
Sb96899
.pdf{
System.out.println("Agent "+inform.getSender().getName()+ " successfully performed the requested action");
}
protected void handleRefuse(ACLMessage refuse)
{
System.out.println("Agent "+refuse.getSender().getName()+ " refused to perform the requested action");
nResponders--;
}
protected void handleFailure(ACLMessage failure)
{
if (failure.getSender().equals(myAgent.getAMS()))
{
//FAILURE notification from the JADE runtime: the receiver
//does not exist
System.out.println("Responder does not exist");
}
else
{
System.out.println("Agent "+failure.getSender().getName()+ " failed to perform the requested action");
}
}
protected void handleAllResultNotifications(Vector notifications)
{
if (notifications.size() < nResponders)
{
// Some responder didn't reply within the specified timeout System.out.println("Timeout expired: missing "+(nResponders –
notifications.size())+" responses");
}
}
} );
}
else
{
System.out.println("No responder specified.");
}
}
}
//Файл maslabs.lab6.FIPARequestResponderAgent.java /*Агент, получающий запрос по протоколу FIPARequest*/ package maslabs.lab6;
import jade.core.Agent; import jade.core.AID;
import jade.lang.acl.ACLMessage; import jade.lang.acl.MessageTemplate; import jade.proto.AchieveREResponder; import jade.domain.FIPANames;
import jade.domain.FIPAAgentManagement.NotUnderstoodException; import jade.domain.FIPAAgentManagement.RefuseException;
import jade.domain.FIPAAgentManagement.FailureException;
public class FIPARequestResponderAgent extends Agent
{
protected void setup()
{
31
System.out.println("Agent "+getLocalName()+" waiting for requests..."); MessageTemplate template = MessageTemplate.and(
MessageTemplate.MatchProtocol(FIPANames.InteractionProtocol.FIPA_REQUEST), MessageTemplate.MatchPerformative(ACLMessage.REQUEST) );
addBehaviour(new AchieveREResponder(this, template)
{
protected ACLMessage prepareResponse(ACLMessage request) throws NotUnderstoodException, RefuseException
{
System.out.println("Agent "+getLocalName()+": REQUEST received from " +request.getSender().getName()+". Action is
"+request.getContent()); if (checkAction())
{
//We agree to perform the action. Note that in the FIPA-Request
//protocol the AGREE message is optional. Return null if you
//don't want to send it.
System.out.println("Agent "+getLocalName()+": Agree"); ACLMessage agree = request.createReply(); agree.setPerformative(ACLMessage.AGREE);
return agree;
}
else
{
// We refuse to perform the action System.out.println("Agent "+getLocalName()+": Refuse"); throw new RefuseException("check-failed");
}
}
protected ACLMessage prepareResultNotification(ACLMessage request, ACLMessage response) throws FailureException
{
if (performAction())
{
System.out.println("Agent "+getLocalName()+": Action successfully performed");
ACLMessage inform = request.createReply(); inform.setPerformative(ACLMessage.INFORM); return inform;
}
else
{
System.out.println("Agent "+getLocalName()+": Action failed"); throw new FailureException("unexpected-error");
}
}
} );
}
private boolean checkAction()
{
// Simulate a check by generating a random number return (Math.random() > 0.2);
}
private boolean performAction()
{
// Simulate action execution by generating a random number return (Math.random() > 0.2);
}
}
32
3. Листинги программ к Лабораторной работе № 7
//Файл maslabs.lab7.DFRegisterAgent.java
/*Агент-держатель курсов, выполняющий регистрацию своих сервисов */ package maslabs.lab7;
import jade.core.Agent; import jade.core.AID;
import jade.domain.DFService; import jade.domain.FIPAException; import jade.domain.FIPANames;
import jade.domain.FIPAAgentManagement.DFAgentDescription; import jade.domain.FIPAAgentManagement.ServiceDescription; import jade.domain.FIPAAgentManagement.Property;
public class DFRegisterAgent extends Agent
{
protected void setup()
{
// Register the service
System.out.println("Agent "+getLocalName()+" registering service "); try
{
DFAgentDescription dfd = new DFAgentDescription(); dfd.setName(getAID());
ServiceDescription sd = new ServiceDescription(); sd.setName("Agent1-Course-Facilitator"); sd.setType("Course-Facilitator");
sd.addProperties(new Property("course_name", "Multiagent system")); dfd.addServices(sd);
DFService.register(this, dfd);
}
catch (FIPAException fe)
{
fe.printStackTrace();
}
}
protected void takeDown()
{
// Deregister from the yellow pages try
{
DFService.deregister(this);
}
catch (FIPAException fe)
{
fe.printStackTrace();
}
//Close the GUI myGui.dispose();
//Printout a dismissal message
System.out.println("Course-Facilitator-agent" + getAID().getName()+ " terminating.");
}
}
//Файл maslabs.lab7.DFRegisterAgent.java
