
- •Table of Contents
- •About the Author
- •About the Technical Reviewer
- •Acknowledgments
- •Introduction
- •Installing Visual Studio
- •Visual Studio 2022 System Requirements
- •Operating Systems
- •Hardware
- •Supported Languages
- •Additional Notes
- •Visual Studio Is 64-Bit
- •Full .NET 6.0 Support
- •Using Workloads
- •The Solution Explorer
- •Toolbox
- •The Code Editor
- •New Razor Editor
- •What’s Available?
- •Hot Reload
- •Navigating Code
- •Navigate Forward and Backward Commands
- •Navigation Bar
- •Find All References
- •Find Files Faster
- •Reference Highlighting
- •Peek Definition
- •Subword Navigation
- •Features and Productivity Tips
- •Track Active Item in Solution Explorer
- •Hidden Editor Context Menu
- •Open in File Explorer
- •Finding Keyboard Shortcut Mappings
- •Clipboard History
- •Go To Window
- •Navigate to Last Edit Location
- •Multi-caret Editing
- •Sync Namespaces to Match Your Folder Structure
- •Paste JSON As Classes
- •Enable Code Cleanup on Save
- •Add Missing Using on Paste
- •Features in Visual Studio 2022
- •Visual Studio Search
- •Solution Filters
- •Visual Studio IntelliCode
- •Whole Line Completions
- •Visual Studio Live Share
- •Summary
- •Visual Studio Project Types
- •Various Project Templates
- •Console Applications
- •Windows Forms Application
- •Windows Service
- •Web Applications
- •Class Library
- •MAUI
- •Creating a MAUI Application
- •Pairing to Mac for iOS Development
- •Consuming REST Services in MAUI
- •The Complete Weather App
- •The Target Platforms
- •The Required NuGet Package
- •The Weather Models
- •The WeatherService
- •The MainViewModel
- •Registering Dependencies
- •Building the MainPage View
- •Using SQLite in a MAUI Application
- •The ToDoItem Model
- •The ToDoService
- •The MainViewModel
- •Registering Dependencies
- •Building the MainPage View
- •Managing NuGet Packages
- •Using NuGet in Visual Studio
- •Hosting Your Own NuGet Feeds
- •Managing nmp Packages
- •Creating Project Templates
- •Creating and Using Code Snippets
- •Creating Code Snippets
- •Using Bookmarks and Code Shortcuts
- •Bookmarks
- •Code Shortcuts
- •Adding Custom Tokens
- •The Server Explorer
- •Running SQL Queries
- •Visual Studio Windows
- •C# Interactive
- •Code Metrics Results
- •Maintainability Index
- •Cyclomatic Complexity
- •Class Coupling
- •Send Feedback
- •Personalizing Visual Studio
- •Adjust Line Spacing
- •Document Management Customizations
- •The Document Close Button
- •Modify the Dirty Indicator
- •Show Invisible Tabs in Italics in the Tab Drop-Down
- •Colorize Document Tabs
- •Tab Placement
- •Visual Studio Themes
- •Summary
- •Setting a Breakpoint
- •Step into Specific
- •Run to Click
- •Run to Cursor
- •Force Run to Cursor
- •Conditional Breakpoints and Actions
- •Temporary Breakpoints
- •Dependent Breakpoints
- •Dragging Breakpoints
- •Manage Breakpoints with Labels
- •Exporting Breakpoints
- •Using DataTips
- •Visualizing Complex Data Types
- •Bonus Tip
- •Using the Watch Window
- •The DebuggerDisplay Attribute
- •Evaluate Functions Without Side Effects
- •Format Specifiers
- •dynamic
- •hidden
- •results
- •Diagnostic Tools
- •CPU Usage
- •Memory Usage
- •The Events View
- •The Right Tool for the Right Project Type
- •Immediate Window
- •Attaching to a Running Process
- •Attach to a Remote Process
- •Remote Debugger Port Assignments
- •Remote Debugging
- •System Requirements
- •Download and Install Remote Tools
- •Running Remote Tools
- •Start Remote Debugging
- •Summary
- •Creating and Running Unit Tests
- •Create and Run a Test Playlist
- •Testing Timeouts
- •Using Live Unit Tests
- •Using IntelliTest to Generate Unit Tests
- •Focus IntelliTest Code Exploration
- •How to Measure Code Coverage in Visual Studio
- •Summary
- •Create a GitHub Account
- •Create and Clone a Repository
- •Create a Branch from Your Code
- •Creating and Handling Pull Requests
- •Multi-repo Support
- •Compare Branches
- •Check Out Commit
- •Line Staging
- •Summary
- •Index
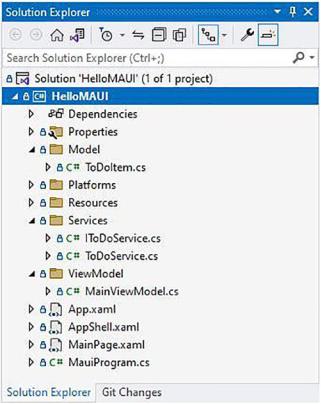
Chapter 2 Working with Visual Studio 2022
All the moving parts should now be hooked up for our weather application to pull data from the API and return the weather information for Los Angeles, including the ten-day forecast. The next topic that I want to touch on is using SQLite in your MAUI application.
