
Schongar P.VBScript unleashed.1997
.pdf<HTML><HEAD>
<SCRIPT LANGUAGE="VBScript">
<!--
dim NOT_FOUND
NOT_FOUND = "NOT_FOUND"
Sub window_onLoad()
Dim lstrTemp, lstrVarName
lstrVarName = "Name"
lstrTemp = ReadVariable(lstrVarName)
if lstrTemp <> NOT_FOUND then txtName.Text = "" & lstrTemp
lstrTemp = ReadVariable("EMail")
if lstrTemp <> NOT_FOUND then txtEMail = cstr(lstrTemp)
end sub
Function ReadVariable(strVariableName)
'these five variables are used in the string manipulation
'code that finds the variable in the cookie.
Dim intLocation
Dim intNameLength
Dim intValueLength
Dim intNextSemicolon
Dim strTemp
'calculate length and location of variable name
intNameLength = Len(strVariableName)
intLocation = Instr(Document.Cookie, strVariableName)
'check for existence of variable name
If intLocation = 0 Then |
|
'variable not found, so it can't |
be read |
ReadVariable = NOT_FOUND |
|
Else |
|
'get a smaller substring to work |
with |
strTemp = Right(Document.Cookie, |
Len(Document.Cookie) - |
intLocation + 1) |
|
'check to make sure we found the full string, not just a substring
If Mid(strTemp, intNameLength + 1, 1) <> "=" Then
'oops, only found substring, not good enough
ReadVariable = NOT_FOUND
'note that this will incorrectly give a not found result if and only if
'a search for a variable whose name is a substring of a preceding
'variable is undertaken. For example, this will fail:
'
'search for: MyVar
'cookie contains: MyVariable=2;MyVar=1
Else
'found full string
intNextSemicolon = Instr(strTemp, ";")
'if not found, then we need the last element of the cookie
If intNextSemicolon = 0 Then intNextSemicolon = Len(strTemp)
+ 1
'check for empty variable (Var1=;)
If intNextSemicolon = (intNameLength + 2) Then
'variable is empty
ReadVariable = ""
Else
'calculate value normally
intValueLength = intNextSemicolon - intNameLength - 2
ReadVariable = Mid(strTemp, intNameLength + 2, intValueLength)
End If
End If
End If
end function
-->
</SCRIPT>
<TITLE>User Information</TITLE>
</HEAD><BODY><pre>
Name: <br> <OBJECT ID="txtName" WIDTH=187 HEIGHT=24
CLASSID="CLSID:8BD21D10-EC42-11CE-9E0D-00AA006002f3">
<PARAM NAME="VariousPropertyBits" VALUE="746604571">
<PARAM NAME="Size" VALUE="4948;635">
<PARAM NAME="FontCharSet" VALUE="0">
<PARAM NAME="FontPitchAndFamily" VALUE="2">
<PARAM NAME="FontWeight" VALUE="0">
</OBJECT>
E-Mail: <br> <OBJECT ID="txtEMail" WIDTH=187 HEIGHT=24
CLASSID="CLSID:8BD21D10-EC42-11CE-9E0D-00AA006002f3">
<PARAM NAME="VariousPropertyBits" VALUE="746604571">
<PARAM NAME="Size" VALUE="4948;635">
<PARAM NAME="FontCharSet" VALUE="0">
<PARAM NAME="FontPitchAndFamily" VALUE="2">
<PARAM NAME="FontWeight" VALUE="0">
</OBJECT>
<SCRIPT LANGUAGE="VBScript">
<!--
Sub cmdSave_Click()
on error resume next
document.Cookie = "Name" & "=" & txtName
if err.number = 438 then
msgbox "Error saving cookie: page is not located on a Web server!"
err.clear
exit sub
elseif err > 0 then
msgbox "Error saving cookie: " & err.description
err.clear
exit sub
end if
document.Cookie = "EMail" & "=" & txtEMail
if err > 0 then
msgbox "Error saving cookie: " & err.description
err.clear
exit sub
end if
MsgBox "Information saved!"
