
lafore_robert_objectoriented_programming_in_c
.pdf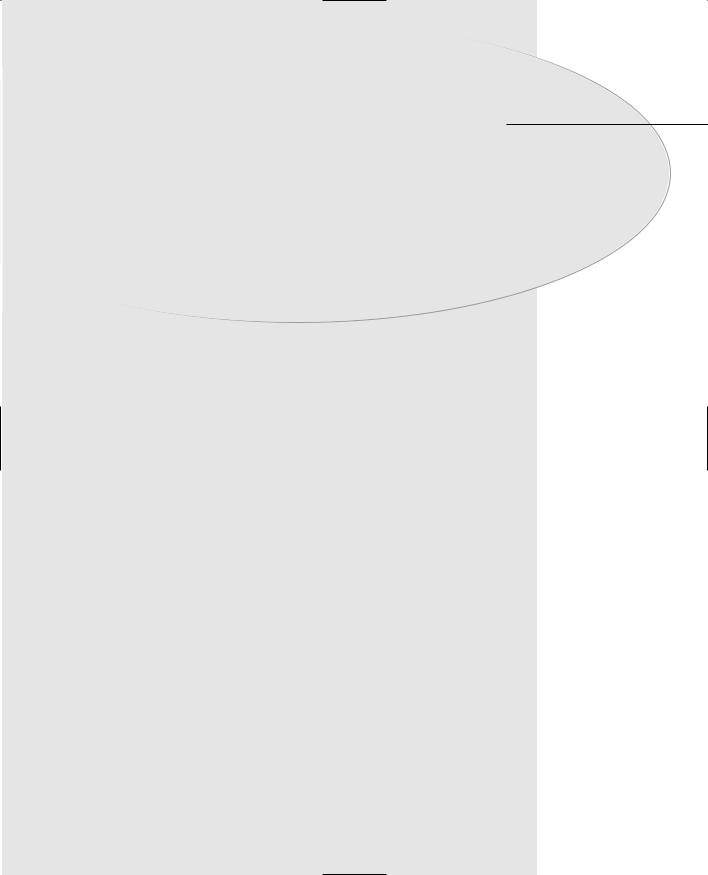
STL Algorithms and Member |
A PPENDI X |
Functions |
F |
|
IN THIS APPENDIX
• Algorithms 896
• Member Functions 907
• Iterators 909
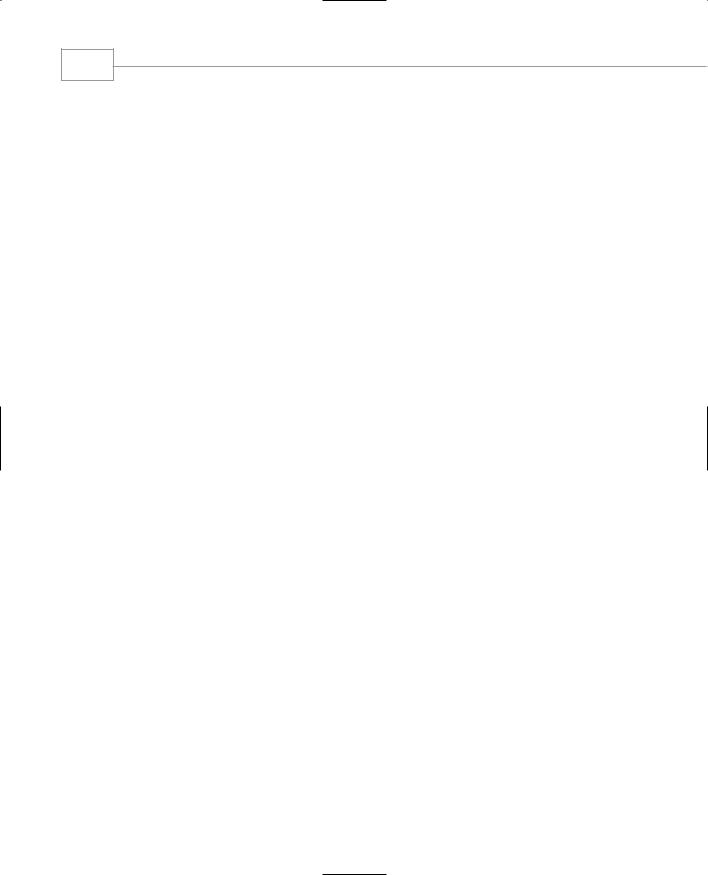
Appendix F
896
This appendix contains charts showing the algorithms and container member functions available in the Standard Template Library (STL). This information is based on The Standard Template Library by Alexander Stepanov and Ming Lee (1995), but we have extensively condensed and revised it, taking many liberties with their original formulation in the interest of quick understanding.
