
- •Foreword
- •About Programmer’s Heaven
- •About Faraz Rasheed
- •Contents In Summary
- •Tools of the trade
- •The C# Language
- •The .Net Architecture and .Net Framework
- •The Common Language Runtime (CLR)
- •MSIL (Microsoft Intermediate Language) Code
- •Just In Time Compilers (JITers)
- •The Framework Class Library (FCL)
- •The Common Language Specification (CLS)
- •The Common Type System (CTS)
- •Garbage Collection (GC)
- •The .Net Framework
- •C# compared to C++
- •The Visual Studio.Net IDE
- •Projects and Solutions
- •Toolbox, Properties and Class View Tabs
- •Writing Your First Hello World Console Application in C#
- •Working Without Visual Studio.Net
- •With Visual Studio.Net
- •Understanding the Hello World Application Code:
- •Namespaces in C#
- •The using Keyword
- •The class Keyword
- •The Main() Method
- •Printing on the Console
- •Comments
- •Important points to remember
- •A more interactive Hello World Application
- •Discussing a more interactive Hello World Application
- •2. C# Language Fundamentals
- •Lesson Plan
- •Basic Data Types and their mapping to CTS (Common Type System)
- •Integral Types
- •Floating Point Types
- •Other Types
- •Variables
- •Constant Variables or Symbols
- •Naming Conventions for variables and methods
- •Operators in C#
- •Arithmetic Operators
- •Operand
- •Prefix and Postfix notation
- •Assignment Operators
- •Operand
- •Relational Operators
- •Operand
- •Operand
- •Other Operators
- •Operand
- •Operator Precedence
- •Flow Control And Conditional Statements
- •The if...else statement
- •The switch...case statement
- •Loops In C#
- •The for Loop
- •Some important points about the for loop
- •The do...while Loop
- •while Loop
- •Arrays in C#
- •Array Declaration
- •Accessing the values stored in an array
- •foreach Loop
- •3. Classes and Objects
- •Lesson Plan
- •Concept of a Class
- •Objects
- •Fields
- •Data Type
- •Methods
- •Instantiating the class
- •Accessing the members of a class
- •Access Modifiers or Accessibility Levels
- •Access Modifier
- •Properties
- •Using Properties
- •Precautions when using properties
- •Static Members of the class
- •Some More about Methods
- •Constructors
- •Finalize() Method of Object class
- •Destructors
- •Method and Constructor Overloading
- •Overloading Constructors
- •Value types (out & ref Keywords)
- •Reference types
- •Some more about references and objects
- •4. Inheritance & Polymorphism
- •Lesson Plan
- •Inheritance
- •Inheritance in C#
- •Implementing inheritance in C#
- •Constructor calls in Inheritance
- •The base keyword - Calling Constructors of the base-class explicitly
- •Protected Access Modifier
- •The Protected internal Access Modifier
- •The sealed keyword
- •Object class - the base of all classes
- •Polymorphism
- •Using the reference of the base type for referencing the objects of child types
- •Using methods with the same name in the Base and the Sub-class
- •Overriding the methods - virtual and override keywords
- •The new keyword
- •Type casting the objects - Up-casting and Down-casting
- •The is and as keywords
- •Boxing and Un-boxing
- •5. Structures, Enumeration, Garbage Collection & Nested Classes
- •Lesson Plan
- •Structures (struct)
- •Defining a struct
- •Instantiating the struct
- •structs as Value Types
- •Enumeration
- •The Need for Enumeration
- •Using Enumeration (enum)
- •More about Enumerations
- •Garbage Collection in .Net
- •Destructors and Performance Overhead
- •System.GC.Collect() method
- •Nested Classes in C#
- •6. Abstract Classes & Interfaces
- •Lesson Plan
- •Abstract Classes
- •Interfaces
- •Implementing More Than One Interface
- •Explicit implementation of methods
- •Casting to an interface using is and as operators
- •An interface inheriting one or more interfaces
- •7. Arrays, Collections & String Manipulation
- •Lesson Plan
- •Arrays Revisited
- •Multidimensional Arrays
- •Instantiating and accessing the elements of multidimensional arrays
- •Instantiating and accessing Jagged Arrays