end sub
-->
</SCRIPT>
<OBJECT ID="cmdSave" WIDTH=96 HEIGHT=32
CLASSID="CLSID:D7053240-CE69-11CD-A777-00DD01143C57">
<PARAM NAME="Caption" VALUE="Save">
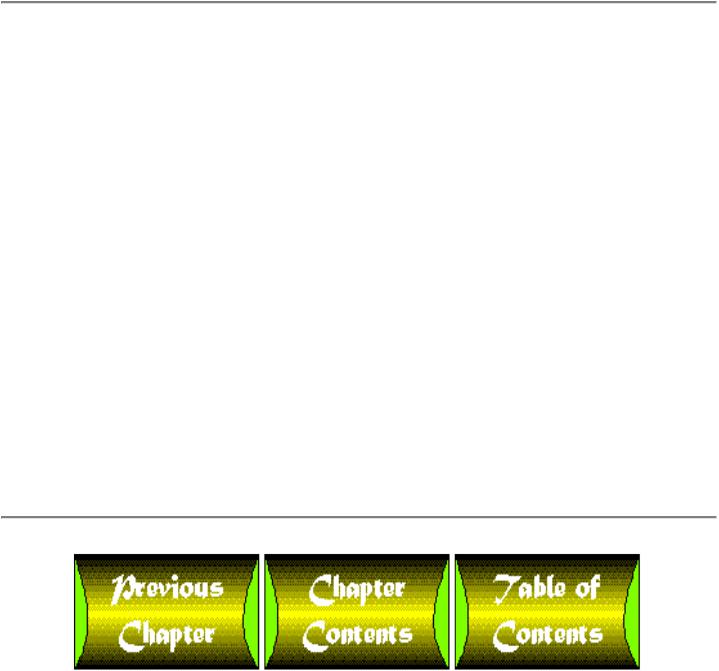
<PARAM NAME="Size" VALUE="2540;846">
<PARAM NAME="FontCharSet" VALUE="0">
<PARAM NAME="FontPitchAndFamily" VALUE="2">
<PARAM NAME="ParagraphAlign" VALUE="3">
<PARAM NAME="FontWeight" VALUE="0">
</OBJECT>
</PRE></BODY></HTML>
In the window_onLoad event, the cookie information is read from the document.cookie property using the ReadVariable() function. If the cookie information (name and e-mail) is found, the data is placed in the appropriate text boxes.
The ReadVariable() parses the cookie property, searching for the variable name specified as the strVariableName parameter. It does this by using the Instr() function to locate the starting position of the string specified in strVariableName. If the string is not found, the constant value NOT_FOUND is returned. If the string is found, the ReadVariable() function then checks to make sure the variable name is not a substring of another variable name. If it passes this test, the value is parsed from the string by taking all the characters between the = and the next semicolon. This string is returned as the function's value.
Finally, the cmdSave_Click event code is responsible for saving the cookie information into the cookie property. It does so by assigning the string variable_name=value to the cookie property. Some error handling is in place to provide the user with any feedback that results from this operation. There are limits to how many cookies can be defined for a site and overstepping these limits would produce an error condition.
Review
This chapter provided you with loads of information useful in creating dynamic Web pages by using VBScript. The examples, although not full-blown applications by themselves, provide the framework for further enhancements. The remainder of this book covers special subjects such as animation and controlling Microsoft Office documents with VBScript code. The final part of the book builds on this part by presenting several real-world examples built using VBScript.
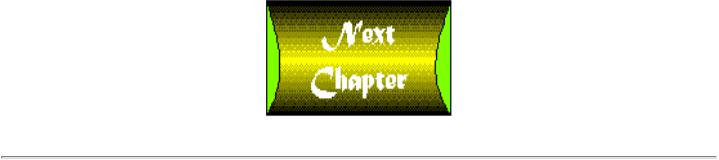
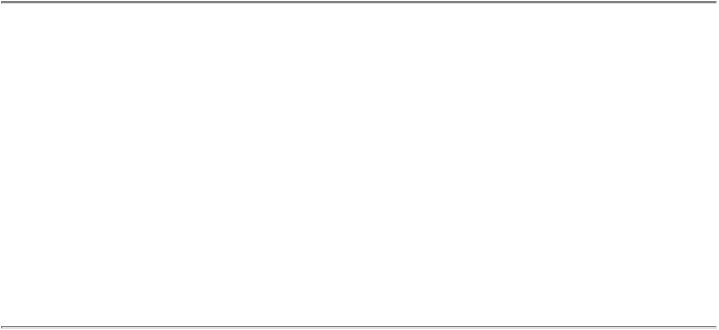
Chapter 25
Order Entry
by Craig Eddy
CONTENTS
●Ensuring Secure Transactions on the Web
Authenticating the Merchant
Authenticating the Purchaser
Encrypting Web Communications
●Reviewing Cookies
●Designing the Order Entry Form
●Creating the Order Entry Form
●Examining the VBScript Code
●Testing the Application
●Review
Perhaps the biggest boon to the growth of the Internet, despite the complaints of many of the Internet's early pioneers, has been the advent of electronic commerce. The World Wide Web has been used for marketing purposes for a few years now. The availability of safe and accurate Web-based ordering is just now becoming a reality, however.
