
Книги_AutoCad_1 / AutoCAD 2006 VBA_A Programmer’s Reference_Joe Sutphin__2005_
.pdf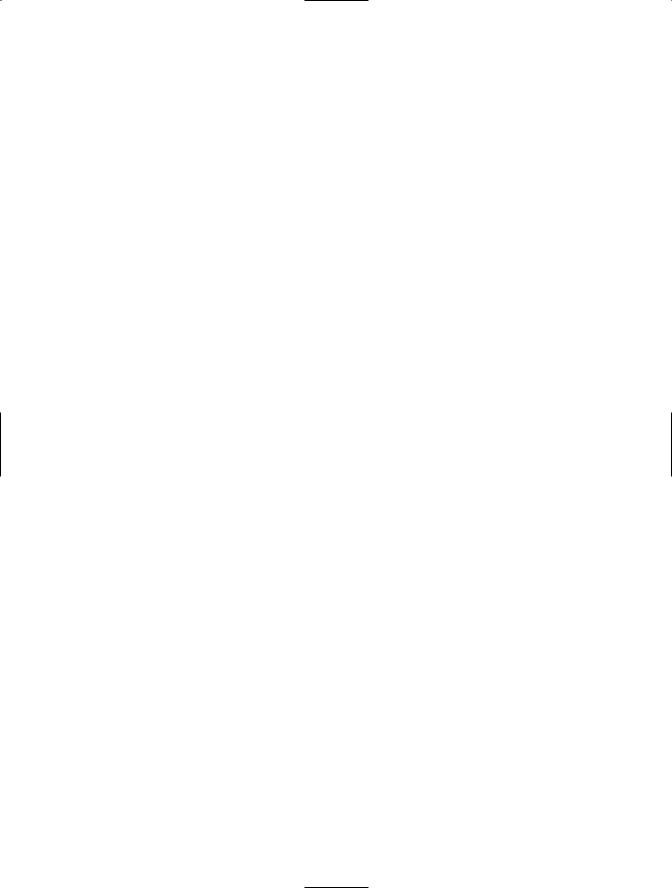
408 C H A P T E R 1 9 ■ C O N N E C T I N G TO E X T E R N A L A P P L I C AT I O N S
Setting the Document Header and Footer
You can set the document’s header and/or footer by setting the Text property of the Headers and/or Footers collection object like this:
oDocument.Sections(1).Headers(wdHeaderFooterPrimary) _
.Range.Text = "This is Header text"
or this:
oDocument.Sections(1).Footers(wdHeaderFooterPrimary) _
.Range.Text
Saving and Exiting Word
Use the SaveAs method of the Document object to save your document:
oDocument.SaveAs "Sample"
To close your document, use the Close method of the Documents collection object:
oWord.Documents("Sample.doc").Close True
Finally, use the Quit method of the Word.Application object to actually close the Word application, as shown here:
oWord.Quit
Connecting to a Microsoft Access Database
Most AutoCAD developers use Microsoft Access to create the database structure file, and then they use the Microsoft ActiveX Data Objects (ADO) library through VBA to access the .mdb file created by Access. You can use the same approach (and quite often the same code) to work with other data sources, such as Microsoft SQL Server, Oracle, DB2, and even Microsoft Exchange. This section details the steps necessary to connect to and access the data contained in a database file in a Microsoft Access application. Please refer to the same database file to follow along with these examples.
Connecting to a Database File
First, you need to add a reference to the Microsoft ActiveX Data Objects library, as shown in Figure 19-2.
