
Beginning ActionScript 2.0 2006
.pdf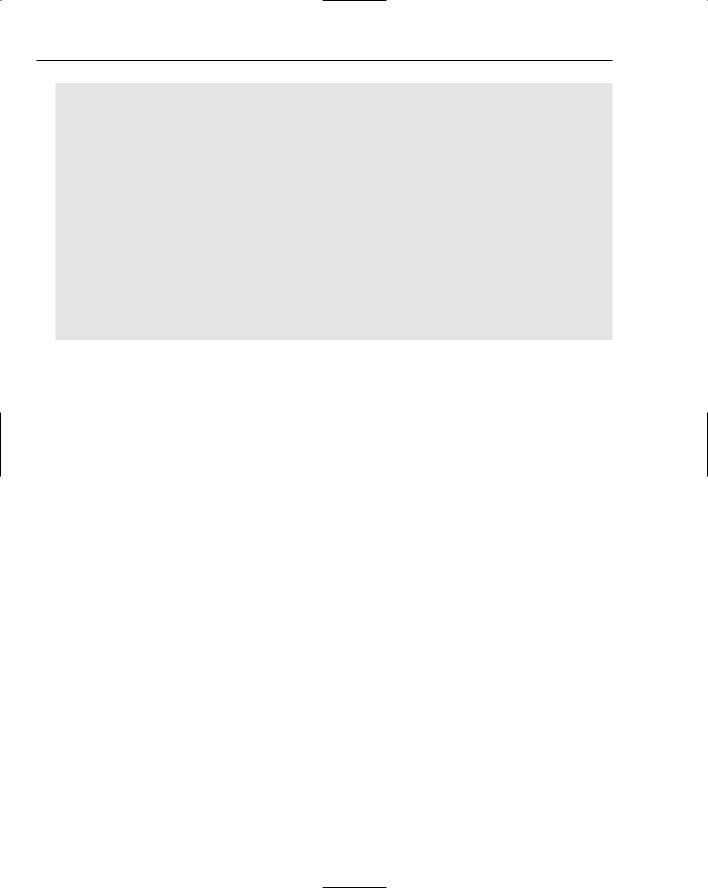
Chapter 7
triangle1Clip.lineTo(100, 100); triangle1Clip.lineTo(50, 0); triangle1Clip.endFill();
triangle1Clip.duplicateMovieClip(“triangle2Clip”, this.getNextHighestDepth(), ; {_x:50, _y:50});
triangle1Clip.onPress = function()
{
this.startDrag();
};
triangle1Clip.onRelease = function()
{
this.stopDrag();
if (this.hitTest(triangle2Clip) == true)
{
trace(“shapes are overlapping”);
}
};
If one triangle is within the rectangle that bounds the other triangle, the hit test returns true even though the triangles themselves may not actually be touching. This limits the usefulness of the hitTest() method for irregular shapes. For more information about using ActionScript to detect collisions, go to www.moock.org/webdesign/flash/actionscript/collision/.
lineStyle()
The lineStyle() method defines the characteristics of a line to draw for any lineTo() method calls. If it isn’t called before a lineTo(), no line is drawn.
This method takes between one and eight parameters:
1.
2.
3.
thickness. A Number indicating the thickness of the line.
color. A Number representing the color to use.
alpha. A Number representing the transparency to use, with 100 being fully opaque.
The thickness, color, and alpha (opacity) parameters work in Flash Player 6, but the remaining parameters require at least Flash Player 8:
4. pixelHinting. A Boolean indicating whether to use pixel hinting, where strokes are made to end on a full pixel.
5. noScale. A String indicating how to scale the line if the parent movie clip scales. Possible values are normal, none, vertical, and horizontal.
6. capsStyle. A String indicating how to cap off the ends of lines. Possible values are round, square, and none.
7. jointStyle. A String indicating how line segment joints are to be displayed. Possible values are round, miter, and none.
8. miterLimit. A Number that controls how large mitered corners are allowed to get before being cut off.
168

Controlling Movie Clips
The following prototype shows the basic form of lineStyle():
baseClip.lineStyle(thickness:Number, [color:Number], [alpha:Number], ; [pixelHinting:Boolean], [noScale:String], [capsStyle:String], ; [jointStyle:String], [miterLimit:Number]) : Void
The method is used in this example, which draws two connected lines:
this.createEmptyMovieClip(“shapeClip”, this.getNextHighestDepth()); shapeClip.lineStyle(5, 0x000000, 100, true, “normal”, “square”, “miter”, 3); shapeClip.moveTo(100, 50);
shapeClip.lineTo(50, 150); shapeClip.lineTo(150, 150);
Multiple calls to this method can be made through the course of drawing to the movie clip. A line style applies to all drawing commands until the next line style call is made, after which all subsequent drawing commands take on the new line style.