This chapter presents an example of a Web-based order entry application. It is written using a combination of HTML forms and VBScript code. The products available on this order form are static-that is, there is no connection to a database of available products. This makes the Web page easier to design and implement. To add such a connection requires using a server-side application to generate the HTML form and the appropriate VBScript code. This example won't delve into such detail.
The VBScript code in this order entry application performs the following functions:
●Accesses the document's cookie property to save and retrieve information about the user of the order entry form
●Calculates subtotals, shipping charges, and sales tax
●Validates the information entered, such as ZIP code
●Submits the validated information to the server-side process, which accepts the order data
This chapter begins with an overview of security as it relates to Web-based commerce such as online ordering. Then, a brief review of HTTP cookies is presented, which were discussed in Chapter 14, "Customize Your Web Page with Cookies." Finally, you'll see the example application itself.
Ensuring Secure Transactions on the Web
When you discuss the Internet, the first and most hotly debated topic to enter the conversation is the subject of security. The concerns about security on the Web are well-founded given the ease with which a hacker can use well-documented methods to steal information from machines located on or communication occurring on a computer network such as the Internet. Although security isn't required to build and run the VBScript application presented in this chapter, a brief overview of various security concerns seems appropriate.
The typical concerns when dealing with electronic commerce follow:
●Verifying that the merchant is authentic
●Authenticating the user
●Preventing unauthorized access to information
These three concerns and possible solutions are touched on in the following sections. Another very good source of information is the Microsoft Internet Security Framework site located at http://www.microsoft.com/intdev/
security/default.htm.
Authenticating the Merchant
It is necessary to authenticate the merchant (or simply the Web site being accessed) because just about anyone these days can post a Web site and use a variety of methods to request credit card numbers or electronic cash payments. As a potential purchaser of products and services on the Web, you'd like to know that companies you're about to purchase from are who they say they are.
The current trend in merchant authentication is to use site certificates. These are issued by an agency that takes the responsibility to verify that organizations requesting the site certificates are who they say they are. Internet Explorer 3.0 supports the use of site certificates for authentication. Figure 25.1 shows a sample site certificate. Notice the small padlock at the right-hand side of the status bar. This indicates that Internet Explorer currently is viewing a secure and verified site.
Figure 25.1 : A sample site certificate for a secure Web site.
Authenticating the Purchaser
Just as you as a purchaser are concerned with the authenticity of the Web site, the merchant also wants to ensure that you are who you say you are. This is less of a concern than authenticating the merchant because the merchant almost always verifies your ability to pay before providing the product or service. Still, with the possibility of credit card fraud and possible means of counterfeiting electronic cash, merchants still want to be able to verify your identity as a purchaser at their Web sites.
Internet Explorer 3.0 supports personal certificates. These are similar to site certificates and also are issued by an agency that verifies you are, indeed, who you claim to be. Currently, personal certificates are provided by an agency named VeriSign. Its Web site provides a very good overview of personal certificates and how they are obtained and used. The URL for the page is http://digitalid.verisign.com/brw_intr.htm.
Encrypting Web Communications
Obviously, if you are willing to send credit card information across a public network like the Internet, you want to know that no one else can view that information. The same holds true for personal information such as household income. Several encryption standards currently are being debated, and some are in place within Web browsers today. Eventually, one or two standards will win out; until at least one gains general acceptance, everyday purchasing on the Web will remain a fantasy. Site certificates also play a vital role in securing the channels of communication.
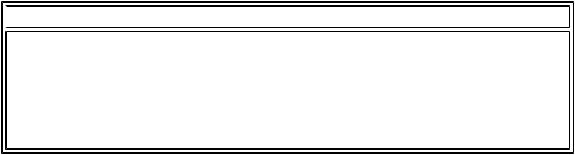
Reviewing Cookies
The term cookie, when used in reference to a Web page, refers to a string of characters that is stored for the particular Web page or site. The cookie can store any type of information you want. Typically, cookies store information that identifies the user of the Web browser (name, address, and phone number) or retain some state information (when the user last accessed the page, what his personal preferences related to the page are, and so on).
Using VBScript, you can access the cookie for a page using the document.cookie property. The format of the string is variable_name=value. Multiple variable/value pairs are stored by separating the pairs with a semicolon. For example, the cookie property may evaluate to this:
LastName=Eddy;FirstName=Craig;FavoriteColor=Green
This cookie has three variables stored: LastName, FirstName, and FavoriteColor.
