
Beginning ActionScript 2.0 2006
.pdf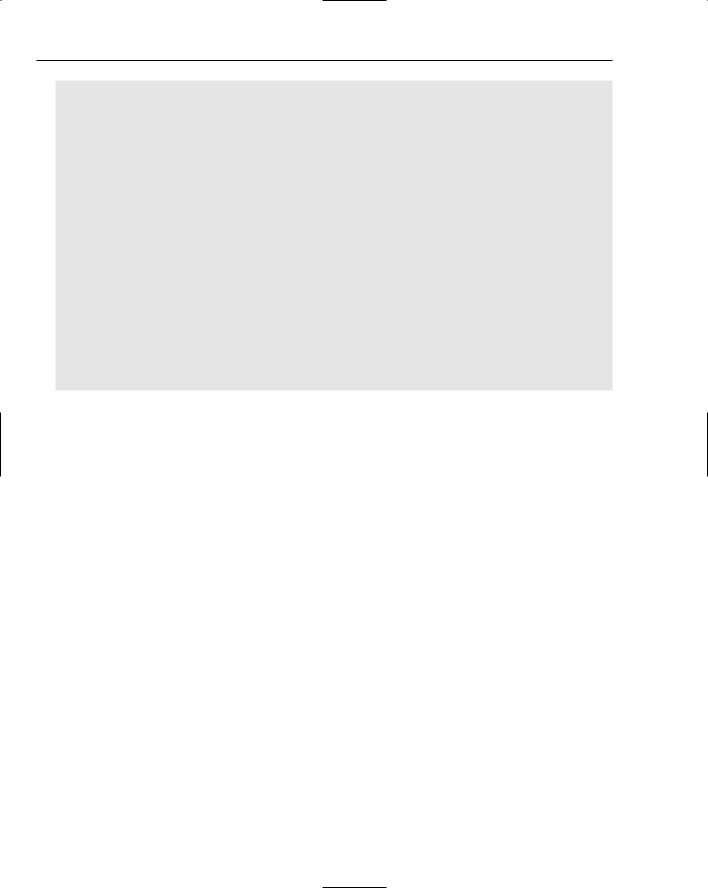
Chapter 10
mediaViewerPane.contentPath = mediaPath; mediaViewerProgress._visible = true; mediaViewerProgress.setProgress(0, 100);
}
}
function loadMediaError() : Void
{
trace(“loadMediaError”); mediaViewerProgress._visible = false; imageList.selectedIndex = null; videoList.selectedIndex = null; mediaViewerPane.contentPath = “”;
}
function setZoom(zoomValue:Number) : Void
{
mediaViewerPane.content._xscale = zoomValue; mediaViewerPane.content._yscale = zoomValue; mediaViewerPane.invalidate();
}
init();
9.Save the file, return to the Macromedia Flash project file, and select Control Test Movie.
How It Works
First, listener objects are created to handle functionality from different parts of the application. The media list listener deals only with handling the interaction between the two list components:
var mediaListListener:Object = new Object(); mediaListListener.change = function(eventObject:Object)
{
if (eventObject.target._name == “imageList”)
{
movieList.selectedIndex = null;
}
else if (eventObject.target._name == “movieList”)
{
imageList.selectedIndex = null;
}
}
The listener checks to see which list component generated the event. If the image list component generated the event, the listener deselects anything that might be selected in the movie list component, and vice versa. This code does not deal with any aspects of loading the media, just with managing the menu. If you were to add additional functionality — say, for example, thumbnail image buttons for selecting images — only this listener would need to be updated to accommodate it.
