
Beginning ActionScript 2.0 2006
.pdf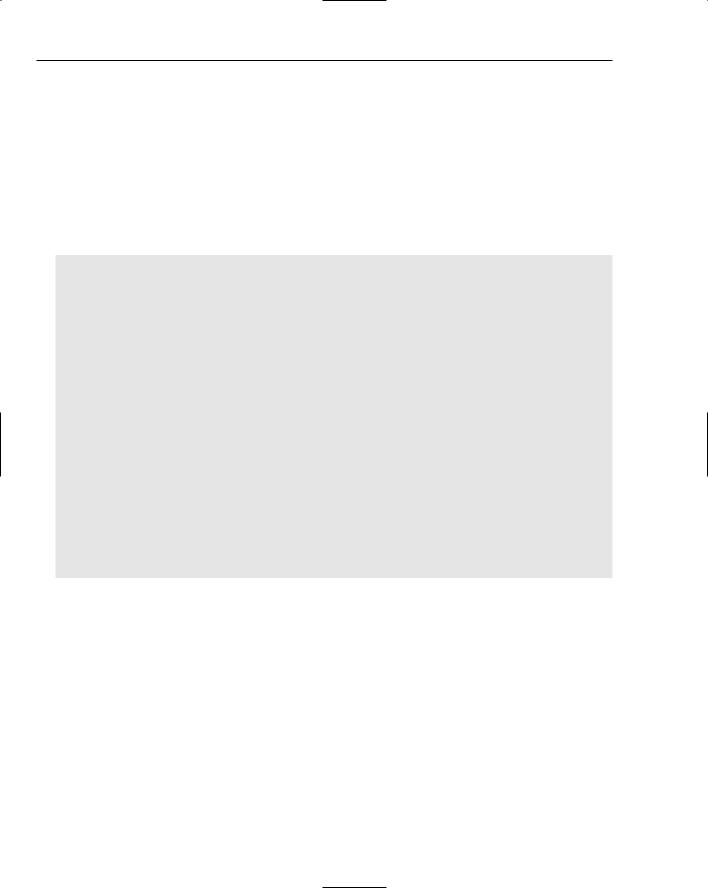


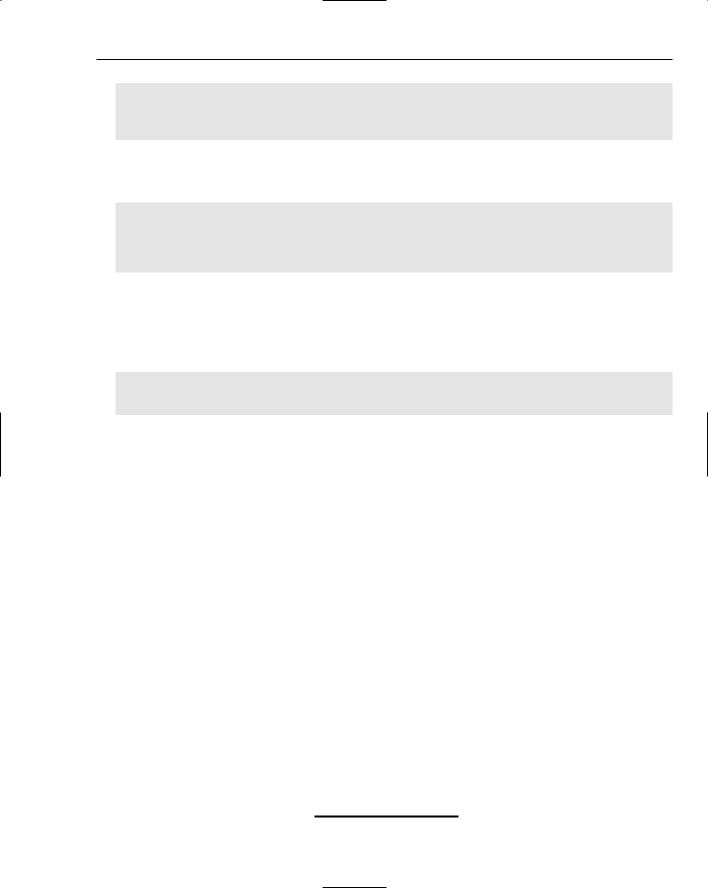
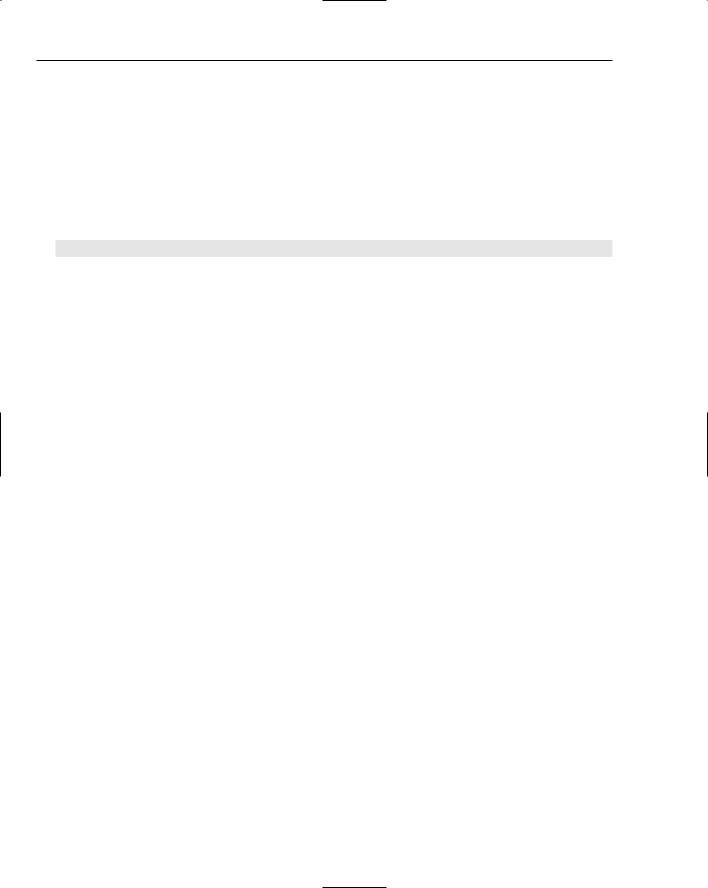



15
Working Directly with Bitmap Data
A bitmap image is a graphic that is created using a grid. The grid is a set of pixels, each with a defined color. When you zoom in on a bitmap image, the grid is obvious. Zooming in on a bitmap causes the pixilated look you’ve probably seen on resized or low-resolution images. Bitmap images are different from vector images in this way. A vector image is defined by points. As a vector image is scaled, the points remain in the same relative position. Lines and fills are drawn between these points as the vector is scaled. Because the lines and fills are redrawn, no pixilation occurs. When vector images are converted to bitmaps based on their current scale within a specified pixel area, they are referred to as rasterized graphics.
Before Flash 8, Flash could not directly manipulate bitmap graphics at the pixel level. Flash has always been able to display bitmap images and modify them as a movieClip object, but ActionScript was never able to fetch the pixel information and work with the pixels directly.
Flash 8 represents a massive maturation of the Flash player capability. Flash can declare anything a Bitmap object. That is, even a vector image can be rasterized in a split millisecond to perform perpixel operation changes at runtime. You can even convert a movie clip to a bitmap so quickly that you can rasterize vector animations, videos, webcam feeds, and more. You also can work with their pixels and apply filters to them as they play, giving you unparalleled freedom in terms of design and implementation.
After you convert a movie clip to a bitmap, you can apply a multitude of effects. In fact, all of the filters described earlier in this book that were applied to movie clips used the Bitmap object to perform the effects. The filters were able to modify the image as a bitmap as needed. Sometimes you can be working with bitmaps in Flash 8 and not even realize it.
The main ingredient when working with bitmap data is the Bitmap object, which is accessed via the display class package. Simply converting a movie clip to a bitmap can improve the frame rate performance of some animation conditions. However, the Bitmap object has a lot more to offer. Robust method and property sets enable you to work with bitmaps directly as well as augment filters, movie clip drawing methods, animations, and more.
