
lafore_robert_objectoriented_programming_in_c
.pdf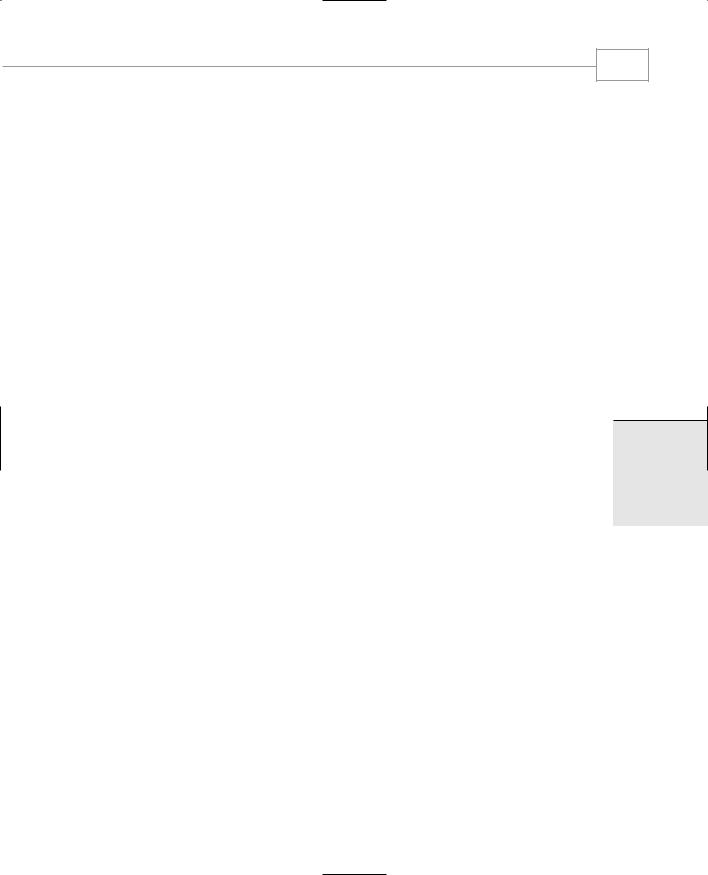
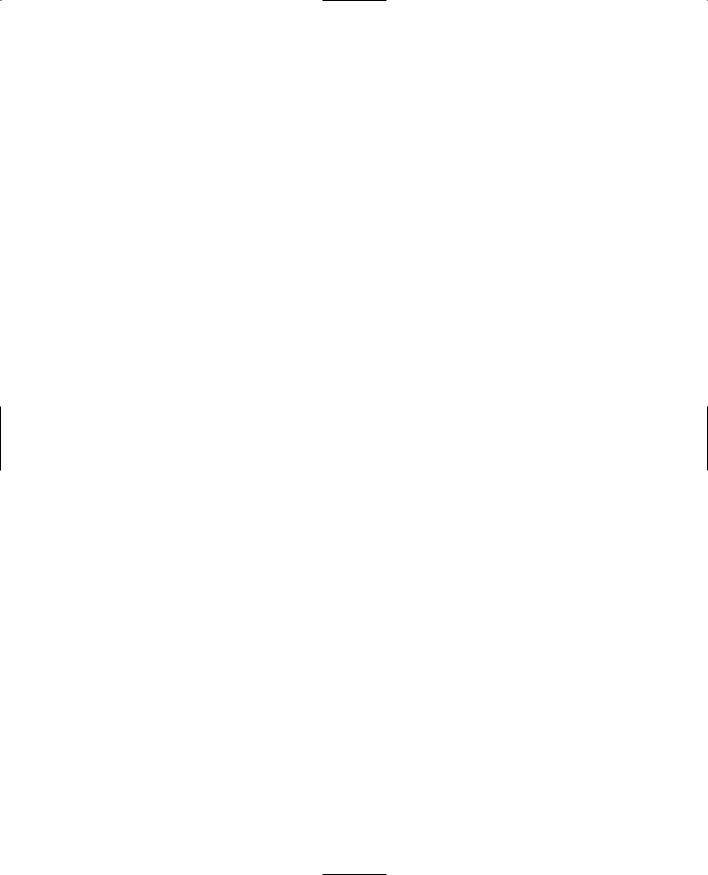
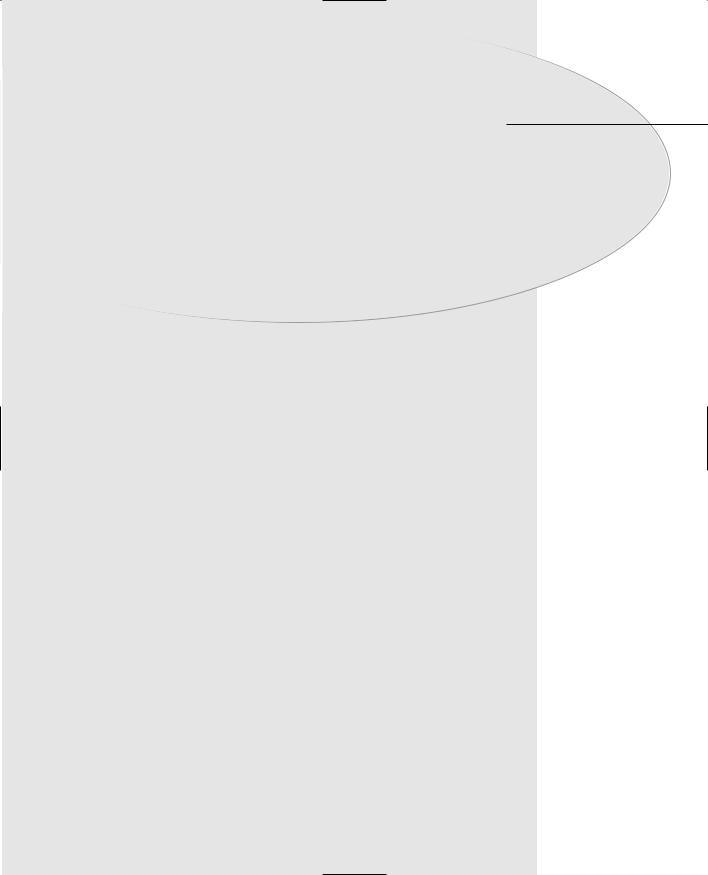