You cannot change just one style attribute with a subsequent lineStyle() call. All styles left unspecified in the method revert to their default values.
lineTo()
The lineTo() method is used to draw a line, and also to designate the bounds for a filled shape.
This method takes just the x and the y coordinates for the new coordinate. The line is drawn from the previous lineTo() coordinates or from the previous moveTo() coordinates and extends to the specified x and y values. lineTo() is used in conjunction with the lineStyle() and moveTo() methods, and is very useful for dynamically drawing buttons, backgrounds, or code-generated animation. The following prototype shows the method’s basic form:
baseClip.lineTo(x:Number, y:Number) : Void
Here’s an example of using lineTo():
this.createEmptyMovieClip(“shapeClip”, this.getNextHighestDepth()); shapeClip.lineStyle(1, 0x000000);
shapeClip.moveTo(100, 50); shapeClip.lineTo(50, 150); shapeClip.lineTo(150, 150);
loadMovie()
loadMovie() is a core method for loading external content. It takes either one or two parameters. The first is the URL for the content to load into the movie clip. The second is an optional parameter for sending or loading variables, with possible values GET and POST. This method can load .jpg and .swf files, and, as of version 8 of the player, it can also load .gif and .png files. The following prototype shows the method’s basic form:
baseClip.loadMovie(url:String, [method:String]) : Void
169
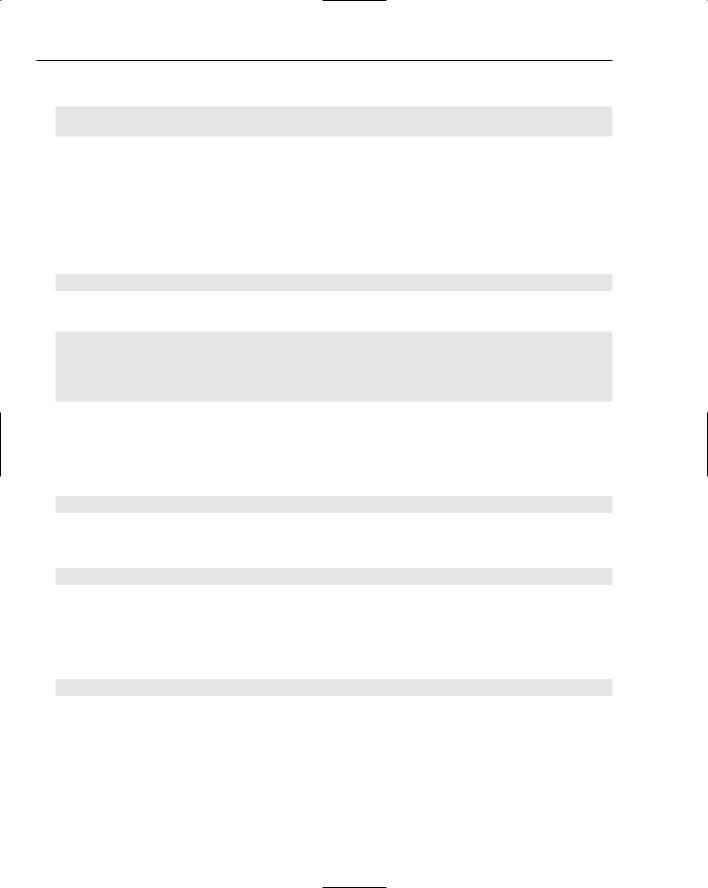
Chapter 7
Here’s a sample implementation of the method:
this.createEmptyMovieClip(“animationHolder”, this.getNextHighestDepth());
animationHolder.loadMovie(“http://www.nathanderksen.com/book/sampleAnimation.swf”);
When loading external content, many methods will not work on that movie clip until it has at least begun to download from the server. A pre-loader is needed to delay these methods’ execution until the movie clip is ready. Pre-loaders are covered in detail in Chapter 8.