Using SQLite in a MAUI Application
In the previous section, we looked at how to consume an API in a MAUI application. For that reason, I will not step through all the details of this application. I reused the existing weather service application and ripped out everything not applicable to the SQLite functionality we wanted to implement. The SQLite app is a simple to-do application, and the solution is seen in Figure 2-27.
Figure 2-27. The SQLite project structure
97
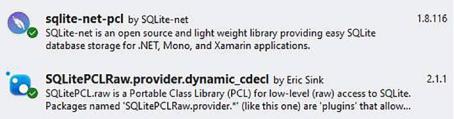
Chapter 2 Working with Visual Studio 2022
As before, I have a Services folder containing the ToDoService and its IToDoService Interface. I have a Model folder containing the ToDoItem class. Lastly, I have the ViewModel folder with the MainViewModel class. You can see that the project structure follows the same pattern as before. The only difference here is that in addition to the Community Toolkit, I have added additional NuGet packages for SQLite, as seen in Figure 2-28.
Figure 2-28. The SQLite NuGet packages
In the next section, let’s start with creating the model for a to-do item.
The ToDoItem Model
After adding the SQLite NuGet package, I can create the ToDoItem model as seen in Listing 2-11.
Listing 2-11. The ToDoItem Model
using SQLite;
namespace HelloMAUI.Model
{
[Table("todo")] public class ToDoItem
{
[PrimaryKey, AutoIncrement] public int Id { get; set; }
[MaxLength(250)]
public string ToDoText { get; set; }
}
}
98
Chapter 2 Working with Visual Studio 2022
I can now bring in the SQLite namespace, which allows me to add SQLite-specific attributes to my model. This model maps to a table in SQLite called todo because I have added the [Table("todo")] attribute to my class. I can now define additional
attributes on my model properties, in this case, specifying that my Id property is an autoincrementing primary key and that the text that contains the to-do item cannot be longer than 250 characters.
The ToDoService
As with the weather application’s service, we are creating an Interface called IToDoService. The code is in Listing 2-12.
Listing 2-12. The IToDoService Interface
using HelloMAUI.Model;
namespace HelloMAUI.Services
{
public interface IToDoService
{
Task Init();
Task AddToDo(string todoItem); Task<IEnumerable<ToDoItem>> GetToDoItems();
}
}
This defines a contract for each implementing class to provide an implementation for initializing the database, adding to-do items, and reading all the to-do items.
Listing 2-13. The ToDoService
using HelloMAUI.Model; using SQLite;
namespace HelloMAUI.Services
{
public class ToDoService : IToDoService
99
Chapter 2 Working with Visual Studio 2022
{
private SQLiteAsyncConnection _db; readonly string _dbPath;
public ToDoService()
{
_dbPath = Path.Combine(Environment.GetFolderPath(Environment. SpecialFolder.MyDocuments), "mydb.db");
}
public async Task Init()
{
if (_db != null) return;
_db = new SQLiteAsyncConnection(_dbPath); _ = await _db.CreateTableAsync<ToDoItem>();
}
public async Task AddToDo(string todoText)
{
await Init();
var todoItem = new ToDoItem
{
ToDoText = todoText
};
var result = await _db.InsertAsync(todoItem);
}
public async Task<IEnumerable<ToDoItem>> GetToDoItems()
{
await Init();
return await _db.Table<ToDoItem>().ToListAsync();
}
}
}
100