
Practical Database Programming With Java
.pdf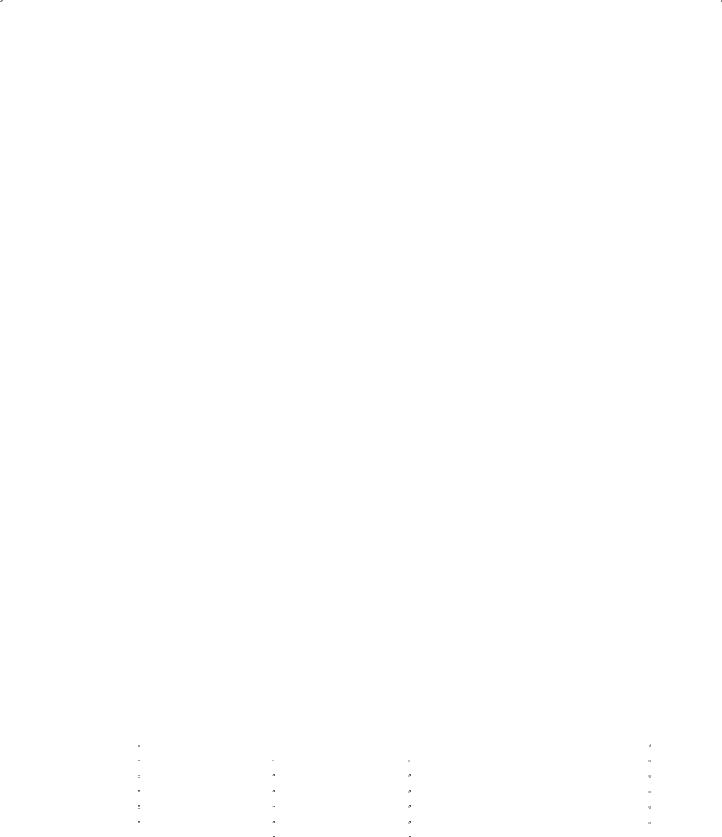
848 Chapter 9 Developing Java Web Services to Access Databases
or methods in our Web service project to perform related Course information query actions.
9.14.6 The Organization of Web Service Operations and Session Bean Methods
The main purpose of using our Web service is to query and manipulate data from the Course table in our sample database. Therefore, we need to add some new operations to the Web service project. We will add five new operations based on the sequence of five operational tasks listed in section 9.14.3. This means that we will add the following five operations into this Web service project to perform related Course information query and manipulations:
•QueryCourseID(): Query all course_id taught by the selected faculty member.
•QueryCourse(): Query detailed information for selected course_id.
•InsertCourse(): Insert a new course record into the Course table.
•UpdateCourse(): Update an existing course record in the Course table.
•DeleteCourse(): Delete a course record from the Course table.
Generally, each operation listed above needs an associated method defined in our session bean to perform the actual database-related actions. Table 9.8 shows this one-to- one relationship between each operation in our Web service and each method in our session bean.
Based on this table, we will divide the coding process into two parts:
•Coding for the Web service operations.
•Coding for the associated methods defined in the Java session bean classes.
The relationship between each operation in our Web service and each user-defined method in our session bean is one-to-one. The function of each operation in our Web service is to: (1) call the associated method in our session bean class to perform the actual data query and actions against our sample Oracle database, (2) collect and process the queried data and send back to the client projects that will be used to consume the Web service and will be developed later. The function of each method defined in our session bean is to handle real database-related operation and business-related logics via Java persistence and entity classes.
Table 9.8. The relationship between each operation and each method
Web Service Operation |
Session Bean Method |
Function |
|
QueryCourseID() |
getCourseID() |
Query all course_id taught by the selected faculty |
|
QueryCourse() |
getCourse() |
Query detailed information for selected course_id |
|
InsertCourse() |
newCourse() |
Insert a new course record into the Course table |
|
UpdateCourse() |
setCourse() |
Update an existing course record in the Course table |
|
DeleteCourse() |
removeCourse() |
Delete a course record from the Course table |

9.14 Build Java Web Service Projects to Access Oracle Databases 849
Because each actual database-related action is performed inside each associated session bean method.Therefore, in this project, in order to provide readers with an understandable and sequential coding process, we will develop and build our data actions in the following ways:
1.Create each session bean method and develop the codes for that method to perform actual course data operations.