Algorithms
Table F.1 shows the algorithms available in the STL. The descriptions in this table offer a quick and condensed explanation of what the algorithms do; they are not intended to be serious mathematical definitions. For more information, including the exact data types to use for arguments and return values, consult one of the books listed in Appendix H, “Bibliography.”
The first column gives the function name, the second explains the purpose of the algorithm, and the third specifies the arguments. Return values are not systematically specified. Some are mentioned in the Purpose column and many are either obvious or not vital to using the algorithm.
In the Arguments column, the names first, last, first1, last1, first2, last2, first3, and middle represent iterators to specific places in a container. Names with numbers (like first1) are used to distinguish multiple containers. The name first1, last1 delimits range 1, and first2, last2 delimits range 2. The arguments function, predicate, op, and comp are function objects. The arguments value, old, new, a, b, and init are values of the objects stored in a container. These values are ordered or compared based on the < or == operators or the comp function object. The argument n is an integer.
In the Purpose column, moveable iterators are indicated by iter, iter1, and iter2. When iter1 and iter2 are used together, they are assumed to move together step by step through their respective containers (or possibly two different ranges in the same container).
TABLE F.1 |
Algorithms |
|
Name |
Purpose |
Arguments |
|
|
|
|
Non-mutating Sequence Operations |
|
for_each |
Applies function to each |
first, last, function |
|
object. |
|
find |
Returns iterator to first |
first, last, value |
|
object equal to value. |
|
find_if |
Returns iterator to first |
|
|
object for which predicate |
first, last, predicate |
|
is true. |
|
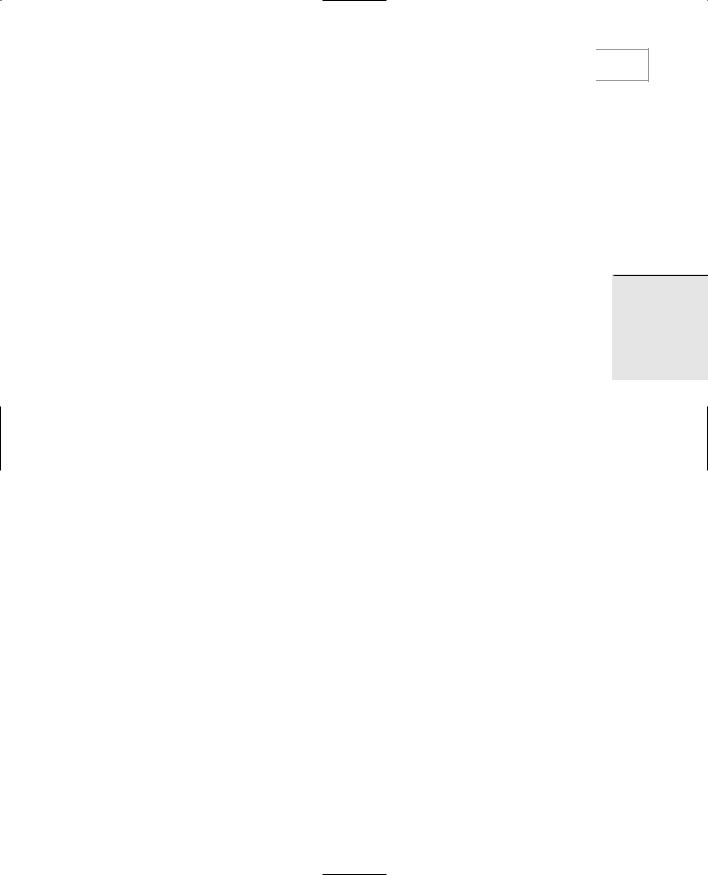
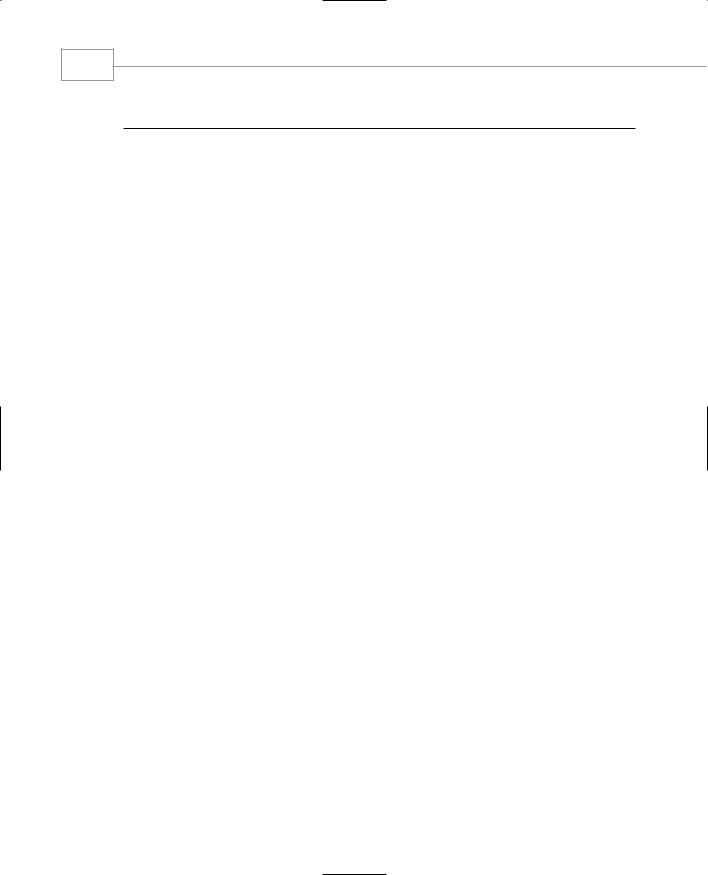
898 |
Appendix F |
|
TABLE F.1 Continued
Name |
Purpose |
Arguments |
copy_backward |
