
- •Table of Contents
- •About the Author
- •About the Technical Reviewer
- •Acknowledgments
- •Introduction
- •What is .NET MAUI?
- •Digging a Bit Deeper
- •Where Did It Come From?
- •How It Differs From the Competition
- •Why Use .NET MAUI?
- •Supported Platforms
- •Code Sharing
- •Developer Freedom
- •Community
- •Fast Development Cycle
- •.NET Hot Reload
- •XAML Hot Reload
- •Performance
- •Strong Commercial Offerings
- •Limitations of .NET MAUI
- •No Web Assembly (WASM) Support
- •No Camera API
- •Apps Won’t Look Identical on Each Platform
- •Lack of Media Playback Out of the Box
- •The Glass Is Half Full, Though
- •How to Build .NET MAUI Applications
- •Visual Studio
- •Visual Studio (Windows)
- •Visual Studio for Mac
- •Rider
- •Visual Studio Code
- •Summary
- •Setting Up Your Environment
- •macOS
- •Visual Studio for Mac
- •Xcode
- •Remote Access
- •Windows
- •Visual Studio
- •Visual Studio to macOS
- •Troubleshooting Installation Issues
- •.NET MAUI Workload Is Missing
- •Visual Studio Installer
- •Command Line
- •Creating Your First Application
- •Creating in Visual Studio
- •Creating in the Command Line
- •Building and Running Your First Application
- •Getting to Know Your Application
- •WidgetBoard
- •Summary
- •Source Code
- •Project Structure
- •/Platforms/ Folder
- •Android
- •MacCatalyst
- •Tizen
- •Windows
- •Summary
- •/Resources/ Folder
- •Fonts
- •Images
- •Generic Host Builder
- •What Is Dependency Injection?
- •Registering Dependencies
- •AddSingleton
- •AddTransient
- •AddScoped
- •Application Lifecycle
- •Application States
- •Lifecycle Events
- •Handling Lifecycle Events
- •Cross-Platform Mappings to Platform Lifecycle Events
- •Platform-Specific Lifecycle Events
- •Android
- •Windows
- •Summary
- •A Measuring Stick
- •Prerequisites
- •Model View ViewModel (MVVM)
- •Model
- •View
- •XAML
- •C# (Code-Behind)
- •ViewModel
- •Model View Update (MVU)
- •Getting Started with Comet
- •Adding Your MVU Implementation
- •XAML vs. C# Markup
- •Plain C#
- •C# Markup
- •Chosen Architecture for This Book
- •Adding IWidgetViewModel
- •Adding BaseViewModel
- •Adding ClockWidgetViewModel
- •Adding Views
- •Adding IWidgetView
- •Adding ClockWidgetView
- •Viewing Your Widget
- •Modifying MainPage.xaml
- •Modifying MainPage.xaml.cs
- •Taking the Application for a Spin
- •MVVM Enhancements
- •MVVM Frameworks
- •Magic
- •Summary
- •Source Code
- •Prerequisites
- •Models
- •BaseLayout.cs
- •FixedLayout.cs
- •Board.cs
- •Pages
- •BoardDetailsPage
- •FixedBoardPage
- •ViewModels
- •AppShellViewModel
- •BoardDetailsPageViewModel
- •FixedBoardPageViewModel
- •App Icons
- •Adding Your Own Icon
- •Platform Differences
- •Android
- •Splash Screen
- •XAML
- •Dissecting a XAML File
- •Building Your First XAML Page
- •Layouts
- •AbsoluteLayout
- •FlexLayout
- •Grid
- •HorizontalStackLayout
- •VerticalStackLayout
- •Data Binding
- •Binding
- •BindingContext
- •Path
- •Mode
- •Source
- •Applying the Remaining Bindings
- •MultiBinding
- •Command
- •Compiled Bindings
- •Shell
- •ShellContent
- •Navigation
- •Registering Pages for Navigation
- •Performing Navigation
- •Navigating Backwards
- •Passing Data When Navigating
- •Flyout
- •FlyoutHeader
- •FlyoutContent
- •Selected Board
- •Navigation to the Selected Board
- •Setting the BindingContext of Your AppShell
- •Register AppShell with the MAUI App Builder
- •Resolve the AppShell Instead of Creating It
- •Tabs
- •Search
- •Taking Your Application for a Spin
- •Summary