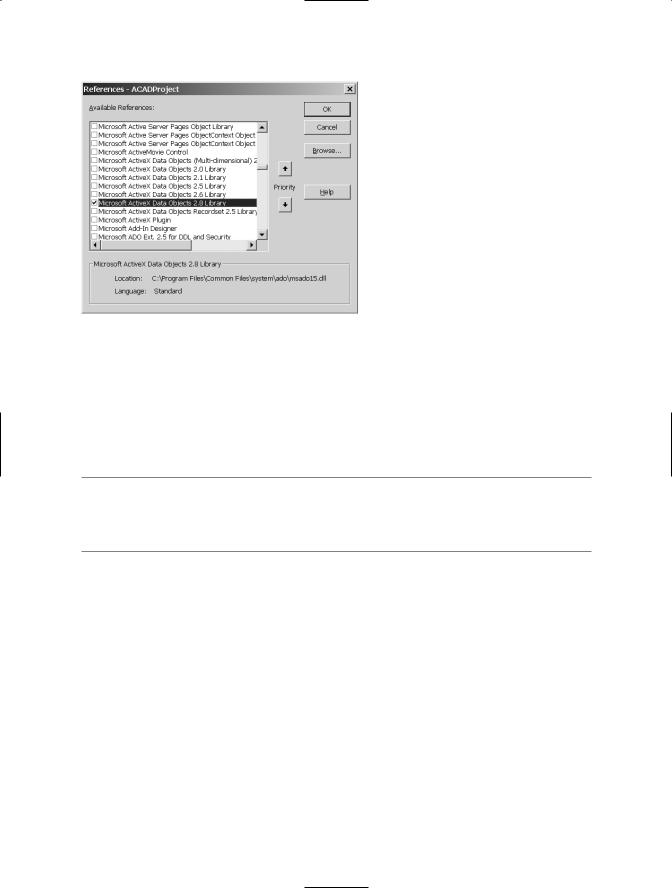
C H A P T E R 1 9 ■ C O N N E C T I N G TO E X T E R N A L A P P L I C AT I O N S |
409 |
Figure 19-2. Microsoft ActiveX Data Objects library reference
Next, you need to establish a Connection object similar to the following example:
Dim oAccess As New ADODB.Connection
oAccess.Open "Provider=Microsoft.Jet.OLEDB.4.0;" & _ "Data Source=" & "C:\AutoCAD-VBA.mdb" & ";"
■Note The example database for this exercise was created in Microsoft Access 2000. If you want to use a different file-format database, such as Access 97 or 2002, you’ll need to change the Provider string appropriately or use an ODBC data source name (DSN) to provide the interface connection directives.
Retrieving a Set of Records
The ability to retrieve the records stored in a database file is essential. The following example illustrates how to retrieve all the records from a table called Layers, and displays each record in a typical MsgBox:
'retrieve records from the Layers table Dim oRecordset As New ADODB.Recordset
oRecordset.Open "Select * From Layers", oAccess, adOpenKeyset
With oRecordset
Do While Not .EOF
MsgBox !LayerName & ", Color is " & !color
.MoveNext Loop
.Close End With
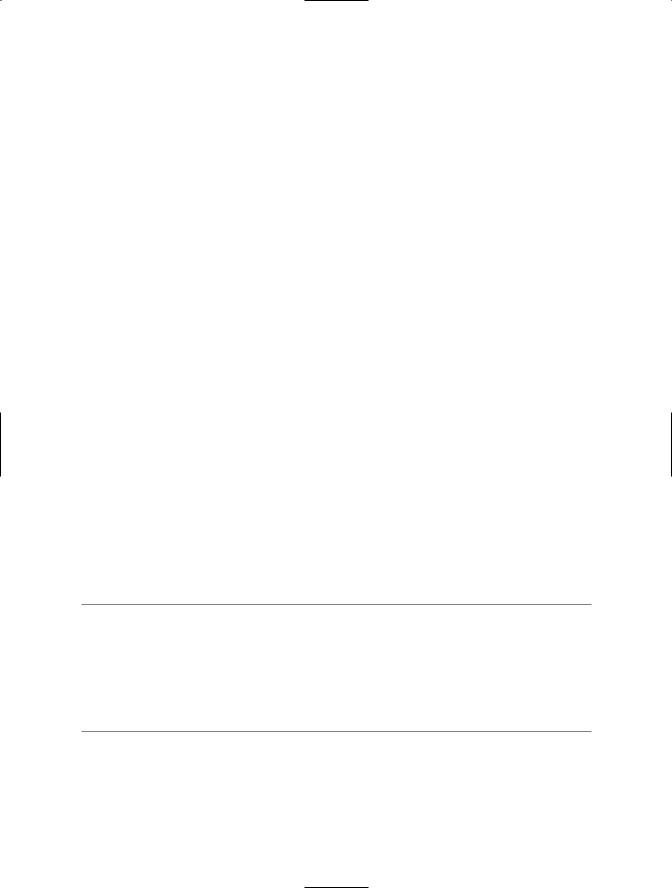
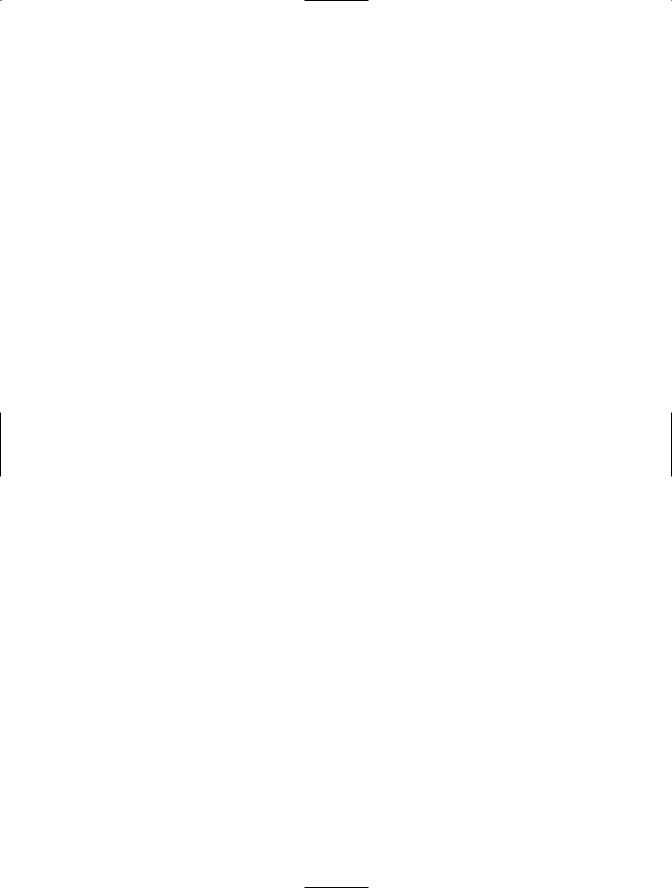
C H A P T E R 1 9 ■ C O N N E C T I N G TO E X T E R N A L A P P L I C AT I O N S |
411 |
desktop databases such as Access, dBASE, and FoxPro. You should be aware of the differences you’ll commonly encounter when you move data from one place to another, and between different database environments.
