
Lab1 / C++ Code Conventions
.pdf
C/C++ Coding Guidlines
1.8Statements
1.8.1Simple Statements
Each line should contain at most one statement. Example:
argv++; |
/* Correct */ |
argc--; |
/* Correct */ |
argv++; argc--; |
/* AVOID! */ |
1.8.2Compound Statements
Compound statements are statements that contain lists of statements enclosed in braces \f statements g\. See the following sections for examples.
The enclosed statements should be indented one more level than the compound statement.
The opening brace should be on a line by itself following the line that begins the compound statement, indented to the same level as that line; the closing brace should begin a line and be indented to the beginning of the compound statement.
Braces are used around all statements, even single statements, when they are part of a control structure, such as a if-else or for statement. This makes it easier to add statements without accidentally introducing bugs due to forgetting to add braces.
1.8.3return Statements
A return statement with a value should not use parentheses unless they make the return value more obvious in some way. Example:
return;
return MyDisk_size();
return (size ? size : defaultSize);
1.8.4if, if-else, if else-if else Statements
The if-else class of statements should have the following form:
if (condition)
{
statements;
}
if (condition)
{
statements;
}
Internal, Informational |
10/17 |
2002/05/02 { 22:26:22
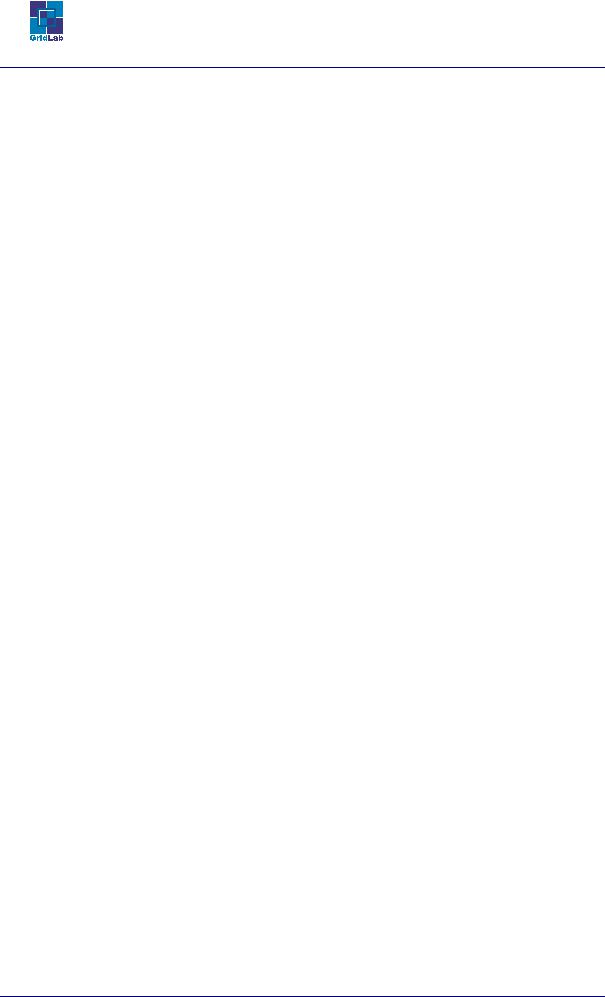
C/C++ Coding Guidlines
else
{
statements;
}
if (condition)
{
statements;
}
else if (condition)
{
statements;
}
else
{
statements;
}
Note:
if statements always use braces fg. Avoid the following error-prone form:
if (condition) /*AVOID! THIS OMITS THE BRACES {}! */ statement;
1.8.5for Statements
A for statement should have the following form:
for (initialization; condition; update)
{
statements;
}
An empty for statement (one in which all the work is done in the initialization, condition, and update clauses) should have the following form:
for (initialization; condition; update);
When using the comma operator in the initialization or update clause of a for statement, avoid the complexity of using more than three variables. If needed, use separate statements before the for loop (for the initialization clause) or at the end of the loop (for the update clause).
1.8.6while Statements
A while statement should have the following form:
while (condition)
{
statements;
}
Internal, Informational |
11/17 |
2002/05/02 { 22:26:22