- •Source Code
- •Extra Assignment
- •Placeholder
- •ILayoutManager
- •BoardLayout
- •BoardLayout.xaml
- •BindableLayout
- •BoardLayout.xaml.cs
- •Adding the LayoutManager Property
- •Adding the ItemsSource Property
- •Adding the ItemTemplateSelector Property
- •Handling the ChildAdded Event
- •Adding Remaining Bits
- •FixedLayoutManager
- •Accepting the Number of Rows and Columns for a Board
- •Adding the NumberOfColumns Property
- •Adding the NumberOfRows Property
- •Building the Board Layout
- •Setting the Correct Row/Column Position for Each Widget
- •Using Your Layout
- •Allowing for the Registration of Widget Views and View Models
- •Creation of a Widget View
- •Creation of a Widget View Model
- •Registering the Factory with MauiAppBuilder
- •Registering Your ClockWidget with the Factory
- •WidgetTemplateSelector
- •Registering the Template Selector with MauiAppBuilder
- •Updating FixedBoardPageViewModel
- •Finally Using the Layout
- •Summary
- •Source Code
- •Extra Assignment
- •What Is Accessibility?
- •Why Make Your Applications Accessible?
- •What to Consider When Making Your Applications Accessible
- •How to Make Your Application Accessible
- •Screen Reader Support
- •SemanticProperties
- •SemanticProperties.Description
- •SemanticProperties.Hint
- •SemanticProperties.HeadingLevel
- •SemanticScreenReader
- •AutomationProperties
- •AutomationProperties.ExcludedWithChildren
- •AutomationProperties.IsInAccessibleTree
- •Suitable Contrast
- •Dynamic Text Sizing
- •Avoiding Fixed Sizes
- •Preferring Minimum Sizing
- •Font Auto Scaling
- •Testing Your Application’s Accessibility
- •Android
- •macOS
- •Windows
- •Accessibility Checklist
- •Summary
- •Source Code
- •Extra Assignment
- •Adding the Ability to Add a Widget to a Board
- •Possible Ways of Achieving Your Goal
- •Showing a Modal Page
- •The Chosen Approach
- •Adding Your Overlay View
- •Updating Your View Model
- •Showing the Overlay View
- •Styling
- •Examining the Default Styles
- •TargetType
- •ApplyToDerivedTypes
- •Setter
- •AppThemeBinding
- •Further Reading
- •Triggers
- •Creating ShowOverlayTriggerAction
- •Using ShowOverlayTriggerAction
- •Further Reading
- •Animations
- •Basic Animations
- •Combining Basic Animations
- •Chaining Animations
- •Concurrent Animations
- •Cancelling Animations
- •Easings
- •Complex Animations
- •Recreating the ScaleTo Animation
- •Creating a Rubber Band Animation
- •Combining Triggers and Animations
- •Summary
- •Source Code
- •Extra Assignment
- •Animate the BoxView Overlay
- •Animate the New Widget
- •What Is Local Data?
- •File System
- •Cache Directory
- •App Data Directory
- •Database
- •Repository Pattern
- •Listing Your Boards
- •SQLite
- •Installing SQLite-net
- •Using Sqlite-net
- •Connecting to an SQLite database
- •Mapping Your Models
- •Creating Your Tables
- •Inserting into an SQLite Database
- •Reading a Collection from an SQLite Database
- •Reading a Single Entity from an SQLite Database
- •Deleting from an SQLite Database
- •Updating an Entity in an SQLite Database
- •LiteDB
- •Installing LiteDB
- •Using LiteDB
- •Connecting to a LiteDB database
- •Mapping Your Models
- •Creating Your Tables
- •Inserting into a LiteDB Database
- •Reading a Collection from a LiteDB Database
- •Reading a Single Entity from a LiteDB Database
- •Deleting from a LiteDB Database
- •Updating an Entity in a LiteDB Database
- •Database Summary
- •Application Settings (Preferences)
- •What Can Be Stored in Preferences?