moveTo()
The moveTo() method moves the drawing pen to a starting position before anything is actually drawn. It takes two parameters: the x and y coordinates to go to. The following prototype shows its basic form:
baseClip.moveTo(x:Number, y:Number) : Void
Here’s an example showing its use:
this.createEmptyMovieClip(“shapeClip”, this.getNextHighestDepth()); shapeClip.lineStyle(1, 0x000000);
shapeClip.moveTo(100, 50); shapeClip.lineTo(50, 150); shapeClip.lineTo(150, 150);
play()
play() has both movie clip method and global function versions. The movie clip method takes no parameters, and it resumes playback of the specified movie clip from the current playhead position. Here’s the prototype of its basic form:
baseClip.play() : Void
The global function version takes no parameters, and it resumes playback of the timeline holding the code from the current playhead position. The following prototype shows its basic form:
play() : Void
removeMovieClip()
The removeMovieClip() method removes a movie clip that has been dynamically placed on the stage through the attachMovie(), duplicateMovieClip(), or createEmptyMovieClip() methods. It takes no parameters. The following prototype shows the method’s basic form:
baseClip.removeMovieClip() : Void
removeMovieClip() is not used to remove movie clips that have been placed on the stage with the authoring tool. These clips are all assigned negative depth values. One work-around is to use
swapDepths() to move the movie clip to a positive depth, and then to delete the clip. Another option, which is actually preferable, is to dynamically place all movie clips on the stage so that they can all be dynamically removed.
170
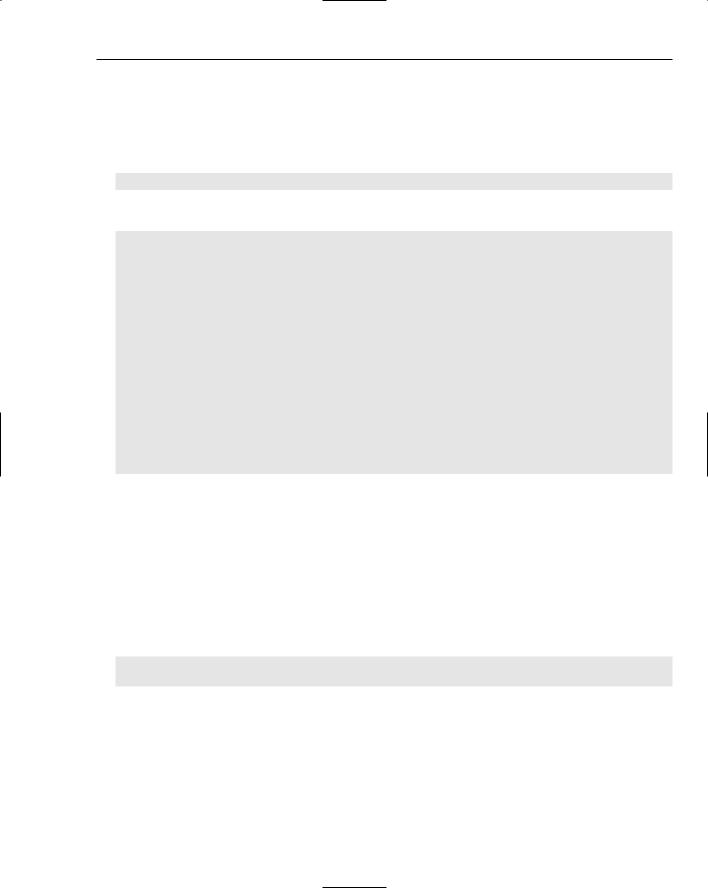
Controlling Movie Clips
setMask()
setMask() uses the shape of one movie clip to define what is visible on another movie clip. It is useful for numerous visual effects and to define a cropping area for a clip that may be scrolled. This method takes a single parameter, which is a movie clip to use for the mask. The following prototype shows the basic form of setMask():
baseClip.setMask(maskClip:Object) : Void
Here’s an example implementation of the method:
this.createEmptyMovieClip(“shapeClip”, this.getNextHighestDepth()); shapeClip.beginFill(0x000066);
shapeClip.moveTo(150, 50); shapeClip.lineTo(50, 250); shapeClip.lineTo(250, 250); shapeClip.lineTo(150, 50); shapeClip.endFill();
this.createEmptyMovieClip(“maskClip”, this.getNextHighestDepth());
maskClip.beginFill(0x660000); maskClip.moveTo(100, 100); maskClip.lineTo(200, 100); maskClip.lineTo(200, 300); maskClip.lineTo(100, 300); maskClip.lineTo(100, 100); maskClip.endFill();
shapeClip.setMask(maskClip);
Only the shape of the mask is used to determine which parts of the main movie clip are to be visible. Semi-transparent areas of the mask have no impact on the transparency of the main movie clip: they just add to the overall shape of the mask.
