
Ответы к экзамену 0
.4.pdf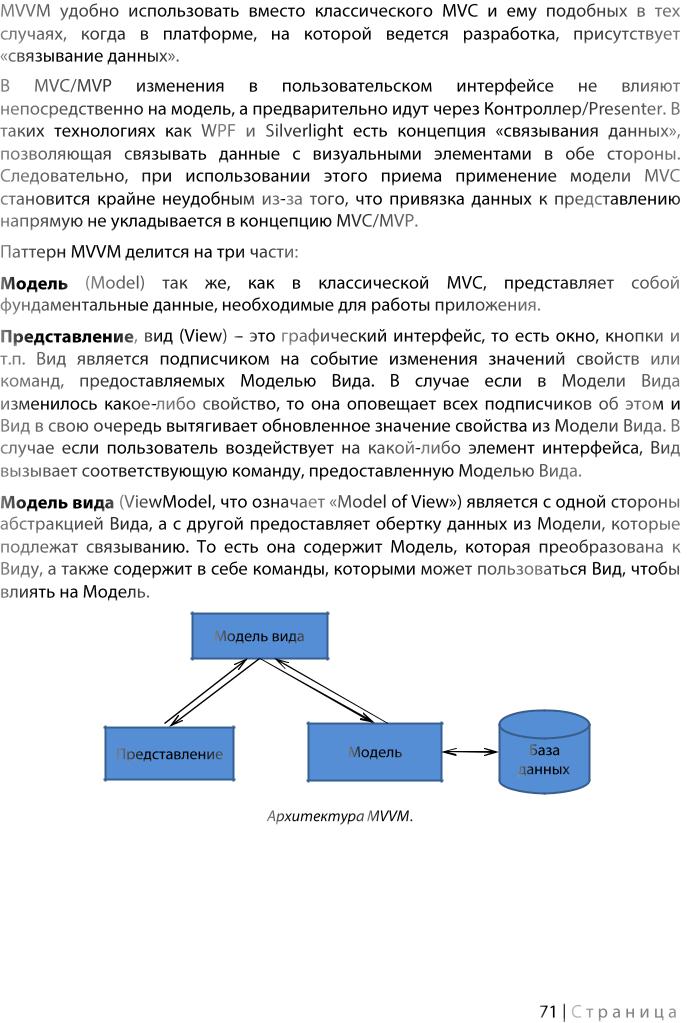

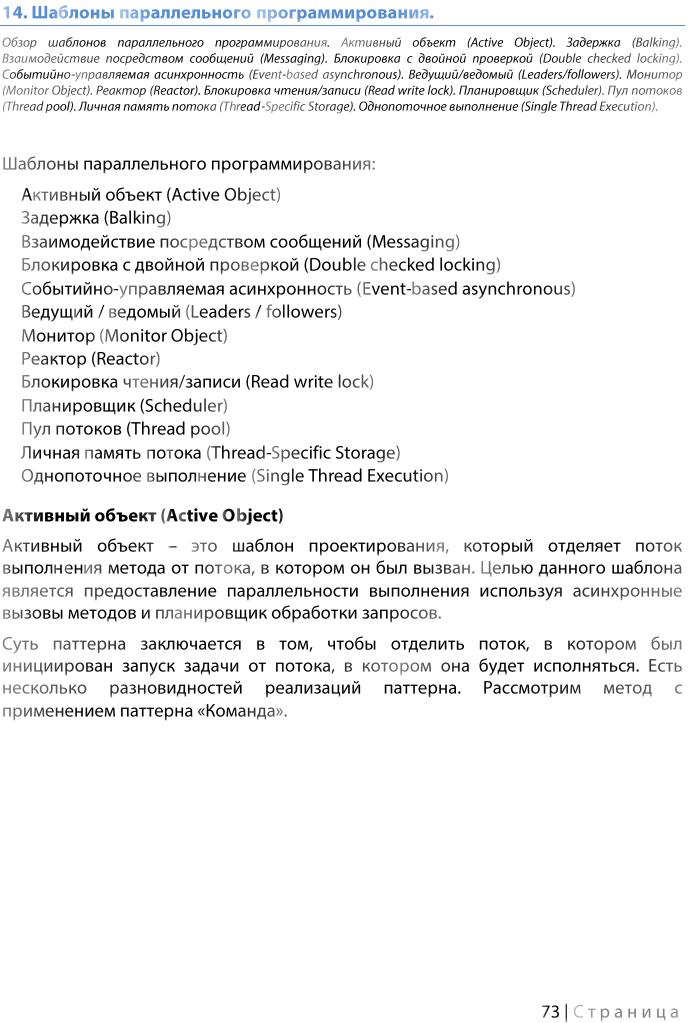


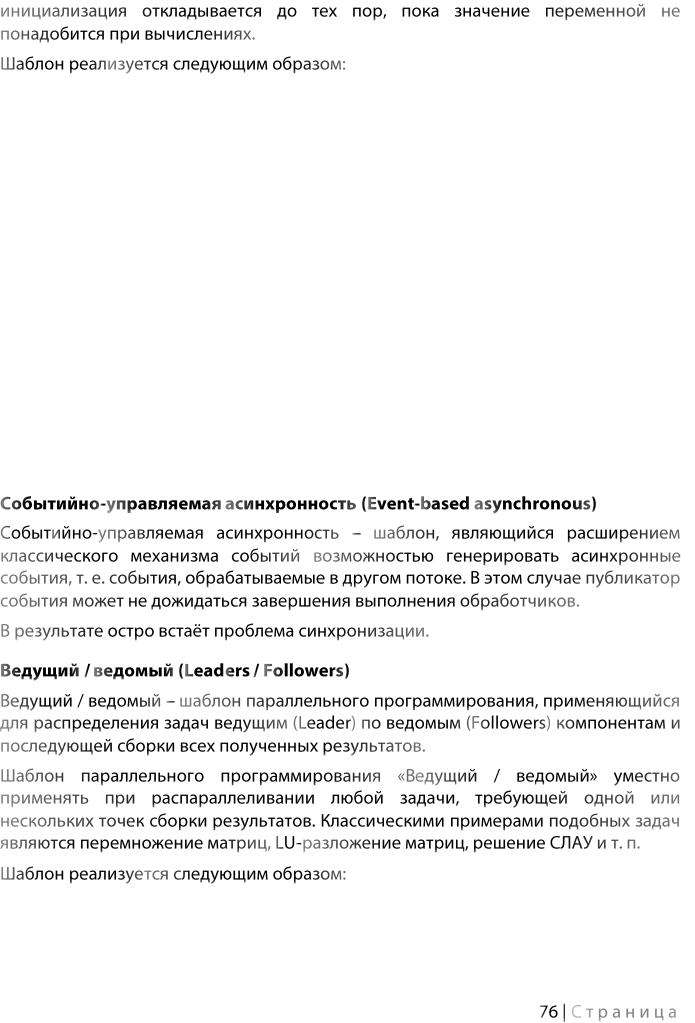
public sealed class Singleton
{
private Singleton()
{
// инициализировать новый экземпляр объекта
}
private static volatile Singleton singletonInstance;
private static readonly Object syncRoot = new Object();
public static Singleton GetInstance()
{
// создан ли объект
if (singletonInstance == null)
{
//нет, не создан
//только один поток может создать его lock (syncRoot)
{
//проверяем, не создал ли объект другой поток if (singletonInstance == null)
{
//нет не создал — создаём
singletonInstance = new Singleton();
}
}
}
return singletonInstance;
}
}
using System; using System.Data;
using System.Drawing; using System.Windows.Forms; using System.Threading;
namespace LeaderFollower

{
class Leader
{
static public int[][] matrixMultiplication(int[][] A, int[][] B)
{
Follower[][] followers = new Follower[A.Length][]; Thread[][] threads = new Thread[A.Length][];
for (int i = 0; i < A.Length; i++)
{
threads[i] = new Thread[A.Length]; followers[i] = new Follower[A.Length]; for (int j = 0; j < A.Length; j++)
{
followers[i][j] = new Follower(A[i], B[j]);
threads[i][j] = new Thread(new ThreadStart(followers[i][j].count)); threads[i][j].Start();
}
}
for (int i = 0; i < A.Length; i++)
for (int j = 0; j < A.Length; j++) threads[i][j].Join();
int[][] result = new int[A.Length][]; for (int i = 0; i < A.Length; i++)
