
- •Table of Contents
- •Chapter 1. Why Shell Programming?
- •2.1. Invoking the script
- •2.2. Preliminary Exercises
- •Part 2. Basics
- •Chapter 3. Exit and Exit Status
- •Chapter 4. Special Characters
- •Chapter 5. Introduction to Variables and Parameters
- •5.1. Variable Substitution
- •5.2. Variable Assignment
- •5.3. Bash Variables Are Untyped
- •5.4. Special Variable Types
- •Chapter 6. Quoting
- •Chapter 7. Tests
- •7.1. Test Constructs
- •7.2. File test operators
- •7.3. Comparison operators (binary)
- •7.4. Nested if/then Condition Tests
- •7.5. Testing Your Knowledge of Tests
- •Chapter 8. Operations and Related Topics
- •8.1. Operators
- •8.2. Numerical Constants
- •Part 3. Beyond the Basics
- •Chapter 9. Variables Revisited
- •9.1. Internal Variables
- •9.2. Manipulating Strings
- •9.2.1. Manipulating strings using awk
- •9.2.2. Further Discussion
- •9.3. Parameter Substitution
- •9.4. Typing variables: declare or typeset
- •9.5. Indirect References to Variables
- •9.6. $RANDOM: generate random integer
- •9.7. The Double Parentheses Construct
- •Chapter 10. Loops and Branches
- •10.1. Loops
- •10.2. Nested Loops
- •10.3. Loop Control
- •10.4. Testing and Branching
- •Chapter 11. Internal Commands and Builtins
- •11.1. Job Control Commands
- •Chapter 12. External Filters, Programs and Commands
- •12.1. Basic Commands
- •12.2. Complex Commands
- •12.3. Time / Date Commands
- •12.4. Text Processing Commands
- •12.5. File and Archiving Commands
- •12.6. Communications Commands
- •12.7. Terminal Control Commands
- •12.8. Math Commands
- •12.9. Miscellaneous Commands
- •Chapter 13. System and Administrative Commands
- •Chapter 14. Command Substitution
- •Chapter 15. Arithmetic Expansion
- •Chapter 16. I/O Redirection
- •16.1. Using exec
- •16.2. Redirecting Code Blocks
- •16.3. Applications
- •Chapter 17. Here Documents
- •Chapter 18. Recess Time
- •Part 4. Advanced Topics
- •Chapter 19. Regular Expressions
- •19.1. A Brief Introduction to Regular Expressions
- •19.2. Globbing
- •Chapter 20. Subshells
- •Chapter 21. Restricted Shells
- •Chapter 22. Process Substitution
- •Chapter 23. Functions
- •23.1. Complex Functions and Function Complexities
- •23.2. Local Variables
- •23.2.1. Local variables make recursion possible.
- •Chapter 24. Aliases
- •Chapter 25. List Constructs
- •Chapter 26. Arrays
- •Chapter 27. Files
- •Chapter 28. /dev and /proc
- •28.2. /proc
- •Chapter 29. Of Zeros and Nulls
- •Chapter 30. Debugging
- •Chapter 31. Options
- •Chapter 32. Gotchas
- •Chapter 33. Scripting With Style
- •33.1. Unofficial Shell Scripting Stylesheet
- •Chapter 34. Miscellany
- •34.2. Shell Wrappers
- •34.3. Tests and Comparisons: Alternatives
- •34.4. Optimizations
- •34.5. Assorted Tips
- •34.6. Oddities
- •34.7. Portability Issues
- •34.8. Shell Scripting Under Windows
- •Chapter 35. Bash, version 2
- •Chapter 36. Endnotes
- •36.1. Author's Note
- •36.2. About the Author
- •36.3. Tools Used to Produce This Book
- •36.3.1. Hardware
- •36.3.2. Software and Printware
- •36.4. Credits
- •Bibliography
- •Appendix A. Contributed Scripts
- •Appendix C. Exit Codes With Special Meanings
- •Appendix D. A Detailed Introduction to I/O and I/O Redirection
- •Appendix E. Localization
- •Appendix F. History Commands
- •Appendix G. A Sample .bashrc File
- •Appendix H. Converting DOS Batch Files to Shell Scripts
- •Appendix I. Exercises