startDrag()
The startDrag() method is used to indicate that if a user clicks and drags over the movie clip, the clip should follow the cursor. It takes between zero and five parameters. The first parameter is a Boolean that, when true, centers the movie clip under the mouse cursor when the drag operation starts. The next four parameters delimit the left, top, right, and bottom edges of a rectangle that restricts the range of the drag motion. The following prototype shows the method’s basic form:
baseClip.startDrag([lockCenter:Boolean], [left:Number], [top:Number], ;
[right:Number], [bottom:Number}) : Void
Setting left and right to the same value restricts the drag to only vertical movement, whereas setting top and bottom to the same value restricts the drag to only horizontal movement. This method is generally called when the mouse button is pressed, and its corresponding method, stopDrag(), is generally called when the mouse button is released. The following example creates a movie clip, draws a shape inside the movie clip, and then sets the movie clip to be draggable when the mouse button has been pressed:
171
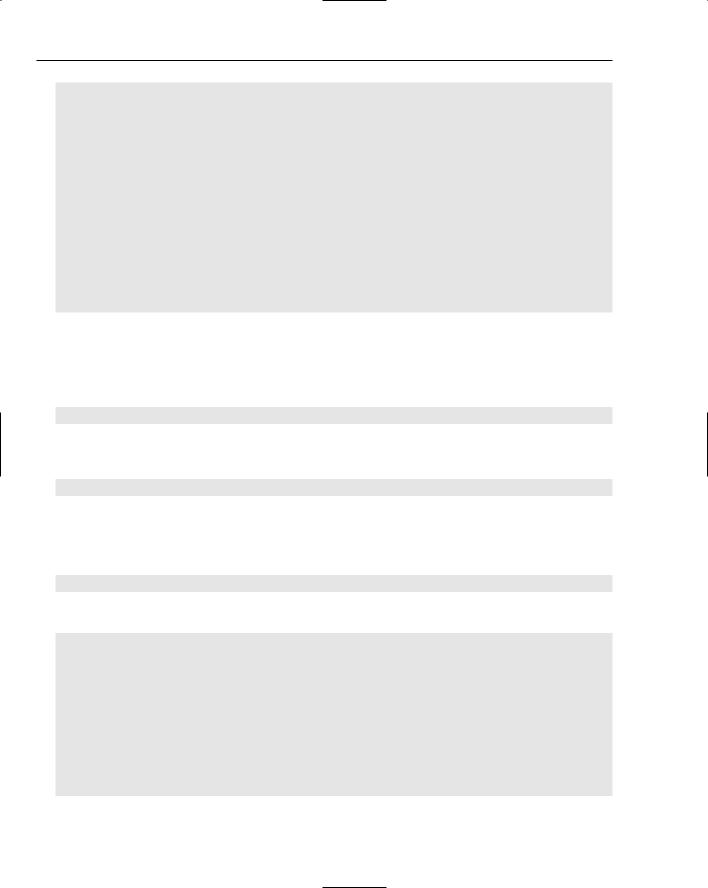
Chapter 7
this.createEmptyMovieClip(“shapeClip”, this.getNextHighestDepth()); shapeClip.beginFill(0x000066);
shapeClip.moveTo(150, 50); shapeClip.lineTo(50, 250); shapeClip.lineTo(250, 250); shapeClip.lineTo(150, 50); shapeClip.endFill();
shapeClip.onPress = function()
{
this.startDrag();
};
shapeClip.onRelease = function()
{
this.stopDrag();
};
stop()
The stop() method has both movie clip method and global function versions. The movie clip method takes no parameters, and it stops playback of the specified movie clip. The following prototype shows its basic form:
baseClip.stop() : Void
The global function version takes no parameters, and it stops playback of the timeline holding the code. Here’s the prototype of its basic form:
stop() : Void
stopDrag()
The stopDrag() method is used to stop a dragging operation, and it is generally called when the mouse button has been released. It takes no parameters. The following prototype shows its basic form:
baseClip.stopDrag() : Void
Here’s an example implementation:
this.createEmptyMovieClip(“shapeClip”, this.getNextHighestDepth()); shapeClip.beginFill(0x000066);
shapeClip.moveTo(150, 50); shapeClip.lineTo(50, 250); shapeClip.lineTo(250, 250); shapeClip.lineTo(150, 50);
shapeClip.endFill();
shapeClip.onPress = function()
{
this.startDrag();
};
172
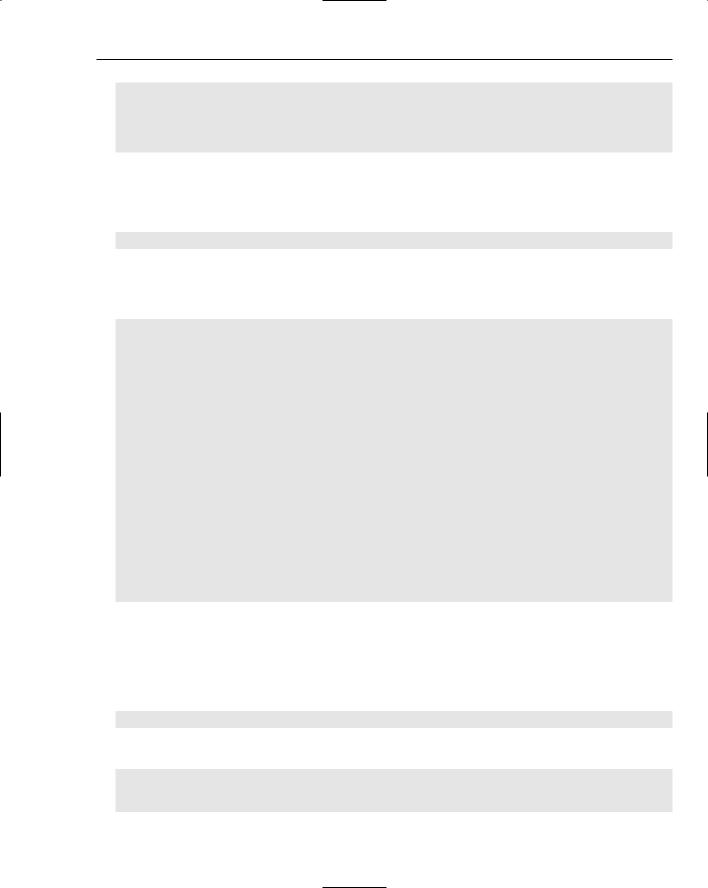
Controlling Movie Clips
shapeClip.onRelease = function()
{
this.stopDrag();
};
swapDepths()
swapDepths() is useful for changing the movie clip stacking order. It takes one parameter, which is either a handle to a movie clip or a depth number. Here’s the prototype of the method’s basic form:
baseClip.swapDepths(secondClip:MovieClip) : Void
If a movie clip handle is passed, the two movie clips swap depths. If a number is passed, the movie clip switches to that depth number. The following example demonstrates the behavior by swapping the two movie clips when the square is clicked:
this.createEmptyMovieClip(“triangleClip”, this.getNextHighestDepth()); triangleClip.beginFill(0x000066);
triangleClip.moveTo(150, 50); triangleClip.lineTo(50, 250); triangleClip.lineTo(250, 250); triangleClip.lineTo(150, 50); triangleClip.endFill();
this.createEmptyMovieClip(“squareClip”, this.getNextHighestDepth()); squareClip.beginFill(0x660000);
squareClip.moveTo(25, 25); squareClip.lineTo(25, 150); squareClip.lineTo(150, 150); squareClip.lineTo(150, 25); squareClip.lineTo(25, 25); squareClip.endFill();
squareClip.onRelease = function()
{
this.swapDepths(triangleClip);
};
For two movie clips to swap depths, they must have the same parent movie clip.
unloadMovie()
The unloadMovie() method is used when removing external content that has been loaded using the loadMovie() method. It takes no parameters. Here’s its basic form:
baseClip.unloadMovie() : Void
The following code example loads an external movie, waits three seconds, and then unloads the movie:
var intervalId:Number;
this.createEmptyMovieClip(“mediaClip”, this.getNextHighestDepth()); mediaClip.loadMovie(“http://www.nathanderksen.com/book/sampleAnimation.swf”);
173
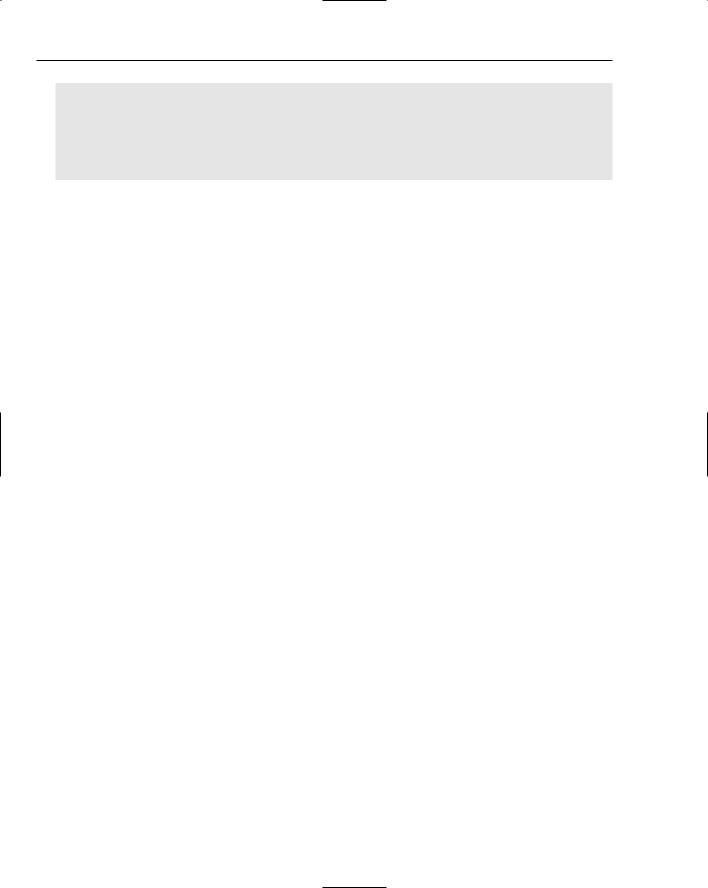
Chapter 7
intervalId = setInterval(unloadContent, 3000);
function unloadContent()
{
mediaClip.unloadMovie();
clearInterval(intervalId);
}
You need to try this from a server for it to work.