{
result[i] = new int[A.Length]; for (int j = 0; j < A.Length; j++)
result[i][j] = followers[i][j].result;
}
return result;
}
}
class Follower
{
public int result = 0; private int[] A; private int[] B;
public Follower(int[] A, int[] B)
{
this.A = A; this.B = B;
}
public void count()
{
for (int i = 0; i < A.Length; i++) result += A[i] * B[i];
}
}
public partial class Form1 : Form
{
private System.Windows.Forms.TextBox[][] A = new System.Windows.Forms.TextBox[3][]; private System.Windows.Forms.TextBox[][] B = new System.Windows.Forms.TextBox[3][]; private System.Windows.Forms.TextBox[][] C = new System.Windows.Forms.TextBox[3][]; private int[][] Amatrix = new int[3][];
private int[][] Bmatrix = new int[3][]; private int[][] Cmatrix = new int[3][];
public Form1() { InitializeComponent();
System.Drawing.Font font = new System.Drawing.Font("Microsoft Sans Serif", 12F, System.Drawing.FontStyle.Regular,System.Drawing.GraphicsUnit.Point,((byte)(204))); HorizontalAlignment center = System.Windows.Forms.HorizontalAlignment.Center; System.Random rand = new System.Random();
for (int i = 0; i < 3; i++) {
A[i] = new System.Windows.Forms.TextBox[4]; Amatrix[i] = new int[4];
for (int j = 0; j < 4; j++) { Amatrix[i][j] = rand.Next(0, 10);
A[i][j] = new System.Windows.Forms.TextBox(); A[i][j].Font = font;
A[i][j].Location = new System.Drawing.Point(40 + 54 * j, 45 + 30 * i);
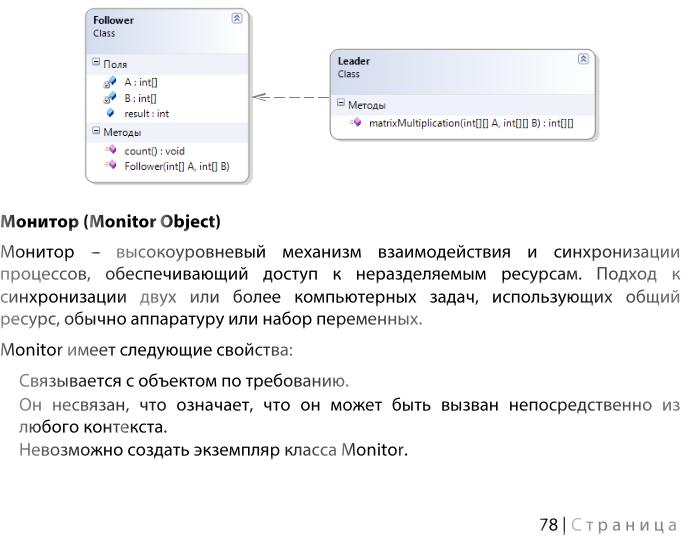
