
ASP.NET 2.0 Instant Results
.pdf




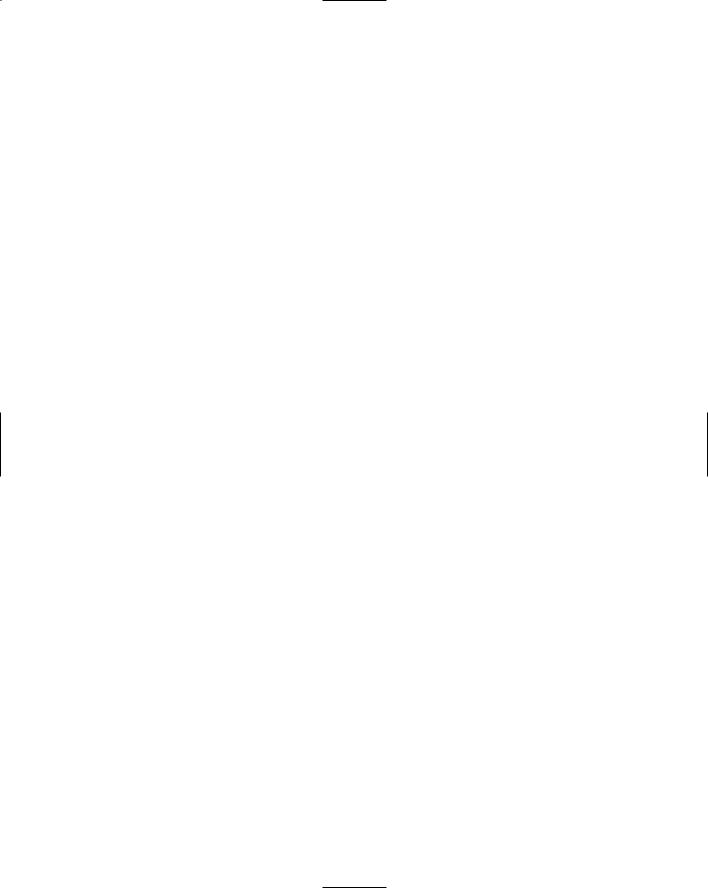
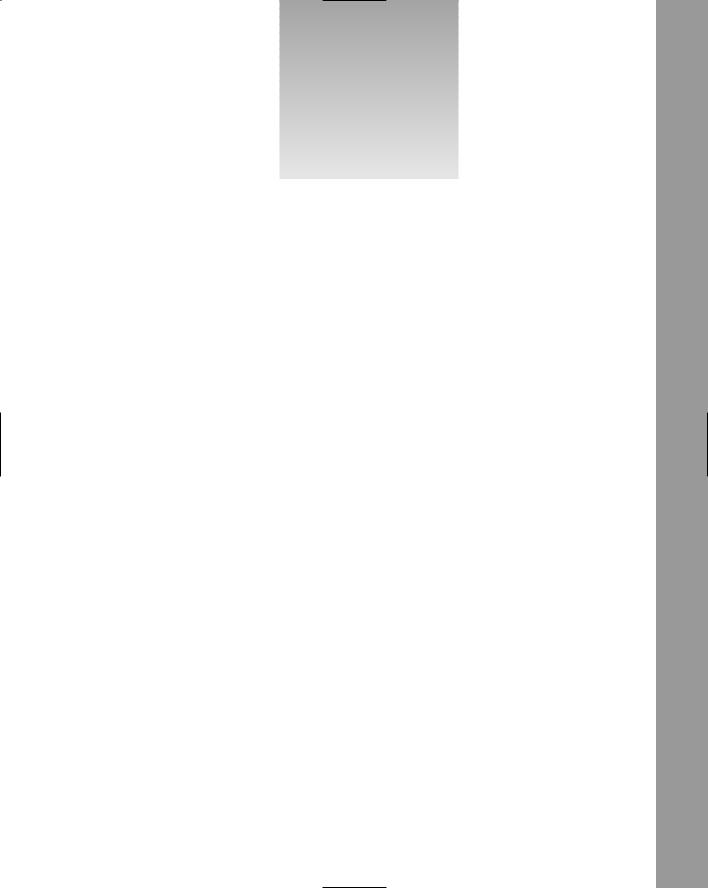
11
Modifying the Greeting Card
Application
The code you have seen in Chapter 11 just scratches the surface of what’s possible with GDI+ in
.NET. There are many classes and methods that enable you to fulfill all your drawing needs. The Graphics class alone has almost 70 methods that allow you to draw rectangles, curves, bezier shapes, lines, pies, and so on. You’ll find many more classes and useful methods, properties, and enumerations in the important drawing namespaces like System.Drawing, System.Drawing
.Drawing2D, System.Drawing.Imaging, and System.Drawing.Text. With these namespaces you could enhance the Toolkit with the following features:
Expand the Toolkit’s Imaging class with a method called AddImageToImage that places an image on top of anther image. This is useful when you want to add your company’s logo to each outgoing image, for example.