C/C++ Coding Guidlines
An empty while statement should have the following form:
while (condition);
1.8.7do-while Statements
A do-while statement should have the following form:
do
{
statements;
}while (condition);
1.8.8switch Statements
A switch statement should have the following form:
switch (condition)
{
case ABC: statements;
/* falls through */ case DEF:
statements;
break; case XYZ:
statements;
break;
default:
statements;
break;
}
Every time a case falls through (doesn't include a break statement), add a comment where the break statement would normally be. This is shown in the preceding code example with the /* falls through */ comment.
Every switch statement should include a default case. The break in the default case is redundant, but it prevents a fall-through error if later the default is changed to a speci c case and a new default is introduced.
1.9White Space
1.9.1Blank Lines
Blank lines improve readability by setting o sections of code that are logically related. Two blank lines should always be used in the following circumstances:
Between sections of a source le
One blank line should always be used in the following circumstances:
Between functions
Internal, Informational |
12/17 |
2002/05/02 { 22:26:22
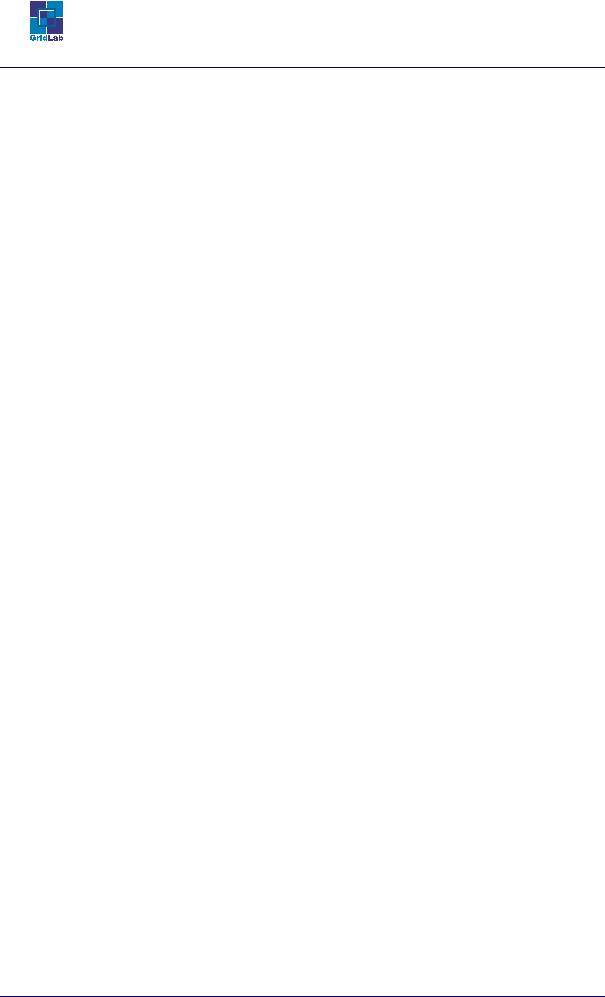
C/C++ Coding Guidlines
Between the local variable declarations in a block and its rst statement
Before a block (see section 1.6.1) or single-line (see section 1.6.1) comment
Between logical sections inside a function to improve readability
1.9.2Blank Spaces
Blank spaces should be used in the following circumstances:
A blank space should appear after commas in argument lists.
All binary operators should be separated from their operands by spaces. Blank spaces should never separate unary operators such as unary minus, increment (\++"), and decrement (\{") from their operands. Example:
a += c + d;
a = (a + b) / (c * d);
while (d++ = s++)
{
n++;
}
The expressions in a for statement should be separated by blank spaces. Example:
for (expr1; expr2; expr3)
Casts should be followed by a blank space. Examples:
MyFunction1((char) aNum, (double) x);
MyFunction2((int) (cp + 5), ((int) (i + 3) + 1);
1.10Naming Conventions
Naming conventions make programs more understandable by making them easier to read.
Internal, Informational |
13/17 |
2002/05/02 { 22:26:22