A[i][j].Size = new System.Drawing.Size(50, 26); A[i][j].Text = Amatrix[i][j].ToString(); A[i][j].TextAlign = center; this.Controls.Add(A[i][j]);
}
}
for (int i = 0; i < 3; i++) {
B[i] = new System.Windows.Forms.TextBox[4]; Bmatrix[i] = new int[4];
for (int j = 0; j < 4; j++) { Bmatrix[i][j] = rand.Next(0, 10);
B[i][j] = new System.Windows.Forms.TextBox(); B[i][j].Font = font;
B[i][j].Location = new System.Drawing.Point(300 + 54 * i, 30 + 30 * j); B[i][j].Size = new System.Drawing.Size(50, 26);
B[i][j].Text = Bmatrix[i][j].ToString(); B[i][j].TextAlign = center; this.Controls.Add(B[i][j]);
}
}
Cmatrix = Leader.matrixMultiplication(Amatrix, Bmatrix);
for (int i = 0; i < 3; i++) {
C[i] = new System.Windows.Forms.TextBox[3]; for (int j = 0; j < 3; j++) {
C[i][j] = new System.Windows.Forms.TextBox(); C[i][j].Font = font;
C[i][j].Location = new System.Drawing.Point(510 + 54 * j, 45 + 30 * i); C[i][j].Size = new System.Drawing.Size(50, 26);
C[i][j].Text = Cmatrix[i][j].ToString(); C[i][j].TextAlign = center; this.Controls.Add(C[i][j]);
}
}
}
}
}

using System;
using System.Threading; using System.Collections;
class MonitorSample
{
const int MAX_LOOP_TIME = 1000; Queue m_smplQueue;
public MonitorSample()
{
m_smplQueue = new Queue();
}
public void FirstThread()
{
int counter = 0; lock (m_smplQueue)
{
while (counter < MAX_LOOP_TIME)
{
//Wait, if the queue is busy. Monitor.Wait(m_smplQueue); //Push one element. m_smplQueue.Enqueue(counter); //Release the waiting thread.