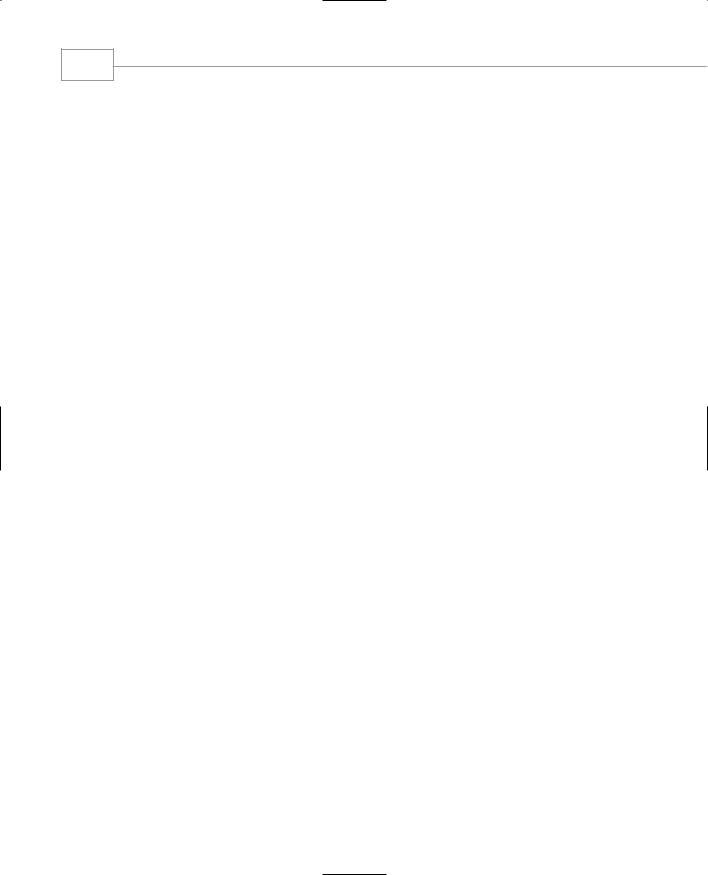
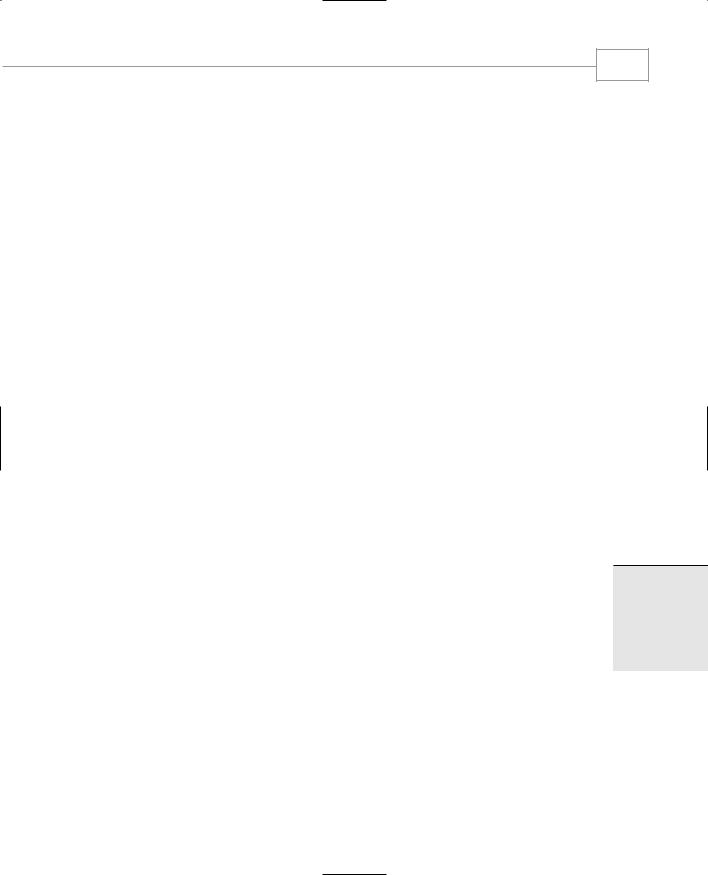
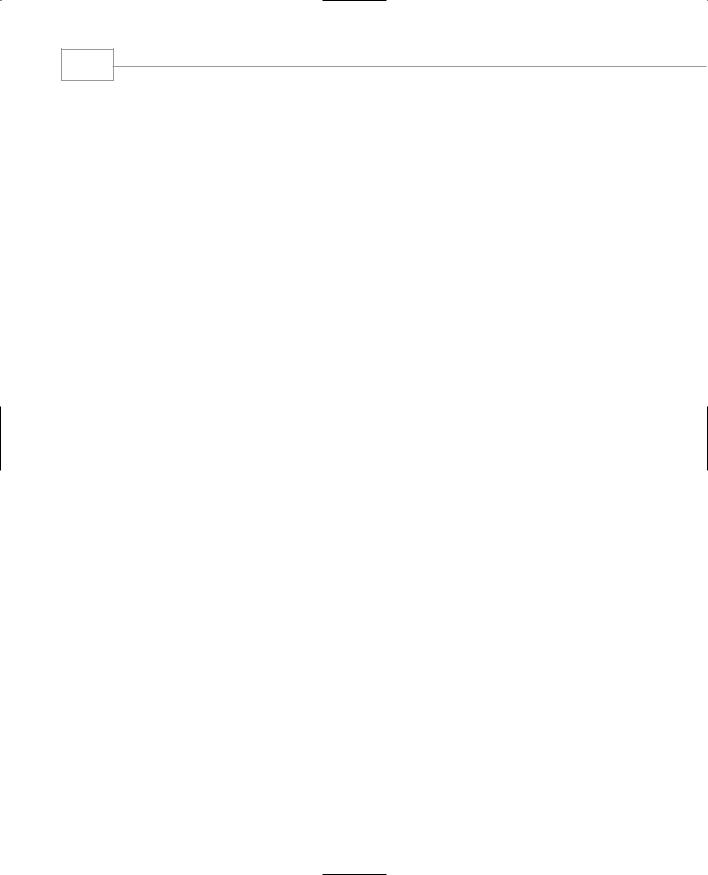
Appendix H
980
Although it’s nominally based on Java, Object-Oriented Design in Java by Stephen Gilbert and Bill McCarty (Waite Group Press, 1998) is a comprehensive, easy-to-read introduction to OO program design in any language.