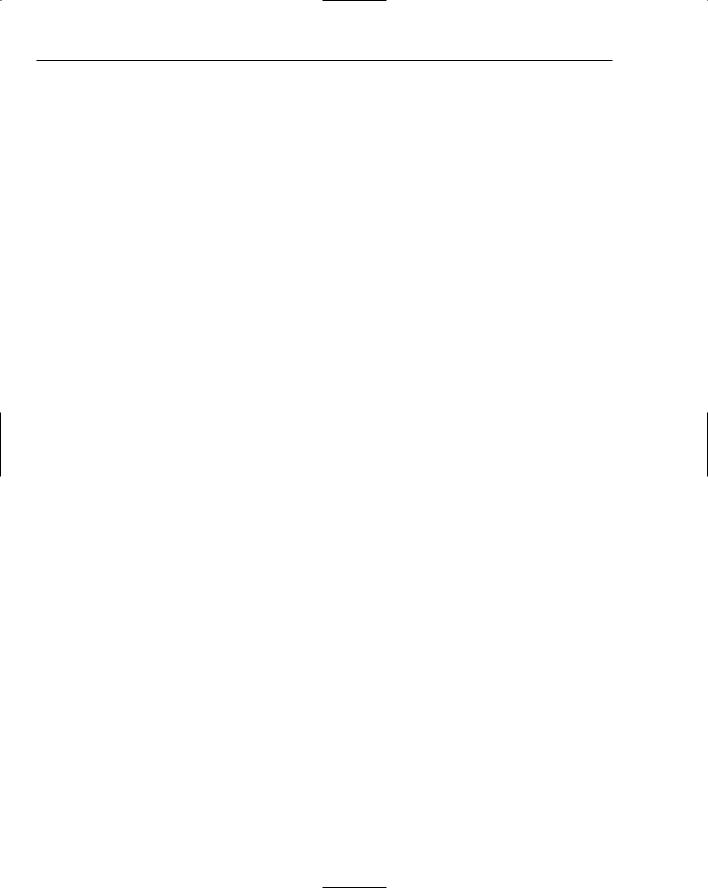
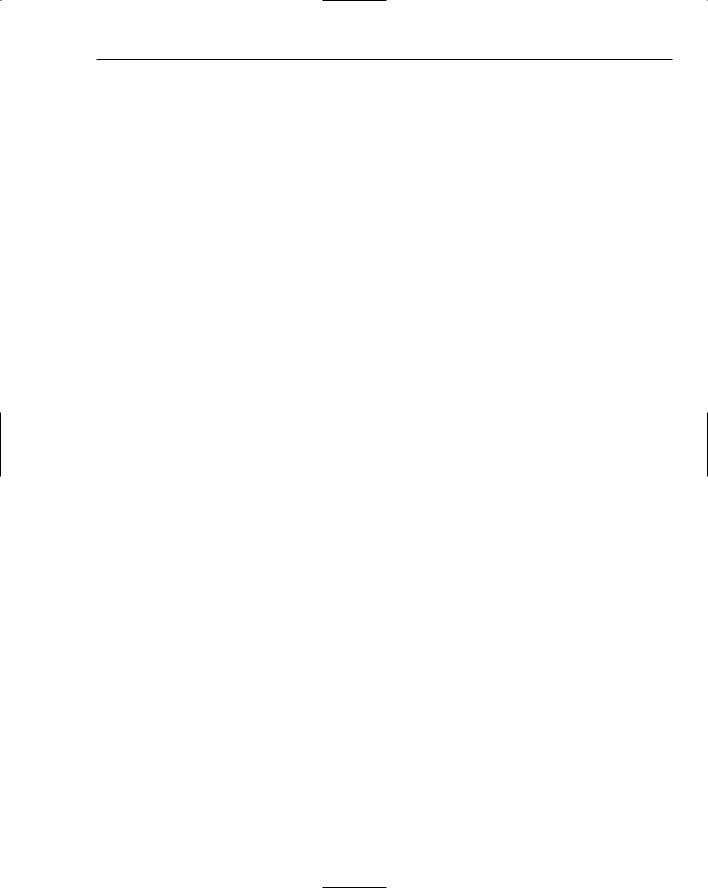