The media viewer listener manages the loading and presentation of the media in the scroll pane component:
var mediaViewerListener:Object = new Object(); mediaViewerListener.change = function(eventObject:Object)
268

Interacting with the User
{
if (eventObject.target._name == “imageList”)
{
loadMedia(eventObject.target.selectedItem.data, “image”);
}
else if (eventObject.target._name == “movieList”)
{
loadMedia(eventObject.target.selectedItem.data, “video”);
}
else if (eventObject.target._name == “zoomStepper”)
{
setZoom(eventObject.target.value);
}
}
Once again, the listener checks to see which component generated the event. If it is one of the list components, it loads the selected media clip into the scroll pane; if the event originates from the zoom box, it adjusts the scaling of the content.
The two loadMedia() functions pass along a parameter selecting the media type. This is not actually used here, but it could handle different media presentation implementations based on the media type. For instance, the video could be placed inside a movie clip instead of the scroll pane because movies generally should not scroll. If this change were to be made, only the media viewer listener would need to change. The media list listener would not need to be touched.
Next, the initialization function is defined. The image list component is loaded with data, and both listeners are registered with this component:
var imageArray:Array = new Array(); imageArray.push({data:”images/aStudyInTexture.jpg”, label:”A Study In Texture”}); imageArray.push({data:”images/buntzenWinter.jpg”, label:”Buntzen Winter”}); imageArray.push({data:”images/flowerInDetail.jpg”, label:”Flower In Detail”}); imageArray.push({data:”images/galianoSunset.jpg”, label:”Galiano Sunset”}); imageList.dataProvider = imageArray;
imageList.addEventListener(“change”, mediaListListener); imageList.addEventListener(“change”, mediaViewerListener);
The movie list component is loaded with data, and both listeners are registered with this component:
// Send data to the video list component var movieArray:Array = new Array();
movieArray.push({data:”images/animation.swf”, label:”Animation”}); movieArray.push({data:”movies/snowStorm.swf”, label:”Snow Storm”}); movieList.dataProvider = movieArray; movieList.addEventListener(“change”, mediaListListener); movieList.addEventListener(“change”, mediaViewerListener);
The zoom stepper is initialized, and the media viewer listener is registered with this component. The listener call takes the place of the separate zoom stepper listener:
// Setup for the zoom stepper component zoomStepper.maximum = 400;
269

Chapter 10
zoomStepper.minimum = 25; zoomStepper.stepSize = 25; zoomStepper.value = 100;
zoomStepper.addEventListener(“change”, mediaViewerListener);
The remaining code deals with the actual implementation of the loading and resizing, and is not changed significantly from the previous exercise with this project.
Manually Invoking Events in a Component
So far, it’s been assumed that component events are always triggered by a user action. That is not always the case; sometimes you may want to manually trigger an event, just so you do not have to write code to do something that’s already been handled. Say you have a list component, and you want to pre-select the first item in the list. You would do this with
componentHandle.selectedIndex = 0;
The problem is that the change event is generated only when a change is initiated by the user, so setting the selected row doesn’t affect the rest of your interface. You could manually call the same code that the event handler calls, but that is a waste. Instead, manually trigger the event using the dispatchEvent() method as follows, and any interested listeners also will be triggered:
componentHandle.dispatchEvent({type:”change”});
Try it out in the following exercise.
Try It Out |
Manually Trigger an Event |
In this exercise you manually trigger an event that otherwise would require a user action.
1.Create a new Macromedia Flash document.
2.Rename the existing layer in the timeline to Script. Create a new layer below it called
Components.
3.Click the keyframe on the Components layer to select it. Open the Components panel’s User Interface section. Drag one List component to the stage.
4.Open the Properties panel, click the button on the stage, and set the instance ID to myList.
