
Schongar P.VBScript unleashed.1997
.pdf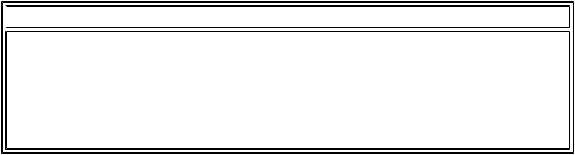
property. To set a cookie that expires on December 31, 1999 at midnight Greenwich mean time, for example, use this code:
Document.Cookie = strCookieName & "=" & strCookieValue & "; expires=31-Dec-99 GMT"
To remove a cookie from the client's machine, set its value to NULL and give it an expires attribute that matches some date in the past. For example:
Document.Cookie = strCookieName & "=NULL;expires=01-Aug-96"
removes the cookie specified in strCookieName.
The section "Using Cookies to Maintain User Information," later in this chapter, demonstrates in more detail how to access cookies using VBScript code. For a technical discussion of cookies in general, see the Netscape page describing them at http://home.netscape.com/newsref/std/cookie_spec.html.
NOTE
To use cookies, the page must be stored on and served by a Web server. This is due to the fact that cookies are actually a part of the HTTP specification and therefore require an HTTP client/server conversation in order to work. Therefore, cookies will not work when you are saving a page to a local hard drive and loading it using Internet Explorer's file browser.
The anchors Collection
The anchors collection is an array of the anchors contained in the document. An anchor is a named reference to a section of the document. Anchors are defined using the HTML tag <a NAME=anchor-name>...</a> and can be referenced in a link by <a HTEF=page-url#anchor-name>. This URL loads the page specified and transfers the user to the place where the anchor is specified.
The anchors collection has a length property. To determine how many anchors are contained in the document, use document.anchors.length. To reference the string for the first anchor in the document, use document. anchors[0].
The links Collection
The links collection is almost identical to the anchors collection. It also has a length property, and you reference items in the array using document.links[x]. The links array contains all the hyperlinks that take you to another page or to an anchor in the current document.
The forms Collection
The forms collection returns an array of all the HTML forms contained in the document. It also has a length property, and individual form objects are referenced using document.forms[x]. You then can reference an individual element of a form using document.forms[x].input1, for example.

Methods
The document object provides several useful methods that can be used to manipulate the contents of the document and open a new document.
The Write and Writeln Methods
These methods are used to print HTML text to the document. The text is printed at the point where the script is placed. The text is placed directly into the document and therefore must be formatted as HTML text. The difference between the two methods is that the Writeln method also appends a newline character to the end of the text written to the document.
The section "Writing the Current Date and Time to the Page," later in this chapter, gives you several examples of using the Write and Writeln methods. As a quick example, the HTML code in Listing 13.1 produces the Web page shown in Figure 13.1.
Figure 13.1 : The Writeln example's resulting page.
Listing 13.1. Writeln sample code.