- •Setting a Value in Preferences
- •Getting a Value in Preferences
- •Checking if a Key Exists in Preferences
- •Secure Storage
- •Storing a Value Securely
- •Reading a Secure Value
- •Removing a Secure Value
- •Platform specifics
- •Android
- •Windows
- •Summary
- •Source Code
- •Extra Assignment
- •What Is Remote Data?
- •Considerations When Handling Remote Data
- •Loading Times
- •Failures
- •Security
- •Webservices
- •The Open Weather API
- •Creating an Open Weather Account
- •Creating an Open Weather API key
- •Using System.Text.Json
- •Creating Your Models
- •Connecting to the Open Weather API
- •Registering Your Widget
- •Testing Your Widget
- •Adding Some State
- •Converting the State to UI
- •Displaying the Loading State
- •Displaying the Loaded State
- •Displaying the Error State
- •Simplifying Webservice Access
- •Prebuilt Libraries
- •Code Generation Libraries
- •Adding the Refit NuGet Package
- •Further Reading
- •Polly
- •Summary
- •Source Code
- •Extra Assignment
- •TODO Widget
- •Quote of the Day Widget
- •NASA Space Image of the Day Widget
- •.NET MAUI Essentials
- •Permissions
- •Checking the Status of a Permission
- •Requesting Permission
- •Handling Permissions in Your Application
- •Using the Geolocation API
- •Registering the Geolocation Service
- •Using the Geolocation Service
- •Displaying Permission Errors to Your User
- •Configuring Platform-Specific Components
- •Android
- •Windows
- •Platform-Specific API Access
- •Platform-Specific Code with Compiler Directives
- •Platform-Specific Code in Platform Folders
- •Overriding the Platform-Specific UI
- •OnPlatform
- •OnPlatform Markup Extension
- •Conditional Statements
- •Handlers
- •Customizing Controls with Mappers
- •Scoping of Mapper Customization
- •Further Reading
- •Summary
- •Source Code
- •Extra Assignment
- •Barometer Widget
- •Geocoding Lookup
- •Unit Testing
- •Unit Testing in .NET MAUI
- •xUnit
- •NUnit
- •MSTest
- •Your Chosen Testing Framework
- •Adding Your Own Unit Tests
- •Adding a Unit Test Project to Your Solution
- •Modify Your Application Project to Target net7.0
- •Adding a Reference to the Project to Test
- •Modify Your Test Project to Use MAUI Dependencies
- •Testing Your View Models
- •Testing BoardDetailsPageViewModel
- •Testing INotifyPropertyChanged
- •Testing Asynchronous Operations
- •Creating Your ILocationService Mock
- •Creating Your WeatherForecastService Mock
- •Creating Your Asynchronous Tests
- •Testing Your Views
- •Creating Your ClockWidgetViewModel Mock
- •Creating Your View Tests
- •Device Testing
- •Creating a Device Test Project
- •Adding a Device-Specific Test
- •Running Device-Specific Tests
- •Snapshot Testing
- •Snapshot Testing Your Application
- •Passing Thoughts
- •Summary
- •Source Code
- •.NET MAUI Graphics
- •Maintaining the State of the Canvas
- •Further Reading
- •Building a Sketch Widget
- •Representing a User Interaction
- •Registering Your Widget
- •Taking Your Widget for a Test Draw
- •Summary
- •Source Code
- •Extra Assignment
- •Distributing Your Application
- •Android
- •Additional Resources
- •Certificate
- •Identifier
- •Capabilities
- •Entitlements
- •Provisioning Profiles
- •Additional Resources
- •macOS
- •Additional Resources
- •Windows
- •Additional Resources
- •Following Good Practices
- •Performance
- •Startup Tracing
- •Image Sizes
- •Linking
- •What Is Linking?
