
- •Table of Contents
- •Chapter 1. Why Shell Programming?
- •2.1. Invoking the script
- •2.2. Preliminary Exercises
- •Part 2. Basics
- •Chapter 3. Exit and Exit Status
- •Chapter 4. Special Characters
- •Chapter 5. Introduction to Variables and Parameters
- •5.1. Variable Substitution
- •5.2. Variable Assignment
- •5.3. Bash Variables Are Untyped
- •5.4. Special Variable Types
- •Chapter 6. Quoting
- •Chapter 7. Tests
- •7.1. Test Constructs
- •7.2. File test operators
- •7.3. Comparison operators (binary)
- •7.4. Nested if/then Condition Tests
- •7.5. Testing Your Knowledge of Tests
- •Chapter 8. Operations and Related Topics
- •8.1. Operators
- •8.2. Numerical Constants
- •Part 3. Beyond the Basics
- •Chapter 9. Variables Revisited
- •9.1. Internal Variables
- •9.2. Manipulating Strings
- •9.2.1. Manipulating strings using awk
- •9.2.2. Further Discussion
- •9.3. Parameter Substitution
- •9.4. Typing variables: declare or typeset
- •9.5. Indirect References to Variables
- •9.6. $RANDOM: generate random integer
- •9.7. The Double Parentheses Construct
- •Chapter 10. Loops and Branches
- •10.1. Loops
- •10.2. Nested Loops
- •10.3. Loop Control
- •10.4. Testing and Branching
- •Chapter 11. Internal Commands and Builtins
- •11.1. Job Control Commands
- •Chapter 12. External Filters, Programs and Commands
- •12.1. Basic Commands
- •12.2. Complex Commands
- •12.3. Time / Date Commands
- •12.4. Text Processing Commands
- •12.5. File and Archiving Commands
- •12.6. Communications Commands
- •12.7. Terminal Control Commands
- •12.8. Math Commands
- •12.9. Miscellaneous Commands
- •Chapter 13. System and Administrative Commands
- •Chapter 14. Command Substitution
- •Chapter 15. Arithmetic Expansion
- •Chapter 16. I/O Redirection
- •16.1. Using exec
- •16.2. Redirecting Code Blocks
- •16.3. Applications
- •Chapter 17. Here Documents
- •Chapter 18. Recess Time
- •Part 4. Advanced Topics
- •Chapter 19. Regular Expressions
- •19.1. A Brief Introduction to Regular Expressions
- •19.2. Globbing
- •Chapter 20. Subshells
- •Chapter 21. Restricted Shells
- •Chapter 22. Process Substitution
- •Chapter 23. Functions
- •23.1. Complex Functions and Function Complexities
- •23.2. Local Variables
- •23.2.1. Local variables make recursion possible.
- •Chapter 24. Aliases
- •Chapter 25. List Constructs
- •Chapter 26. Arrays
- •Chapter 27. Files
- •Chapter 28. /dev and /proc
- •28.2. /proc
- •Chapter 29. Of Zeros and Nulls
- •Chapter 30. Debugging
- •Chapter 31. Options
- •Chapter 32. Gotchas
- •Chapter 33. Scripting With Style
- •33.1. Unofficial Shell Scripting Stylesheet
- •Chapter 34. Miscellany
- •34.2. Shell Wrappers
- •34.3. Tests and Comparisons: Alternatives
- •34.4. Optimizations
- •34.5. Assorted Tips
- •34.6. Oddities
- •34.7. Portability Issues
- •34.8. Shell Scripting Under Windows
- •Chapter 35. Bash, version 2
- •Chapter 36. Endnotes
- •36.1. Author's Note
- •36.2. About the Author