2.Create each Web service operation and develop the codes to call the associated session bean method to query and manipulate data.
3.Deploy and test each Web service operation to confirm its function.
4.After all Web service operations have been developed and deployed, some client projects will be built to consume our whole Web service project.
Before we can do the coding for any session bean method and Web service operation, we need first to add or inject the related session bean class, CourseFacade.java, into our Web service project to enable each operation to recognize this session bean and to call related method that is defined inside this session bean and will be developed later to perform desired data query or actions.
9.14.7 Add the Session Bean Classes CourseFacade into Our Web Service
Perform the following operations to add our Java session bean class CourseFacade.java into our Web service project:
1.Click on the Source button on the top of this window to open the code window of our Web service project.
2.Right click on any place inside our Web service class and choose Insert Code item, then select Call Enterprise Bean item.
3.Expand our Web service application WebServiceOracleApp, and choose our session bean class CourseFacade. Click on the OK button to complete this process.
Immediately, you can find that the session bean CourseFacade has been injected into our Web service project with the following two statements:
@EJB private CourseFacade courseFacade;
Now we are ready to create and build each session bean method and the associated Web service operation to perform the desired data query and data action.
9.14.8 Create and Build the Session Bean Methods and Web Service Operations
Let’s start to create each session bean method and develop the codes for each of them. First, let’s start from the getCourseID() method.
Recall that when we built our sample database in Chapter 2, especially when we built the Course table, there is no faculty_name column available in the Course table, and the

850 Chapter 9 Developing Java Web Services to Access Databases
only relationship between each course_id and each faculty member is the faculty_id column in the Course table. This is a many-to-one relationship between the course_id and the faculty_id in this table, which means that many courses (course_id) can be taught by a single faculty (faculty_id). However, in the Faculty table, there is a one-to-one relationship between each faculty_name and each faculty_id column.
Therefore, in order to query all courses, exactly all course_id, taught by the selected faculty member, exactly the faculty_name, we need to perform two queries from two tables.
•First, we need to perform a query to the Faculty table to get a matched faculty_id based on the selected faculty member (faculty_name).
•Then we need to perform another query to the Course table to get all course_id taught by the selected faculty_id that is obtained from the first query.
Based on this discussion, now let’s perform the following operations to add a new method getCourseID() into our session bean CourseFacade.java to perform this course_id query:
9.14.8.1 Create and Build Session Bean Method getCourseID()
Perform the following operations to create a new method getCourseID() in our session bean class CourseFacade.java:
1.Open our Web service application project WebServiceOracleApp and double click on our session bean class CourseFacade.java from the Projects window to open it.
2.Right click on any place inside our session bean class body, then choose the Insert Code item and select the Add Business Method item to open the Add Business Method wizard.
3.Enter getCourseID into the Name field and click on the Browse button that is next to the
Return Type combo box. On the opened Find Type wizard, type list into the top field and select the List (java.util) from the list and click on the OK button.
4.Click on the Add button to add one argument for this method. Enter fname into the Name column and keep the default data type java.lang.String unchanged. Your finished Add
Business Method wizard should match the one that is shown in Figure 9.80. Click on the OK button to complete this business method creation process.
Now let’s develop the codes for this method.
Click on the Source button on the top of this window to open the code window of our session bean class CourseFacade.java. Enter the codes that are shown in Figure 9.81 into this code window and the new method getCourseID().
Let’s have a closer look at this piece of codes to see how it works.
A.First, an MsgDialog instance msgDlg is created since we need to use this object to track and display some debugging information as we test our Web service later.
B.A List instance courseList is created and initialized, and we need to use it to hold the query result, which are all course_id taught by the selected faculty member.