Copies objects from range 1 |
first1, last1, first2 |
|
to range 2, inserting them |
|
|
backwards, from last2 to |
|
|
first2. |
|
swap |
Interchanges two objects. |
a, b |
iter_swap |
Interchanges objects pointed |
iter1, iter2 |
|
to by two iterators. |
|
swap_ranges |
Interchanges corresponding |
first1, last1, first2 |
|
elements in two ranges. |
|
transform |
Transforms objects in range |
first1, last1, first2, |
|
1 into new objects in range |
operator |
|
2 by applying operator. |
|
transform |
Combines objects in range 1 |
first1, last1, first2, |
|
and range 2 into new objects |
first3, operator |
|
in range 3 by applying |
|
|
operator. |
|
replace |
Replaces all objects equal |
first, last, old, new |
|
to old with objects equal |
|
|
to new. |
|
replace_if |
Replaces all objects that |
first, last, predicate, |
|
satisfy predicate with |
new |
|
objects equal to new. |
|
replace_copy |
Copies from range 1 to |
first1, last1, first2, |
|
range 2, replacing all |
old, new |
|
objects equal to old with |
|
|
objects equal to new. |
|
replace_copy_if |
Copies from range 1 to range |
first1, last1, first2, |
|
2, replacing all objects that |
predicate, new |
|
satisfy predicate with |
|
|
objects equal to new. |
|
fill |
Assigns value to all |
first, last, value |
|
objects in range. |
|
fill_n |
Assigns value to all |
first, n, value |
|
objects from first to |
|
|
first+n. |
|
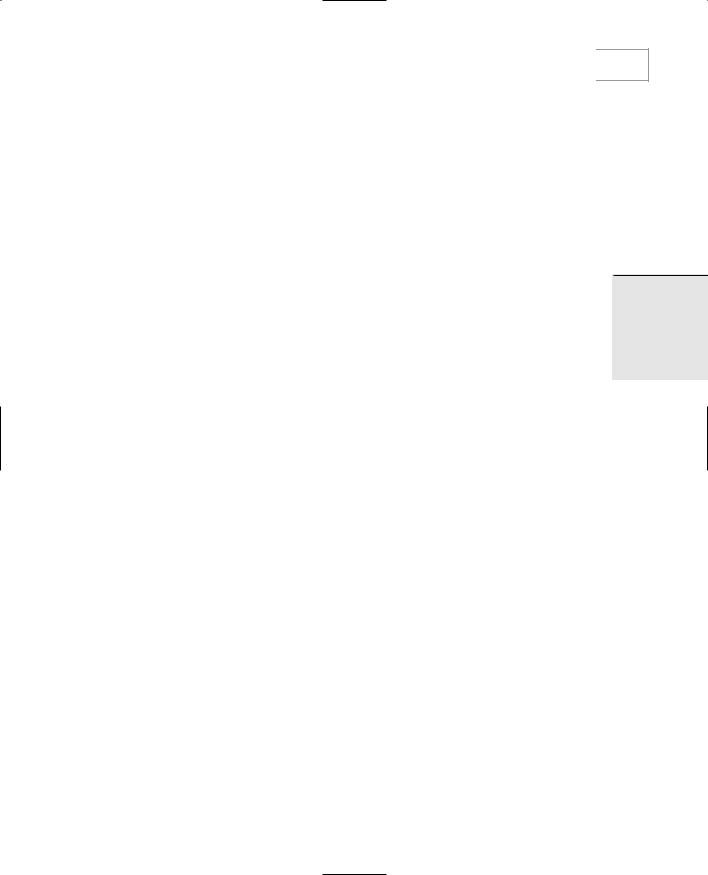
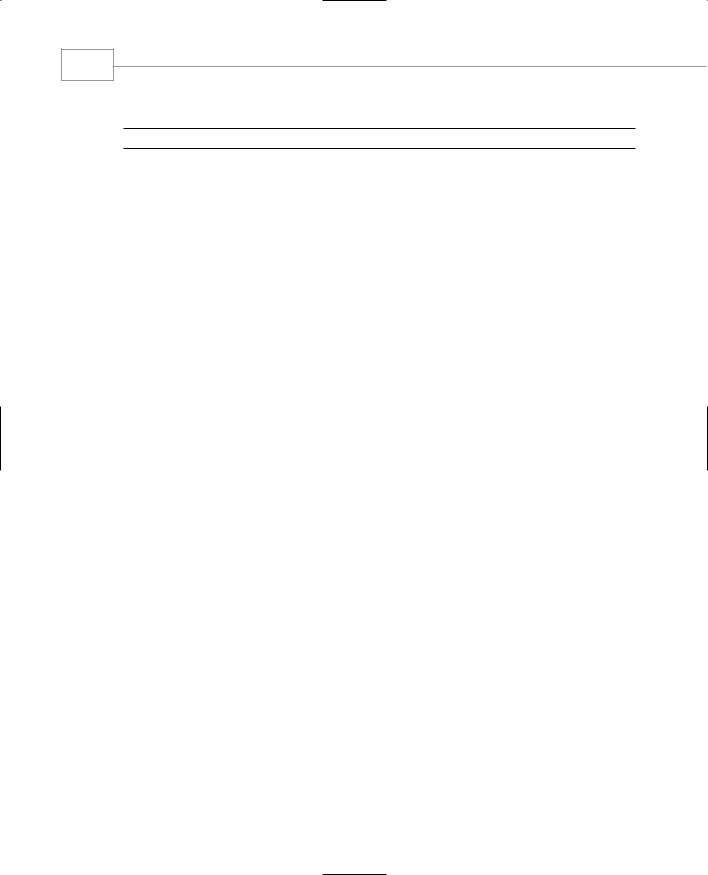
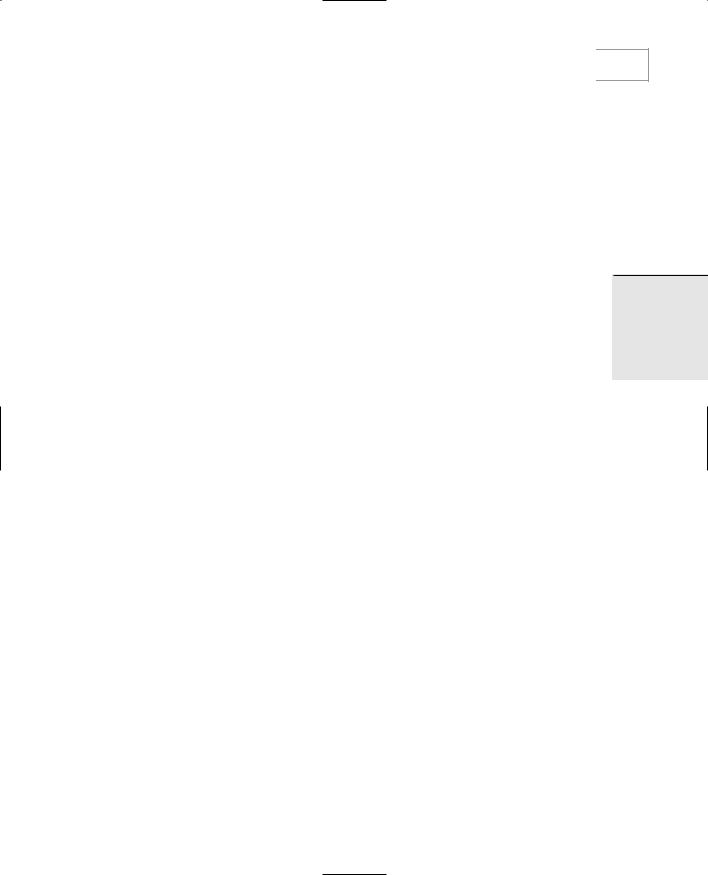
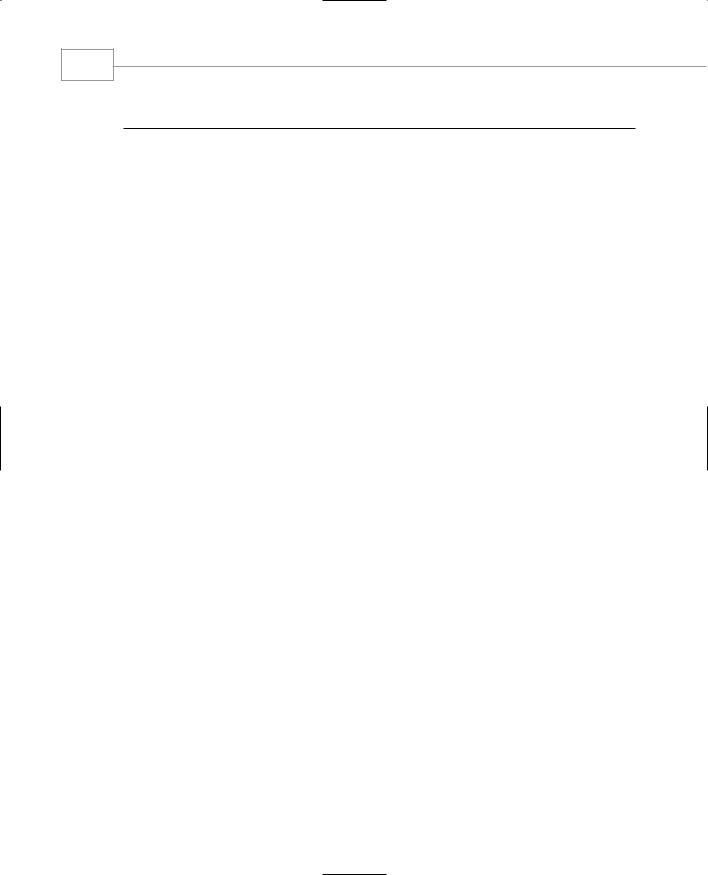
902 |
Appendix F |
|
TABLE F.1 Continued
Name |
Purpose |
Arguments |
upper_bound |
Returns iterator to last |
first, last, value |
|
position into which value |
|
|
could be inserted without |
|
|
violating the ordering. |
|
upper_bound |
Returns iterator to last |
first, last, value, comp |
|
position into which value |
|
|
could be inserted without |
|
|
violating an ordering based |
|
|
on comp. |
|
equal_range |
Returns a pair containing the |
first, last, value |
|
lower bound and upper bound |