For example, the data type aspects can vary widely and you should never assume data types are consistent between such products as Microsoft Access, Microsoft SQL Server, DB2, and Oracle. For instance, when you convert databases in Access 2000 to SQL Server 2000, memo fields are converted into ntext or nvarchar fields, which are very different. Other common differences relate to date and time values, currency, and special fields such as Pictures, Hyperlinks, and BLOBs (binary large objects).
Connectivity Automation Objects
The dbConnect feature added in AutoCAD 2000 provides a powerful means for linking drawing entities to external data sources such as Excel spreadsheets, Access databases, and other ODBC data sources. It also provides a means for automatically labeling entities using Leader callouts with special control over the text labeling that is driven directly by linked values in the external data source.
This functionality is provided through a special API called Connectivity Automation Objects, or CAO. CAO is a very simple, compact object model, with a small set of objects, properties, methods, and so on (compared to AutoCAD, anyway). It exposes all of the power and capability of dbConnect to developers, both from within and from outside of AutoCAD. Like most ActiveX features in AutoCAD, you can employ CAO from VBA within AutoCAD, or from VBA in an external application.
Advanced Database Issues
Don’t be lulled into thinking that because you feel comfortable with the AutoCAD drawing “database” environment, you can translate it quickly into the world of database development. The differences are staggering. Although many similarities exist, you’ll encounter numerous issues in database development that aren’t present in AutoCAD. For example, referential integrity, normalization, indexing, views, and job scheduling are all common in mainstream database development. And although these topics may seem unimportant or remote to you as an AutoCAD developer, they’re crucial to database developers and the work they do every day.