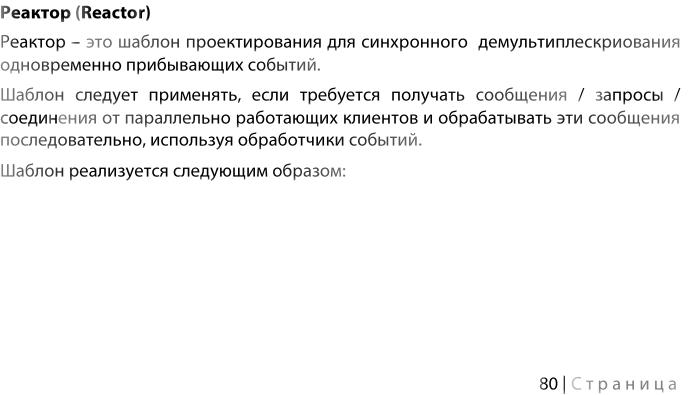
Monitor.Pulse(m_smplQueue);
counter++;
}
}
}
public void SecondThread()
{
lock (m_smplQueue)
{
//Release the waiting thread. Monitor.Pulse(m_smplQueue);
//Wait in the loop, while the queue is busy. //Exit on the time-out when the first thread stops. while (Monitor.Wait(m_smplQueue, 1000))
{
//Pop the first element.
int counter = (int)m_smplQueue.Dequeue(); //Print the first element. Console.WriteLine(counter.ToString()); //Release the waiting thread. Monitor.Pulse(m_smplQueue);
}
}
}
//Return the number of queue elements. public int GetQueueCount()
{
return m_smplQueue.Count;
}
static void Main(string[] args)
{
//Create the MonitorSample object. MonitorSample test = new MonitorSample(); //Create the first thread.
Thread tFirst = new Thread(new ThreadStart(test.FirstThread)); //Create the second thread.
Thread tSecond = new Thread(new ThreadStart(test.SecondThread)); //Start threads.
tFirst.Start();
tSecond.Start();
//wait to the end of the two threads tFirst.Join();
tSecond.Join();
//Print the number of queue elements.
Console.WriteLine("Queue Count = " + test.GetQueueCount().ToString());
}
}
using System;
using System.Collections.Generic; using System.Text;
using System.Threading; using System.Net;
using System.Net.Sockets; using System.Linq;
public interface ISynchronousEventDemultiplexer
{
IList<TcpListener> Select(ICollection<TcpListener> listeners);
}