
- •Credits
- •Foreword
- •About the Authors
- •About the Reviewers
- •www.PacktPub.com
- •Table of Contents
- •Preface
- •Introducing SFML
- •Downloading and installation
- •A minimal example
- •A few notes on C++
- •Developing the first game
- •The Game class
- •Game loops and frames
- •Input over several frames
- •Vector algebra
- •Frame-independent movement
- •Fixed time steps
- •Other techniques related to frame rates
- •Displaying sprites on the screen
- •File paths and working directories
- •Real-time rendering
- •Adapting the code
- •Summary
- •Defining resources
- •Resources in SFML
- •Textures
- •Images
- •Fonts
- •Shaders
- •Sound buffers
- •Music
- •A typical use case
- •Graphics
- •Audio
- •Acquiring, releasing, and accessing resources
- •An automated approach
- •Finding an appropriate container
- •Loading from files
- •Accessing the textures
- •Error handling
- •Boolean return values
- •Throwing exceptions
- •Assertions
- •Generalizing the approach
- •Compatibility with sf::Music
- •A special case – sf::Shader
- •Summary
- •Entities
- •Aircraft
- •Alternative entity designs
- •Rendering the scene
- •Relative coordinates
- •SFML and transforms
- •Scene graphs
- •Scene nodes
- •Node insertion and removal
- •Making scene nodes drawable
- •Drawing entities
- •Connecting entities with resources
- •Aligning the origin
- •Scene layers
- •Updating the scene
- •One step back – absolute transforms
- •The view
- •Viewport
- •View optimizations
- •Resolution and aspect ratio
- •View scrolling
- •Zoom and rotation
- •Landscape rendering
- •SpriteNode
- •Landscape texture
- •Texture repeating
- •Composing our world
- •World initialization
- •Loading the textures
- •Building the scene
- •Update and draw
- •Integrating the Game class
- •Summary
- •Polling events
- •Window events
- •Joystick events
- •Keyboard events
- •Mouse events
- •Getting the input state in real time
- •Events and real-time input – when to use which
- •Delta movement from the mouse
- •Playing nice with your application neighborhood
- •A command-based communication system
- •Introducing commands
- •Receiver categories
- •Command execution
- •Command queues
- •Handling player input
- •Commands in a nutshell
- •Implementing the game logic
- •A general-purpose communication mechanism
- •Customizing key bindings
- •Why a player is not an entity
- •Summary
- •Defining a state
- •The state stack
- •Adding states to StateStack
- •Handling updates, input, and drawing
- •Input
- •Update
- •Draw
- •Delayed pop/push operations
- •The state context
- •Integrating the stack in the Application class
- •Navigating between states
- •Creating the game state
- •The title screen
- •Main menu
- •Pausing the game
- •The loading screen – sample
- •Progress bar
- •ParallelTask
- •Thread
- •Concurrency
- •Task implementation
- •Summary
- •The GUI hierarchy, the Java way
- •Updating the menu
- •The promised key bindings
- •Summary
- •Equipping the entities
- •Introducing hitpoints
- •Storing entity attributes in data tables
- •Displaying text
- •Creating enemies
- •Movement patterns
- •Spawning enemies
- •Adding projectiles
- •Firing bullets and missiles
- •Homing missiles
- •Picking up some goodies
- •Collision detection and response
- •Finding the collision pairs
- •Reacting to collisions
- •An outlook on optimizations
- •An interacting world
- •Cleaning everything up
- •Out of view, out of the world
- •The final update
- •Victory and defeat
- •Summary
- •Defining texture atlases
- •Adapting the game code
- •Low-level rendering
- •OpenGL and graphics cards
- •Understanding render targets
- •Texture mapping
- •Vertex arrays
- •Particle systems
- •Particles and particle types
- •Particle nodes
- •Emitter nodes
- •Affectors
- •Embedding particles in the world
- •Animated sprites
- •The Eagle has rolled!
