
Mastering Enterprise JavaBeans™ and the Java 2 Platform, Enterprise Edition - Roman E
..pdf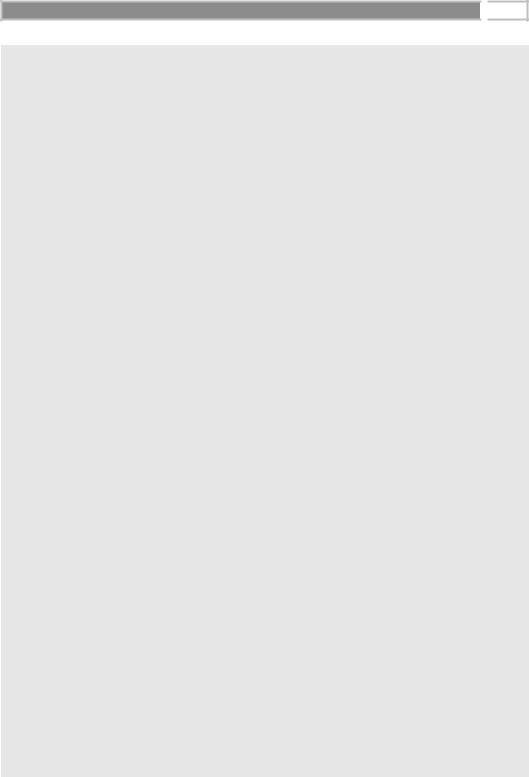
J2EE in the Real World: Combining Servlets with Enterprise JavaBeans 471
package com.wiley.compBooks.ecommerce;
import java.io.*; import java.util.*; import javax.servlet.*; import javax.naming.*;
/**
*This servlet stores the list of products that the
*user can purchase.
*
*This Generic Servlet is a helper servlet called by
*other servlets. There is no user interface logic
*in this servlet because this servlet is never called
*directly from clients.
*/
public class ProductBaseServlet extends GenericServlet {
// Vector of Product EJB Objects private Vector products;
/**
*The servlet engine calls this method once to
*initialize a servlet instance.
*
*In the body of this method, we need to acquire the
*EJB Product Home Object. We then acquire all the
*products.
*/
public void init(ServletConfig config) throws ServletException {
super.init(config);
try { /*
*Get the deployed properties from the
*config object. We externalize the JNDI
*initialization params to the servlet
*properties file to allow end users to
*dynamically reconfigure their environment
*without recompilation.
*/
String initCtxFactory = getInitParameter(Context.INITIAL_CONTEXT_FACTORY);
String providerURL = getInitParameter(Context.PROVIDER_URL);
/*
* Add the JNDI init parameters to a
Source 15.4 ProductBaseServlet.java (continues).
Go back to the first page for a quick link to buy this book online!
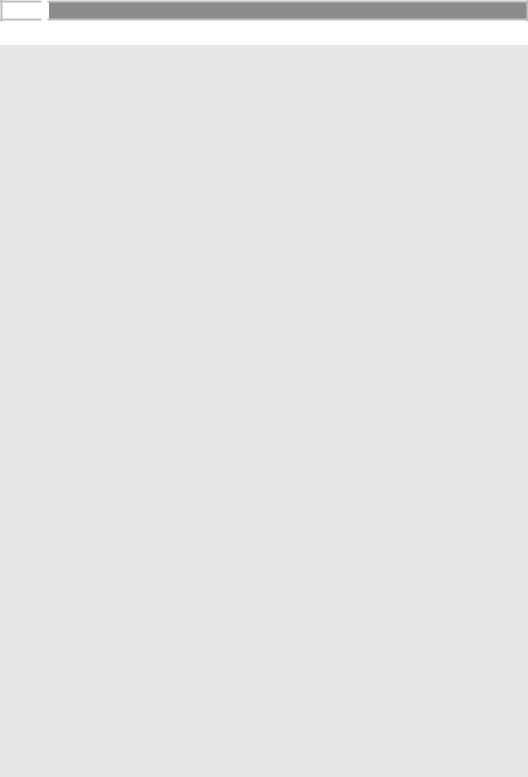
472 M A S T E R I N G E N T E R P R I S E J A V A B E A N S
* properties object. */
Properties env = new Properties(); env.put(Context.INITIAL_CONTEXT_FACTORY, initCtxFactory); env.put(Context.PROVIDER_URL, providerURL);
/*
*Get the initial JNDI context using the
*above startup params.