5.The code for this example is small, so enter it in the Actions panel instead of creating a separate ActionScript file. Click the first frame in the timeline, open the Actions panel, and type in the following ActionScript code:
var listListener:Object = new Object(); listListener.change = function(eventObject:Object)
{
trace(“Selected item: “ + eventObject.target.selectedItem.data);
270
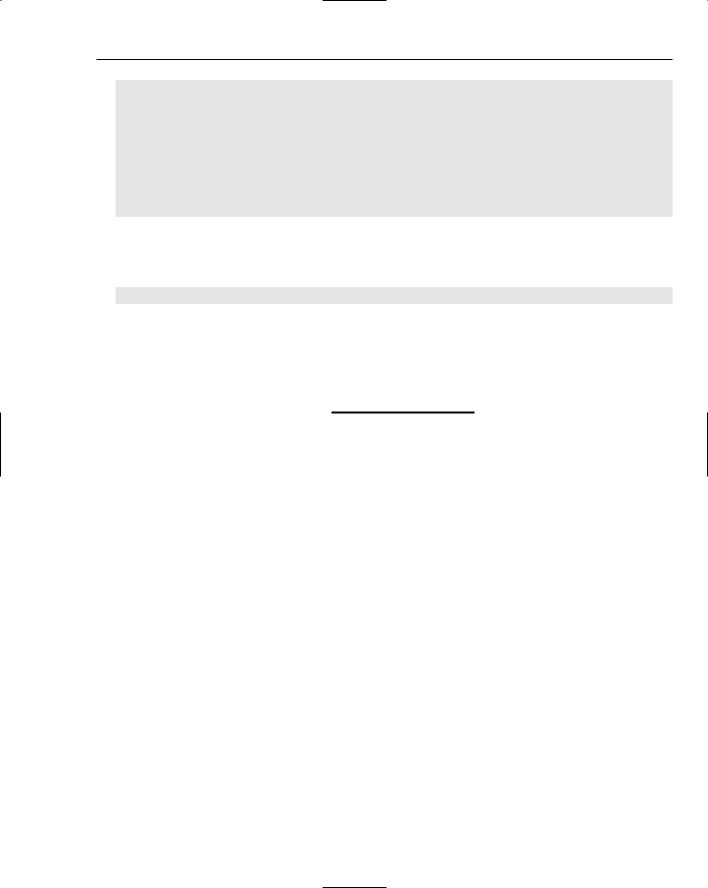
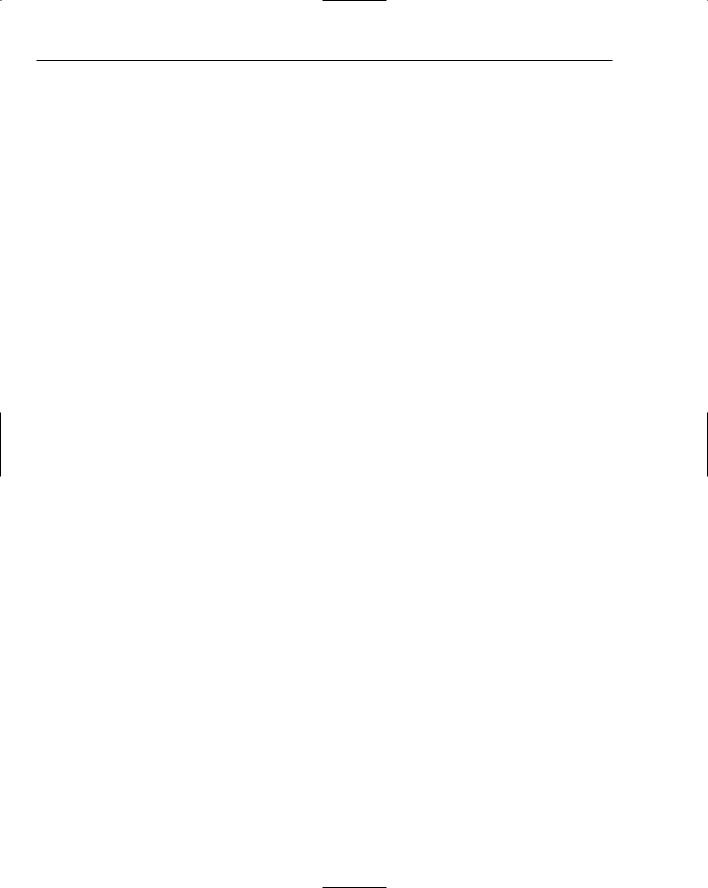
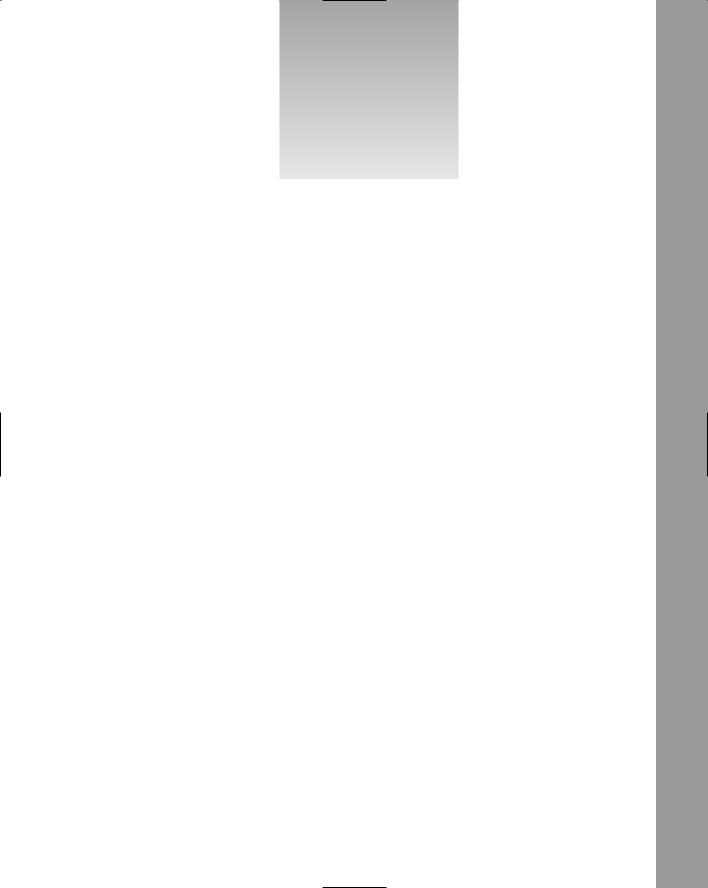
11
Controlling Components
Macromedia Flash has, since MX 2004, the capability for components to pass data between each other automatically. This is done through the concept of data binding, in which component inputs and outputs are linked to each other. You can set up data bindings either through the Component Inspector panel or by using the set of bindings classes. This book introduces how to set up bindings only through the Component Inspector panel because using the bindings classes is a complicated process that would almost require its own book.
Introducing the Component
Inspector Panel
The Component Inspector panel (Window Component Inspector) contains a tabbed interface as shown in Figure 11-1.
The Parameters tab, open in Figure 11-1, is a duplicate of the Parameters tab in the Properties panel. It contains basic startup information such as the URL for the XML file to load for the XMLConnector component.
The Bindings tab (see Figure 11-2) provides the means to link components together. One component can bind to multiple properties on multiple different components.
The Schema tab, open in Figure 11-3, shows the properties that are available for binding with other components. For the XMLConnector component, you can link specific tags in your XML file to different components by adding information about the XML data. You can also define custom properties that can send and receive data.
