
Ответы к экзамену 0
.4.pdf
#include <cstdio> #include <stdexcept>
class file
{
public:
file( const char* filename ) : m_file_handle(std::fopen(filename, "w+"))
{
if( !m_file_handle )
throw std::runtime_error("file open failure") ;
}
~file()
{
if(std::fclose(m_file_handle) != 0)
{
// fclose() может вернуть ошибку при записи на диск последних изменений
}
}
void write(const char* str)
{
if(std::fputs(str, m_file_handle) == EOF)
throw std::runtime_error("file write failure");
}
private:
std::FILE* m_file_handle;
//Копирование и присваивание не реализовано. Предотвратим их использование,
//объявив соответствующие методы закрытыми.
file( const file &);
file & operator = (const file & ) ;
};
int _tmain(int argc, _TCHAR* argv[])
{
// открываем файл (захватываем ресурс) file logfile("logfile.txt") ;
logfile.write("hello logfile!") ;
//продолжаем использовать logfile...
//Можно возбуждать исключения или выходить из функции не беспокоясь о закрытии файла;
//он будет закрыт автоматически когда переменная logfile выйдет из области видимости. return 0;
}
using System;
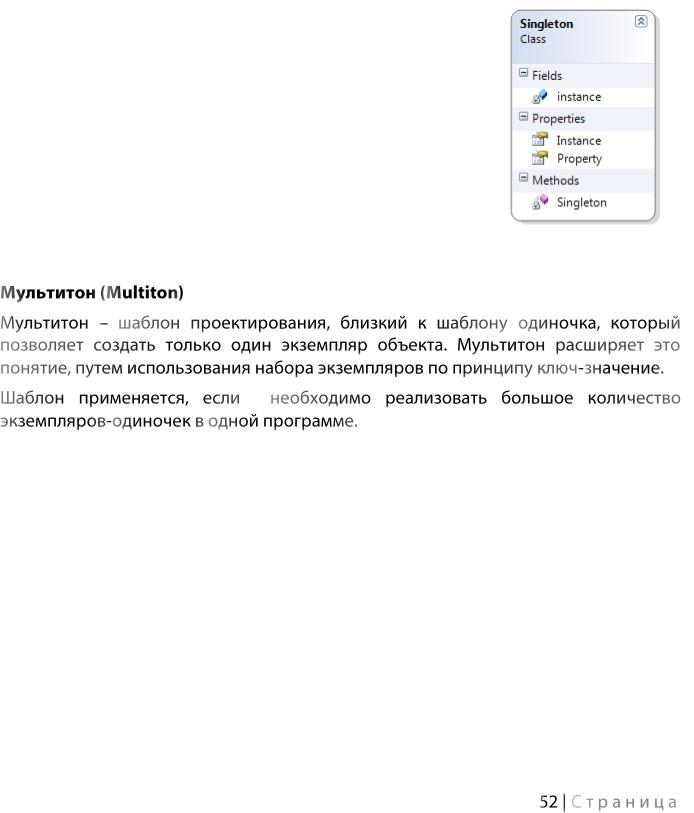
class Singleton
{
static Singleton instance;
private Singleton() { }
public static Singleton Instance
{
get
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
public int Property
{
get; set;
}
}
class Program
{
static void Main(string[] args)
