
- •Table of Contents
- •About the Author
- •About the Technical Reviewer
- •Acknowledgments
- •Introduction
- •What is .NET MAUI?
- •Digging a Bit Deeper
- •Where Did It Come From?
- •How It Differs From the Competition
- •Why Use .NET MAUI?
- •Supported Platforms
- •Code Sharing
- •Developer Freedom
- •Community
- •Fast Development Cycle
- •.NET Hot Reload
- •XAML Hot Reload
- •Performance
- •Strong Commercial Offerings
- •Limitations of .NET MAUI
- •No Web Assembly (WASM) Support
- •No Camera API
- •Apps Won’t Look Identical on Each Platform
- •Lack of Media Playback Out of the Box
- •The Glass Is Half Full, Though
- •How to Build .NET MAUI Applications
- •Visual Studio
- •Visual Studio (Windows)
- •Visual Studio for Mac
- •Rider
- •Visual Studio Code
- •Summary
- •Setting Up Your Environment
- •macOS
- •Visual Studio for Mac
- •Xcode
- •Remote Access
- •Windows
- •Visual Studio
- •Visual Studio to macOS
- •Troubleshooting Installation Issues
- •.NET MAUI Workload Is Missing
- •Visual Studio Installer
- •Command Line
- •Creating Your First Application
- •Creating in Visual Studio
- •Creating in the Command Line
- •Building and Running Your First Application
- •Getting to Know Your Application
- •WidgetBoard
- •Summary
- •Source Code
- •Project Structure
- •/Platforms/ Folder
- •Android
- •MacCatalyst
- •Tizen
- •Windows
- •Summary
- •/Resources/ Folder
- •Fonts
- •Images
- •Generic Host Builder
- •What Is Dependency Injection?
- •Registering Dependencies
- •AddSingleton
- •AddTransient
- •AddScoped
- •Application Lifecycle
- •Application States
- •Lifecycle Events
- •Handling Lifecycle Events
- •Cross-Platform Mappings to Platform Lifecycle Events
- •Platform-Specific Lifecycle Events
- •Android
- •Windows
- •Summary
- •A Measuring Stick
- •Prerequisites
- •Model View ViewModel (MVVM)
- •Model
- •View
- •XAML
- •C# (Code-Behind)
- •ViewModel
- •Model View Update (MVU)
- •Getting Started with Comet
- •Adding Your MVU Implementation
- •XAML vs. C# Markup
- •Plain C#
- •C# Markup
- •Chosen Architecture for This Book
- •Adding IWidgetViewModel
- •Adding BaseViewModel
- •Adding ClockWidgetViewModel
- •Adding Views
- •Adding IWidgetView
- •Adding ClockWidgetView
- •Viewing Your Widget
- •Modifying MainPage.xaml
- •Modifying MainPage.xaml.cs
- •Taking the Application for a Spin
- •MVVM Enhancements
- •MVVM Frameworks
- •Magic
- •Summary
- •Source Code
- •Prerequisites
- •Models
- •BaseLayout.cs
- •FixedLayout.cs
- •Board.cs
- •Pages
- •BoardDetailsPage
- •FixedBoardPage
- •ViewModels
- •AppShellViewModel
- •BoardDetailsPageViewModel
- •FixedBoardPageViewModel
- •App Icons
- •Adding Your Own Icon
- •Platform Differences
- •Android
- •Splash Screen
- •XAML
- •Dissecting a XAML File
- •Building Your First XAML Page
- •Layouts
- •AbsoluteLayout
- •FlexLayout
- •Grid
- •HorizontalStackLayout
- •VerticalStackLayout
- •Data Binding
- •Binding
- •BindingContext
- •Path
- •Mode
- •Source
- •Applying the Remaining Bindings
- •MultiBinding
- •Command
- •Compiled Bindings
- •Shell
- •ShellContent
- •Navigation
- •Registering Pages for Navigation
- •Performing Navigation
- •Navigating Backwards
- •Passing Data When Navigating
- •Flyout
- •FlyoutHeader
- •FlyoutContent
- •Selected Board
- •Navigation to the Selected Board
- •Setting the BindingContext of Your AppShell
- •Register AppShell with the MAUI App Builder
- •Resolve the AppShell Instead of Creating It
- •Tabs
- •Search
- •Taking Your Application for a Spin
- •Summary
