
- •About the Author
- •Dedication
- •Author’s Acknowledgments
- •Contents at a Glance
- •Table of Contents
- •Introduction
- •Who Should Buy This Book
- •How This Book Is Organized
- •Part I: Programming a Computer
- •Part II: Learning Programming with Liberty BASIC
- •Part III: Advanced Programming with Liberty BASIC
- •Part VI: Internet Programming
- •Part VII: The Part of Tens
- •How to Use This Book
- •Foolish assumptions
- •Icons used in this book
- •Why Learn Computer Programming?
- •How Does a Computer Program Work?
- •What Do I Need to Know to Program a Computer?
- •The joy of assembly language
- •C: The portable assembler
- •High-level programming languages
- •Database programming languages
- •Scripting programming languages
- •The program’s users
- •The target computer
- •Prototyping
- •Choosing a programming language
- •Defining how the program should work
- •The Life Cycle of a Typical Program
- •The development cycle
- •The maintenance cycle
- •The upgrade cycle
- •Writing Programs in an Editor
- •Using a Compiler or an Interpreter
- •Compilers
- •Interpreters
- •P-code: A combination compiler and interpreter
- •So what do I use?
- •Squashing Bugs with a Debugger
- •Writing a Help File
- •Creating an Installation Program
- •Why Learn Liberty BASIC?
- •Liberty BASIC is easy
- •Liberty BASIC runs on Windows
- •You can start using Liberty BASIC today
- •Installing Liberty BASIC
- •Loading Liberty BASIC
- •Your First Liberty BASIC Program
- •Running a Liberty BASIC program
- •Saving a Liberty BASIC program
- •Getting Help Using Liberty BASIC
- •Exiting Liberty BASIC
- •Getting input
- •Displaying output
- •Sending Data to the Printer
- •Storing Data in Variables
- •Creating a variable
- •Assigning a value to a variable
- •Declaring your variables
- •Using Constants
- •Commenting Your Code
- •Using variables
- •Working with precedence
- •Using parentheses
- •Manipulating Strings
- •Declaring variables as strings
- •Smashing strings together
- •Counting the length of a string
- •Playing with UPPERCASE and lowercase
- •Trimming the front and back of a string
- •Inserting spaces
- •Yanking characters out of a string
- •Looking for a string inside another string
- •Using Boolean Expressions
- •Using variables in Boolean expressions
- •Using Boolean operators
- •Exploring IF THEN Statements
- •IF THEN ELSE statements
- •Working with SELECT CASE Statements
- •Checking a range of values
- •Checking a relational operator
- •Boolean expression inside the loop
- •Looping a Fixed Number of Times
- •Counting with different numbers
- •Counting in increments
- •Anatomy of a Computer Bug
- •Syntax Errors
- •Fun with Logic Errors
- •Stepping line by line
- •Tracing through your program
- •Designing a Window
- •Creating a new window
- •Defining the size and location of a window
- •Adding color to a window
- •Putting Controls in a Window
- •Creating a command button
- •Displaying text
- •Creating a check box
- •Creating a radio button
- •Creating text boxes
- •Creating list boxes
- •Creating combo boxes
- •Creating group boxes
- •Storing Stuff in Text Files
- •Creating a new text file
- •Putting stuff in a text file
- •Adding new stuff to an existing text file
- •Retrieving data from a text file
- •Creating a new binary file
- •Saving stuff in a binary file