- •Some other important points about multidimensional arrays
- •The foreach Loop
- •Collections
- •The ArrayList class
- •The Stack class
- •The Queue class
- •Dictionaries
- •The Hashtable class
- •Constructing a Hashtable
- •Adding items to a Hashtable
- •Retrieving items from the Hashtable
- •Removing a particular item
- •Getting the collection of keys and values
- •Checking for the existence of a particular item in a hashtable
- •The SortedList class
- •String Handling in C#
- •The string class and its members
- •The StringBuilder class
- •8. Exception Handling
- •Lesson Plan
- •Exceptions Basics
- •The need for Exceptions
- •Exceptions in C# and .Net
- •Handling Exceptions using the try...catch...finally blocks
- •Use of the try...catch block
- •Exception class' Message and StackTrace Properties
- •The finally block
- •Catching Multiple Exceptions using multiple catch blocks
- •An important point to remember in multiple catch blocks
- •Other important points about Exception Handling in C#
- •Defining your own custom exceptions
- •Exception Hierarchy in the .Net Framework
- •Throwing an exception: the throw keyword
- •9. Delegates & Events
- •Lesson Plan
- •Delegates Basics
- •The type or signature of the method the delegate can point to
- •The delegate reference, that can be used to reference a method
- •3.The actual method referenced by the delegate
- •Calling the actual method through its delegate
- •Confusion in terminology
- •Delegates in the .Net Framework
- •Passing delegates to methods
- •Multicast Delegates
- •Implementing a Multicast Delegate
- •Removing a method from the multicast delegate's invocation list
- •Events and Event Handling
- •Event Handling in C#
- •A Clock Timer Example
- •Multicast events
- •Passing some data with the Event: Sub-classing System.EventArgs
- •10. WinForms & Windows Applications
- •Lesson Plan
- •Windows Applications and .Net
- •WinForm Basics
- •Building the "Hello WinForm" Application
- •Understanding the Code
- •Adding Event Handling
- •Visual Studio.Net & its IDE (Integrated Development Environment)
- •IntelliSense and Hot Compiler
- •Code Folding
- •Integrated Compiler, Solution builder and Debugger
- •Form Designer
- •Solution Explorer
- •Menus in the Visual Studio .Net IDE
- •Using Visual Studio.Net to build the "Hello WinForm" Application
- •Creating a new Project
- •Setting various properties of the form
- •Adding Controls to the Form
- •Adding Event Handling
- •Executing the application
- •The code generated by the Form Designer
- •Using More Controls
- •Using various controls in an application: Programmer's Shopping Cart
- •Designing the form and placing the controls
- •Writing Code for Event Handling
- •Some Important Points for designing Windows Applications
- •11. More Windows Controls & Standard Dialog Boxes
- •Lesson Plan
- •Collection Controls
- •List Box Control
- •Adding items to the list box
- •Accessing items in the list box
- •Removing items from the list box
- •List Box Events
- •Combo Box Control
- •Tree View
- •The TreeNode Editor
- •Adding/Removing items at runtime
- •Tree View Events
- •Image List Control
- •Attaching An Image List to different controls
- •List View Control
- •Two Image Lists in the List View Control
- •Adding items to the list view control using designer
- •Adding Items at runtime using code
- •Events for List View Control
- •Main Menu
- •Tool Bar
- •Date Time Picker
- •Windows Standard Dialog Boxes
- •Open File Dialog Box
- •Using the Open File Dialog Box
- •Save File Dialog Box
- •Font and Color Dialog Boxes
- •12. Data Access using ADO.Net
- •Lesson Plan
- •Introducing ADO.Net
- •Different components of ADO.Net
- •A review of basic SQL queries
- •SQL SELECT Statement
- •SQL INSERT Statement
- •SQL UPDATE Statement
- •SQL DELETE Statement
- •Performing common data access tasks with ADO.Net
- •Accessing Data using ADO.Net
- •Defining the connection string
- •Defining a Connection
- •Defining the command or command string