- •Post effects and shaders
- •Fullscreen post effects
- •Shaders
- •The bloom effect
- •Summary
- •Music themes
- •Loading and playing
- •Use case – In-game themes
- •Sound effects
- •Loading, inserting, and playing
- •Removing sounds
- •Use case – GUI sounds
- •Sounds in 3D space
- •The listener
- •Attenuation factor and minimum distance
- •Positioning the listener
- •Playing spatial sounds
- •Use case – In-game sound effects
- •Summary
- •Playing multiplayer games
- •Interacting with sockets
- •Socket selectors
- •Custom protocols
- •Data transport
- •Network architectures
- •Peer-to-peer
- •Client-server architecture
- •Authoritative servers
- •Creating the structure for multiplayer
- •Working with the Server
- •Server thread
- •Server loop
- •Peers and aircraft
- •Hot Seat
- •Accepting new clients
- •Handling disconnections
- •Incoming packets
- •Studying our protocol
- •Understanding the ticks and updates
- •Synchronization issues
- •Taking a peek in the other end – the client
- •Client packets
- •Transmitting game actions via network nodes
- •The new pause state
- •Settings
- •The new Player class
- •Latency
- •Latency versus bandwidth
- •View scrolling compensation
- •Aircraft interpolation
- •Cheating prevention
- •Summary
- •Index
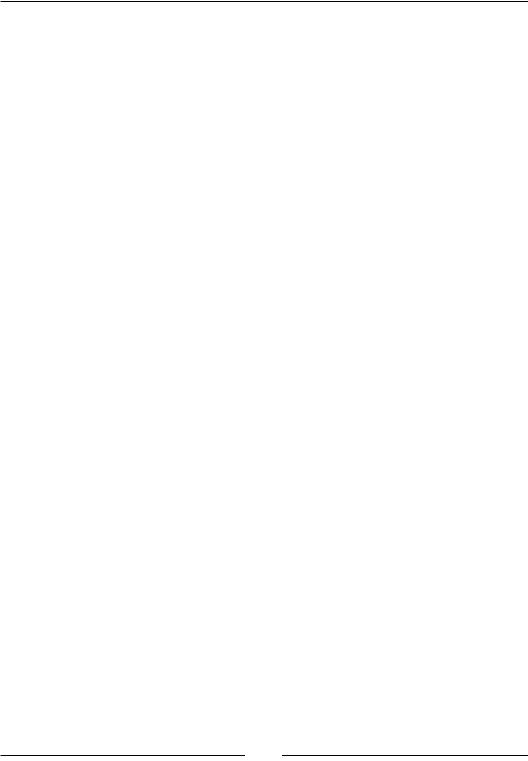
Chapter 5
The state context
In general, every screen will need to display some text or sprites, draw to the screen, among other common things. Due to this fact, and to avoid unnecessary memory wasting by loading the same texture or font to memory in multiple places, we introduced the State::Context structure. It works as a holder of shared objects between all states of our game.
Essentially, every state will now have access to the getContext() method, which itself contains the pointer to the window used to draw its objects and resource holders such as font and texture managers. Here's the declaration of the structure:
struct Context
{
|
Context(sf::RenderWindow& window, |
|
TextureHolder& textures, |
|
FontHolder& fonts, |
|
Player& player); |
sf::RenderWindow* |
window; |
TextureHolder* |
textures; |
FontHolder* |
fonts; |
Player* |
player; |
}; |
|
The usefulness of the state context is undeniable. It will save system memory by reusing the same fonts and textures for every state. It will also provide access to the window's view at all times, which is a necessity when positioning and resizing our objects relatively to the view's dimensions.
Integrating the stack in the Application class
Since we have now more states than the game itself, we create a new class Application that controls input, logic updates, and rendering. Having a ready StateStack implementation waiting to be used, it is time to promote it into the Application class. We will plug our new state architecture into our Application class and then start using it!
[ 121 ]
www.it-ebooks.info
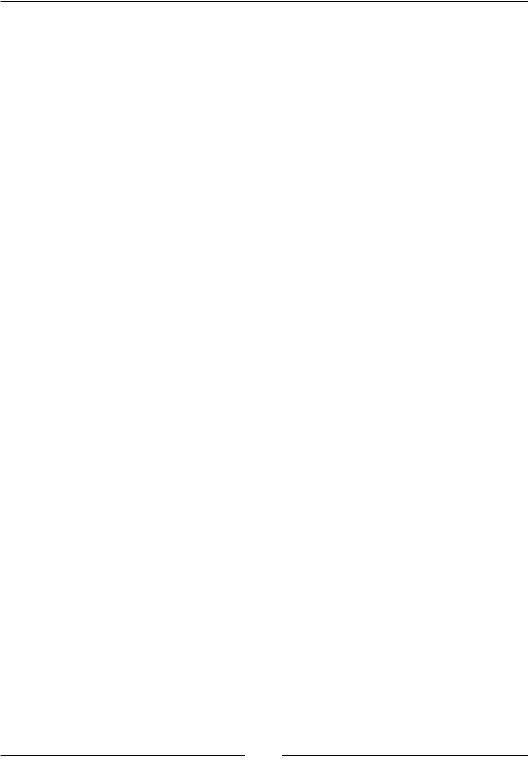
Diverting the Game Flow – State Stack
First, we add the mStateStack member variable to Application. We register all the states in an own method:
void Application::registerStates()
{
mStateStack.registerState<TitleState>(States::Title);
mStateStack.registerState<MenuState>(States::Menu);
mStateStack.registerState<GameState>(States::Game);
mStateStack.registerState<PauseState>(States::Pause);
}
Now there are a few more things we must care about for a full integration of our state architecture:
•Feeding it with events in the Application::processInput() function:
while (mWindow.pollEvent(event))
{
mStateStack.handleEvent(event);
}
•Updating with the elapsed time:
void Application::update(sf::Time dt)
{
mStateStack.update(dt);
}
•Rendering of the stack, in the middle of the frame draw:
mStateStack.draw();
•Closing the game when no more states are left:
if (mStateStack.isEmpty()) mWindow.close();
And now that everything is plugged in and ready to go, in the end of our constructor we make the machine start with the title screen!
mStateStack.pushState(States::Title);
[ 122 ]
www.it-ebooks.info