C.Two queries should be performed to get all course_id taught by the selected faculty member, (1) query to the Faculty table to get a matched faculty_id based on the selected faculty name, and (2) query to the Course table to get all course_id based on the faculty_
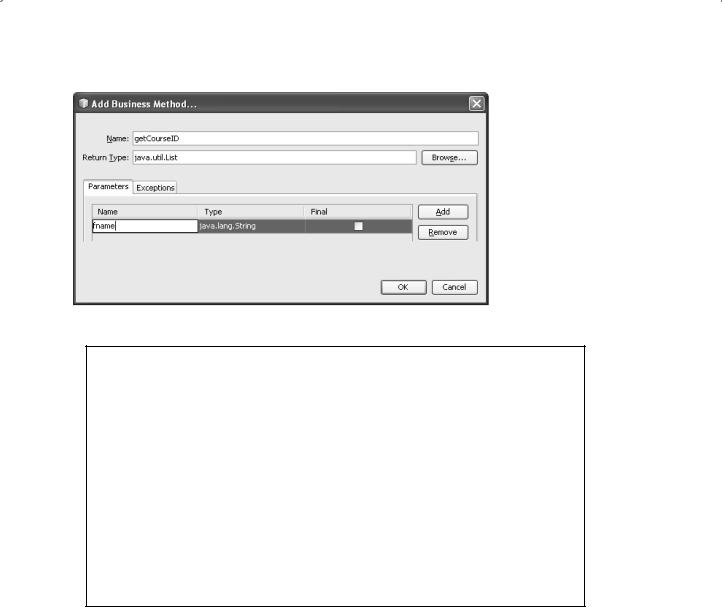
9.14 Build Java Web Service Projects to Access Oracle Databases 851
Figure 9.80. The finished Add Business Method wizard.
@Stateless
public class CourseFacade {
@PersistenceContext(unitName = "WebServiceOracleAppPU") private EntityManager em;
AMsgDialog msgDlg = new MsgDialog(new javax.swing.JFrame(), true);
………
public List getCourseID(String fname) {
BList courseList = null;
CString f_query = "SELECT f.facultyId FROM Faculty f WHERE f.facultyName = :FacultyName";
DQuery fQuery = em.createQuery(f_query);
EfQuery.setParameter("FacultyName", fname);
FString fid = fQuery.getSingleResult().toString();
GString c_query = "SELECT c.courseId FROM Course c WHERE c.facultyId = :FacultyID";
HQuery cQuery = em.createQuery(c_query);
IFaculty f = new Faculty(fid);
JcQuery.setParameter("FacultyID", f);
KcourseList = cQuery.getResultList();
Lreturn courseList;
}
}
Figure 9.81. The codes for the method getCourseID().
id obtained from the first query. The query statement of the first query is created at this step.
D.The createQuery() method is executed to create the first query object fQuery based on the query statement created in step C.
E.Since the query statement in the first query contains a named dynamic parameter FacultyName, the setParameter() method is executed to set up this parameter with an actual value fname, which is the input argument of this method.
F.Then the getSingleResult() method is called to perform the first query to get a matched faculty_id and return it to a local variable fid.
G.The second query statement is created with a named dynamic parameter FacultyID.
H.The createQuery() method is executed to create the second query object cQuery based on the query statement created in step G.

852Chapter 9 Developing Java Web Services to Access Databases
I.A tricking issue arises in the next step. As we know, the data type for the second argument in the setParameter() method should be an Object. However, the data type for the queried faculty_id, or fid, from the first query is a String. In order to enable the setParameter() method to be executed correctly, we must create a new Faculty object with the queried faculty_id as the argument in this step.
J.Then the setParameter() method is executed to set up this correct faculty_id that is involved in the newly created Faculty object in step I.
K.The getResultList() method is executed to perform the second query and return the query result to the local variable courseList we created in step B.
L.Finally, the query result is returned to the calling method or operation.
During the coding process, you may encounter some real-time compiling errors. Most of these errors are introduced by missing some packages that contain classes or components used in this file. To fix these errors, just right click on this code window and select the Fix Imports item to load and import those missed packages to the top of this code window.