- •36.3. Tools Used to Produce This Book
- •36.3.1. Hardware
- •36.3.2. Software and Printware
- •36.4. Credits
- •Bibliography
- •Appendix A. Contributed Scripts
- •Appendix C. Exit Codes With Special Meanings
- •Appendix D. A Detailed Introduction to I/O and I/O Redirection
- •Appendix E. Localization
- •Appendix F. History Commands
- •Appendix G. A Sample .bashrc File
- •Appendix H. Converting DOS Batch Files to Shell Scripts
- •Appendix I. Exercises
- •Appendix J. Copyright
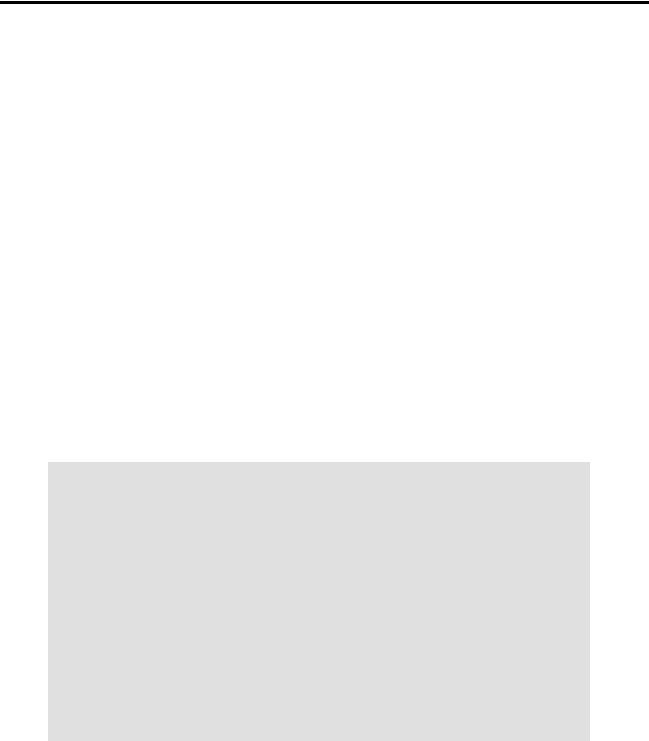
Chapter 5. Introduction to Variables and Parameters
Variables are at the heart of every programming and scripting language. They appear in arithmetic operations and manipulation of quantities, string parsing, and are indispensable for working in the abstract with symbols − tokens that represent something else. A variable is nothing more than a location or set of locations in computer memory holding an item of data.
5.1. Variable Substitution
The name of a variable is a placeholder for its value, the data it holds. Referencing its value is called variable substitution.
$
Let us carefully distinguish between the name of a variable |
and its value. If variable1 is the name |
of a variable, then $variable1 is a reference to its value, |
the data item it contains. The only time a |
variable appears "naked", without the $ prefix, is when declared or assigned, when unset, when exported, or in the special case of a variable representing a signal (see Example 30−4). Assignment may be with an = (as in var1=27), in a read statement, and at the head of a loop (for var2 in 1 2 3).
Enclosing a referenced value in double quotes (" ") does not interfere with variable substitution. This is called partial quoting, sometimes referred to as "weak quoting". Using single quotes (' ') causes the variable name to be used literally, and no substitution will take place. This is full quoting, sometimes referred to as "strong quoting". See Chapter 6 for a detailed discussion.
Note that $variable is actually a simplified alternate form of ${variable}. In contexts where the $variable syntax causes an error, the longer form may work (see Section 9.3, below).
Example 5−1. Variable assignment and substitution
#!/bin/bash
# Variables: assignment and substitution
a=375 hello=$a
#−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−
#No space permitted on either side of = sign when initializing variables.
#If "VARIABLE =value",
#+ script tries to run "VARIABLE" command with one argument, "=value".
#If "VARIABLE= value",
#+ script tries to run "value" command with
#+ the environmental variable "VARIABLE" set to "". #−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−
Chapter 5. Introduction to Variables and Parameters |
23 |
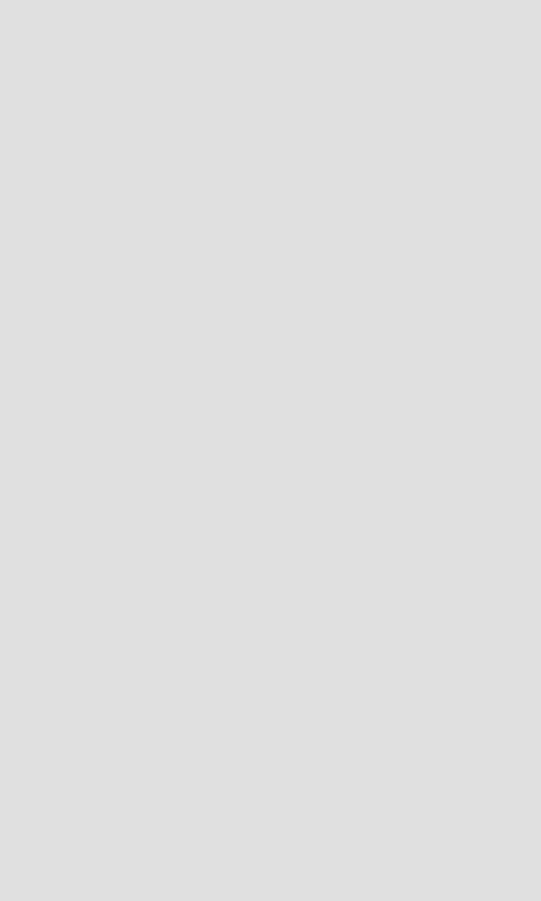
Advanced Bash−Scripting Guide
echo hello |
# Not a variable reference, just the string "hello". |
echo $hello
echo ${hello} #Identical to above.