If you aren’t a seasoned database developer, it often helps to partner with one while you’re learning the ropes of database programming. Although you can certainly learn database development skills, it’s truly a world unto its own. Finally, before you begin building a large, complex database application with AutoCAD, consult an experienced database developer to make sure you don’t reinvent the wheel or waste precious time building a solution that would have been easier or more robust if it were instead developed on the database end.
Working with Services and Other APIs
Like applications, services are processes that run within the operating-system-protected namespaces. Unlike applications, though, services normally don’t provide a graphical interface and are instead intended for under-the-hood purposes such as monitoring, logging, reacting, or protecting other system resources. Many services are very useful for making sure the overall environment is known.
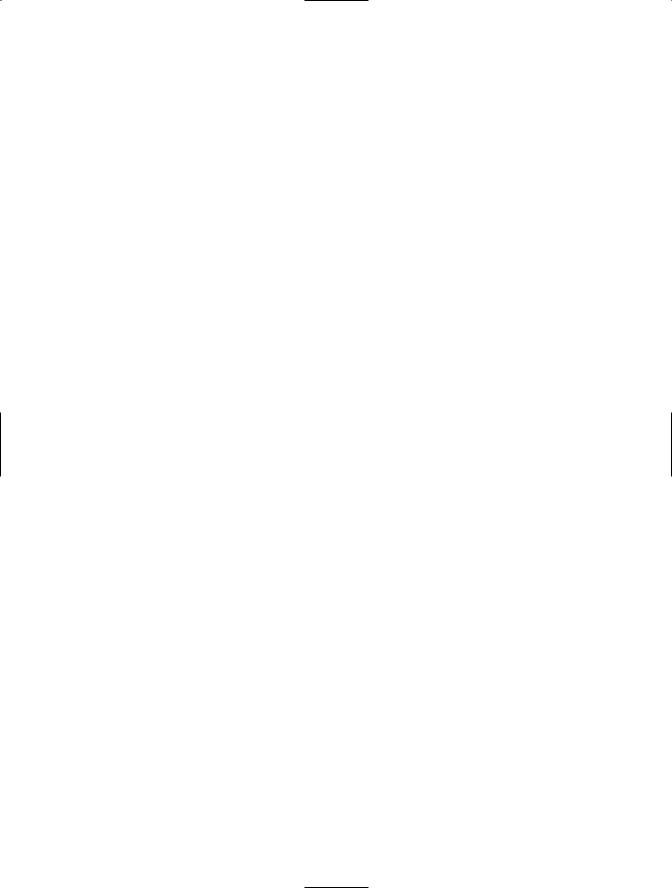
412 C H A P T E R 1 9 ■ C O N N E C T I N G TO E X T E R N A L A P P L I C AT I O N S
One service you might be interested in using is the Active Directory Service Interfaces (ADSI) object for accessing Active Directory Services on a Windows 2000 or 2003 network. An example of a commonly used API is the FileSystemObject (FSO), which is used by many applications to create, edit, read, and delete files, as well as perform file system operations on drives, folders, and so forth.
To find out the drive type and file system in use on the C: drive, for example, you could access the FSO Drive object for C: and then query its DriveType and FileSystem properties:
Function ShowDriveType(drvpath)
Dim fso, d, dt
Set fso = CreateObject("Scripting.FileSystemObject")
Set d = fso.GetDrive(drvpath)
Select Case d.DriveType
Case 0: dt = "Unknown"
Case 1: dt = "Removable"
Case 2: dt = "Fixed"
Case 3: dt = "Network"
Case 4: dt = "CD-ROM"