- •Defining the Data Adapter
- •Creating and filling the DataSet
- •A Demonstration Application
- •Loading tables
- •Filling the controls on the Form
- •Navigating through the records
- •Updating the table
- •Building the Application
- •Loading the table and displaying data in the form's controls
- •Initialing Commands
- •Adding Parameters to the commands
- •The ToggleControls() method of our application
- •Editing (or Updating) Records
- •Event Handler for the Save Button
- •Event Handler for the Cancel Button
- •Inserting Records
- •Deleting a Record
- •Using Stored Procedures
- •Sample Stored Procedures
- •UPDATE Stored Procedure
- •INSERT Stored Procedure
- •DELETE Stored Procedure
- •SELECT Stored Procedure
- •Using Stored Procedures with ADO.Net in C#
- •The modified InitializeCommands() method
- •Using Data Grid Control to View .Net data
- •A Demonstration Application for Data Grid Control
- •Second Demonstration - Using multiple related tables
- •Retrieving data using the SELECT command
- •Updating Records using INSERT, UPDATE and DELETE commands
- •13. Multithreading
- •Lesson Plan
- •What is Multithreading
- •Multithreading in C#
- •Thread Functionality
- •Static members of the System.Threading.Thread class
- •Instance members of the System.Threaing.Thread class
- •Thread Demonstration Example - Basic Operations
- •Thread Demonstration Example - Thread Priority
- •Thread Demonstration Example - Thread Execution Control
- •Using Join() to wait for running threads
- •Thread Synchronization
- •The C# Locking Mechanism
- •Threads may cause Deadlock
- •14. The File System & Streams
- •Lesson Plan
- •Working with the File System
- •Obtaining the Application’s Environment Information – The System.Environment class
- •Demonstration Application – Environment Information
- •Obtaining the paths of various Windows Standard folders – Environment.GetFolderPath()
- •Manipulating Files using System.IO.File and System.IO.FileInfo classes
- •System.IO.File class
- •Creating a file using Create() method
- •Copying and Moving a file using Copy() and Move() methods
- •Checking the existence of the file using Exists() method
- •Getting Attributes of a file using GetAttributes() method
- •System.IO.FileInfo class
- •A quick and simple example
- •Manipulating Directories (folders) using System.IO.Directory and System.IO.DirectoryInfo classes
- •System.IO.Directory class
- •Creating, deleting and checking for the existence of directories
- •Getting the contents (files and sub-directories) of a directory
- •System.IO.DirectoryInfo class
- •Demonstration application for the DirectoryInfo class
- •Streams
- •An overview of the different types of streams
- •The System.Stream class – the base of all streams in the .Net framework
- •Different types of file streams – Reading and Writing to files
- •Using System.IO.FileStream to read and write data to files
- •A string representing the path and name of the file
- •Opening and reading from a file
- •Using BinaryReader and BinaryWriter to read and write primitives to files
- •Using StreamReader and StreamWriter to read and write text files
- •Serialization and De-serialization
- •Implementing Serialization and Deserialization – A simple example
- •Formatters in Serialization
- •Preventing certain elements from Serializing – The [NonSerialized] attribute
- •Getting notified when Deserializing - the IDeserializationCallBack interface
- •Asynchronous Reading and Writing with Streams
- •A demonstration application
- •Issues Regarding Asynchronous Read/Write
- •Important points regarding the use of Streams
- •15. New Features In C# 2.0
- •C# evolves
- •The need for generics
- •Generic collections
- •Creating generic types
- •Constraining type parameters
- •Final thoughts on generics
- •Partial types
- •Nullable types
- •Anonymous methods in event handling
- •Adventures with anonymous methods
- •Final thoughts on C# 2.0
- •16. The Road Ahead
- •Learning More
- •Getting Help
- •Book.revision++
- •Good Luck!