Windows Game Programming for Dummies by André LaMothe (IDG Books, 1998) is a fascinating look at the details of game programming. André’s book explains (among many other things) how to use the Windows console graphics routines, which form the basis of Console Graphics Lite routines discussed in Appendix E of this book. If you have any interest in writing game programs, buy this book.
The C Programming Language, Second Edition by Brian Kernighan and Dennis Ritchie (Prentice Hall PTR, 1988) is the definitive book about C, the language on which C++ was based. It’s not a primer, but once you know some C it’s the reference you’ll want.
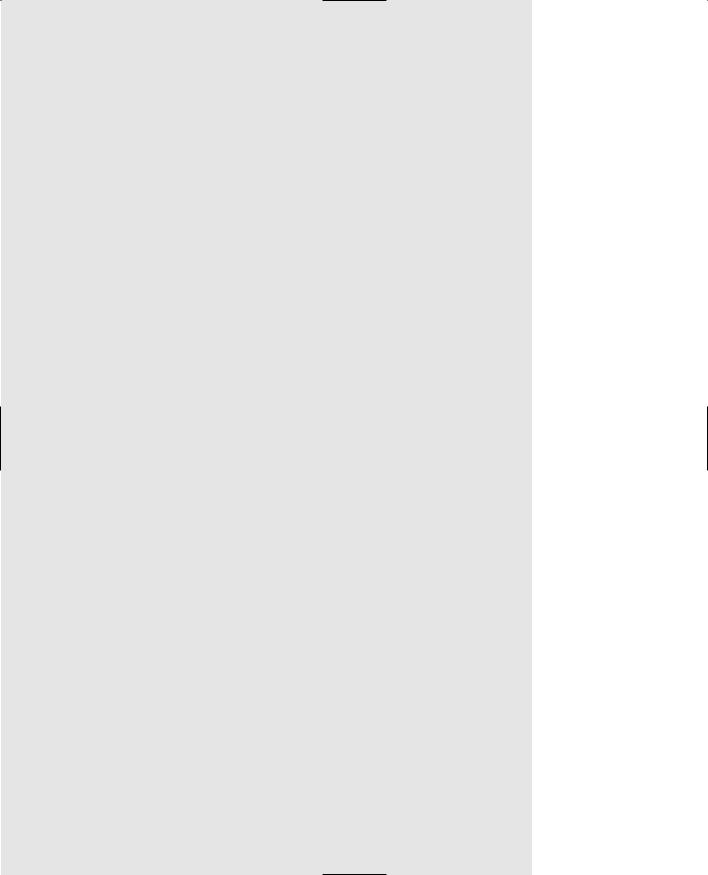
INDEX
SYMBOLS
# (number sign), 35
&& (AND) logical operator, 115-116 ! (NOT) logical operator, 116-117
|| (OR) logical operator, 116-117 & (address-of) operator, 431-433 + (arithmetic) operator, 60
= (assignment) operator, 532-536, 539-546
— (decrement) operator, 65, 320 / (division) operator, 60
. (dot) operator, 360
== (equal to) operator, 77
>> (extraction) operator, 46-47
++ (increment) operator, 63-65, 320-322 << (insertion) operator, 34, 47, 54
* (multiplication) operator, 60 != (not equal to) operator, 77 % (remainder) operator, 61
[ ] (subscript) operator, 340-343 - (subtraction) operator, 60
A
abstract base classes, 392-393 abstract classes, 510
access specifiers default, 398
private access specifier, 377-379, 398-399 protected access specifier, 377, 379 public access specifier, 377-379, 399
tips for selecting, 399
access violation error message, 493 accessibility and inheritance, 376-377, 379
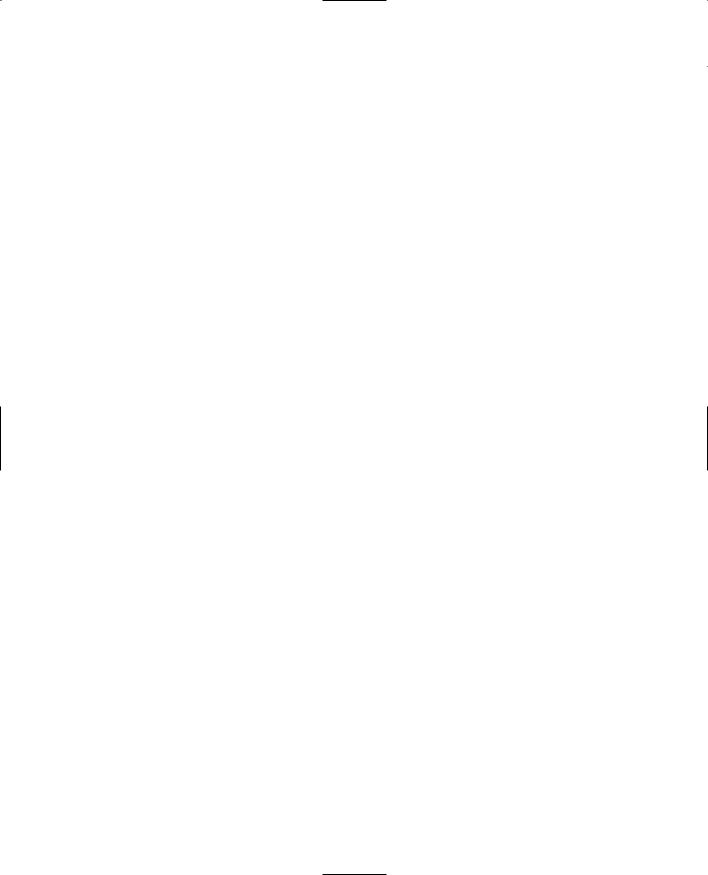
982 |
accessing |
|
|
|
|
|
|
||
accessing |
adjacent_find algorithm, |
lower_bound algorithm, |
||
|
||||
|
array elements, 267 |
897 |
901 |
|
|
pointers, 441-442 |
Advanced Use Case |
make_heap algorithm, 904 |
|
|
structures, 278 |
Modeling, 979 |
max algorithm, 905 |
|
|
base class members, |
aggregates, 830-831 |
max_element algorithm, |
|
|
376-377, 379 |
algorithms |
905 |
|
|
characters in string objects, |
accumulate algorithm, |
merge algorithm, 733, 738, |
|
|
