
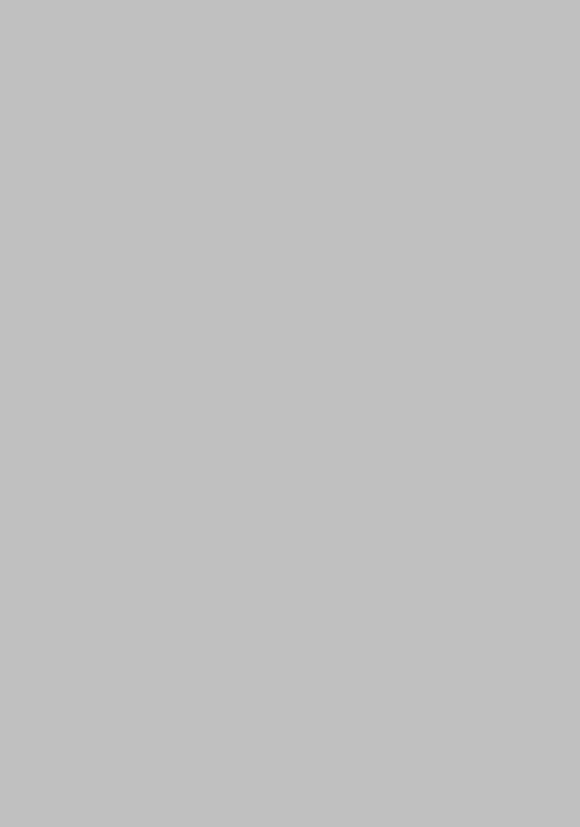
.NET Book Zero |
Charles Petzold |
class Date
{
//Private fields int year = 1;
int month = 1; int day = 1;
//Public properties public int Year
{
set
{
if (!IsConsistent(value, Month, Day))
throw new ArgumentOutOfRangeException("Year");
year = value;
}
get
{
return year;
}
}
public int Month
{
set
{
if (!IsConsistent(Year, value, Day))
throw new ArgumentOutOfRangeException("Month = " + value);
month = value;
}
get
{
return month;
}
}
public int Day
{
set
{
if (!IsConsistent(Year, Month, value))
throw new ArgumentOutOfRangeException("Day");
day = value;
}
get
{
return day;
}
}
Version 1.1 |
Page 160 |
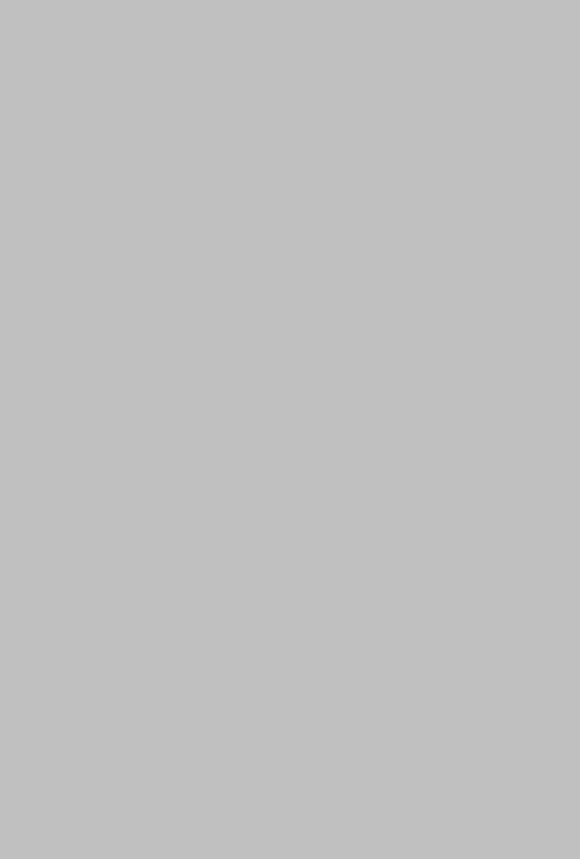
.NET Book Zero |
Charles Petzold |
//Parameterless constructor public Date()
{
}
//Parametered constructor
public Date(int year, int month, int day)
{
Year = year; Month = month; Day = day;
}
// Private method used by the properties
static |
bool IsConsistent(int year, int month, int day) |
||
{ |
|
|
|
if |
(year < |
1) |
|
|
return |
false; |
|
if |
(month < 1 || month > 12) |
||
|
return false; |
|
|
if |
(day < 1 || day > 31) |
||
|
return false; |
|
|
if |
(day == 31 && (month == 4 || month == 6 || |
||
|
|
|
month == 9 || month == 11)) |
|
return false; |
|
|
if |
(month == 2 && |
day > 29) |
|
|
return false; |
|
|
if |
(month == 2 && |
day == 29 && !IsLeapYear(year)) |
|
|
return false; |
|
|
return true; |
|
||
} |
|
|
|
// Public properties |
|
||
public |
static bool IsLeapYear(int year) |
{
return year % 4 == 0 && (year % 100 != 0 || year % 400 == 0);
}
static int[] daysCumulative = { 0, 31, 59, 90, 120, 151,
181, 212, 243, 273, 304, 334 };
public int DayOfYear()
{
return daysCumulative[Month - 1] + Day + (Month > 2 && IsLeapYear(Year) ? 1 : 0);
}
static string[] strMonths = { "Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"};
Version 1.1 |
Page 161 |
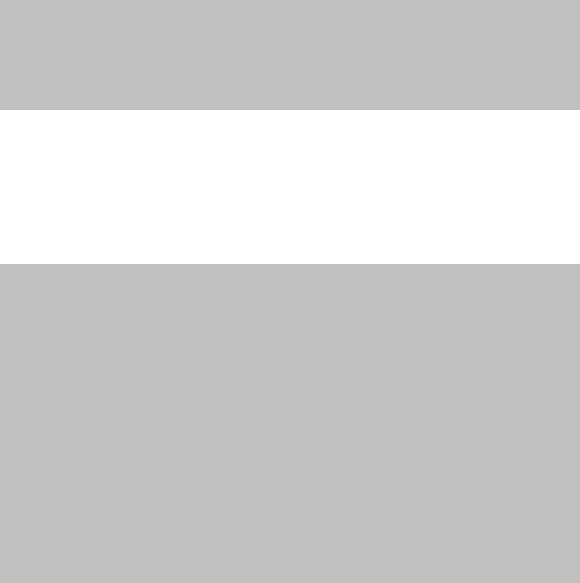
.NET Book Zero |
Charles Petzold |
public override string ToString()
{
return String.Format("{0} {1} {2}", Day, strMonths[Month - 1], Year);
}
}
Notice that the three-parameter constructor sets the properties rather than the fields. Even the set accessors of the properties refer to the other properties rather than access the fields. This is not a requirement.
Methods in a class can use either the class properties or the fields themselves. But you‘ll see shortly why I like to structure my classes so that field accesses are kept to a minimum.
Here‘s a simple program to test out the Date class:
PropertyTest.cs
//---------------------------------------------