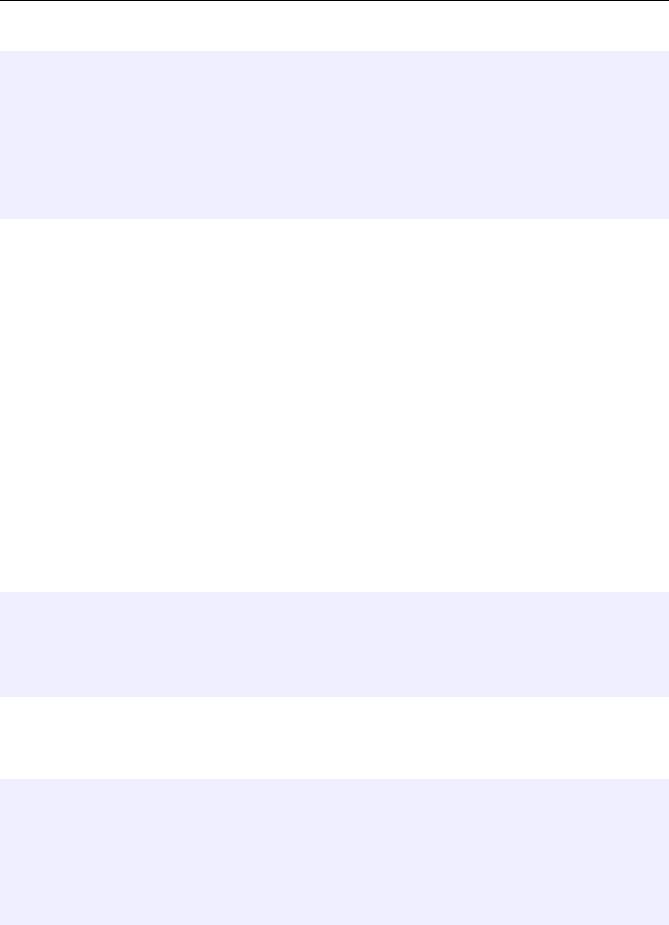
Programmers Heaven: C# School
private int age;
public Student(string name, int age)
{
this.name = name; this.age = age;
}
}
Here in the constructor when we use name or age, we actually get the variables passed in the method which overshadow the instance members (fields) with same name. Hence, to use our fields, we had to use this to distinguish our instance members (fields) with the members passed through the parameters.
This is an extremely useful, widely and commonly used construct. I recommend you practice with "this" for some time until you feel comfortable with it.