309-310 |
733, 906 |
902 |
|
|
data with iterators, |
adjacent_difference algo- |
min algorithm, 905 |
|
|
759-760 |
rithm, 907 |
min_element algorithm, |
|
|
elements of arrays, 267 |
adjacent_find algorithm, |
905 |
|
|
pointers, 441-442 |
897 |
mismatch algorithm, 897 |
|
|
structures, 278 |
binary_search algorithm, |
next_permutation algo- |
|
|
member function data with |
902 |
rithm, 906 |
|
|
this pointer, 547-548 |
containers, 755, 758-759 |
nth_element algorithm, |
|
|
members of static func- |
copy algorithm, 733, 897 |
901 |
|
|
tions, 531 |
copy_backward algorithm, |
partial_sort algorithm, 901 |
|
|
members of structures, |
898 |
partial_sort_copy algo- |
|
|
136-137, 142-143 |
count algorithm, 732, 736, |
rithm, 901 |
|
|
namespace members, 648 |
897 |
partial_sum algorithm, |
|
|
accumulate algorithm, |
count_if algorithm, 897 |
906-907 |
|
|
733, 906 |
equal algorithm, 732, 897 |
partition algorithm, 900 |
|
|
activity diagrams, 808, |
equal_range algorithm, |
pop_heap algorithm, 904 |
|
|
815-816 |
902 |
prev_permutation algo- |
|
|
adapters |
fill algorithm, 733, 898 |
rithm, 906 |
|
|
containers, 731-732 |
fill_n algorithm, 898 |
push_heap algorithm, 904 |
|
|
iterators |
find algorithm, 732, |
random_shuffle algorithm, |
|
|
insert iterator, 763-766 |
735-736, 896 |
900 |
|
|
raw storage iterator, |
find_if algorithm, 896 |
remove algorithm, 899 |
|
|
763 |
for_each algorithm, 733, |
remove_copy algorithm, |
|
|
reverse iterator, |
742, 896 |
899 |
|
|
763-764 |
function objects, 739 |
remove_copy_if algorithm, |
|
|
addition (+) operator, 60 |
generate algorithm, 899 |
899 |
|
|
address-of & operator, |
generate_n algorithm, 899 |
remove_if algorithm, 899 |
|
|
431-433 |
if algorithm, 740-741 |
replace algorithm, 898 |
|
|
addresses in memory and |
includes algorithm, 903 |
replace_copy algorithm, |
|
|
pointers, 430-431 |
inner_product algorithm, |
898 |
|
|
accessing variable pointed |
906 |
replace_copy_if algorithm, |
|
|
to, 436-439 |
inplace_merge algorithm, |
898 |
|
|
address-of & operator, |
902-903 |
replace_if algorithm, 898 |
|
|
431-433 |