- •Source Code
- •Extra Assignment
- •Placeholder
- •ILayoutManager
- •BoardLayout
- •BoardLayout.xaml
- •BindableLayout
- •BoardLayout.xaml.cs
- •Adding the LayoutManager Property
- •Adding the ItemsSource Property
- •Adding the ItemTemplateSelector Property
- •Handling the ChildAdded Event
- •Adding Remaining Bits
- •FixedLayoutManager
- •Accepting the Number of Rows and Columns for a Board
- •Adding the NumberOfColumns Property
- •Adding the NumberOfRows Property
- •Building the Board Layout
- •Setting the Correct Row/Column Position for Each Widget
- •Using Your Layout
- •Allowing for the Registration of Widget Views and View Models
- •Creation of a Widget View
- •Creation of a Widget View Model
- •Registering the Factory with MauiAppBuilder
- •Registering Your ClockWidget with the Factory
- •WidgetTemplateSelector
- •Registering the Template Selector with MauiAppBuilder
- •Updating FixedBoardPageViewModel
- •Finally Using the Layout
- •Summary
- •Source Code
- •Extra Assignment
- •What Is Accessibility?
- •Why Make Your Applications Accessible?
- •What to Consider When Making Your Applications Accessible
- •How to Make Your Application Accessible
- •Screen Reader Support
- •SemanticProperties
- •SemanticProperties.Description
- •SemanticProperties.Hint
- •SemanticProperties.HeadingLevel
- •SemanticScreenReader
- •AutomationProperties
- •AutomationProperties.ExcludedWithChildren
- •AutomationProperties.IsInAccessibleTree
- •Suitable Contrast
- •Dynamic Text Sizing
- •Avoiding Fixed Sizes
- •Preferring Minimum Sizing
- •Font Auto Scaling
- •Testing Your Application’s Accessibility
- •Android
- •macOS
- •Windows
- •Accessibility Checklist
- •Summary
- •Source Code
- •Extra Assignment
- •Adding the Ability to Add a Widget to a Board
- •Possible Ways of Achieving Your Goal
- •Showing a Modal Page
- •The Chosen Approach
- •Adding Your Overlay View
- •Updating Your View Model
- •Showing the Overlay View
- •Styling
- •Examining the Default Styles
- •TargetType
- •ApplyToDerivedTypes
- •Setter
- •AppThemeBinding
- •Further Reading
- •Triggers
- •Creating ShowOverlayTriggerAction
- •Using ShowOverlayTriggerAction
- •Further Reading
- •Animations
- •Basic Animations
- •Combining Basic Animations
- •Chaining Animations
- •Concurrent Animations
- •Cancelling Animations
- •Easings
- •Complex Animations
- •Recreating the ScaleTo Animation
- •Creating a Rubber Band Animation
- •Combining Triggers and Animations
- •Summary
- •Source Code
- •Extra Assignment
- •Animate the BoxView Overlay
- •Animate the New Widget
- •What Is Local Data?
- •File System
- •Cache Directory
- •App Data Directory
- •Database
- •Repository Pattern
- •Listing Your Boards
- •SQLite
- •Installing SQLite-net
- •Using Sqlite-net
- •Connecting to an SQLite database
- •Mapping Your Models
- •Creating Your Tables
- •Inserting into an SQLite Database
- •Reading a Collection from an SQLite Database
- •Reading a Single Entity from an SQLite Database
- •Deleting from an SQLite Database
- •Updating an Entity in an SQLite Database
- •LiteDB
- •Installing LiteDB
- •Using LiteDB
- •Connecting to a LiteDB database
- •Mapping Your Models
- •Creating Your Tables
- •Inserting into a LiteDB Database
- •Reading a Collection from a LiteDB Database
- •Reading a Single Entity from a LiteDB Database
- •Deleting from a LiteDB Database
- •Updating an Entity in a LiteDB Database
- •Database Summary
- •Application Settings (Preferences)
- •What Can Be Stored in Preferences?