<HTML><HEAD>
<TITLE>New Page</TITLE>
</HEAD><BODY>
<SCRIPT LANGUAGE="VBScript">
<!--
window.Document.Writeln "appCodeName: " & window.navigator. appCodeName & "<br>"
window.Document.Writeln "appName: " & window.navigator.appName & "<br>"
window.Document.Writeln "appVersion: " & window.navigator.appVersion & "<br>"
window.Document.Writeln "userAgent: " & window.navigator.UserAgent & "<br>"
window.Document.Writeln "LastModified: " & window.Document. LastModified & "<br>"
window.Document.Writeln "Referrer: " & window.Document.Referrer & "<br>"
window.Document.Writeln "Title: " & window.Document.Title & "<br>"
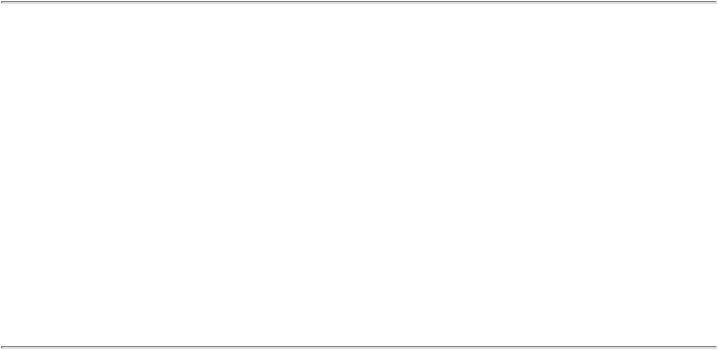
window.Document.Writeln "Location: " & window.Document.Location & "<br>"
-->
</SCRIPT>
<H1>Document.Writeln Example</H1>
<SCRIPT LANGUAGE="VBScript">
<!--
Document.Writeln "appCodeName: " & window.navigator.appCodeName & "<br>"
-->
</SCRIPT>
</BODY>
</HTML>
The Open, Close, and Clear Methods
The Open, Close, and Clear methods are used to create and write to a completely new document object. The Open method is used to start a new document. It clears the current document and prepares it for writing. The Close method is used after HTML text is written to the document to display all the text written to the document since the Open method was invoked. The text is not displayed until the Close method is used. The Clear method is used to clear the document of any existing data.
As a quick example, the code in Listing 13.2 produces the Web page shown in Figure 13.2. Note that only the text from the window_onLoad event code is shown-not the HTML contained in the <BODY>...</BODY> section of the listing. When the page loads, the <H1> section's text shows up briefly and then is replaced by the text from the onLoad event.
Figure 13.2 : The Open/Close example's resulting page.
Listing 13.2. Using the Open and Close methods.
<HTML><HEAD>
<SCRIPT LANGUAGE="VBScript">

<!--
Sub window_onLoad()
window.document.open "text/html"
window.document.writeln "<H2>"
window.document.writeln "This text is from the window_onLoad event!"
window.document.writeln "</H2>"
window.document.close
end sub
-->
</SCRIPT>
<TITLE>New Page</TITLE>
</HEAD>
<BODY>
<H1>This Doesn't Show Up!!!</H1>
</BODY>
</HTML>
Using the ActiveX Timer Control
Now that you've learned how to manipulate the Document object, it's time to put that knowledge to use. This section describes the ActiveX Timer object and how to use it to create dynamic documents.
You can download the ActiveX Timer control from the Microsoft Web site at http://www.microsoft.com/
workshop/activex/gallery/ms/timer/sample.htm. Before you go to the trouble, however, check to see
whether you already have a copy on your system. The best way to do so is to run the ActiveX Control Pad and choose the Edit | Insert ActiveX Control menu. The Timer control appears as Timer Object in the Control Type list box.
The Timer control has three properties that are useful within VBScript code: Interval, Enabled, and ID. There is only one event: Timer. The Interval property determines the amount of time, in milliseconds, between calls of the Timer event. The Enabled property enables and disables the control. The ID property serves as the control's name. The Timer event is the event that fires with each passing of the time specified by the Interval property.
You can use the Timer control to create any type of dynamic effect you want. You can use it to randomly change the status bar text, open new documents, navigate to another Web page, and so on. The code in Listing 13.3 shows an example of opening a new document two seconds after the page loads and writing some text to it. Figure 13.3 shows the resulting page.

Figure 13.3 : The resulting page from the ActiveX Timer control example.
Listing 13.3. Using the ActiveX Timer control.
<HTML><HEAD>
<TITLE>New Page</TITLE>
</HEAD>
<BODY>
<H1>This Will Disappear Soon!!!</H1>
<SCRIPT LANGUAGE="VBScript">
<!--
Sub IeTimer1_Timer()
window.document.open "text/html"
window.document.writeln "<H2>"
window.document.writeln "This text is from the "
window.document.writeln "Timer event!"