- •Issues That Crop Up
- •Crashes/Analytics
- •Sentry
- •App Center
- •Obfuscation
- •Distributing Test Versions
- •Summary
- •Looking at the Final Product
- •Taking the Project Further
- •Useful Resources
- •StackOverflow
- •GitHub
- •YouTube
- •Gerald Versluis
- •James Montemagno
- •Social Media
- •Yet More Goodness
- •Looking Forward
- •Comet
- •Testing
- •Index
Chapter 8 Advanced UI Concepts
<DataTrigger.ExitActions>
<triggers:ShowOverlayTriggerAction ShowOverlay="False" />
</DataTrigger.ExitActions>
</DataTrigger>
This can now be interpreted as, when the IsAddingWidget property value changes to true, a ShowOverlayTriggerAction will be invoked with ShowOverlay set to true. This will result in the AddWidgetFrame control becoming visible. Then, when the IsAddingWidget property value changes to false, a ShowOverlayTriggerAction will be invoked with ShowOverlay set to false. This will result in the AddWidgetFrame control becoming invisible.
It is also worth noting that you can define triggers in styles, meaning this type of functionality can be reused multiple times without having to duplicate the code.
Let’s take a break from triggers for now to take a look at how you can animate controls in .NET MAUI. Then you will return and combine triggers and animations together to really show off the power of the action you just created.
Further Reading
You have only scratched the surface on the functionality that can be achieved with triggers. I recommend checking out the
Microsoft documentation to see more ways triggers can be useful: https://learn.microsoft.com/dotnet/maui/fundamentals/triggers.
Animations
This feels like it could be a challenging topic to show off in printed form given the dynamic nature of an animation but it is one of my favorite topics so I am going to show it off as best I can. Animations provide you with the building blocks to make your applications feel much more natural and organic.
242

Chapter 8 Advanced UI Concepts
.NET MAUI provides two main ways to perform an animation against any VisualElement. You will take a look at each approach and how some animations can be built using them.
Basic Animations
.NET MAUI ships with a set of prebuilt animations available via extension methods. These methods provide the ability to rotate, translate, scale, and fade a VisualElement over a period of time. Each of these methods have a To suffix, for example ScaleTo. It is worth noting that each of the methods for animating are asynchronous and will therefore need to be awaited
if you wish to know when they have finished. The full list of animation methods are as follows:
Method |
Description |
|
|
FadeTo |
Animates the Opacity property of a VisualElement |
RelScaleTo |
Applies an animated incremental increase or decrease to the |
|
Scale property of a VisualElement |
RotateTo |
Animates the Rotation property of a VisualElement |
RelRotateTo |
Applies an animated incremental increase or decrease to the |
|
Rotation property of a VisualElement |
RotateXTo |
Animates the RotationX property of a VisualElement |
RotateYTo |
Animates the RotationY property of a VisualElement |
ScaleTo |
Animates the Scale property of a VisualElement |
ScaleXTo |
Animates the ScaleX property of a VisualElement |
ScaleYTo |
Animates the ScaleY property of a VisualElement |
TranslateTo |
Animates the TranslationX and TranslationY properties of |
|
a VisualElement |
|
|
243
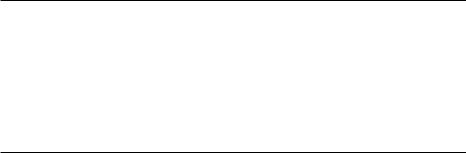
Chapter 8 Advanced UI Concepts
The overlay view you added in the previous section just shows immediately and disappears immediately based on the IsVisible binding you created. What if you animate your overlay to grow from nothing up to the required size? Don’t worry about adding this code to your application just yet. You will look over some examples and then add it to Visual Studio in the “Combining Triggers and Animations” section. The main reason for not adding it immediately is because the animations API relies on direct access to the view-related information, and this breaks the MVVM pattern. However, once you look over how to animate, you can take this learning and add it into your ShowOverlayTriggerAction implementation.
The code to animate a VisualElement is surprisingly small, as you can see in the following example:
AddWidgetFrame.Scale = 0;
await AddWidgetFrame.ScaleTo(1, 500);
First, you make sure that the AddWidgetFrame has a Scale of 0 and then you call ScaleTo, telling it to grow to a Scale of 1 (which is 100%) over a duration of 500 milliseconds.
All of the prebuilt animation methods apart from the ones that start with Rel perform the animation against the VisualElements existing value (e.g., for ScaleTo it will change from the existing Scale property value). This means that it is entirely possible that no animation will take place if both the existing property and the value provided to the method are the same.
244