// PropertyTest.cs (c) 2006 by Charles Petzold //---------------------------------------------
using System;
class PropertyTest
{
static void Main()
{
Date dateMoonWalk = new Date();
dateMoonWalk.Year = 1969; dateMoonWalk.Month = 7; dateMoonWalk.Day = 20;
Console.WriteLine("Moon walk: {0}, Day of Year: {1}", dateMoonWalk, dateMoonWalk.DayOfYear());
}
}
Both Date.cs and PropertyTest.cs are part of the PropertyTest project.
You can experiment with other dates to make sure the class is working as it should. Of course, in a real program, any code that has the potential of causing Date to raise an exception should be put in a try block.
Sometimes it‘s not clear whether a particular chunk of code should be a method or a property. Properties are often considered characteristics of an object, whereas methods often perform actions. If the object itself is a noun, a property is an adjective and a method is a verb. If you can associate the words get and set with a method, you probably want to make it a property. I‘d be inclined to make DayOfYear a read-only property, for example. The only real rule is this: If it needs a parameter to get the value or an extra parameter to set the value, it‘s got to be a method.
Version 1.1 |
Page 162 |
.NET Book Zero |
Charles Petzold |
When we first embarked on the idea of encapsulating a date, we explored implementing it as a class or a structure. It soon became clear that using a structure had distinct problems. When an object is created from a structure using a parameterless constructor, there is no way to initialize the fields to anything but 0 or null.
Let‘s ask ourselves the question again: How can we implement Date as a structure and prevent an invalid date when the parameterless constructor is used to create the object?
Big hint: The get accessor of a property doesn‘t necessarily have to return simply the value of a field.
The get accessor could return the value of the field plus one. In other words, the private year, month, and day fields could be zero-based, but the public Year, Month, and Day properties could be one-based. Here‘s a revised Year property that uses this technique:
public int Year
{
set
{
if (!IsConsistent(value, Month, Day))
throw new ArgumentOutOfRangeException("Year");
year = value - 1;
}
get
{
return year + 1;
}
}
The only difference is that the set accessor sets the year field to value minus one, and the get accessor returns the year field plus one. The default year field is 0 but the Year property returns 1. Properties provide a type of buffer around fields so you can make the internals of a class or structure different from how it looks from outside.