iter_swap algorithm, 733, |
reverse algorithm, 900 |
|
|
constants, 435-436 |
898 |
reverse_copy algorithm, |
|
|
variables, 433-434 |
iterators, 757-758, |
900 |
|
|
adjacent_difference |
761-763, 909-911 |
rotate algorithm, 900 |
|
|
algorithm, 907 |
lexicographical_compare |
rotate_copy algorithm, 900 |
|
|
|
algorithm, 905 |
search algorithm, 733, |
|
|
|
|
737-738, 897 |
|
|
|
|
|
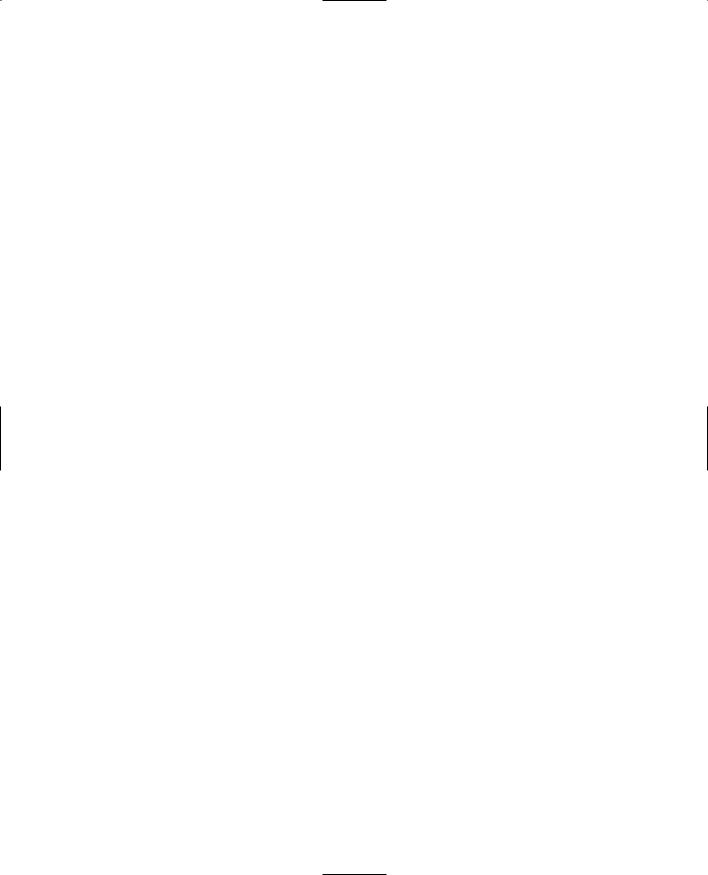
|
|
arrays |
983 |
|
|
|
|
||
set_difference algorithm, |
arguments |
arrays, 728 |
||
|
||||
903-904 |
exceptions, 714-717 |
arguments (functions), |
|
|
set_intersection algorithm, |
function templates, |
446-448 |
|
|
903 |
686-689 |
bounds, 284-285 |
|
|
set_symmetric_difference |
functions, 31, 66 |
buffers, 292 |
|
|
algorithm, 904 |
arrays, 274-276, |
C-strings, 297-298 |
|
|
set_union algorithm, 903 |
446-448 |
class members, 279-283 |
|
|
sort algorithm, 733, 737, |
C-strings, 453-454 |
data types, 264, 290 |
|
|
900 |
const function argu- |
defining, 265-266, 271 |
|
|
sort_heap algorithm, |
ments, 208-209, 254 |
elements |
|
|
904-905 |
constants, 167-169 |
accessing, 267, 271, |
|
|
stable_partition algorithm, |
default arguments, |
441-442 |
|
|
900 |
197-199 |
averaging, 267-268 |
|
|
stable_sort algorithm, 900 |
function templates, 686 |
overview, 265-267 |
|
|
STL, 726-727, 732-733, |
naming, 176 |
sorting, 448-451 |
|
|
735-743, 896-907 |
objects, 233-234, 237 |
examples, 264-265, |
|
|
swap algorithm, 733, 898 |
overloaded functions, |
286-290 |
|
|
swap_ranges algorithm, |
189-193 |
functions |
|
|
898 |
parameters, 169 |
calling, 276 |
|
|
transform algorithm, |
passing, 167-176 |
declarations, 275-276 |
|
|
742-743, 898 |
passing by pointers, |
definitions, 276 |
|
|
unique algorithm, 899 |
444-448 |
passing, 275 |
|
|
unique_copy algorithm, |
passing by reference, |
index, 267 |
|
|
899 |
443-444 |
index numbers, 264 |
|
|
upper_bound algorithm, |
references, 182-188 |
initializing, 268-270, |
|
|
902 |
structures, 171-176 |
273-274 |
|
|
user-written functions, |
values, 170-171 |
memory management, |
|
|
739-740 |
variables, 169-170 |
458-459 |