{
Singleton instance = Singleton.Instance; instance.Property = 3;
Console.WriteLine("Значение свойства: " + instance.Property); instance.Property = 2;
Console.WriteLine("Значение свойства: " + instance.Property);
Console.ReadKey();
}
}
class Multiton
{
static Dictionary<object, Multiton> instances = new Dictionary<object, Multiton>();
Multiton() { }
public static Multiton Instance(object key)
{
Multiton instance;
if (!instances.TryGetValue(key, out instance))
{
instance = new Multiton(); instances.Add(key, instance);
}
return instance;
}
public int Property { get; set; }
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Новый объект");
Multiton instance1 = Multiton.Instance("Ключ 1"); instance1.Property = 3;
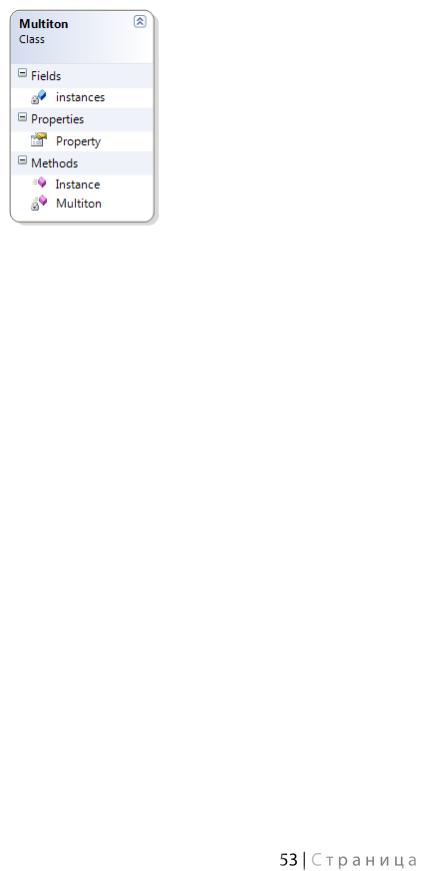
Console.WriteLine("Значение свойства: " + instance1.Property); instance1.Property = 2;
Console.WriteLine("Значение свойства: " + instance1.Property);
Multiton instance2 = Multiton.Instance(123); instance2.Property = 11; Console.WriteLine("Новый объект");
Console.WriteLine("Значение свойства: " + instance2.Property); Console.Read();
}
}
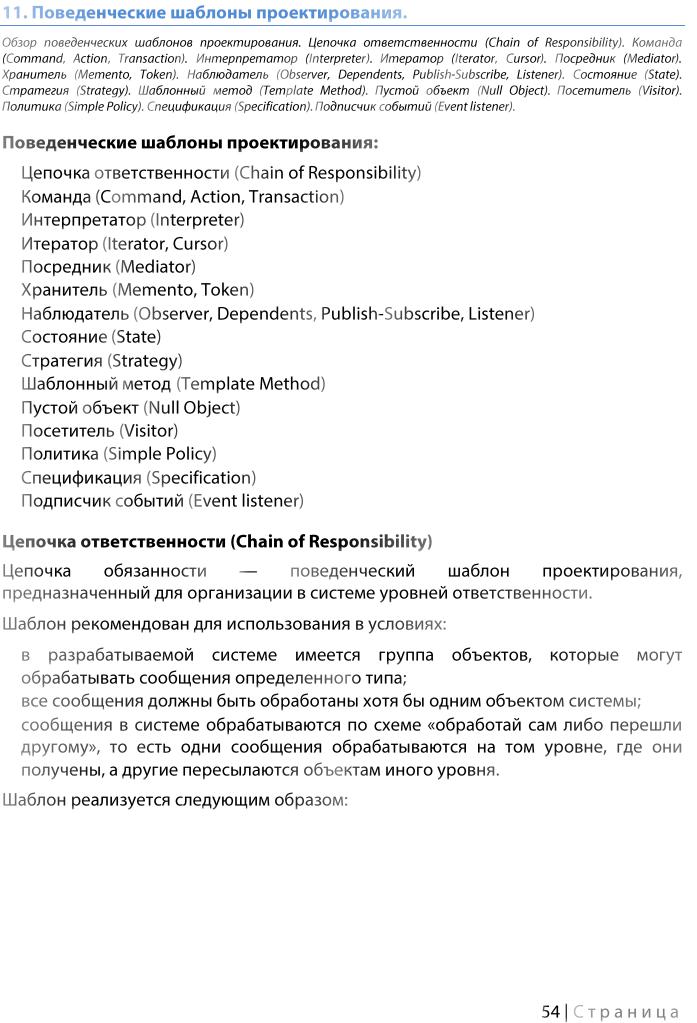
abstract class Worker
{
protected Worker successor;
public void SetSuccessor(Worker successor)
{
this.successor = successor;
}
public abstract void Execute(int difficulty);
}
class Novice : Worker