Static Members of the class
All the members of the classes that we have seen up till now are instance members, meaning they belong to the object being created. For example, if you have an instance field name in your Person class then each object of our Person class will have a separate field name of its own. There is another class of members which are called static. Static members belong to the whole class rather than to individual object. For example, if you have a static phoneNumber field in your Student class, then there will be the single instance of this field and all the objects of this class will share this single field. Changes made by one object to phoneNumber will be realized by the other object. Static members are defined using keyword static
class Student
{
public static int phoneNumber; public int rollNumber;
}
Static members are accessed with the name of class rather than reference to objects. Let’s make our Test class containing Main method
class Test
{
public static void Main()
{
Student st1 = new Student();
Student st2 = new Student();
st1.rollNumber = 3;
65
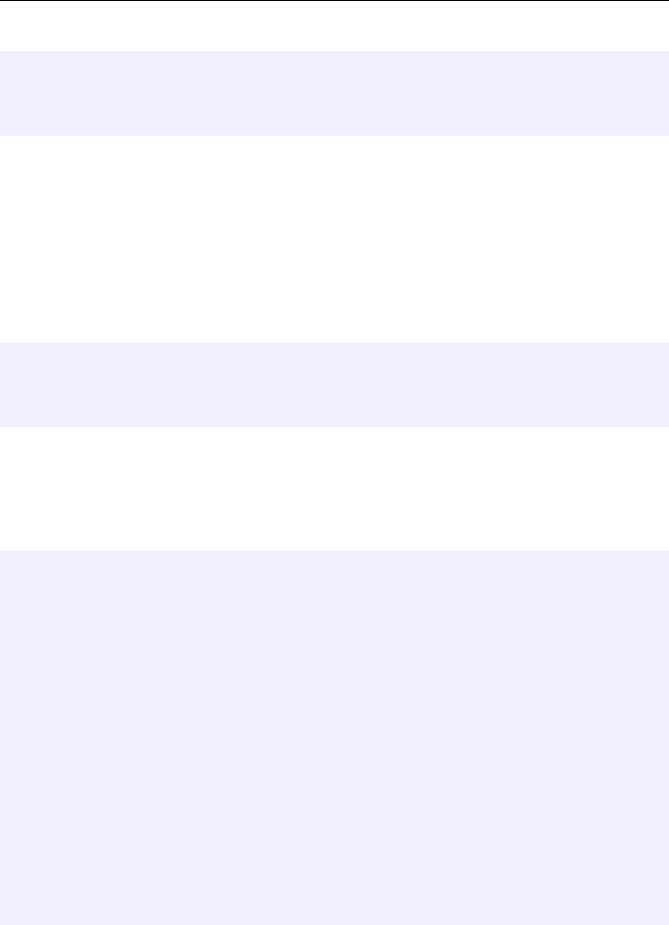
Programmers Heaven: C# School
st2.rollNumber = 5;
Student.phoneNumber = 4929067;
}
}
Here you can see that the phoneNumber is accessed without any reference to the object but with the name of the class it belongs. Static methods are very useful while programming. In fact, the WriteLine() and ReadLine() methods that we are using from the start are static methods of Console class. That is the reason why we used to call them with reference to their class rather than making an object of the Console class. I hope now you are able to understand the syntax of the Main method in C# in full. It is declared static as CLR calls it without making any instance of our class. Static variables are useful when you want to cache data that should be available to all objects of the class. You can use static fields, methods, properties and even constructors which will be called before any instance of the class is created. Static constructor are declared like
static Student()
{
name="unknown";
}