|
|
uses, 726 |
manipulators, 572 |
multidimensional arrays |
|
|
AND logical operator, |
overloaded operators, 323 |
accessing elements, 271 |
|
|
115-116 |
arithmetic assignment |
defining, 271 |
|
|
animation loops, 84 |
operator, 61-63, 337-339 |
formatting numbers, |
|
|
The Annotated C++ |
arithmetic expressions, |
272-273 |
|
|
Reference Manual, 978 |
479-484 |
initializing, 273-274 |
|
|
answer key to questions, |
arithmetic operators |
overview, 270-271 |
|
|
914-975 |
addition (+) operator, 60 |
objects, 283-284 |
|
|
append() member func- |
arithmetic assignment |
accessing, 285-286 |
|
|
tion, 307 |
operator, 61-63, 337-339 |
examples, 286-290 |
|
|
applications. See also pro- |
division (/) operator, 60 |
in other computer lan- |
|
|
grams |
modulus operator, 61 |
guages, 264 |
|
|
console-mode applications |
multiplication (*) operator, |
passing to functions, |
|
|
Borland C++Builder, |
60 |
274-275 |
|
|
872-880 |
overloading, 328-334 |
pointers, 440-443 |
|
|
Microsoft Visual C++, |
remainder (%) operator, 61 |
to objects, 467-468 |
|
|
864-870 |
subtraction (-) operator, 60 |
sorting array elements, |
|
|
Landlord application, 809, |
Armour, Frank, 979 |
448-451 |
|
|
811-843 |
|
to strings, 456-458 |
|
|
|
|
|
|
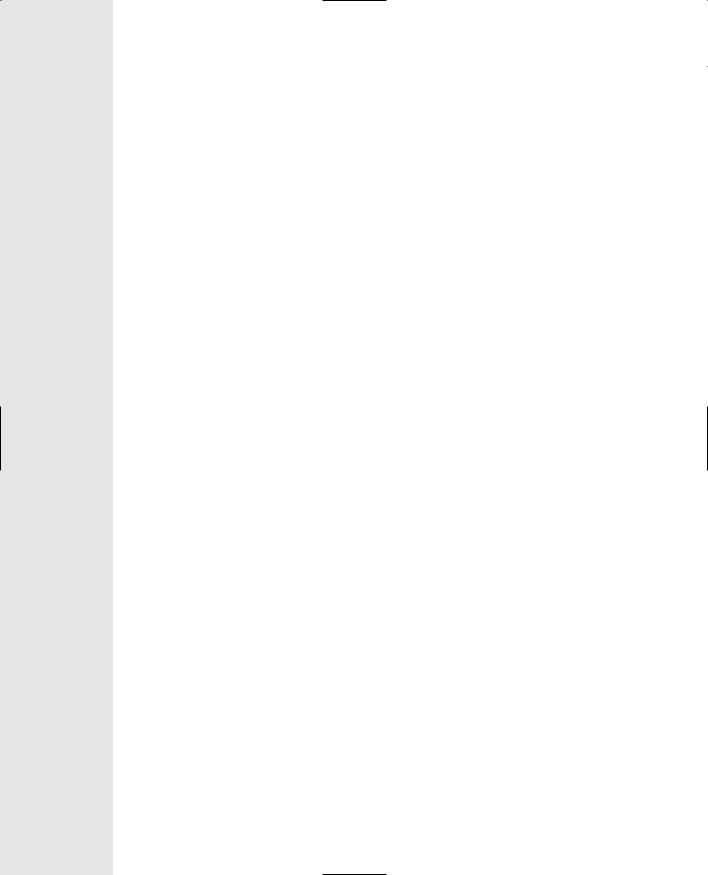
984 |
arrays |
|
|
|
size, 265 |
attributes |
arithmetic operators, |
||
|
||||
|
structures, 277-279 |
classes, 830 |
328-334 |
|
|
accessing elements, 278 |
definition, 15 |
comparison operators, |
|
|
defining, 278 |
objects, 13 |
334-337 |
|
|
similarities, 264 |
auto keyword, 860 |
binary_search algorithm, |
|
|
uses, 264 |
averaging, array ele- |
902 |
|
|
ASCII character set, 43, |
ments, 267-268 |
binding, 509 |
|
|
121 |
|
blanks in C-strings, |
|
|
asm keyword, 860 |
|
293-294 |
|
|
assigning |
B |
blocks of code, 82 |
|
|
string objects, 303-304 |
Booch, Grady, 23, 804, |
||
|
values to variables, 40-41 |
back() member function, |
978 |
|
|
assignment expressions, |
books about C++, 978-980 |
||
|
745-746 |
|||
|
101-102 |
bool keyword, 860 |
||
|
bad alloc class, 717-718 |
|||
|
Assignment operator |