echo "$hello" echo "${hello}"
echo
hello="A B C D" echo $hello
echo "$hello"
# Now, echo $hello and echo "$hello" give different results.
# Quoting a variable preserves whitespace.
echo
echo '$hello'
#Variable referencing disabled by single quotes, #+ which causes the "$" to be interpreted literally.
#Notice the effect of different types of quoting.
hello= |
# Setting |
it to a null value. |
echo "\$hello (null |
value) = $hello" |
#Note that setting a variable to a null value is not the same as #+ unsetting it, although the end result is the same (see below).
#−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−
#It is permissible to set multiple variables on the same line, #+ if separated by white space.
#Caution, this may reduce legibility, and may not be portable.
var1=variable1 var2=variable2 var3=variable3 echo
echo "var1=$var1 var2=$var2 var3=$var3"
#May cause problems with older versions of "sh".
#−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−−
echo; echo
numbers="one two three" other_numbers="1 2 3"
# If whitespace within a variable, then quotes necessary. echo "numbers = $numbers"
echo "other_numbers = $other_numbers" echo
echo "uninitialized_variable = $uninitialized_variable"
# Uninitialized variable has null value (no value at all). uninitialized_variable= # Declaring, but not initializing it
#+ (same as setting it to a null value, as above). echo "uninitialized_variable = $uninitialized_variable"
# It still has a null value.
uninitialized_variable=23 |
# |
Set it. |
unset uninitialized_variable |
# |
Unset it. |
Chapter 5. Introduction to Variables and Parameters |
24 |
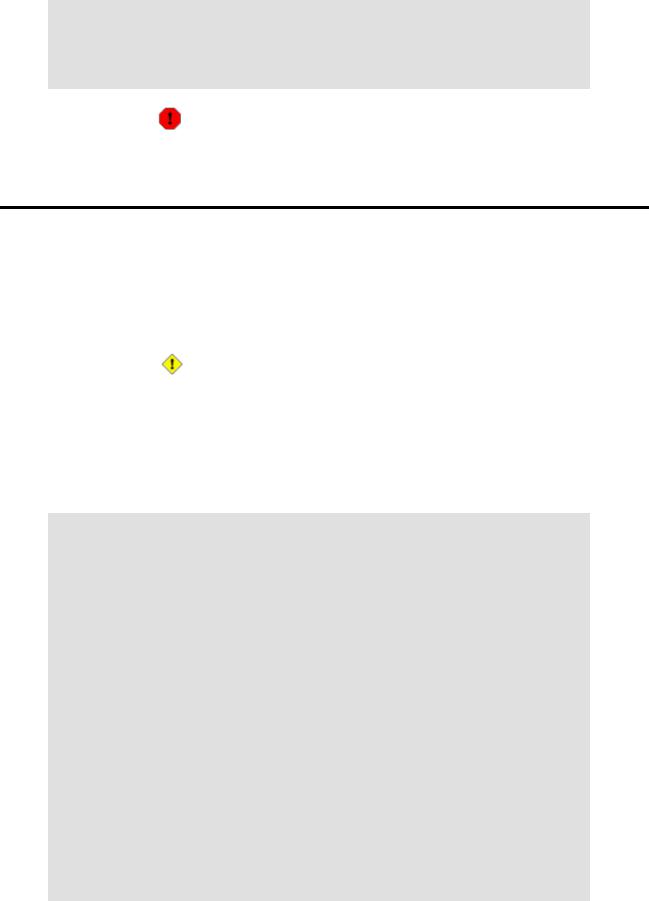
Advanced Bash−Scripting Guide
echo "uninitialized_variable = $uninitialized_variable"
# It still has a null value.
echo
exit 0
An uninitialized variable has a "null" value − no assigned value at all (not zero!). Using a variable before assigning a value to it will inevitably cause problems.