|
|
between which value could |
|
|
be inserted without violating |
|
|
the ordering. |
|
equal_range |
Returns a pair containing |
first, last, value, comp |
|
the lower bound and upper |
|
|
bound between which value |
|
|
could be inserted without |
|
|
violating an ordering based |
|
|
on comp. |
|
binary_search |
Returns true if value is in |
first, last, value |
|
the range. |
|
binary_search |
Returns true if value is |
first, last, value, comp |
|
in the range, where the |
|
|
ordering is determined by |
|
|
comp. |
|
merge |
Merges sorted ranges 1 and |
first1, last1, first2, |
|
2 into sorted range 3. |
last2, first3 |
merge |
Merges sorted ranges 1 and 2 |
first1, last1, first2, |
|
into sorted range 3, where |
last2, first3, comp |
|
the ordering is determined |
|
|
by comp. |
|
inplace_merge |
Merges two consecutive |
first, middle, last |
|
sorted ranges, first, |
|
|
middle and middle, last |
|
|
into first, last. |
|
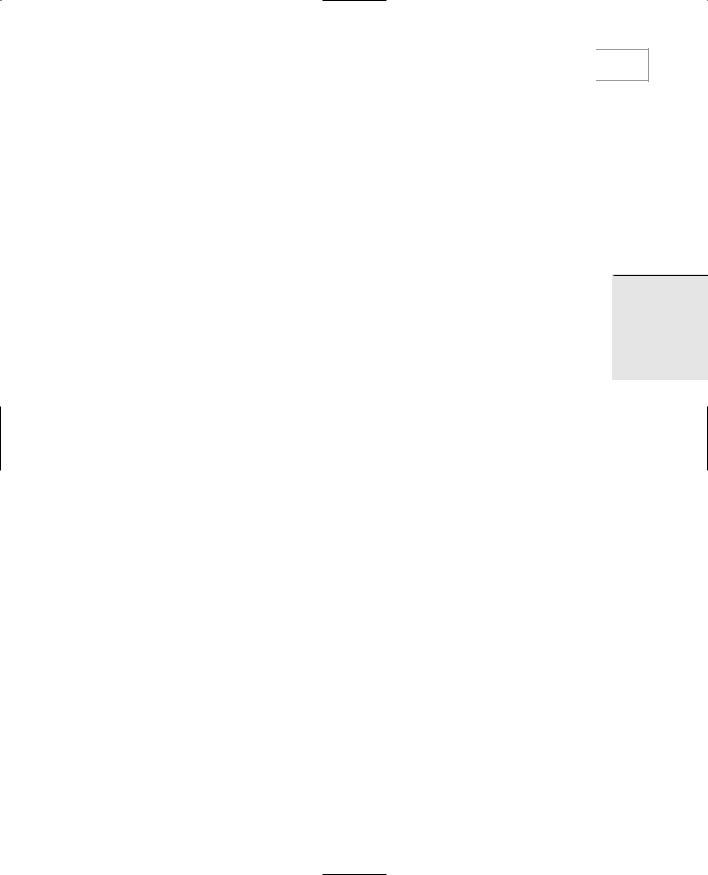
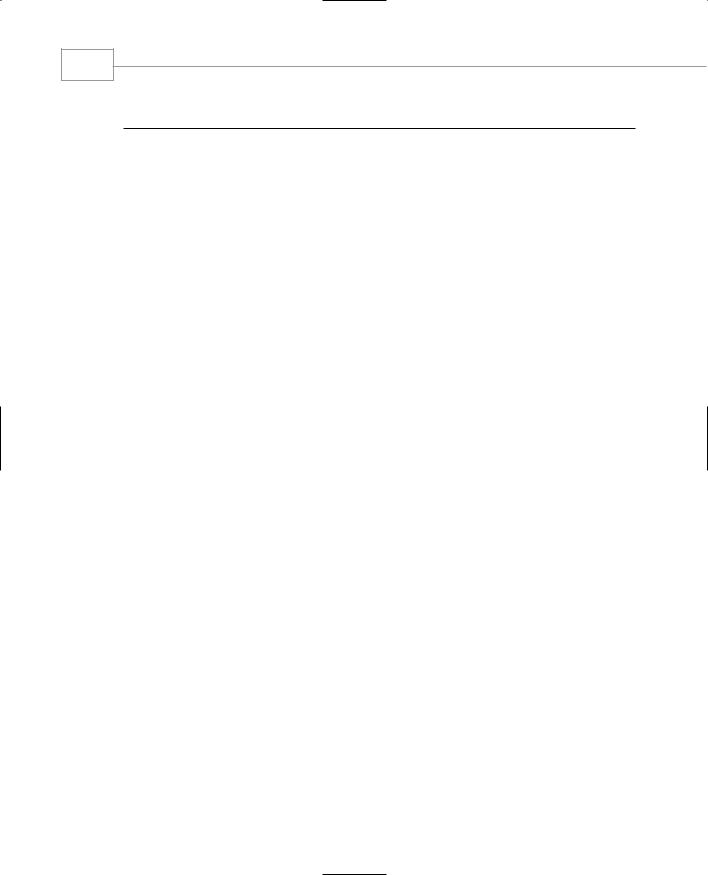