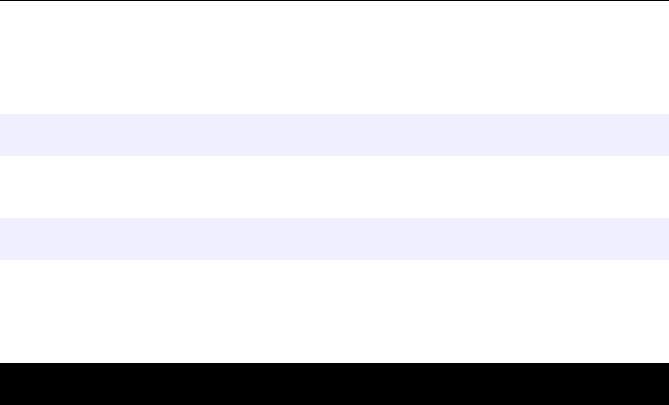
Programmers Heaven: C# School
case when the compiler finds {0}, it replaces it with (0+1)th, i.e., the 1st variable ('name') following the string. So at run-time, the CLR will read it as:
Console.WriteLine
("Hello Faraz, Good Luck in C#");
if the value of 'name' is Faraz at run-time. Alternatively, it can also be written as:
Console.WriteLine
("Hello " + name + ", Good Luck in C#");
removing the substitution parameter. Here we concatenate (add) the strings together to form a message. (The first approach is similar to C's printf() function while the second is similar to Java's System.out.println() method) When we compile and run this program the output will be:
Please enter your name: Faraz
Hello Faraz, Good Luck in C#
30