- •Changing stuff in a binary file
- •Retrieving stuff from a binary file
- •Creating a Graphics Control
- •Using Turtle Graphics
- •Defining line thickness
- •Defining line colors
- •Drawing Circles
- •Drawing Boxes
- •Displaying Text
- •Making Sounds
- •Making a beeping noise
- •Playing WAV files
- •Passing Data by Value or by Reference
- •Using Functions
- •Defining a function
- •Passing data to a function
- •Calling a function
- •Exiting prematurely from a function
- •Using Subroutines
- •Defining a subroutine
- •Passing data to a subroutine
- •Calling a subroutine
- •Exiting prematurely from a subroutine
- •Writing Modular Programs
- •Introducing Structured Programming
- •Sequential instructions
- •Branching instructions
- •Looping instructions
- •Putting structured programming into practice
- •The Problem with Software
- •Ways to Make Programming Easier
- •Breaking Programs into Objects
- •How to use objects
- •How to create an object
- •Creating an object
- •Starting with a Pointer
- •Defining the parts of a linked list
- •Creating a linked list
- •Managing a linked list
- •Making Data Structures with Linked Lists
- •Stacks
- •Queues
- •Trees
- •Graphs
- •Creating a Record
- •Manipulating Data in Records
- •Storing data in a record
- •Retrieving data from a record
- •Using Records with Arrays
- •Making an Array
- •Making a Multidimensional Array
- •Creating Dynamic Arrays
- •Insertion Sort
- •Bubble Sort
- •Shell Sort
- •Quicksort
- •Sorting Algorithms
- •Searching Sequentially
- •Performing a Binary Search
- •Hashing
- •Searching by using a hash function
- •Dealing with collisions
- •Picking a Searching Algorithm
- •Choosing the Right Data Structure
- •Choosing the Right Algorithm
- •Put the condition most likely to be false first
- •Put the condition most likely to be true first
- •Clean out your loops
- •Use the correct data types
- •Using a Faster Language
- •Optimizing Your Compiler
- •Programming Computer Games
- •Creating Computer Animation
- •Making (And Breaking) Encryption
- •Internet Programming
- •Fighting Computer Viruses and Worms
- •Hacking for Hire
- •Participating in an Open-Source Project
- •Niche-Market Programming
- •Teaching Others about Computers
- •Selling Your Own Software
- •Trying Commercial Compilers
- •Windows programming
- •Macintosh and Palm OS programming
- •Linux programming
- •Testing the Shareware and
- •BASIC compilers
- •C/C++ and Java compilers
- •Pascal compilers
- •Using a Proprietary Language
- •HyperCard
- •Revolution
- •PowerBuilder
- •Shopping by Mail Order
- •Getting Your Hands on Source Code
- •Joining a Local User Group
- •Frequenting Usenet Newsgroups
- •Playing Core War
- •Programming a Battling Robot
- •Toying with Lego Mindstorms
- •Index
- •End-User License Agreement
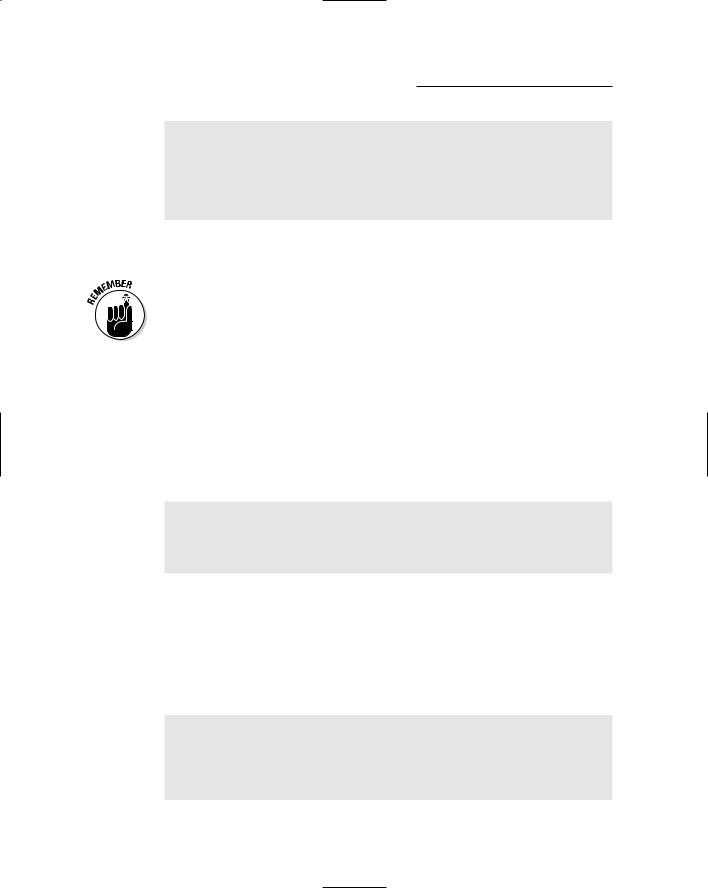
132 Part II: Learning Programming with Liberty BASIC
I = 1
WHILE I <= 10
PRINT “This is I = “; I
IF I = 5 THEN EXIT WHILE
I = I + 1
WEND
END
Normally this WHILE-WEND loop would repeat ten (10) times but the IF I = 5 THEN EXIT WHILE command tells the program to stop after the program has looped only five (5) times.
The EXIT WHILE command can be useful to make sure a WHILE-WEND loop eventually stops running, but make sure that you don’t exit out of a loop sooner than you really want to.