window.document.writeln "</H2>"
window.document.close
end sub
-->
</SCRIPT>
<OBJECT ID="IeTimer1" WIDTH=39 HEIGHT=39
CLASSID="CLSID:59CCB4A0-727D-11CF-AC36-00AA00A47DD2">
<PARAM NAME="_ExtentX" VALUE="1032">
<PARAM NAME="_ExtentY" VALUE="1032">
<PARAM NAME="Interval" VALUE="2000">
</OBJECT>
</BODY>
</HTML>
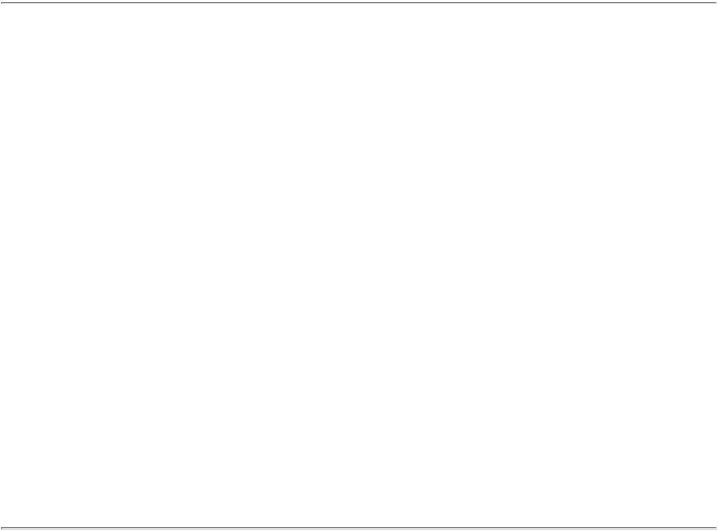
The page initially loads and displays the message This Will Disappear Soon!!!. After two seconds, the value of the Interval property, the IeTimer1_Timer event, fires. This event uses the Document object's Open, Writeln, and Close methods to create a new document and print the text This text is from the Timer event! Note that on the new document there is no Timer control, so the code executes only once. Because you're writing HTML to the document, however, you could have placed the Timer object and its associated code into the new document. This could be used to create a slide show but, due to the cascading nature of the code you'd have to enter into the script, it would be hard to maintain.
Writing the Current Date and Time to the Page
Perhaps one of the most useful features for creating dynamic Web pages is the capability to write the current date and time to the page. This is accomplished using the Document object's Writeln property and VBScript's various date and time functions.
The concept is simple: As the page loads, an embedded script uses the Document.writeln method to print a string containing the current date and time at the point in the HTML file where the script appears. Using the various date/time functions, you can format the string in any manner you want. The example presented in this section formats the date as Friday, August 9, 1996 and leaves the time in the format returned by the VBScript Time function.
Although the example presented here is not all that useful by itself, when combined with a "real" Web page, it provides a nice touch for your site. Figure 13.4 shows the output from the script. The code is presented in Listing 13.4.
Figure 13.4 : The current date/time written to the page.
Listing 13.4. The code for the date/time example.
<HTML><HEAD>
<TITLE>Current Date & Time</TITLE>
</HEAD><BODY>
<center>
<SCRIPT LANGUAGE="VBScript">
<!--
Dim DayOfWeek
Dim MonthText
Dim strTemp
select case Weekday(Date)
case 1
DayOfWeek = "Sunday"
case 2
DayOfWeek = "Monday"
case 3
DayOfWeek = "Tuesday"
Case 4
DayOfWeek = "Wednesday"
case 5
DayOfWeek = "Thursday"
case 6
DayOfWeek = "Friday"
case 7
DayOfWeek = "Saturday"
end select
Select Case Month(Date)
case 1
MonthText = "January"
case 2
MonthText = "February"
case 3
MonthText = "March"
case 4
MonthText = "April"
case 5
MonthText = "May"
case 6
MonthText = "June"

case 7
MonthText = "July"
case 8
MonthText = "August"
case 9
MonthText = "September"
case 10
MonthText = "October"
case 11
MonthText = "November"
case 12
MonthText = "December"
end select
strTemp = DayOfWeek & ", " & MonthText & " "
strTemp = strTemp & Day(Date) & ", " & Year(Date) & "<br>"
document.writeln strTemp
document.writeln Time
-->
</SCRIPT>
</center>
</BODY></HTML>
The script code shown in Listing 13.4 is embedded within the HTML file's <BODY> section. This section contains the HTML that will be visible in the document window of the Web browser. The <CENTER> tag is used to center the date and time strings within the document window. The code begins by declaring three local variables: DayOfWeek, MonthText, and strTemp. These are used to construct the date string that will be displayed. The script then uses a Select Case construct to place the name of the current day of the week in DayOfWeek. The Weekday() function returns an integer corresponding to the day of the week for the date passed to it as its only parameter. In this case, the Date function is used; it returns the current date.