Case 5: dt = "RAM Disk"
End Select
ShowDriveType = dt
End Function
Function ShowFileSystemType(drvspec)
Dim fso,d
Set fso = CreateObject("Scripting.FileSystemObject")
Set d = fso.GetDrive(drvspec)
ShowFileSystemType = d.FileSystem
End Function
MsgBox "C: Drive Type is " & ShowDriveType("C")
MsgBox "C: File System is " & ShowFileSystemType("C")
The ADSI object is built into Windows 2000, Windows XP Professional, and corresponding server versions of these products. It provides an API for interfacing with directory services such as Lightweight Directory Access Protocol (LDAP) and Active Directory. A common task might be to retrieve a list of all the users, groups, or computers on your domain. To do this, you could invoke the ADSI interface to the WinNT object, as shown here:
Sub ShowAllComputers()
Dim adsDomain
Set adsDomain = GetObject("WinNT://MyDomainName")
For each objMember in adsDomain
If Ucase(objMember.Class) = "COMPUTER" Then
Debug.Print objMember.Name & vbCrLf
End If
Next
Set adsDomain = Nothing
End Sub
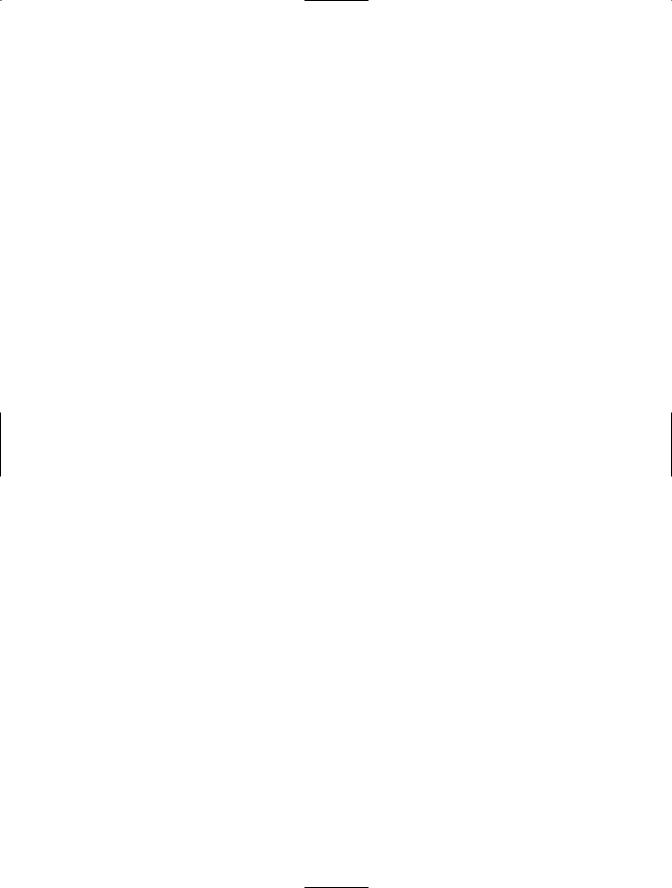
C H A P T E R 1 9 ■ C O N N E C T I N G TO E X T E R N A L A P P L I C AT I O N S |
413 |
You might have noticed in the preceding code that GetObject is used instead of CreateObject. This is because a true service is always available through the GetObject interface and doesn’t require instantiation to obtain an instance.
The FSO, ADSI, and LanManServer API references are all published on the MSDN Web site, as are many other useful APIs and services such as WMI/WBEM, CDO/CDONTS, LDAP, and Windows Scripting Host (WSH).
Summary
This chapter briefly touched upon the capabilities that VBA provides for working with external applications; it’s by no means exhaustive. You can interface with an almost limitless number of external applications, services, and processes from within AutoCAD VBA. The more you look and experiment, the more you’ll be amazed at what you can do.