To save this piece of codes, click on the Clean and Build Main Project button on the top to build our project.
Next let’s create and build our first Web service operation QueryCourseID() to call this session bean method getCourseID() to query all course_id taught by the selected faculty.
9.14.8.2 Create and Build Web Service Operation QueryCourseID()
Now let’s begin the coding process for operations in our Web service project. First let’s create the first operation in our Web service to query all course_id from the Course table.
Perform the following operations to add a new operation QueryCourseID() into our Web service project to perform this course_id query:
1.Double click on our Web service project WebServiceOracle.java from the Projects window to open it.
2.Click on the Design button on the top of the window to open the Design View of our Web service project WebServiceOracle.
3.Click on the Add Operation button to open the Add Operation wizard.
4.Enter QueryCourseID into the Name field and click on the Browse button that is next to
the Return Type combo box. Type arraylist into the Type Name field and select the item ArrayList (java.util) from the list, and click on the OK button.
5.Click on the Add button and enter fname into the Name parameter field. Keep the default type java.lang.String unchanged and click on the OK button to complete this new operation creation process.
Your finished Add Operation wizard should match the one that is shown in Figure 9.82.
Click on the Source button on the top of this window to open the code window of our Web service project. Let’s perform the coding for this newly added operation.
On the opened code window, enter the codes that are shown in Figure 9.83 into this code window and this newly added operation.
Let’s have a closer look at this piece of codes to see how it works.
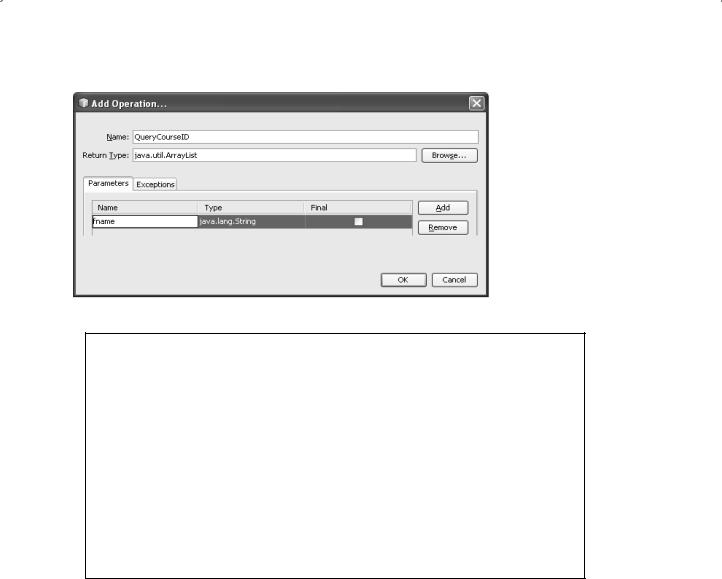
9.14 Build Java Web Service Projects to Access Oracle Databases 853
Figure 9.82. The finished Add Operation wizard.
@WebService()
public class WebServiceOracle { @EJB
private CourseFacade courseFacade;
AMsgDialog msgDlg = new MsgDialog(new javax.swing.JFrame(), true);
@WebMethod(operationName = "QueryCourseID")
public ArrayList QueryCourseID(@WebParam(name = "fname") String fname) {
//TODO write your implementation code here:
BArrayList<String> al = new ArrayList<String>(); List courseList = null;
CcourseList = courseFacade.getCourseID(fname);
Dfor (int col = 0; col < courseList.size(); col++) { al.add(courseList.get(col).toString());
}
E return al;
}
}
Figure 9.83. The codes for the Web service operation QueryCourseID().
A.First, a class-level variable msgDlg is created. This variable is used to track and display the debug information when this Web service project is tested later.
B.An ArrayList instance al and a List instance courseList are created. The first variable is an array list instance used to collect and store our query result, and return to the consuming project. The second variable is used to hold and store the query result from the execution of the session bean method getCourseID().
C.The session bean method getCourseID() is called to query all course_id taught by the selected faculty member fname that works as an argument of that method. The query result is returned and assigned to the local variable courseList.