*/
Context ctx = new InitialContext(env);
/*
* Look up the Product Home Object. */
ProductHome productHome = (ProductHome) ctx.lookup("Ecommerce.ProductHome");
/*
* Get all the products. */
Enumeration e = productHome.findAllProducts(); this.products = new Vector();
while (e.hasMoreElements()) { this.products.addElement(e.nextElement());
}
}
catch (Exception e) { e.printStackTrace();
}
}
public void service(ServletRequest req, ServletResponse res) throws ServletException, IOException {
throw new UnavailableException(this,
"This servlet does not accept client requests.");
}
/**
*Retrieves a product based upon it's product id.
*Called by other servlets.
*@return null if no match.
*/
public Product getProduct(String productID) throws Exception { ProductPK pk = new ProductPK(productID);
for (int i=0; i < products.size(); i++) {
Source 15.4 ProductBaseServlet.java (continues).
Go back to the first page for a quick link to buy this book online!

J2EE in the Real World: Combining Servlets with Enterprise JavaBeans 473
Product prod = (Product) products.elementAt(i); if (prod.getPrimaryKey().equals(pk)) {
return prod;
}
}
return null;
}
public Vector getAllProducts() { return products;
}
public int getNumberOfProducts() { return products.size();
}
public String getServletInfo() {
return "The ProductBase servlet contains all the products." + "It is called by other servlets, not directly by a user.";
}
}
Source 15.4 ProductBaseServlet.java (continued).
all the Products via the findAllProducts() custom finder method on the Product home interface.
Other servlets can access the products available via the getAllProducts(), getNumberOfProducts(), and getProduct() methods. If an HTTP client attempts to connect to our servlet, our service() method will throw an exception—thin clients should never be calling this servlet directly.
The Product Detail Servlet
The Product Detail servlet (ProductDetailServlet.java) is responsible for displaying the page to the user enumerating the low-level details of a particular Product. The user can add a Product to his or her Quote from here as well. The code is shown in Source 15.5.
Our Product Detail servlet always expects a URL parameter passed to it—the parameter is the particular product ID that should be detailed. It then retrieves the Product EJB object corresponding to that ID by calling the Product Base servlet. Finally, it prints out the Product’s details by calling the get methods on the Product. The user can add this Product to his or her Quote as well—if so, the user will be redirected back to the Catalog.
Go back to the first page for a quick link to buy this book online!
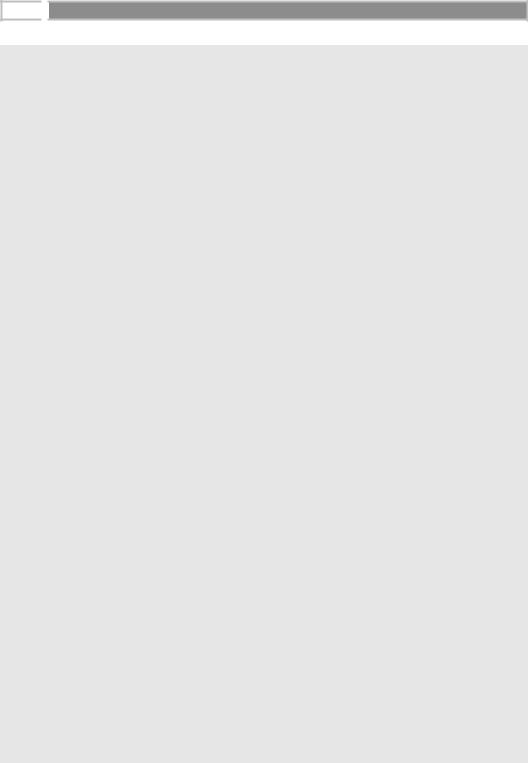
474 M A S T E R I N G E N T E R P R I S E J A V A B E A N S
package com.wiley.compBooks.ecommerce;
import java.io.*; import javax.servlet.*;
import javax.servlet.http.*;
/**
* This servlet displays detailed information about a
*particular product in the catalog.