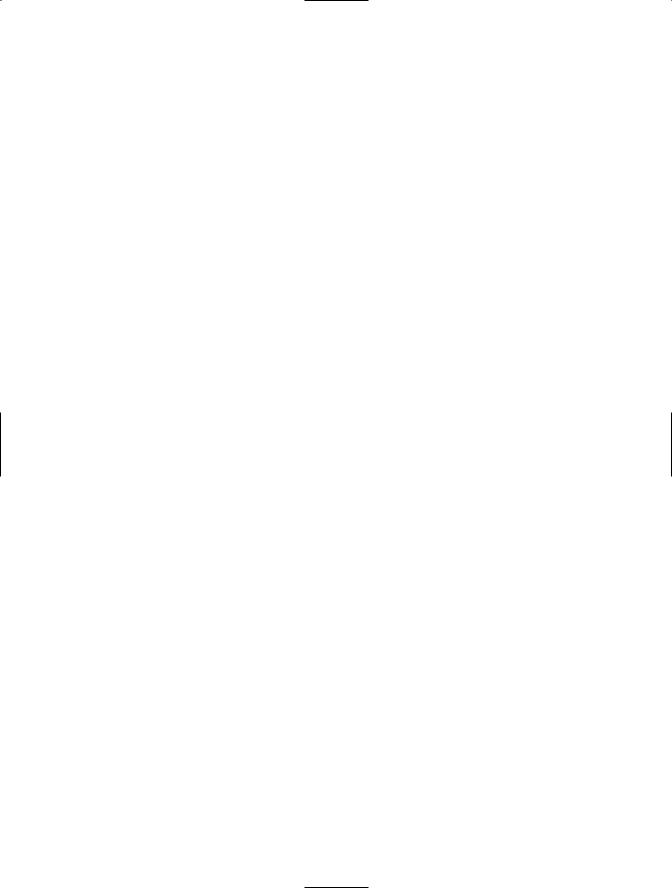
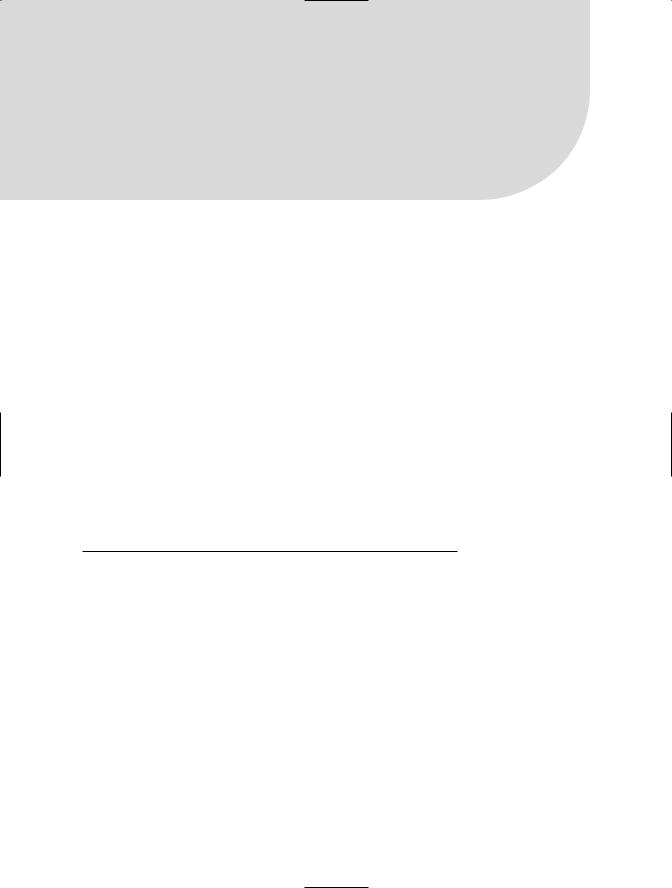
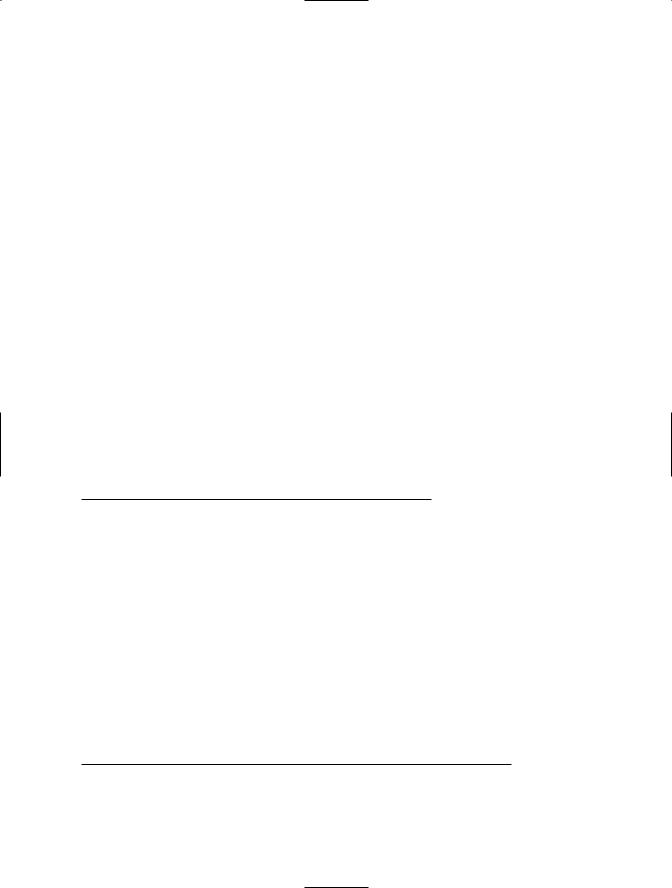
416 C H A P T E R 2 0 ■ C R E AT I N G TA B L E S
On Error Resume Next
With ThisDrawing.Utility
vInsertionPoint = .GetPoint(, vbCr & "Pick the insertion point: ") lNumberOfRows = .GetInteger(vbCr & "Enter number of rows: ") lNumberOfColumns = .GetInteger(vbCr & "Enter number of columns: ") dRowHeight = .GetReal(vbCr & "Enter row height: ")
dColumnWidth = .GetReal(vbCr & "Enter column width: ") End With
If Err Then Exit Sub
Set oTable = ThisDrawing.ModelSpace.AddTable(vInsertionPoint, _ lNumberOfRows, lNumberOfColumns, dRowHeight, dColumnWidth)
End Sub
The RegenerateTableSuppressed Property
The RegenerateTableSuppressed property specifies whether the table will be repainted each time a change to the table is made.