Suppose you wanted to make the days of the month available as a public property. You could define a public static property of type string array and simply return the entire array of month names:
public static string[] MonthNames
{
get
{
return strMonths;
}
}
Then, if a program wanted the abbreviated name for January, for example, the expression would be Date.MonthNames[0]. It seems as if the property returns the whole array, but it‘s really only returning a
Version 1.1 |
Page 163 |
.NET Book Zero |
Charles Petzold |
reference to the array, which is then indexed by the code accessing the property. (The DateTimeFormatInfo class in the System.Globalization namespace has a MonthNames property that returns a string array but the month names are specific to a particular culture and language.)
It‘s also possible for a class to have a special member called an indexer that is declared somewhat like a property. An indexer is intended for classes or structure that store collections of items, so it‘s not quite appropriate for the Date class.
But just suppose that you have a particular application that for a particular Date object named dt, for example, it is sometimes convenient to refer to the property dt.Year as dt[0], and refer to the property dt.Month as dt[1], and the property dt.Day as dt[2]. You actually want to index the object as if it were an array.
Here‘s how such an indexer would look:
public int this[int i]
{
get
{
switch (i)
{
case 0: return Year; case 1: return Month; case 2: return Day;
default: throw ArgumentOutOfRangeException("index = " + i);
}
}
}
Notice that the declaration of the indexer begins by resembling a property named this, which is then followed by square brackets containing the value of the indexer. As implemented here, this is a read-only indexer, but you could come up with set logic as well if you wanted.
You can have multiple indexers if the index is a different type. It doesn‘t have to be an integer. This one lets you specify a text index:
public int this[string str]
{
get
{
switch (str.ToLower())
{
case "year": return Year; case "month": return Month; case "day": return Day;
default: throw ArgumentOutOfRangeException("index = " + i);
}
}
}
Version 1.1 |
Page 164 |
.NET Book Zero |
Charles Petzold |
With an indexer like this, if dt is an object of type Date, then dt["MONTH"] obtains the month.
In the .NET documentation, indexers often show up as the property name Item. (In the String class, the name is Chars.) When programming in C#, you never use that name to refer to indexers. You simply index the object. As with properties, C# fabricates indexers by creating methods named get_Item and set_Item.
Version 1.1 |
Page 165 |
.NET Book Zero |
Charles Petzold |
Chapter 18. Inheritance
No class is an island. All classes are related to each other in some way. All classes automatically have methods named ToString and Equals because these two methods are defined in the class System.Object, a class also referred to by the C# keyword object. All other classes contain the ToString and Equals methods because all classes ultimately derive (or inherit) from System.Object.
Inheritance is one of the primary features of object-oriented programming. When a new class derives from an existing class (or subclasses an existing class), it inherits all the non-private methods, properties, and fields declared in the existing class. The new class can then extend the existing class by adding to or replacing those methods, properties, and fields. The process is cumulative. A class contains all the non-private methods, properties, and fields declared in itself and all its ancestor classes going back to System.Object.
It‘s all about reusing code. Inheritance provides a structured way to reuse code that‘s already been written, but inheritance also provides a way to alter or enhance the code in ways that make it more useful or convenient.
The ability to inherit is one of the big differences between classes and structures. Structures do not allow inheritance. All structures implicitly derive from System.ValueType, which itself derives from System.Object.
But you can‘t define a class that inherits from a structure, or define a structure that inherits from another structure. Once you define a structure, that‘s the end of the line.
There are many reasons why you‘d want to use inheritance. Suppose you have a class that‘s fine for most of your requirements, but it needs just a few little changes. Even if you have access to the source code, you might not want to change the original class. Maybe the class is working well in some other application, and you wisely respect the ―if it ain‘t broke don‘t fix it‖ rule. Or perhaps you don‘t have access to the code. Maybe the class is available only in compiled form in a dynamic link library.
(Another reason to use inheritance that may seem a bit lame is this:
Suppose you‘re writing a book about object-oriented programming. In the previous chapter you‘ve just presented a complete class named Date, and now you‘d like to add a few features to it. Why make the previous class even longer than it is if you can just derive from it and show the new features that way?)
Version 1.1 |
Page 166 |
.NET Book Zero |
Charles Petzold |
But mostly inheritance is used as an architectural mechanism that allows programmers to avoid duplicating code. Often a programmer will see that a particular job requires several classes. With further work and design, it appears that two of these classes might be able share about 75 percent of their code. It makes sense for one of these classes to inherit from the other, perhaps replacing a method or two, perhaps adding a method or two, whatever‘s required. Object-oriented design is an art (and a science) in itself, and this book will only scratch the surface.