There is an apparent bug in version 8 of the Flash player that mistakenly identifies a remote file as trying to manipulate a local file and prevents the action, when it is actually the other way around.
MovieClip Class Properties
The MovieClip class comes with some properties that describe a number of aspects of the movie clip. For instance, the _x, _y, _width, _height, _xscale, and _yscale properties enable you to specify the position and the size of any movie clip. You use the _alpha property to set how transparent a movie clip is, and the _visible property indicates whether a movie clip is being rendered to the stage. Any property that starts with an underscore character is a core movie clip property that has for the most part been available in the Flash Player since version 5. In version 6, some of the new properties used the underscore convention, and some did not. In version 7 and later, the convention was to not use the underscore as a part of property names.
The following table describes the core properties available for your use:
Property |
Type |
Description |
|
|
|
_alpha |
Number |
The movie clip transparency. |
_currentframe |
Number |
The frame number where the playhead is currently posi- |
|
|
tioned. (Read only.) |
_droptarget |
String |
The path to the movie clip that a draggable clip was dropped |
|
|
on. (Read only.) |
_focusrect |
Boolean |
Indicates whether the movie clip currently has keyboard focus. |
_framesloaded |
Number |
The number of frames of an externally loaded movie clip that |
|
|
have loaded so far. (Read only.) |
_height |
Number |
The height of the movie clip. |
_lockroot |
Boolean |
Designates the specified movie clip as the target for all refer- |
|
|
ences to _root that occur in code within that movie clip. |
_name |
String |
The instance name of the movie clip. |
_parent |
Movie Clip |
The handle to the movie clip that contains the specified clip. |
_quality |
String |
Sets the rendering quality used to display movie clip content. |
|
|
Can be low, medium, high, or best. |
|
|
|
174
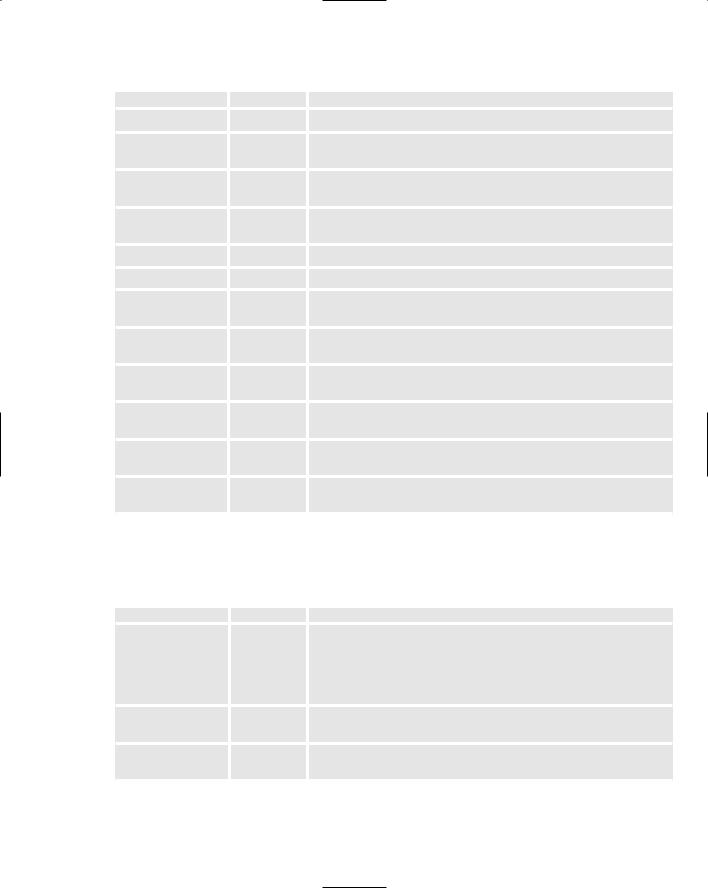
|
|
|
Controlling Movie Clips |
|
|
|
|
|
Property |
Type |
Description |
|
|
|
|
|
_rotation |
Number |
Sets the number of degrees to rotate the movie clip. |
|
_target |
String |
Gets the path to the movie clip relative to the main timeline in |
|
|
|
slash notation. (Read only.) |
|
_totalframes |
Number |
Gets the total number of frames used by the movie clip. |
|
|
|
(Read only.) |
|
_url |
String |
Gets the address from which an SWF, JPG, GIF, or PNG file |
|
|
|
was loaded into the movie clip. (Read only.) |
|
_visible |
Boolean |
The visibility of the movie clip. |
|
_width |
Number |
The width of the movie clip. |
|
_x |
Number |
The x coordinate of the movie clip relative to the parent |
|
|
|
movie clip. |
|
_xmouse |
Number |
The x coordinate of the mouse pointer relative to the main |
|
|
|
timeline. (Read only.) |