{
public override void Execute(int difficulty)
{
if (difficulty < 1)
{
Console.WriteLine(this.GetType().Name, difficulty);
}
else if (successor != null)
{
successor.Execute(difficulty);
}
}
}
class Specialist : Worker
{
public override void Execute(int difficulty)
{
if (difficulty < 2)
{
Console.WriteLine(this.GetType().Name, difficulty);
}
else if (successor != null)
{
successor.Execute(difficulty);
}
}
}
class Expert : Worker
{
public override void Execute(int difficulty)
{
if (difficulty < 3)
{
Console.WriteLine(this.GetType().Name, difficulty);
}
else if (successor != null)
{
successor.Execute(difficulty);
}
}
}
class Program
{
static void Main(string[] args)
{
Worker novice = new Novice();
Worker specialist = new Specialist(); Worker expert = new Expert(); novice.SetSuccessor(specialist); specialist.SetSuccessor(expert);
novice.Execute(1);
Console.Read();
}
}

interface Command
{
void Execute();
}
class Command1 : Command
{
Reciever reciever;
public Command1(Reciever reciever)
{
this.reciever = reciever;
}
public void Execute()
{
reciever.setOn();
}
}
class Command2 : Command
{
Reciever reciever;
public Command2(Reciever reciever)
{
this.reciever = reciever;
}
public void Execute()
{
reciever.setOff();

}
}
class Invoker
{
private Command command;
public void setCommand(Command command)
{
this.command = command;
}
public void invokeCommand()
{
command.Execute();
}
}
class Reciever
{
bool field;
public void showField()
{
Console.WriteLine(field);
}
public void setOn()
{
field = true;
}
public void setOff()
{
field = false;
}
}
class Program
{
static void Main(string[] args)
{
Invoker invoker = new Invoker();
Reciever reciever = new Reciever();
Command1 c1 = new Command1(reciever); Command2 c2 = new Command2(reciever);
invoker.setCommand(c1);
invoker.invokeCommand();
reciever.showField();
invoker.setCommand(c2);
invoker.invokeCommand();
reciever.showField();
Console.Read();
}
}

abstract class Expression
{
public void Interpret(Context context)
{
if (context.Input.Length == 0) return;
if (context.Input.StartsWith(Nine()))
{
context.Output += (9 * Multiplier()); context.Input = context.Input.Substring(2);
}
else if (context.Input.StartsWith(Four()))
{
context.Output += (4 * Multiplier()); context.Input = context.Input.Substring(2);
}
else if (context.Input.StartsWith(Five()))
{
context.Output += (5 * Multiplier()); context.Input = context.Input.Substring(1);
}
while (context.Input.StartsWith(One()))
{
context.Output += (1 * Multiplier()); context.Input = context.Input.Substring(1);
}
}
public abstract string One(); public abstract string Four();

public abstract string Five(); public abstract string Nine(); public abstract int Multiplier();
}
class ThousandExpression : Expression
{
public override string One() { return "M"; } public override string Four() { return " "; } public override string Five() { return " "; } public override string Nine() { return " "; } public override int Multiplier() { return 1000; }
}
class HundredExpression : Expression
{
public override string One() { return "C"; } public override string Four() { return "CD"; } public override string Five() { return "D"; } public override string Nine() { return "CM"; } public override int Multiplier() { return 100; }
}
class TenExpression : Expression
{
public override string One() { return "X"; } public override string Four() { return "XL"; } public override string Five() { return "L"; } public override string Nine() { return "XC"; } public override int Multiplier() { return 10; }
}
class OneExpression : Expression
{
public override string One() { return "I"; } public override string Four() { return "IV"; } public override string Five() { return "V"; } public override string Nine() { return "IX"; } public override int Multiplier() { return 1; }
}
class OneExpression
{
static void Main(string[] args)
{
string roman = "MCMXXVIII";
Context context = new Context(roman); List<Expression> tree = new List<Expression>();
tree.Add(new ThousandExpression()); tree.Add(new HundredExpression()); tree.Add(new TenExpression()); tree.Add(new OneExpression());
foreach (Expression exp in tree) exp.Interpret(context);
Console.WriteLine("{0} = {1}", roman, context.Output); Console.ReadKey();
}
}

using System;
using System.Collections.Generic; using System.Linq;
using System.Text;
namespace MediatorPattern
{
class Colleague
{
bool isON;
public bool IsON
{
get { return isON; } set {
mediator.Send(this, value); this.isON = value;
}
}
string info;
public string Info
{
get { return info; } set { info = value; }
}
Mediator mediator;