With any luck, programmers who have come before you have used their skills to create object-oriented libraries that exhibit an intelligent structure of inheritance. If you‘ll be writing Windows applications using the Windows Presentation Foundation, you‘ll be working a lot with controls, which are visual user interface objects such as buttons, scrollbars, and so forth. These controls are declared in a vast hierarchy of inheritance, just a little bit of which is shown here:
Control ContentControl
Label ButtonBase
Button RepeatButton ToggleButton
CheckBox RadioButton
RangeBase ScrollBar ProgressBar Slider
This format is a standard way of showing inheritance. Each additional level of indentation shows another level of inheritance. For example,
ContentControl derives from Control, and both Label and ButtonBase derive from ContentControl. (Not shown is the fact that Control derives from FrameworkElement, which derives from UIElement, which derives from Visual, which derives from DependencyObject, which derives from
DispatcherObject, which derives from Object.)
Typically, as classes derive from other classes, they get less generalized and more specific. The Windows Presentation Foundation lets you subclass from the existing controls to make them even more specific—for example, a button that always has red italic text.
In this chapter I‘ll be creating a class named ExtendedDate that derives from the final Date class shown in the previous chapter.
The simplest subclassing syntax is:
Version 1.1 |
Page 167 |
.NET Book Zero |
Charles Petzold |
class ExtendedDate: Date
{
}
The name of the ExtendedDate class is followed by a colon and then the name of the class that it‘s subclassing, in this case Date. Date is known as the base class to ExtendedDate. Many programmers put a space before the colon but it‘s not required. If you don‘t indicate a base class when you‘re declaring a class, then the class is assumed to derive from object. A class can inherit from only one other class. Multiple inheri- tance—a feature of some object-oriented languages in which a class can inherit from multiple classes—is not supported by C#.
With the simple declaration of ExtendedDate, a new class has been defined that contains all the non-private methods, properties, and fields in Date.
What ExtendedDate does not inherit from Date are the constructors.
Constructors are not inherited. I repeat: Constructors are not inherited.
Because the simple ExtendedDate class shown above does not inherit any of the constructors in Date, C# automatically provides a parameterless constructor. This constructor allows you to create an ExtendedDate object like so:
ExtendedDate exdt = new ExtendedDate();
You can then use the Year, Month, and Day properties that ExtendedDate inherits from Date. But you cannot use the threeparameter constructor declared in Date to create an ExtendedDate object.
If you‘d like to provide a three-parameter constructor in ExtendedDate, you must explicitly declare it. Once you do that, then C# no longer automatically provides a parameterless constructor, so you‘ll probably want to provide one was well. Here‘s a version of ExtendedDate that has the same constructors as Date:
class ExtendedDate: Date
{
public ExtendedDate()
{
}
public ExtendedDate(int year, int month, int day)
{
Year = year; Month = month; Day = day;
}
}
Notice that the three-parameter constructor refers to the three properties defined by Date. That‘s fine because ExtendedDate has inherited those
Version 1.1 |
Page 168 |
.NET Book Zero |
Charles Petzold |
properties. But a better way to declare the three-parameter constructor in ExtendedDate is for it to make use of the three-paremeter constructor in Date. This requires a special syntax:
class ExtendedDate: Date
{
public ExtendedDate()
{
}
public ExtendedDate(int year, int month, int day) : base(year, month, day)
{
}
}
Notice the colon following the parameter list. This colon is followed by the keyword base, which refers to the base class of Date. The base keyword is followed by three arguments in parentheses, so it refers to the threeparameter constructor in Date. When the three-parameter ExtendedDate constructor executes, the three-parameter constructor in Date is called first, and then execution continues with the code in the body of the ExtendedDate constructor (if any).
I showed you a feature similar to this in Chapter 15 but using the keyword this rather than base. Collectively, these constructor calls are called constructor initializers. A constructor initializer causes another constructor in the same class or the base class to be executed before the code in the body of the constructor.
Only one constructor initializer is allowed per constructor. If you don‘t provide one, then one is provided for you that calls the parameterless constructor in the base class. In other words, you‘ll never need to provide a constructor initializer of base(). That initializer is generated automatically if you don‘t provide an alternative.
Imagine a series of classes: Instrument derives from object, and
Woodwind derives from Instrument and Oboe derives from Woodwind. All these classes have parameterless constructors with no explicit constructor initializers. When you create an object of type Oboe, the constructor in Oboe first executes the parameterless constructor in
Woodwind, which first executes the parameterless constructor in
Instrument, which first executes the parameterless constructor in object, which doesn‘t actually do anything. The final result in this chain of nested constructor calls is that all the parameterless constructors are executed beginning with object, then Instrument, then Woodwind, and finally Oboe. This happens even if you‘re using a constructor in Oboe with parameters. If that constructor has no explicit constructor initializer, the parameterless constructors in object, Instrument, and
Woodwind are executed before the constructor code in Oboe.
Version 1.1 |
Page 169 |