Add a method to the Imaging class called AddWatermark. This method can do the same as the AddImageToImage that was just mentioned, but in the AddWatermark you could make the image more or less transparent.
Add methods that give you finer control over the text being added to images. For example, you could create overloads or separate methods that can underline text, or make it semitransparent so it doesn’t disturb the image too much. This is a great enhancement if you want to protect your images with a small copyright notice.
Add drop shadow support to the AddTextToImage method. Using a tiny drop shadow effect on the text that is added to the image can make a big difference in how the text looks.
In addition to changing the Toolkit, you can also change the Greeting Card application. Since it’s just built to showcase the possibilities of GDI+ and the generic Toolkit, the application lacks a lot of features that you’d expect from a true Greeting Card application. Besides building user controls to implement the behavior suggested in the previous list, you could enhance the Greeting Card application with the following features:
Write clean-up code in Session_Start or at a specified interval that cleans up the Temp folder. Right now, the application leaves behind many temporary images in that folder that will fill up your server’s hard drive in no time. You could write code that uses the System.IO namespace to delete all images that are older than an hour or so.
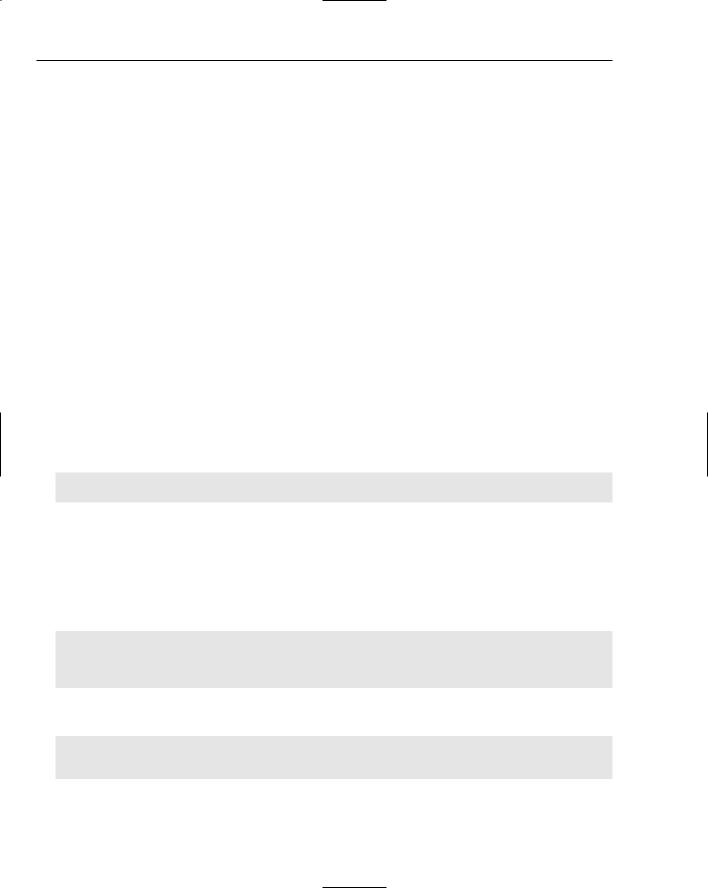