|
_xscale |
Number |
The percentage horizontal scaling of the clip. A value of 100 |
|
|
|
designates normal width. |
|
_y |
Number |
The y coordinate of the movie clip relative to the parent |
|
|
|
movie clip. |
|
_ymouse |
Number |
The y coordinate of the mouse pointer relative to the main |
|
|
|
timeline. (Read only.) |
|
_yscale |
Number |
The percentage vertical scaling of the clip. A value of 100 |
|
|
|
designates normal height. |
Properties such as blendMode and filters provide the capability to create unique effects, and scale9grid and transform are powerful new tools to manipulate movie clips. The following properties supplement the core movie clip properties:
Property |
Type |
Description |
|
|
|
blendMode |
Object |
The type of blending that the movie clip uses with content |
|
|
behind it. Possible values are normal, layer, multiply, |
|
|
screen, lighten, darken, difference, add, subtract, |
|
|
invert, alpha, erase, overlay, and hardlight. (Flash |
|
|
Player 8 only.) |
cacheAsBitmap |
Boolean |
Sets whether the player will store a bitmap representation of |
|
|
the movie clip for faster rendering. (Flash Player 8 only.) |
enabled |
Boolean |
If set to false, blocks event handlers from being called by the |
|
|
movie clip. |
|
|
Table continued on following page |
175

Chapter 7
Property |
Type |
Description |
|
|
|
filters |
Object |
Stores an array of filters that have been applied to the movie |
|
|
clip. (Flash Player 8 only.) |
focusEnabled |
Boolean |
Allows a movie clip to receive focus, even if it is not a button. |
hitArea |
Object |
Allows the clickable area of a movie clip used as a button to |
|
|
be defined by the shape of another movie clip. |
menu |
Object |
Associates a contextual menu object with the movie clip. |
opaque |
Number |
Sets the transparent portions of the movie clip to the selected |
Background |
|
color. (Flash Player 8 only.) |
scale9Grid |
Rectangle |
Allows a movie clip to be scaled so that some areas |
|
|
stretch while other areas maintain their dimensions. |
|
|
(Flash Player 8 only.) |
scrollRect |
Rectangle |
Defines a cropping rectangle for movie clip content. Content |
|
|
that falls outside that area is hidden until scrolled to. (Flash |
|
|
Player 8 only.) |
tabChildren |
Boolean |
Determines which child movie clips are included in the auto- |
|
|
matic tab ordering. |
tabEnabled |
Boolean |
Determines whether the movie clip is to be included in the |
|
|
automatic tab ordering. |
tabIndex |
Number |
Determines the position of the movie clip in the tab ordering. |
trackAsMenu |
Boolean |
When set to true, changes the button click behavior so that |
|
|
clicking down on one button, dragging onto another button, |
|
|
and then releasing the mouse will engage the second button. |
transform |
Transform |
Holds an object that records details of the movie clip’s trans- |
|
|
formation matrix, color transform details, and pixel bounds. |