- •Setting a Value in Preferences
- •Getting a Value in Preferences
- •Checking if a Key Exists in Preferences
- •Secure Storage
- •Storing a Value Securely
- •Reading a Secure Value
- •Removing a Secure Value
- •Platform specifics
- •Android
- •Windows
- •Summary
- •Source Code
- •Extra Assignment
- •What Is Remote Data?
- •Considerations When Handling Remote Data
- •Loading Times
- •Failures
- •Security
- •Webservices
- •The Open Weather API
- •Creating an Open Weather Account
- •Creating an Open Weather API key
- •Using System.Text.Json
- •Creating Your Models
- •Connecting to the Open Weather API
- •Registering Your Widget
- •Testing Your Widget
- •Adding Some State
- •Converting the State to UI
- •Displaying the Loading State
- •Displaying the Loaded State
- •Displaying the Error State
- •Simplifying Webservice Access
- •Prebuilt Libraries
- •Code Generation Libraries
- •Adding the Refit NuGet Package
- •Further Reading
- •Polly
- •Summary
- •Source Code
- •Extra Assignment
- •TODO Widget
- •Quote of the Day Widget
- •NASA Space Image of the Day Widget
- •.NET MAUI Essentials
- •Permissions
- •Checking the Status of a Permission
- •Requesting Permission
- •Handling Permissions in Your Application
- •Using the Geolocation API
- •Registering the Geolocation Service
- •Using the Geolocation Service
- •Displaying Permission Errors to Your User
- •Configuring Platform-Specific Components
- •Android
- •Windows
- •Platform-Specific API Access
- •Platform-Specific Code with Compiler Directives
- •Platform-Specific Code in Platform Folders
- •Overriding the Platform-Specific UI
- •OnPlatform
- •OnPlatform Markup Extension
- •Conditional Statements
- •Handlers
- •Customizing Controls with Mappers
- •Scoping of Mapper Customization
- •Further Reading
- •Summary
- •Source Code
- •Extra Assignment
- •Barometer Widget
- •Geocoding Lookup
- •Unit Testing
- •Unit Testing in .NET MAUI
- •xUnit
- •NUnit
- •MSTest
- •Your Chosen Testing Framework
- •Adding Your Own Unit Tests
- •Adding a Unit Test Project to Your Solution
- •Modify Your Application Project to Target net7.0
- •Adding a Reference to the Project to Test
- •Modify Your Test Project to Use MAUI Dependencies
- •Testing Your View Models
- •Testing BoardDetailsPageViewModel
- •Testing INotifyPropertyChanged
- •Testing Asynchronous Operations
- •Creating Your ILocationService Mock
- •Creating Your WeatherForecastService Mock
- •Creating Your Asynchronous Tests
- •Testing Your Views
- •Creating Your ClockWidgetViewModel Mock
- •Creating Your View Tests
- •Device Testing
- •Creating a Device Test Project
- •Adding a Device-Specific Test
- •Running Device-Specific Tests
- •Snapshot Testing
- •Snapshot Testing Your Application
- •Passing Thoughts
- •Summary
- •Source Code
- •.NET MAUI Graphics
- •Maintaining the State of the Canvas
- •Further Reading
- •Building a Sketch Widget
- •Representing a User Interaction
- •Registering Your Widget
- •Taking Your Widget for a Test Draw
- •Summary
- •Source Code
- •Extra Assignment
- •Distributing Your Application
- •Android
- •Additional Resources
- •Certificate
- •Identifier
- •Capabilities
- •Entitlements
- •Provisioning Profiles
- •Additional Resources
- •macOS
- •Additional Resources
- •Windows
- •Additional Resources
- •Following Good Practices
- •Performance
- •Startup Tracing
- •Image Sizes
- •Linking
- •What Is Linking?