Endless loops #1: Failing to modify the
Boolean expression inside the loop
One of the trickiest parts of using a loop is avoiding an endless loop. An endless loop occurs whenever the Boolean expression of a loop never changes to tell the computer to stop running the instructions inside the loop as in the following program:
I = 1
WHILE I < 5
PRINT “This loop never ends.”
WEND
END
This loop never stops because the value of I never changes. Thus the Boolean expression I < 5 always remains true, so the WHILE-WEND loop keeps running the instructions between the WHILE and WEND commands. In this case, the program just keeps printing, This loop never ends.
To fix this problem, you need to insert a command inside the WHILE-WEND loop that changes the variable in the Boolean expression such as inserting the command I = I + 1, as shown in the following example:
I = 1
WHILE I < 5
PRINT “This loop eventually ends.”
I = I + 1
WEND
END
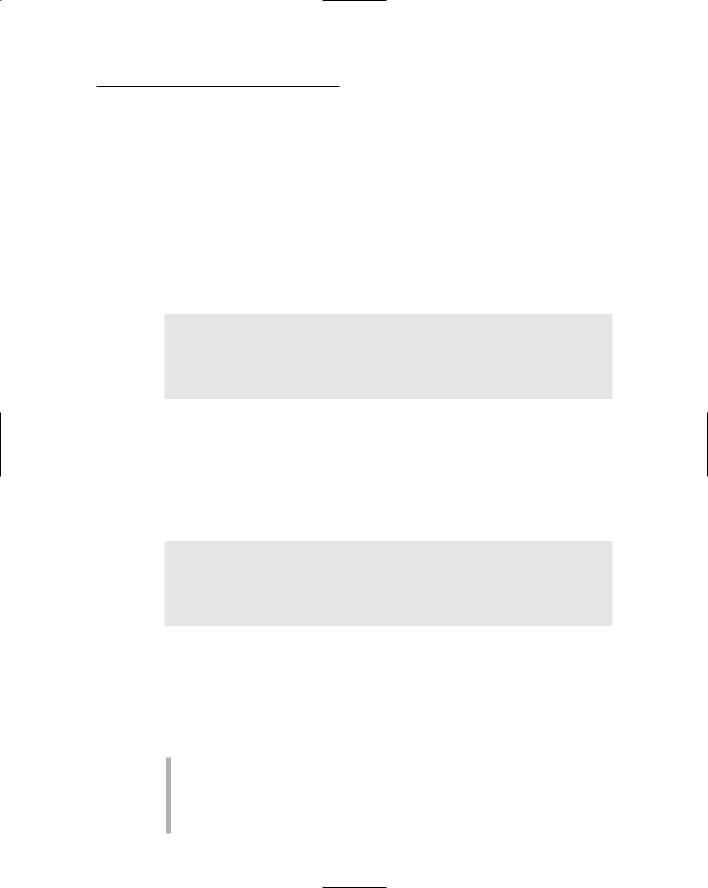
Chapter 10: Repeating Yourself with Loops 133
Endless loops are a common bug that keeps a program from working correctly. You can often recognize an endless loop if a program never responds to anything you try to do and you can’t do anything except shut your computer off and start all over again.
Endless loops #2: Failing to initialize a Boolean expression outside the loop
Another variation of the endless loop occurs if you forget to define the value of a variable outside the WHILE-WEND loop, as in the following program:
WHILE I < 5
I = 1
PRINT “This loop never ends.”
I = I + 1
WEND
END
In this example, the value of variable I is always set to one (1) at the beginning of the WHILE-WEND loop and then gets incremented to two (2) at the end of the WHILE-WEND loop. But each time the WHILE-WEND loop runs, the value of I is reset to one (1) so the Boolean expression I < 5 always remains true, and thus the loop never ends.
The correct way to use the preceding WHILE-WEND loop is to place the statement I = 1 right in front of the WHILE-WEND loop, as in the following example:
I = 1
WHILE I < 5
PRINT “This loop will eventually end.”
I = I + 1
WEND
END
In this example, the value of the I variable is set to one (1) before the computer runs the WHILE-WEND loop. Once inside the WHILE-WEND loop, the value of I steadily increases until the value of I is equal to 5, which makes the Boolean expression I < 5 false; therefore, the WHILE-WEND loop ends.
To avoid endless loops in your program, always remember the following two points:
Initialize all variables that make up a Boolean expression right before a WHILE-WEND loop. This makes sure that your variable starts out with a value that you intended.
Make sure that the variable of the Boolean expression changes inside the WHILE-WEND loop.This makes sure that your loop eventually ends.