/*Агент, выполняющий поиск агентов, предоставляющих учебные курсы */
33
package maslabs.lab7; import jade.core.Agent; import jade.core.AID;
import jade.domain.DFService; import jade.domain.FIPAException; import jade.domain.FIPANames;
import jade.domain.FIPAAgentManagement.DFAgentDescription; import jade.domain.FIPAAgentManagement.ServiceDescription; import jade.domain.FIPAAgentManagement.SearchConstraints; import jade.util.leap.Iterator;
public class DFSearchAgent extends Agent
{
protected void setup()
{
// Search for services of type " Course-Facilitator " System.out.println("Agent "+getLocalName()+" searching for services of
type \"Course-Facilitator\"");
try
{
// Build the description used as template for the search DFAgentDescription template = new DFAgentDescription(); ServiceDescription templateSd = new ServiceDescription(); templateSd.setType("Course-Facilitator"); template.addServices(templateSd);
SearchConstraints sc = new SearchConstraints(); // We want to receive 10 results at most sc.setMaxResults(new Long(10));
DFAgentDescription[] results = DFService.search(this, template, sc); if (results.length > 0)
{
System.out.println("Agent "+getLocalName()+" found the following Course-Facilitator services:");
for (int i = 0; i < results.length; i++)
{
DFAgentDescription dfd = results[i]; AID provider = dfd.getName(); Iterator it = dfd.getAllServices(); while (it.hasNext())
{
ServiceDescription sd = (ServiceDescription) it.next(); if (sd.getType().equals("Course-Facilitator"))
{
System.out.println("- Service \""+sd.getName()+"\" provided by agent "+provider.getName());
}
}
}
}
else
{
System.out.println("Agent "+getLocalName()+" did not find any Course-Facilitator service");
}
}
catch (FIPAException fe)
{
fe.printStackTrace();
}
}
}
34
СОДЕРЖАНИЕ |
|
ПРЕДИСЛОВИЕ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
3 |
Лабораторная работа № 4. Протоколы взаимодействия и сложные |
|
типы поведения агентов . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
3 |
4.1. Теоретические сведения . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
4 |
4.2. Порядок выполнения работы . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
4 |
4.3. Содержание отчета . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
5 |
4.4. Вопросы для самоконтроля . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
6 |
Лабораторная работа № 5. Программирование пользовательских |
|
онтологий в JADE. Язык содержания FIPA-SL0 . . . . . . . . . . . . . . . . . . |
6 |
5.1. Теоретические сведения . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
6 |
5.2. Порядок выполнения работы . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
11 |
5.3. Содержание отчета . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
14 |
5.4. Вопросы для самоконтроля . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
14 |
Лабораторная работа № 6. Использование стандартизованных |
|
протоколов взаимодействия агентов . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
14 |
6.1. Теоретические сведения . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
14 |
6.2. Порядок выполнения работы . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
21 |
6.3. Содержание отчета . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
21 |
6.4. Вопросы для самоконтроля . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
21 |
Лабораторная работа № 7. Работа с сервисом «желтых страниц» DF |
21 |
7.1. Теоретические сведения . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
21 |
7.2. Порядок выполнения работы . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
23 |
7.3. Содержание отчета . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
23 |
7.4. Вопросы для самоконтроля . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
24 |
СПИСОК ЛИТЕРАТУРЫ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
24 |
ПРИЛОЖЕНИЯ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
25 |
1. Листинги программ к Лабораторной работе № 5 . . . . . . . . . . . . . . . . |
25 |
2. Листинги программ к Лабораторной работе № 6 . . . . . . . . . . . . . . . . |
30 |
3. Листинги программ к Лабораторной работе № 7 . . . . . . . . . . . . . . . . |
33 |
35
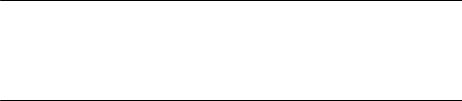
Жандаров Владимир Владимирович, Пантелеев Михаил Георгиевич
Построение мультиагентных систем в среде JADE
Учебно-методическое пособие
Редактор Н. В. Кузнецова
Подписано в печать 25.06.18. Формат 60×84 1/16. Бумага офсетная. Печать цифровая. Печ. л. 2,25.
Гарнитура «Times New Roman». Тираж 44 экз. Заказ 109.
Издательство СПбГЭТУ «ЛЭТИ» 197376, С.-Петербург, ул. Проф. Попова, 5
36