- •Appendix J. Copyright
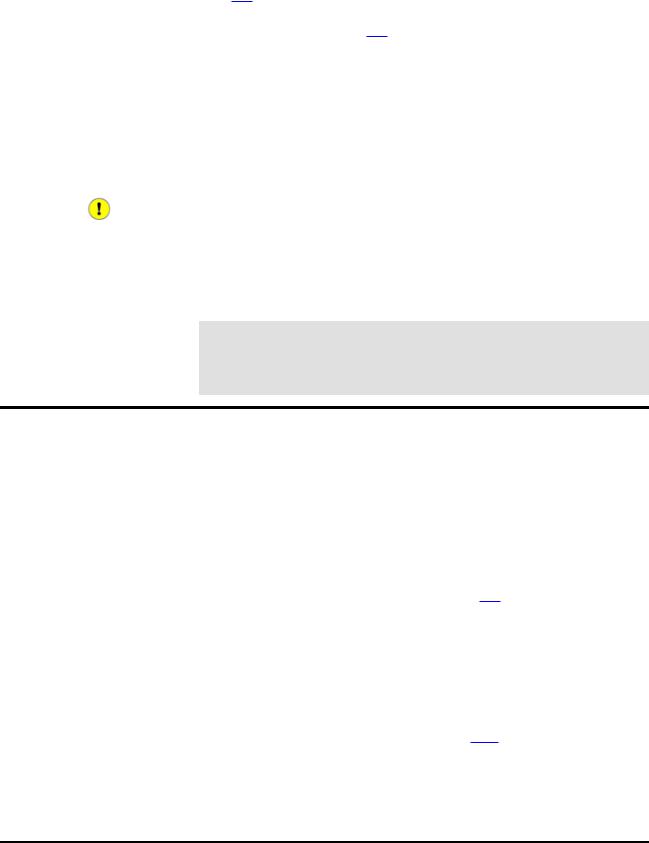
Advanced Bash−Scripting Guide
Each of the above script header lines calls a different command interpreter, be it /bin/sh, the default shell (bash in a Linux system) or otherwise. [6] Using #!/bin/sh, the default Bourne Shell in most commercial variants of UNIX, makes the script portable to non−Linux machines, though you may have to sacrifice a few Bash−specific features (the script will conform to the POSIX [7] sh standard).
Note that the path given at the "sha−bang" must be correct, otherwise an error message, usually "Command not found" will be the only result of running the script.
#! can be omitted if the script consists only of a set of generic system commands, using no internal shell directives. Example 2, above, requires the initial #!, since the variable assignment line, lines=50, uses a shell−specific construct. Note that #!/bin/sh invokes the default shell interpreter, which defaults to /bin/bash on a Linux machine.
This tutorial encourages a modular approach to constructing a script. Make note of and collect "boilerplate" code snippets that might be useful in future scripts. Eventually you can build a quite extensive library of nifty routines. As an example, the following script prolog tests whether the script has been invoked with the correct number of parameters.
if [ $# −ne Number_of_expected args ] then
echo "Usage: `basename $0` whatever" exit $WRONG_ARGS
fi
2.1. Invoking the script
Having written the script, you can invoke it by sh |
scriptname, [8] or alternately bash scriptname. |
||
|
|
|
|
(Not recommended is using sh <scriptname, |
since this effectively disables reading from stdin within |
the script.) Much more convenient is to make the script itself directly executable with a chmod.
Either:
chmod 555 scriptname (gives everyone read/execute permission) [9]
or
chmod +rx scriptname (gives everyone read/execute permission)
chmod u+rx scriptname (gives only the script owner read/execute permission)
Having made the script executable, you may now test it by ./scriptname. [10] If it begins with a "sha−bang" line, invoking the script calls the correct command interpreter to run it.
As a final step, after testing and debugging, you would likely want to move it to /usr/local/bin (as root, of course), to make the script available to yourself and all other users as a system−wide executable. The script could then be invoked by simply typing scriptname [ENTER] from the command line.
2.1. Invoking the script |
5 |

Advanced Bash−Scripting Guide
2.2.Preliminary Exercises
1.System administrators often write scripts to automate common tasks. Give instances where such scripts would be useful.
2.Write a script that upon invocation shows the time and date, lists all logged−in users, and gives the system uptime. The script then saves this information to a logfile.
Part 2. Basics
Table of Contents
3.Exit and Exit Status
4.Special Characters
5.Introduction to Variables and Parameters
5.1.Variable Substitution
5.2.Variable Assignment
5.3.Bash Variables Are Untyped
5.4.Special Variable Types
6.Quoting
7.Tests
7.1.Test Constructs
7.2.File test operators
7.3.Comparison operators (binary)
7.4.Nested if/then Condition Tests
7.5.Testing Your Knowledge of Tests
8.Operations and Related Topics
8.1.Operators
8.2.Numerical Constants
2.2. Preliminary Exercises |
6 |