|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
C/C++ Coding Guidlines |
|||||
|
|
|
|
|
|
|
|||
Externally visible functions |
should have a short pre x uniquely iden- |
||||||||
|
|
|
|
tifying the module, followed by an under- |
|
||||
|
|
|
|
score, followed by the rest of the identi- |
|
||||
|
|
|
|
er which should consist of words, each of |
|
||||
|
|
|
|
which begins with a capital letter, with no |
|
||||
|
|
|
|
underscores. E.g. GAT |
|
FindResource. |
|
||
|
|
|
|
|
|||||
Static functions |
should follow the same convention as ex- |
|
|||||||
|
|
|
|
ternally visible functions, but need not |
|
||||
|
|
|
|
have the pre x. |
|
||||
|
|
|
|
|
|||||
Variable names |
should be short yet meaningful. The |
|
|||||||
|
|
|
|
choice of a variable name should be |
|
||||
|
|
|
|
mnemonicthat is, designed to indicate |
|
||||
|
|
|
|
to the casual observer the intent of its |
|
||||
|
|
|
|
use. One-character variable names should |
|
||||
|
|
|
|
be avoided except for temporary \throw- |
|
||||
|
|
|
|
away" variables. Common names for tem- |
|
||||
|
|
|
|
porary variables are i, j, k, m, and n for |
|
||||
|
|
|
|
integers; c, d, and e for characters. |
|
||||
|
|
|
|
|
|||||
#de nes |
should be all uppercase. |
|
|||||||
typedefs and structures |
should have a short pre x, followed by un- |
|
|||||||
|
|
|
|
derscore, followed by the rest of the iden- |
|
||||
|
|
|
|
ti er which should consist of words, each |
|
||||
|
|
|
|
of which begins with a capital letter, with |
|
||||
|
|
|
|
no underscores. E.g. GAT |
|
PropList. |
|
||
|
|
|
|
|
|
|
|
|
|
1.11Programming Practices
1.11.1Adherence to Standards
All code should adhere to the ISO C or C++ standards. The presence or absence of library functions not speci ed by the Posix standard on a particular platform should be detected by use of Autoconf and appropriate logic emplaced to either work around the absence or provide a good error message.
1.11.2Use typedef in preference to #de nes
New types should be introduced via typedefs rather than by #de nes { these types are then visible in debuggers and the compiler can do stronger type-checking.
1.11.3Use of const quali er
Where possible pointers should be passed using the const quali er. This is especially important for strings.
1.11.4Global and Static variables
Don't make any variable global or static without good reason. Access to module level statics in other les can often be granted via a function call rather than by making the variable global.
Internal, Informational |
14/17 |
2002/05/02 { 22:26:22

C/C++ Coding Guidlines
1.11.5Constants
Numerical constants (literals) should not be coded directly, except for -1, 0, and 1, or other numbers in loop counters.
1.11.6Variable Assignments
Avoid assigning several variables to the same value in a single statement. It is hard to read. Example:
fchar = lchar = 'c'; /* AVOID! */
Do not use the assignment operator in a place where it can be easily confused with the equality operator. Example:
if (file = fopen(...)) { /* AVOID! */
...
}
should be written as
if ((file = fopen(...)) != NULL)
{
...
}
Do not use embedded assignments in an attempt to improve run-time performance. This is the job of the compiler. Example:
d = (a = b + c) + r; /* AVOID! */
should be written as
a = b + c; d = a + r;
1.11.7Miscellaneous Practices
Parentheses It is generally a good idea to use parentheses liberally in expressions involving mixed operators to avoid operator precedence problems. Even if the operator precedence seems clear to you, it might not be to others-you shouldn't assume that other programmers know precedence as well as you do.
if (a == b && c == d) |
/* AVOID! */ |
|
if ((a == b) && (c == d)) /* RIGHT */ |
|
|
|
|
|
|
Internal, Informational |
15/17 |
2002/05/02 { 22:26:22

C/C++ Coding Guidlines
Returning Values Try to make the structure of your program match the intent. Example:
if (booleanExpression)
{
return true;
}
else
{
return false;
}
should instead be written as
return booleanExpression;
Similarly,
if (condition)
{
return x;
}
return y;
should be written as
return (condition ? x : y);
There should only be one return statement in a function. Multiple returns can lead to confusion when reading the code and is a freqent source of errors.
if(foo)
{
return a;
}
...
lots of code
...
return b;
should be written as
Internal, Informational |
16/17 |
2002/05/02 { 22:26:22

C/C++ Coding Guidlines
if(foo)
{
retval = a;
}
else
{
...
lots of code
...
retval = b;
}
return retval;
Expressions before `?' in the Conditional Operator If an expression containing a binary operator appears before the ? in the ternary ?: operator, it should be parenthesised. Example:
(x >= 0) ? x : -x;
Special Comments Use XXX in a comment to ag something that is bogus but works. Use FIXME to ag something that is bogus and broken.
Compilation with warnings enabled It is recommended that developers compile with all warnings enabled. Compiler warnings often ag dubious practices and common coding errors.
Internal, Informational |
17/17 |
2002/05/02 { 22:26:22