As static methods may be called without any reference to object, you can not use instance members inside static methods or properties, while you may call a static member from a non-static context. The reason for being able to call static members from non-static context is that static members belong to the class and are present irrespective of the existence of even a single object. The definition of MyMethod() in following code will not compile
class Student
{
public static int phoneNumber; public int rollNumber;
public void DoWork()
{
// legal, static method called in non-static context MyMethod();
}
public static void MyMethod()
{
//legal, static field used in static context phoneNumber++;
//illegal, non-static field used in static context rollNumber++;
//illegal, non-static method used in static context
66
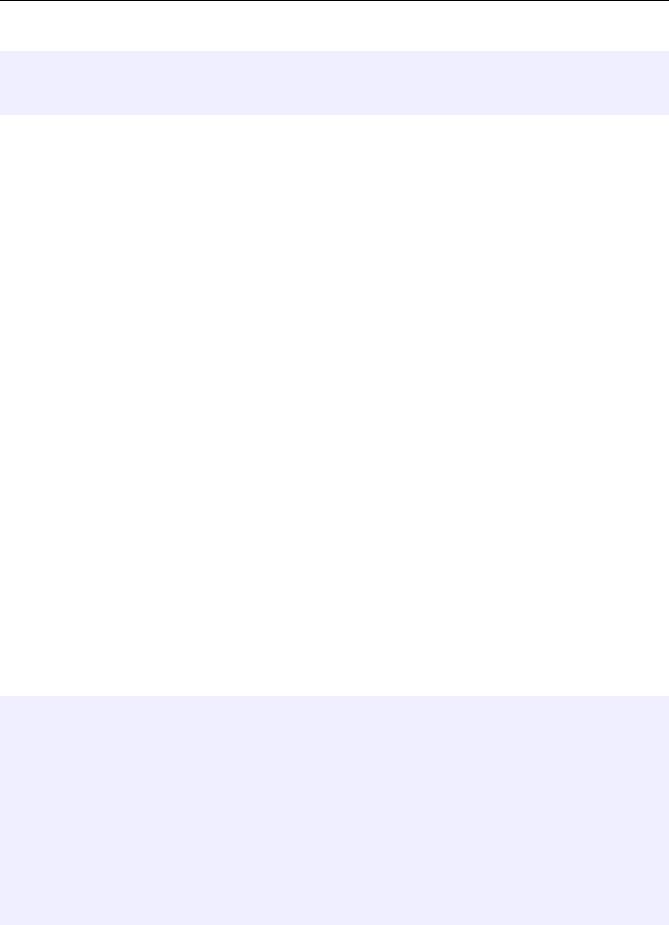
Programmers Heaven: C# School
DoWork();
}
}
Some precautionary points in the end
•Don't put too many static methods in your class as it is against the object oriented design principles and makes your class less extensible.
•Don't try to make a class with only the static methods and properties unless you have very good reason for doing this.
•You tend to loose a number of object oriented advantages while using static methods, as static methods can't be overridden which means it can not be used polymorphically, something widely used in the Object Oriented Paradigm of programming.
Some More about Methods
We mentioned earlier that there are two kinds of 'types' in C#: Value types and Reference types. Value types, such as implicit data types, are passed to methods by value. Reference types, like objects and arrays, are passed by reference.
Constructors
Constructors are a special kind of method. A Constructor has the following properties:
•It has the same name as its containing class
•It has no return type
•It is automatically called when a new instance or object of a class is created, hence why it's called a constructor.
•The constructor contains initialization code for each object, like assigning default values to the fields. Let us see some examples.
using System;
class Person
{
// field
private string name;
// constructor public Person()
{
name = "unknown";
67
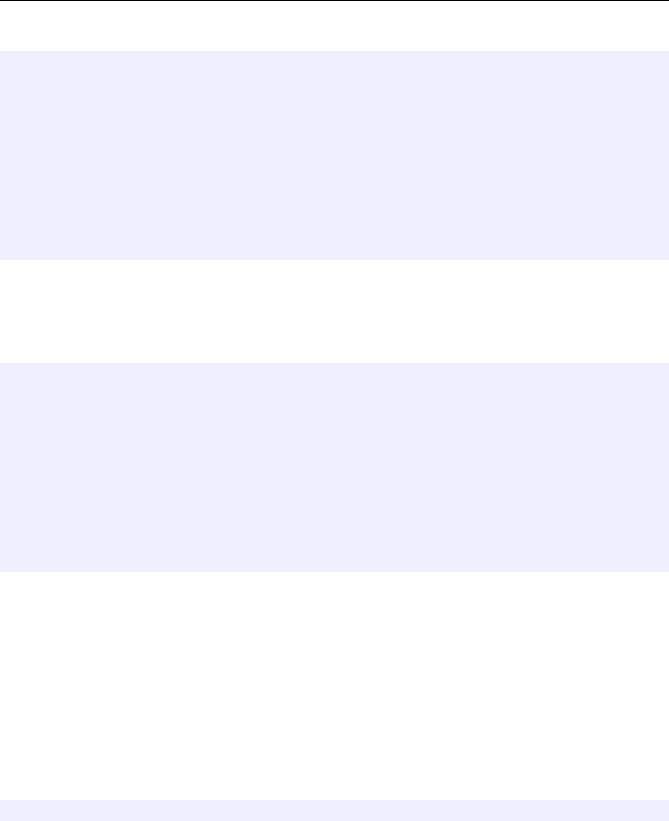
Programmers Heaven: C# School
Console.WriteLine("Constructor called...");
}
// property
public string Name
{
get { return name; } set { name = value; }
}
}