5.2. Variable Assignment
=
the assignment operator (no space before & after)
Do not confuse this with = and −eq, which test, rather than assign!
Note that = can be either an assignment or a test operator, depending on context.
Example 5−2. Plain Variable Assignment
#!/bin/bash
echo
#When is a variable "naked", i.e., lacking the '$' in front?
#When it is being assigned, rather than referenced.
#Assignment
a=879
echo "The value of \"a\" is $a"
# Assignment using 'let' let a=16+5
echo "The value of \"a\" is now $a"
echo
# In a 'for' loop (really, a type of disguised assignment) echo −n "The values of \"a\" in the loop are "
for a in 7 8 9 11 do
echo −n "$a " done
echo echo
5.2. Variable Assignment |
25 |
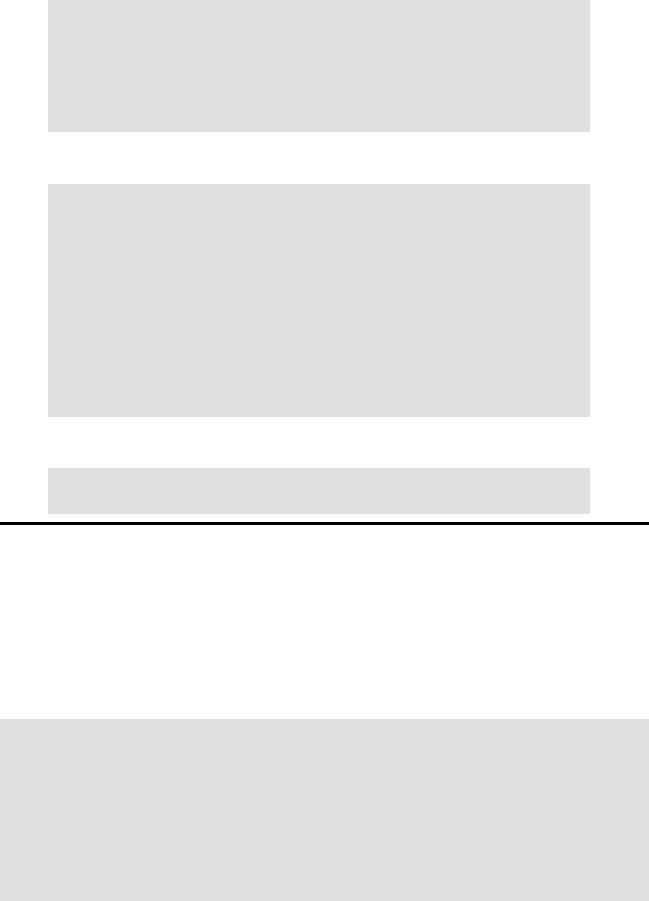
Advanced Bash−Scripting Guide
# In a 'read' statement (also a type of assignment) echo −n "Enter \"a\" "
read a
echo "The value of \"a\" is now $a"
echo
exit 0
Example 5−3. Variable Assignment, plain and fancy
#!/bin/bash |
|
a=23 |
# Simple case |
echo $a |
|
b=$a |
|
echo $b |
|
# Now, getting a little bit fancier...
a=`echo Hello!` |
# Assigns |
result of |
'echo' command to 'a' |
echo $a |
|
|
|
a=`ls −l` |
# Assigns |
result of |
'ls −l' command to 'a' |
echo $a |
|
|
|
exit 0 |
|
|
|
Variable assignment using the $(...) mechanism (a newer method than backquotes)
# From /etc/rc.d/rc.local R=$(cat /etc/redhat−release) arch=$(uname −m)
5.3. Bash Variables Are Untyped
Unlike many other programming languages, Bash does not segregate its variables by "type". Essentially, Bash variables are character strings, but, depending on context, Bash permits integer operations and comparisons on variables. The determining factor is whether the value of a variable contains only digits.
Example 5−4. Integer or string?
#!/bin/bash
# int−or−string.sh
# Integer or string?
a=2334 |
# Integer. |
let "a += 1" |
|
echo "a = $a " |
# Integer, still. |
echo |
|
b=${a/23/BB} |
# Transform into a string. |
echo "b = $b" |
# BB35 |
declare −i b |
# Declaring it an integer doesn't help. |
5.3. Bash Variables Are Untyped |
26 |