You set the cookie for a page by assigning a string formatted as variable_name=value to the document. cookie property. For example,
document.cookie = "FavoriteColor=Yellow"
assigns or changes the setting for the variable FavoriteColor.
Cookie variables also can be set to expire at a certain time. This is accomplished by appending the string ; expires=date/time. The cookie variable then is invalid after the date/time specified.
To remove a cookie variable, set its value to the string NULL and append an expires with a value set to some date in the past.
NOTE
Cookies are valid only if the page being accessed is stored on a Web server. This is due to the fact that cookies are actually part of the transport protocol used to communicate on the Web. If you attempt to assign a value to the cookie property for a page not located on a Web server, your script code produces a trappable runtime error.
Designing the Order Entry Form
The order entry form created in this chapter is an order form that is static. For the sake of simplicity, no attempt is made to retrieve data from a product database and assemble an order form from that data.
The products sold using this form are framed portraits of United States presidents (hey, why not?). The user will be able to order any quantity of the available portraits. Shipping and handling, as well as sales tax, are calculated by the VBScript code so that users always know exactly how much the purchase will cost them. The script code also verifies the entered phone number and ZIP code for proper formatting before submitting the form data.
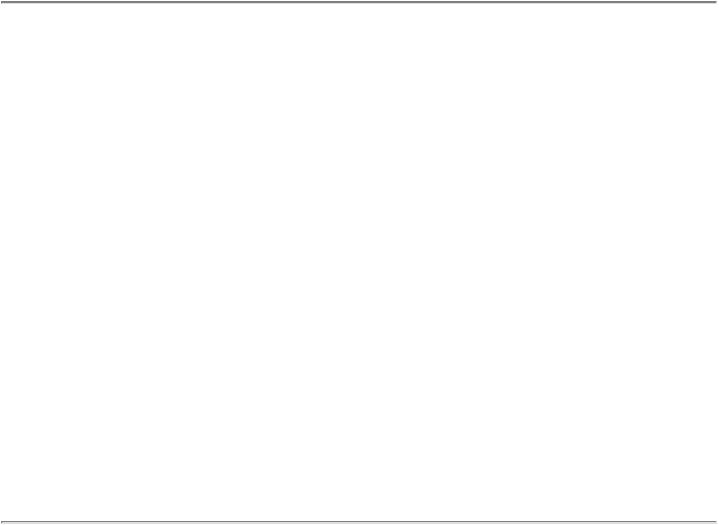
Figure 25.2 shows the order entry form. The top of the form contains header information: customer name, address, and phone number. This is followed by the order form that gives the user the details about each portrait: description, cost, and shipping charge. The user enters the quantity of each portrait to be ordered, and the script code updates the various subtotals and the grand total. The shipping cost, product total cost, and grand total are displayed in a separate frame that always appears at the bottom of the browser, regardless of where the user has scrolled to on the order form.
Figure 25.2 : The order entry form displayed with Internet Explorer.
Listing 25.1 shows the HTML used to create the frames. You can type it in or copy it from the CD-ROM. Save it to a Web server directory if possible. The name is irrelevant, but you'll need to remember what it is because this is the file that you'll open with Internet Explorer when it's time to test the application.
Listing 25.1. The frame container for the order entry page.
<HTML>
<HEAD>
<TITLE>Portrait Order Form</TITLE>
</HEAD>
<BODY>
<FRAMESET FRAMEBORDER=NO ROWS="90,10">
<FRAME SCROLLING=YES SRC=25-3.htm>
<FRAME SCROLLING=NO MARGINHEIGHT=5 MARGINWIDTH=5 SRC=25-2.htm>
</FRAMESET>
</BODY>
</HTML>
This Web page makes pretty basic use of frames. The <FRAMESET> tag specifies the start of a frame definition. The FRAMEBORDER=NO specifies that there should be no borders around the frames. ROWS="90,10" specifies that the frames will be stacked vertically. The top frame will take up 90 percent of the browser's viewing area, and the bottom frame uses 10 percent.
The <FRAME SCROLLING=YES SRC=25-3.htm> tag for the first frame in the frameset specifies that the frame is to be scrollable and that a file named 25-3.htm should be used as the content for the frame.
The <FRAME SCROLLING=NO MARGINHEIGHT=5 MARGINWIDTH=5 SRC=25-2.htm> tag for the second frame in the frameset specifies that the frame is not scrollable and that a file named 25-2.htm should be used as the content for the frame. This is the bottom frame shown in Figure 25.2.