*By the time this servlet is called, the user has
*logged in (via the Login servlet) and has a shopping
*cart started (i.e., Quote bean). Because this servlet is
*pooled and reused for different user requests, the
*servlet code does not store any information specific
*to any user. Rather, we store a reference to the
*user's Quote in the user's HttpSession object, which
*is globally accessible to all servlets.
*/
public class ProductDetailServlet extends HttpServlet {
/**
*The servlet engine calls this method when the user's
*desktop browser sends an HTTP GET request.
*/
public void doGet (HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
/*
*Get the user's HttpSession, and from that get
*the user's current quote.
*/ try {
HttpSession session = request.getSession(false);
Quote quote = (Quote) session.getValue(session.getId());
}
catch (Exception e) {
throw new ServletException(
"Error: You don't have a current quote going. Re-login.");
}
/*
*Now, we write the response. Set content-type
*header, indicating that we will be writing in HTML.
*/
response.setContentType("text/html"); PrintWriter out = response.getWriter();
Source 15.5 ProductDetailServlet.java (continues).
Go back to the first page for a quick link to buy this book online!

J2EE in the Real World: Combining Servlets with Enterprise JavaBeans 475
/*
* Then write the response. Start with the header. */
out.println( "<html>" +
"<head><title> Computer Catalog </title></head>" +
"<body bgcolor=\"#ffffff\">" + "<center>" +
"<hr> <br> " + "<h1>" +
"<font size=\"+3\" color=\"red\">Jasmine's </font>" + "<font size=\"+3\" color=\"purple\">Computer </font>" + "<font size=\"+3\" color=\"green\">Parts</font>" + "</h1>" +
"</center>" +
"<br> <hr> <br> ");
/*
*Get our ProductBase Servlet which contains all
*the available products.
*/
ProductBaseServlet productBase = (ProductBaseServlet) getServletConfig().getServletContext().getServlet("productbase");
/*
*To print out the product information, we first
*must figure out which product to show. We
*assume that the product ID has been passed to us
*as a URL parameter, called "productID".
*/
String productID = request.getParameter("productID"); if (productID != null) {
/*
*Retrieve the product from the ProductBase
*servlet, keyed on product ID.
*/
Product product = null; try {
product = productBase.getProduct(productID);
}
catch (Exception e) {
throw new ServletException(e.toString());
}
Source 15.5 ProductDetailServlet.java (continues).
Go back to the first page for a quick link to buy this book online!
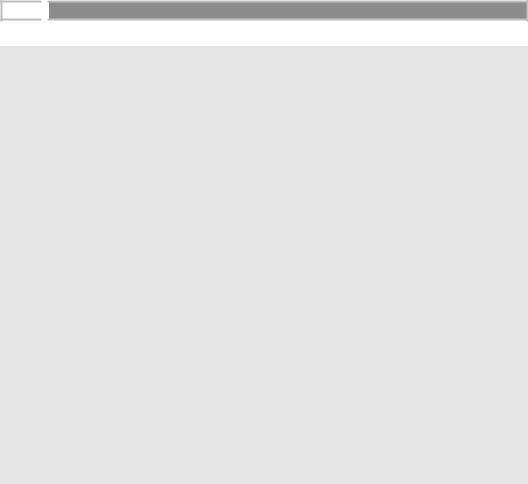
476 M A S T E R I N G E N T E R P R I S E J A V A B E A N S
/*
* Print out the information obtained */
out.println(
"<h2>" + product.getName() + "</h2>" +
"<h4>Description: </h4>" + product.getDescription() +
"<h4>Base price (before discounts): $" + product.getBasePrice() + "</h4>" +
"<center>" + "<p><a href=\"" +
response.encodeUrl("/servlet/catalog?Buy=" + productID) +
"\"> Add this item to your quote.</a></p>" + "</center>");
}
out.println("</body></html>");
out.close();
}
public String getServletInfo() {
return "The ProductDetail servlet returns information about" + "any product that is available from the catalog.";
}
}
Source 15.5 ProductDetailServlet.java (continued).
The Quote Viewing Servlet
The user is able to view and modify his or her Quote at any time by going to a separate page, manifested by the Quote View servlet in ShowQuoteServlet.java. The user can view each individual Quote Line Item stateful session bean that constitutes the Quote. The user can also change the desired quantities of the selected Products. Every time the user changes something, our Quote View servlet recalculates the price of the Quote by calling the Pricer stateless session bean. The code is rather involved, and it is shown in Source 15.6.