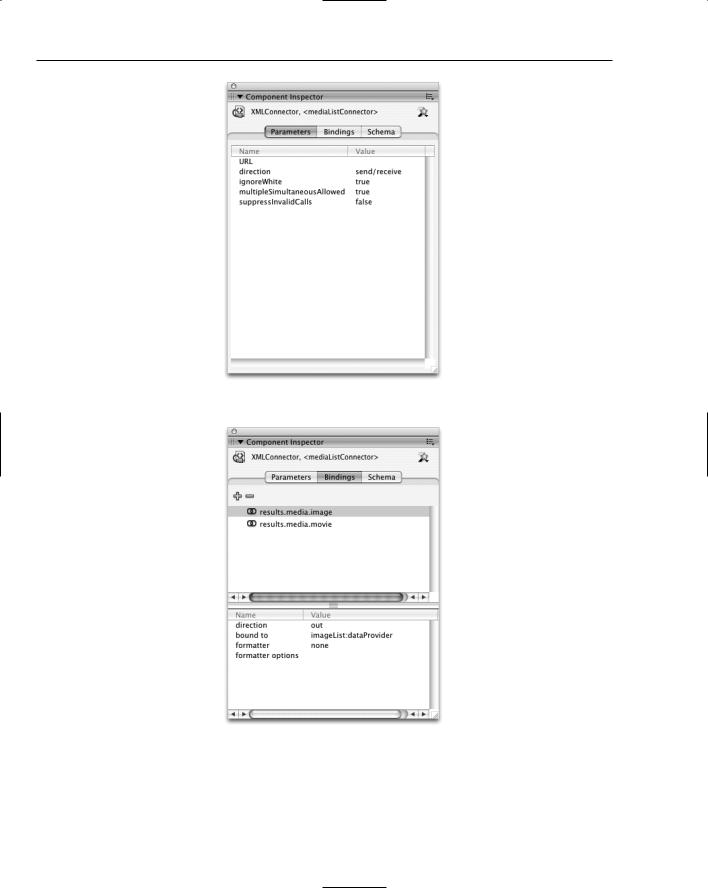
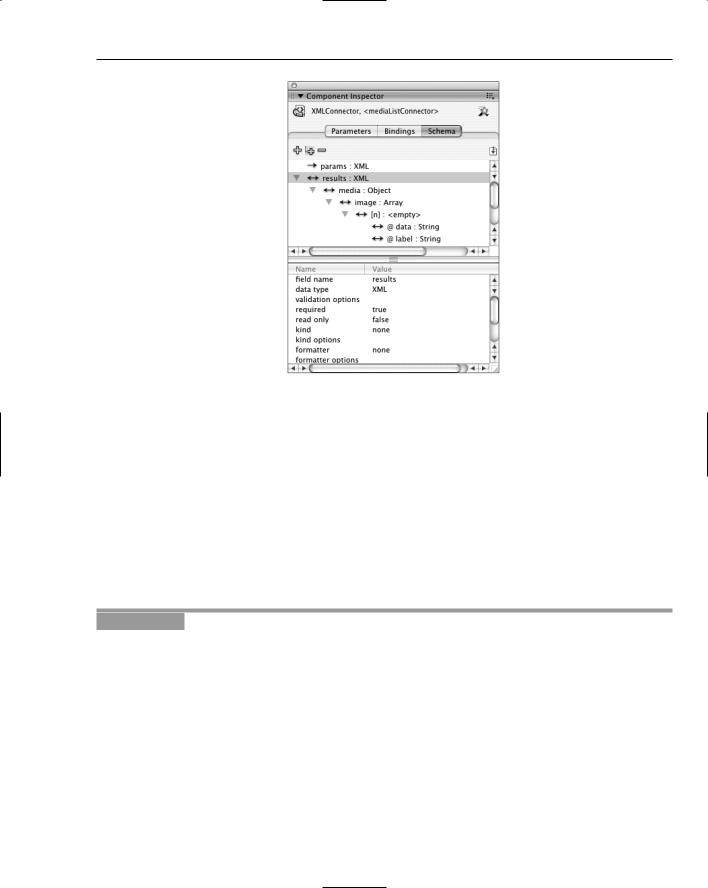