bool variables, 51-52, 114 |
||
|
badbit flag, 577 |
|||
|
invoked message, 535 |
boolalpha flag, 571 |
||
|
base classes, 20, 372-376 |
|||
|
assignment operators |
Boole, George, 52 |
||
|
abstract base classes, |
|||
|
arithmetic assignment |
Borland C++Builder |
||
|
392-393 |
|||
|
operator, 61-63 |
capabilities, 872 |
||
|
accessing members, |
|||
|
chaining, 535 |
Console Graphics Lite, |
||
|
376-377, 379 |
|||
|
inheritance, 536 |
878 |
||
|
destructors, 517-518 |
|||
|
overloading, 539-546 |
debugging programs, |
||
|
instantiating objects of, |
|||
|
overloading and self- |
878-879 |
||
|
510 |
|||
|
assignment, 552-556 |
breakpoints, 879-880 |
||
|
pure virtual functions, |
|||
|
prohibiting copying, 539 |
single-stepping, 879 |
||
|
510-511 |
|||
|
assignment statements |
tracing into functions, |
||
|
virtual base classes, |
|||
|
overview, 40-41 |
879 |
||
|
518-520 |
|||
|
structures, 139 |
watching variables, 879 |
||
|
begin() member function, |
|||
|
associations (classes), |
executable programs, |
||
|
731 |
|||
|
357-358 |
875-876 |
||
|
behavior of objects, 13 |
|||
|
associative containers, |
file extensions, 872 |
||
|
bidirectional iterators, |
|||
|
727, 729, 771 |
header files, 876-877 |
||
|
733-734, 754-755 |
|||
|
keys, 729-730, 771 |
projects, 873-878 |
||
|
binary I/O, 589-591 |
|||
|
maps, 729-730, 771, |
running example programs |
||
|
binary operators, 117 |
|||
|
775-778 |
in, 872 |
||
|
arithmetic assignment |
|||
|
multimaps, 730, 771, |
screen elements, 873 |
||
|
operators, 337-339 |
|||
|
775-778 |
Borland compilers, 30, |
||
|
arithmetic operators, |
|||
|
multisets, 730, 771-775 |
885 |
||
|
328-334 |
|||
|
sets, 729-730, 771-775 |
bounds of arrays, 284-285 |
||
|
comparison operators, |
|||
|
associativity of operators, |
braces in functions, 31 |
||
|
334-337 |
|||
|
447-448 |
break keyword, 109, 860 |
||
|
overloading, 328 |
|||
|
at() member function, 309 |
break statement, 109, |
||
|
arithmetic assignment |
|||
|
atoi() library function, 580 |
119-121 |
||
|
|
operators, 337-339
bsort() function, 450-451 bubble sorts, 450-451