|
|
(Flash Player 8 only.) |
useHandCursor |
Boolean |
Changes the cursor to a hand when hovering over the |
|
|
movie clip. |
MovieClip Class Events
Movie clips provide a means to follow up with your own action when certain actions take place, such as when the user mouses over or clicks a movie clip. When such actions take place, the movie clip generates an event, which is a signal that something of note has happened. You can write code that responds by assigning a function to that event. The following example shows that assigning a function to the onRelease event causes the code within the function to be executed every time the movie clip is clicked:
this.createEmptyMovieClip(“triangleClip”, this.getNextHighestDepth()); triangleClip.beginFill(0x000066);
triangleClip.moveTo(150, 50); triangleClip.lineTo(50, 250);
176
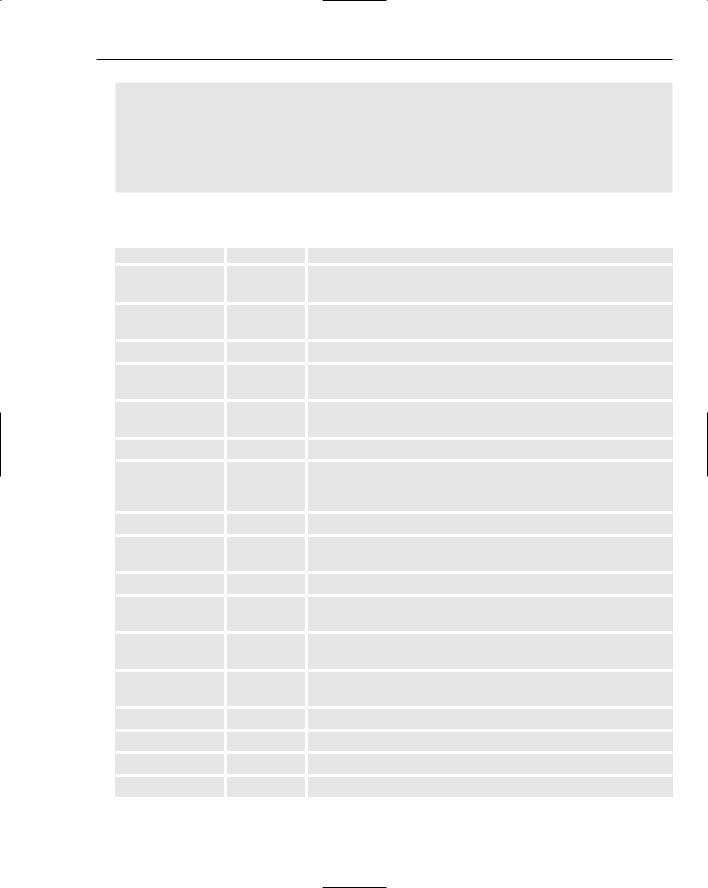
Controlling Movie Clips
triangleClip.lineTo(250, 250); triangleClip.lineTo(150, 50); triangleClip.endFill();
triangleClip.onRelease = function()
{
trace(“Clicked on triangle”);
};
The following movie clip events are available for you to use:
Event |
Type |
Description |
|
|
|
onDragOut |
Function |
Fires when the mouse button has been pressed and the mouse |
|
|
leaves the bounds of the movie clip. |
onDragOver |
Function |
Fires when the mouse button has been pressed and the mouse |
|
|
leaves and re-enters the bounds of the movie clip. |
onEnterFrame |
Function |
Fires every time the playhead moves to the next frame. |
onKeyDown |
Function |
Fires when the movie clip has input focus and a key on the |
|
|
keyboard is pressed. |
onKeyUp |
Function |
Fires when the movie clip has input focus and a key on the |
|
|
keyboard is released. |
onKillFocus |
Function |
Fires when the movie clip loses keyboard focus. |
onLoad |
Function |
Fires when a new movie clip instance appears on the timeline. |
|
|
Does not fire when external content is loaded into an existing |
|
|
movie clip. |
onMouseDown |
Function |
Fires when the mouse button has been pressed. |
onMouseMove |
Function |
Fires when the position of the mouse changes from the previ- |
|
|
ous time the event fired. |
onMouseUp |
Function |
Fires when the mouse button has been released. |
onPress |
Function |
Fires when the mouse button has been pressed while over the |
|
|
movie clip. |
onRelease |
Function |
Fires when the mouse button has been released while over the |
|
|
movie clip. |
onRelease |
Function |
Fires when the mouse button has been pressed while over the |
Outside |
|
movie clip, moved, and then released outside the movie clip. |
onRollOut |
Function |
Fires when the mouse leaves the bounds of the movie clip. |
onRollOver |
Function |
Fires when the mouse enters the bounds of the movie clip. |
onSetFocus |
Function |
Fires when the movie clip gains keyboard focus. |
onUnload |
Function |
Fires after the movie clip is removed from the timeline. |
177