object.RegenerateTableSuppressed
Table 20-2 shows the RegenerateTableSuppressed property’s parameters.
Table 20-2. The RegenerateTableSuppressed Property’s Parameters
Value |
Description |
True |
The table regeneration is suppressed or off. |
False |
The table regeneration is not suppressed or on. |
|
|
The GetText Method
Text within a Table can be read using the GetText method to retrieve the text string value of a specified row and column of an existing table.
The GetText method returns a string and has the following syntax:
object.GetText Row, Column
Table 20-3 explains this method’s parameters.
Table 20-3. The GetText Method’s Parameters
Name |
Data Type |
Description |
Row |
Long |
A zero-based row index for the cell containing text |
Column |
Long |
A zero-based column index for the cell containing text |
|
|
|
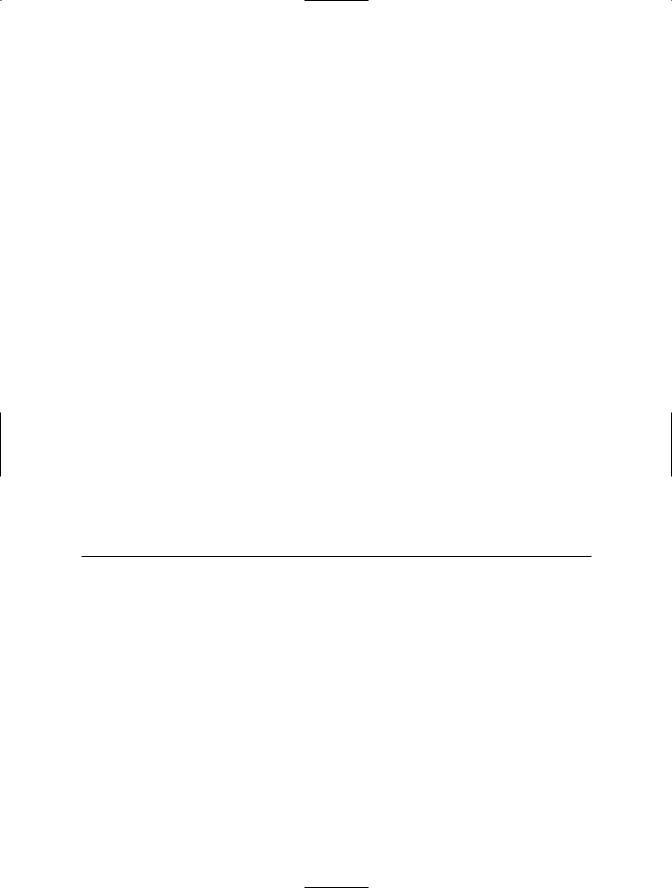