D.A for loop is used to pick up each queried course_id and add it into the ArrayList instance al. The reason we used an ArrayList, not a List instance, as the returned object is that the former is a concrete class but the latter is an abstract class, and a runtime exception may be encountered if an abstract class is used as a returned object to the calling method.
E.The queried result is returned to the consuming project.
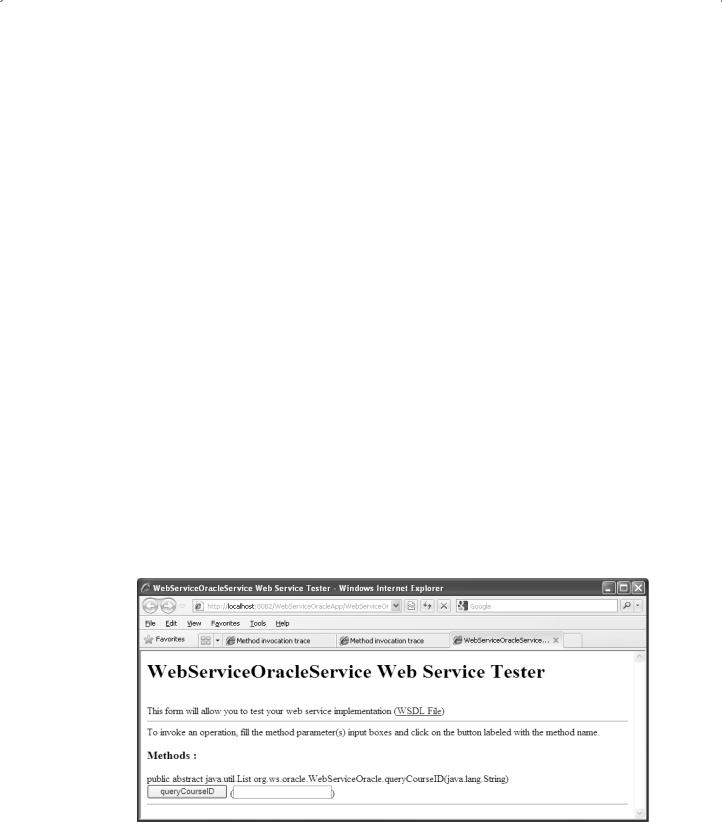
854 Chapter 9 Developing Java Web Services to Access Databases
During the coding process, you may encounter some in-time compiling errors. The main reason for those errors is that some packages are missed. To fix these errors, just right click on any space inside this code window, and select the Fix Imports item to find and add those missed packages.
At this point, we have finished all coding process for the course_id query. Now let’s build and test our Web service to test this course_id query function.
9.14.8.3 Build and Run the Web Service to Test the course_id Query Function
Click on the Clean and Build Main Project button on the top of the window to build our Web service project. Then right click on our Web service application project
WebServiceOracleApp and choose the Deploy item to deploy our Web service. Enter the appropriate username and password to the Glassfish v3 server, such as
admin and reback, which are used for this application, and click on the OK button to start the application server.
If everything is fine, expand the Web Services node under our Web service project and right click on our Web service target file WebServiceOracle, and choose the Test Web Service item to run our Web service project. The running status of our Web service is shown in Figure 9.84.
Enter a desired faculty name, such as Jenney King, into the text field, and click on the queryCourseID button to test this query function. The testing result is shown in Figure 9.85.
It can be found from Figure 9.85 that all course_id taught by the selected faculty member Jenney King have been retrieved and displayed at the bottom of this page, and our course_id query via Web service is successful!
Next, let’s handle creating and coding process for the second session bean method getCourse() and Web service operation QueryCourse() to query details for a selected course_id.
Figure 9.84. The running status of our Web service operation QueryCourseID().

9.14 Build Java Web Service Projects to Access Oracle Databases 855
Figure 9.85. The running result of our Web service operation QueryCourseID().