After the DayOfWeek value is assigned, another Select Case is used to assign a value to MonthText. This time, the Month() function is called in order to retrieve the number of the current month. Finally, the value for strTemp is assigned by combining the previous variables with the Day() and Year() functions. The value of strTemp is written

to the document window using the writeln method. Then, the current time (returned by the Time function) also is written to the document.
Notice that this produces a static display. After the page is loaded, the date and time are not updated. If you want to create an updating date/time display, simply add ActiveX Label and Timer controls. Set the timer's Interval property to be the time between updates of the display and use 1000 for one second between updates. Then, in the Timer event, simply assign the strings created in the preceding example to the label's Caption property. Listing 13.5 provides an example of how this can be accomplished.
Listing 13.5. The code for the date/time using a label example.
<HTML>
<HEAD> <SCRIPT LANGUAGE="VBScript">
<!--
Sub window_onLoad()
Call IeTimer1_Timer
end sub
-->
</SCRIPT>
<TITLE>Updating Clock</TITLE>
</HEAD>
<BODY>
<CENTER>
<OBJECT ID="lblDate" WIDTH=259 HEIGHT=27
CLASSID="CLSID:99B42120-6EC7-11CF-A6C7-00AA00A47DD2">
<PARAM NAME="_ExtentX" VALUE="6853">
<PARAM NAME="_ExtentY" VALUE="714">
<PARAM NAME="Caption" VALUE="">
<PARAM NAME="Angle" VALUE="0">
<PARAM NAME="Alignment" VALUE="1">
<PARAM NAME="Mode" VALUE="1">
<PARAM NAME="FillStyle" VALUE="0">
<PARAM NAME="FillStyle" VALUE="0">
<PARAM NAME="ForeColor" VALUE="#000000">
<PARAM NAME="BackColor" VALUE="#C0C0C0">
<PARAM NAME="FontName" VALUE="Arial">
<PARAM NAME="FontSize" VALUE="12">
<PARAM NAME="FontItalic" VALUE="0">
<PARAM NAME="FontBold" VALUE="0">
<PARAM NAME="FontUnderline" VALUE="0">
<PARAM NAME="FontStrikeout" VALUE="0">
<PARAM NAME="TopPoints" VALUE="0">
<PARAM NAME="BotPoints" VALUE="0">
</OBJECT>
<br>
<OBJECT ID="lblTime" WIDTH=259 HEIGHT=39
CLASSID="CLSID:99B42120-6EC7-11CF-A6C7-00AA00A47DD2">
<PARAM NAME="_ExtentX" VALUE="6853">
<PARAM NAME="_ExtentY" VALUE="1032">
<PARAM NAME="Caption" VALUE="">
<PARAM NAME="Angle" VALUE="1">
<PARAM NAME="Alignment" VALUE="1">
<PARAM NAME="Mode" VALUE="1">
<PARAM NAME="FillStyle" VALUE="0">
<PARAM NAME="FillStyle" VALUE="0">
<PARAM NAME="ForeColor" VALUE="#000000">
<PARAM NAME="BackColor" VALUE="#C0C0C0">
<PARAM NAME="FontName" VALUE="Arial">
<PARAM NAME="FontSize" VALUE="12">
<PARAM NAME="FontItalic" VALUE="0">
<PARAM NAME="FontBold" VALUE="0">
<PARAM NAME="FontUnderline" VALUE="0">