Let’s see exactly what this code is doing.
The init() method grabs a Pricer EJB object for performing pricing operations. It does this via JNDI, calling create() on the Pricer’s home object.
Go back to the first page for a quick link to buy this book online!
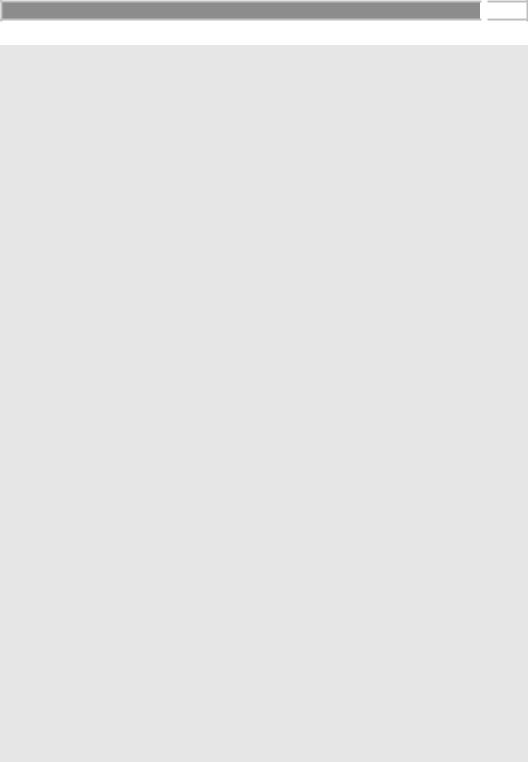
J2EE in the Real World: Combining Servlets with Enterprise JavaBeans 477
package com.wiley.compBooks.ecommerce;
import java.io.*; import java.util.*; import java.text.*; import javax.servlet.*;
import javax.servlet.http.*; import javax.naming.*;
/**
*This servlet allows the user to view and modify his or her
*current selections.
*
*By the time this servlet is called, the user has
*logged in (via the Login servlet) and has a shopping
*cart started (i.e., Quote bean). Because this servlet is
*pooled and reused for different user requests, the
*servlet code does not store any information specific
*to any user. Rather, we store a reference to the user's
*Quote in the user's HttpSession object, which is globally
*accessible to all servlets.
*/
public class ShowQuoteServlet extends HttpServlet {
// Pricer EJB Object for pricing the quote private Pricer pricer;
/**
*The servlet engine calls this method once to initialize
*a servlet instance.
*
*In the body of this method, we need to acquire a Pricer
*EJB Object for pricing the quotes.
*/
public void init(ServletConfig config) throws ServletException {
/*
*Call parent to store the config object, so that
*getServletConfig() can return it.
*/
super.init(config);
try { /*
*Get the JNDI initialization parameters.
*We externalize these settings to the
*servlet properties file to allow end-users
Source 15.6 ShowQuoteServlet.java (continues).
Go back to the first page for a quick link to buy this book online!
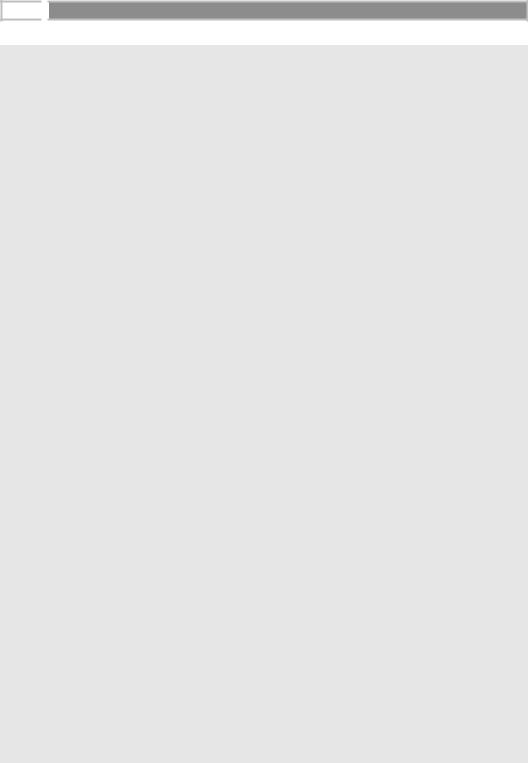
478 M A S T E R I N G E N T E R P R I S E J A V A B E A N S
*to dynamically reconfigure their
*environment without recompilation.