In the Person class above, we have a private field name, a public constructor which initializes the name field with string "unknown" and prints that it has been called, then we have a public property to read/write the private field name. Lets make another class Test which contains the Main() method and which uses the Person class
class Test
{
public static void Main()
{
Person thePerson = new Person();
Console.WriteLine("The name of person in object thePerson is " + thePerson.Name); thePerson.Name = "Faraz";
Console.WriteLine("The name of person in object thePerson is " + thePerson.Name);
}
}
In our Test class, we made an object of the Person class and printed the name of person. We then changed the value of Name and printed the Name again. The result of the program is:
Constructor |
called... |
|
|
|
|
|||
The |
name |
of |
person |
in object |
thePerson |
is |
unknown |
|
The |
name |
of |
person |
in |
object |
thePerson |
is |
Faraz |
|
|
|
|
|
|
|
|
|
Note that the constructor is called just as we created a new instance of Person class and initialized the field name with string "unknown". In fact, when we create a new object, we actually call the constructor of the class:
Person thePerson = new Person();
That is why constructor is usually made public. If you make your constructor private, no one would be able to make an object of your class outside of it (though a method in the class of course could). That is, if the Person class is defined as:
68
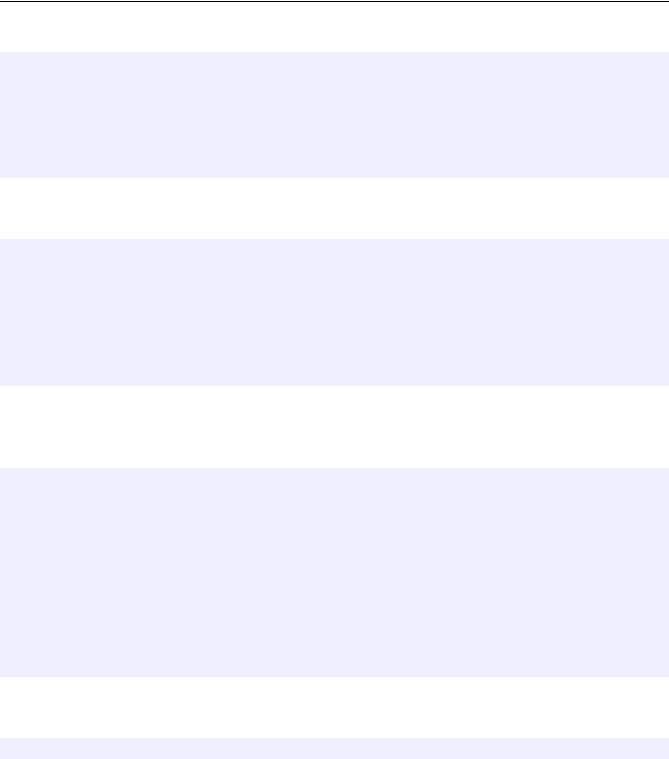
Programmers Heaven: C# School
class Person
{
private Person()
{
}
}
then it would cause an error to write:
class Test
{
public static void Main()
{
Person thePerson = new Person(); // error
}
}
The constructors shown so far have been parameter-less, i.e. they do not take any parameters. We can define constructors which take some parameters.
class Person
{
private string name;
public Person(string theName)
{
name = theName; Console.WriteLine("Constructor called...");
}
}
Now, the object of class Person can only be created by passing a string into the constructor.
Person thePerson = new Person("Faraz");
If you don't define any constructor for your class, the compiler will generate an empty parameter-less constructor for you. That is why we were able to make our Student object even we did not specify any constructor for the Student class.
69