- •Issues That Crop Up
- •Crashes/Analytics
- •Sentry
- •App Center
- •Obfuscation
- •Distributing Test Versions
- •Summary
- •Looking at the Final Product
- •Taking the Project Further
- •Useful Resources
- •StackOverflow
- •GitHub
- •YouTube
- •Gerald Versluis
- •James Montemagno
- •Social Media
- •Yet More Goodness
- •Looking Forward
- •Comet
- •Testing
- •Index
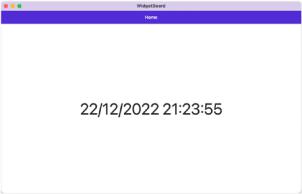
Chapter 4 An Architecture to Suit You
Figure 4-4. The clock widget rendered in the application running on macOS
You have looked at ways to optimize your codebase when using MVVM but I would like to provide some further details on how you can leverage the power of the community in order to further improve your experience.
MVVM Enhancements
There are two key parts I will cover regarding how you can utilize existing packages to reduce the amount of code you are required to write.
MVVM Frameworks
There are several MVVM frameworks that can expand on this by providing a base class implementation for you with varying levels of other extra features. To list a few,
•\ CommunityToolkit.Mvvm
•\ MVVMLight
•\ FreshMVVM
•\ Prism
101
Chapter 4 An Architecture to Suit You
•\ Refractored.MVVMHelpers
•\ ReactiveUI
These packages will ultimately provide you with a base class very similar to the BaseViewModel class that you created earlier. For example, the Prism library provides the BindableBase class that you could use. It offers yet another optimization in terms of less code that you need to write and ultimately maintain.
You can go a step further, but you need to believe…
Magic
Yes, that’s right: magic is real! These approaches involve auto generating the required boilerplate code so that we as developers do not have to do it. There are two main packages that offer this functionality. They provide it through different mechanisms, but they work equally well.
•\ |
Fody: IL generation, https://github.com/Fody/Home |
•\ |
CommunityToolkit.Mvvm: Source generators (yes, this |
|
gets a second mention), https://learn.microsoft. |
|
com/dotnet/communitytoolkit/mvvm/ |
In the past, I was skeptical of using such packages. I felt like I was losing control of parts that I needed to hold on to. Now I can appreciate that I was naïve, and this is impressive.
Let’s look at how these packages can help to further reduce the code. This example uses CommunityToolkit.Mvvm, which provides the ObservableObject base class and a wonderful way of adding attributes
([ObservableProperty]) to the fields you wish to trigger PropertyChanged events when their value changes. This will then generate a property with the same name as the field but with a capitalized first character, so time becomes Time.
102
Chapter 4 An Architecture to Suit You
public partial class ClockWidgetViewModel : ObservableObject
{
[ObservableProperty] private DateTime time;
public ClockWigetViewModel()
{
SetTime(DateTime.Now);
}
public void SetTime(DateTime dateTime)
{
Time = dateTime;
scheduler.ScheduleAction(
TimeSpan.FromSeconds(1),
() => SetTime(DateTime.Now));
}
}
That’s 17 lines down to 2 from the original example! The part that I really like is that it reduces all the noise of the boilerplate code so there is a bigger emphasis on the code that we need to write as developers.
You may have noticed that you are still referring to the Time property in the code but you haven’t supplied the definition for this property. This is where the magic comes in! If you right-click the Time property and select Go to Definition… it will open the following source code so you can view what the toolkit has created for you:
// <auto-generated/> #pragma warning disable #nullable enable
namespace WidgetBoard.ViewModels
103
Chapter 4 An Architecture to Suit You
{
partial class ClockWidgetViewModel
{
/// <inheritdoc cref="time"/> [global::System.CodeDom.Compiler. GeneratedCode("CommunityToolkit.Mvvm.SourceGenerators. ObservablePropertyGenerator", "8.0.0.0")] [global::System.Diagnostics.CodeAnalysis. ExcludeFromCodeCoverage]
public global::System.DateTime Time
{
get => time; set
{
if (!global::System.Collections.Generic. EqualityComparer<global::System.DateTime>. Default.Equals(time, value))
{
OnTimeChanging(value);
OnPropertyChanging(global::CommunityToo lkit.Mvvm.ComponentModel.__Internals.__ KnownINotifyPropertyChangingArgs.Time); time = value;
OnTimeChanged(value);
OnPropertyChanged(global::CommunityTool kit.Mvvm.ComponentModel.__Internals.__ KnownINotifyPropertyChangedArgs.Time);
}
}
}
104