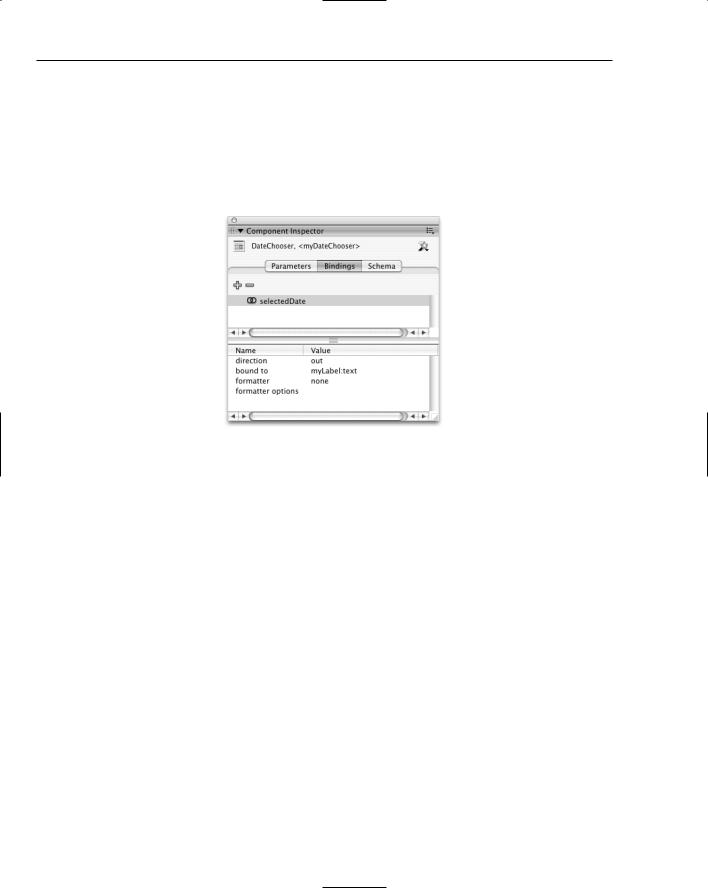
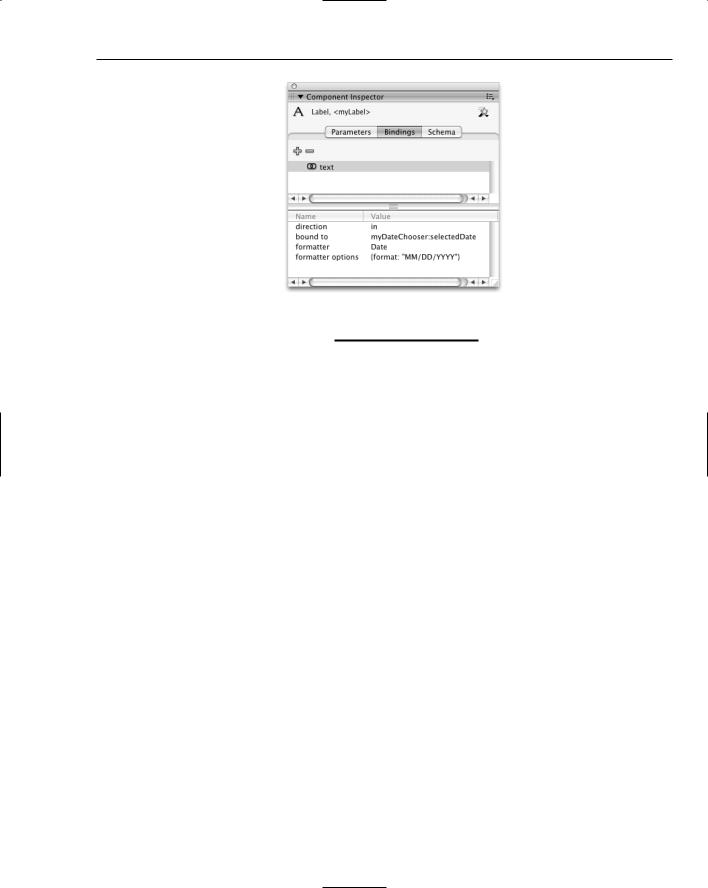