*/
String initCtxFactory = getInitParameter(Context.INITIAL_CONTEXT_FACTORY);
String providerURL = getInitParameter(Context.PROVIDER_URL);
/*
*Add the JNDI init parameters to a
*properties object.
*/
Properties env = new Properties(); env.put(Context.INITIAL_CONTEXT_FACTORY, initCtxFactory); env.put(Context.PROVIDER_URL, providerURL);
/*
*Get the initial context using the above
*startup params.
*/
Context ctx = new InitialContext(env);
/*
* Look up the Home Object. */
PricerHome pricerHome = (PricerHome) ctx.lookup("Ecommerce.PricerHome");
/*
* Create a Pricer EJB Object. */
pricer = pricerHome.create();
}
catch (Exception e) { log(e);
throw new ServletException(e.toString());
}
}
/**
*The servlet engine calls this method when the user's
*desktop browser sends an HTTP GET request.
*/
public void doGet (HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
/*
* Get the user's HttpSession, and from that get
Source 15.6 ShowQuoteServlet.java (continues).
Go back to the first page for a quick link to buy this book online!

J2EE in the Real World: Combining Servlets with Enterprise JavaBeans 479
* the user's current quote. */
HttpSession session = request.getSession(false); if (session == null) {
/*
* Redirect user to login page if no session */
response.sendRedirect(response.encodeUrl("/servlet/login"));
return;
}
Object obj = session.getValue("quote"); if (obj == null) {
/*
* Redirect user to login page if no session */
response.sendRedirect(response.encodeUrl("/servlet/login"));
return;
}
Quote quote = (Quote) obj;
/*
*If the user clicked the 'Order' button, he
*wants to purchase his selections. We forward
*the user to the servlet that handles purchasing.
*/
if (request.getParameter("Order") != null) { response.sendRedirect(response.encodeUrl("/servlet/purchase")); return;
}
/*
*Now, we write the response. Set content-type
*header, indicating that we will be writing in
*HTML.
*/
response.setContentType("text/html"); PrintWriter out = response.getWriter();
/*
*Then write the data of the response.
*Start with the header.
*/
out.println( "<html>" +
"<head><title> Computer Catalog </title></head>" +
"<body bgcolor=\"#ffffff\">" +
Source 15.6 ShowQuoteServlet.java (continues).
Go back to the first page for a quick link to buy this book online!

480 M A S T E R I N G E N T E R P R I S E J A V A B E A N S
"<center>" +
"<hr> <br> " + "<h1>" +
"<font size=\"+3\" color=\"red\">Jasmine's </font>" + "<font size=\"+3\" color=\"purple\">Computer </font>" + "<font size=\"+3\" color=\"green\">Parts</font>" + "</h1>" +
"</center>" +
"<br> <hr> <br> ");
/*
*Get our ProductBase Servlet which contains
*all the available products.
*/
ProductBaseServlet productBase = (ProductBaseServlet) getServletConfig().getServletContext().getServlet("productbase");
/*
*Next, we need to figure out what button the user
*clicked, if any. These come to us as form
*parameters. We need to loop through each
*parameter and interpret it.
*/
Enumeration paramNames = request.getParameterNames(); while (paramNames.hasMoreElements()) {
String paramName = (String) paramNames.nextElement();
String paramValue = request.getParameter(paramName);
/*
*If user clicked 'Update' button, then the user
*wants to change the quantities of each product
*he is ordering. We'll process those quantities
*below.
*/
if (paramName.equals("Update")) {
}
/*
* If the user wants to clear the form */
else if (paramName.equals("Clear")) {
quote.clear();
out.println(
"<font color=\"#ff0000\" size=\"+2\">" + "<strong>You just cleared your quote!" + "</strong> <br> <br> </font>");
Source 15.6 ShowQuoteServlet.java (continues).
Go back to the first page for a quick link to buy this book online!