9.14.8.4 Create and Build Session Bean Method getCourse()
Perform the following operations to create a new method getCourse() in our session bean class CourseFacade.java:
1.Open our Web service application project WebServiceOracleApp and double click on our session bean class CourseFacade.java from the Projects window to open it.
2.Right click on any place inside our session bean class body, choose the Insert Code item, and select the Add Business Method item to open the Add Business Method wizard.
3.Enter getCourse into the Name field and click on the Browse button that is next to the Return Type combo box. On the opened Find Type wizard, type course into the top field and select the Course (org.ws.entity) from the list and click on the OK button.
4.Click on the Add button to add one argument for this method. Enter cid into the Name column and keep the default data type java.lang.String unchanged. Your finished Add
Business Method wizard should match the one that is shown in Figure 9.86. Click on the OK button to complete this business method creation process.
Now let’s develop the codes for this method.
Click on the Source button on the top of this window to open the code window of our session bean class CourseFacade.java. Enter the codes that are shown in Figure 9.87 into this new method getCourse(). The newly added codes have been highlighted in bold.
The codes for this method is very simple since we utilized a built-in method find() that is created and added into this session bean class automatically when this session bean is created. Let’s have a closer look at this piece of codes to see how it works.

856 Chapter 9 Developing Java Web Services to Access Databases
Figure 9.86. The finished Add Business Method wizard.
@Stateless
public class CourseFacade {
@PersistenceContext(unitName = "WebServiceOracleAppPU") private EntityManager em;
MsgDialog msgDlg = new MsgDialog(new javax.swing.JFrame(), true);
………
public Course find(Object id) { return em.find(Course.class, id);
}
public Course getCourse(String cid) {
ACourse result = find(cid);
Breturn result;
}
}
Figure 9.87. The codes for the method getCourse().
A.The built-in method find() is called with the given course_id as the argument. The function of the find() method is to try to find a course record whose primary key is the given course_id. The query result is returned and assigned to a local Course object result.
B.The query result is returned to the associated Web operation.
The point to be noted is that the returned object of this method is a Course instance, whose protocol is the entity class Course defined in our entity class Course.java.
During the coding process, you may encounter some real-time compiling errors. Most of these errors are introduced by missing some packages that contain classes or components used in this file. To fix these errors, just right click on this code window and select the Fix Imports item to load and import those missed packages to the top of this code window.
To save this piece of codes, click on the Clean and Build Main Project button on the top to build our project.
Next, let’s create and build our second Web service operation QueryCourse() to call this session bean method getCourse() to query the details for a given course_id.

9.14 Build Java Web Service Projects to Access Oracle Databases 857
9.14.8.5 Create and Build Web Service Operation QueryCourse()
Perform the following operations to add a new operation QueryCourse() into our Web service project to perform this course details query:
1.Double click on our Web service project WebServiceOracle.java from the Projects window to open it.
2.Click on the Design button on the top of the window to open the Design View of our Web service project WebServiceOracle.
3.Click on the Add Operation button to open the Add Operation wizard.
4.Enter QueryCourse into the Name field and click on the Browse button that is next to the
Return Type combo box. Type arraylist into the Type Name field, and select the item ArrayList (java.util) from the list, and click on the OK button.
5.Click on the Add button and enter courseID into the Name parameter field. Keep the default type java.lang.String unchanged and click on the OK button to complete this new operation creation process.
Your finished Add Operation wizard should match the one that is shown in Figure 9.88.
Click on the Source button on the top of this window to open the code window of our Web service project. Let’s perform the coding for this newly added operation.
On the opened code window, enter the codes that are shown in Figure 9.89 into this newly added operation.
Let’s have a closer look at this piece of codes to see how it works.
A.An ArrayList instance al is created and this variable is an array list instance used to collect and store our query result, and return to the consuming project.
B.Before we can call the session bean method getCourse() to query the course details, we need first to clean up the ArrayList instance al to make sure that it is empty.
C.The session bean method getCourse() is called to query the details for a given course_id. The courseID, which is an input parameter, works as the argument for that method. The query result is returned and assigned to the local variable result.
Figure 9.88. The finished Add Operation wizard.